1.截屏
1.1 手动截屏,通过其他第三方软件发送截图,或者从手机取出截图
1.2 使用脚本截图,将截图保存到手机,再拉取到电脑
脚本有2个参数:
- 第一个参数为图片名,默认年月日-时分秒
- 第二个参数为图片保存路径,限定当前位置和固定目录,避免文件乱放
#!/bin/sh
#运行 sh screenshot name
picName=$1
filePath=$2
#定义name
if [ ! ${picName} ];then
picName=`date +"%F_%H%M%S"`
fi
#定义path
if [[ ${filePath} == '.' ]];then
filePath=`pwd`
else
filePath='/Users/chenshanju/Desktop/caps'
if [ ! -d ${filePath} ];then
mkdir ${filePath}
fi
fi
#截图
for i in `adb devices|grep -w 'device'|awk '{print $1}'`;do
adb -s $i shell /system/bin/screencap -p /sdcard/${i}-${picName}.png
adb -s $i pull /sdcard/${i}-${picName}.png ${filePath}
adb -s $i shell rm -rf /sdcard/$i-${picName}.png
done
执行效果:
如果使用tcp-ip指定设备,发送给开发时提示文件错误,因此将脚本修改为使用ro.product.model代替device
#!/bin/sh
#运行 sh screenshot name
picName=$1
filePath=$2
#定义name
if [ ! ${picName} ];then
picName=`date +"%F_%H%M%S"`
fi
#定义path
if [[ ${filePath} == '.' ]];then
filePath=`pwd`
else
filePath='/Users/chenshanju/Desktop/caps'
if [ ! -d ${filePath} ];then
mkdir ${filePath}
fi
fi
#截图
for i in `adb devices|grep -w 'device'|awk '{print $1}'`;do
#修改name,将其设置为model,不再使用devices
#写个for循环,观测下截图传入model和pull时使用model的耗时
deviceName=`adb -s $i shell getprop|grep ro.product.model|awk -F ':' '{print $2}'|awk -F '[' '{print $2}'|awk -F ']' '{print $1}'`
deviceName=${deviceName// /}
adb -s $i shell /system/bin/screencap -p /sdcard/${deviceName}-${picName}.png
adb -s $i pull /sdcard/${deviceName}-${picName}.png ${filePath}
adb -s $i shell rm -rf /sdcard/${deviceName}-${picName}.png
done
写个for循环,查看这2中情况下的耗时,没有差别。
#!/bin/sh
start=`date +%s`
for i in `seq 1 10`;do
sh /Users/chenshanju/sh_script/screenshot.sh 1 .
done
end=`date +%s`
mi=$(( ${end} - ${start} ))
echo ${mi}
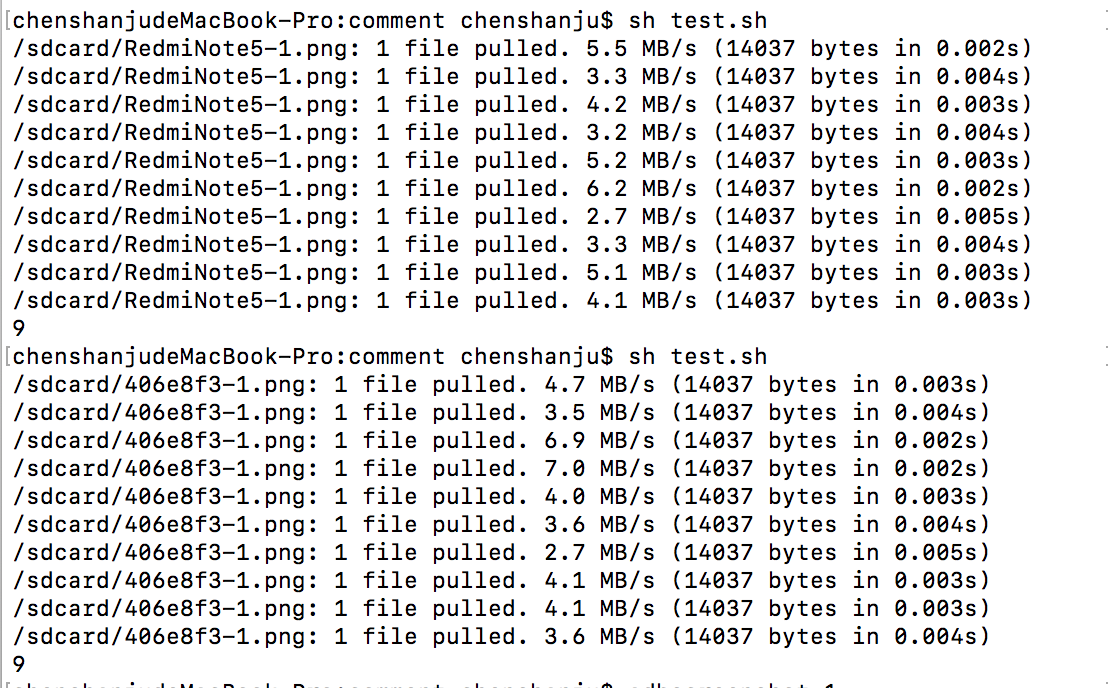
1.3 直接保存到手机
adb shell screencap -p | sed 's/
$//' > screen.png
Mac执行提示
sed: RE error: illegal byte sequence
参考别人的博客 但截屏不全。
adb shell /system/bin/screencap -p |LC_CTYPE=C sed 's/
$//' > screen.png
1.4 Windows电脑专用
对bat语法不熟,只能借助同事的电脑调试,还得等她有时间才可以。这是我写得最费劲的脚本,凑合能用
:适用于Windows脚本安卓手机截屏
adb shell /system/bin/screencap -p /sdcard/test.png
set date0=%date:~0,10%
set time0=%time:~0,8%
set dttm=%date0:/=%%time0::=%
adb pull /sdcard/test.png caps/%dttm%.png
2 录屏
注意:录屏脚本针对单台设备,如果需要多台同时执行,请百度shell的多线程。
脚本传入4个参数
- 第一个参数:时长,最大180秒
- 第二个参数:路径,支持当前路径和固定路径
- 第三个参数:保存的文件名称,默认年月日-时分秒
- 第四个参数:设备,多台手机需要指定,单台可忽略
#!/bin/sh
videotime=$1
path=$2
filename=$3
device=$4
#定义path
if [[ ${path} == '.' ]];then
path=`pwd`
else
path='/Users/chenshanju/Desktop/caps'
if [ ! -d ${path} ];then
mkdir ${path}
fi
fi
#定义文件名
if [ ! -z $3 ];then
filename=$3
else
filename=`date +"%F_%H%M%S"`
fi
#指定设备
if [ -z ${device} ];then5
if [ ! -z ${videotime} ];then
adb shell screenrecord --time-limit ${videotime} /sdcard/${filename}.mp4
else
adb shell screenrecord /sdcard/${filename}.mp4
fi
adb pull /sdcard/${filename}.mp4 ${filePath}
adb shell rm /sdcard/${filename}.mp4
else
if [ ! -z ${videotime} ];then
adb -s ${device} shell screenrecord --time-limit ${videotime} /sdcard/${filename}.mp4
else
adb -s ${device} shell screenrecord /sdcard/${filename}.mp4
fi
adb -s ${device} pull /sdcard/${filename}.mp4 ${filePath}
adb -s ${device} shell rm /sdcard/${filename}.mp4
fi
执行效果:
3.输入
#!/bin/sh
a=$1
if [ ! $a ];then
a='123456'
fi
for i in `adb devices|grep -w device|awk '{print $1}'`;do
adb -s $i shell input text ${a}
done
4.获取机型信息
#!/bin/sh
#获取手机品牌、机型、分辨率、安卓版本
#定义path
echo `date`
path=$1
if [[ ${path} == '.' ]];then
path=`pwd`
else
path='/Users/chenshanju/Desktop/caps'
if [ ! -d ${path} ];then
mkdir ${path}
fi
fi
for i in `adb devices|grep -w device|awk '{print $1}'`;do
echo "手机品牌:" `adb -s ${i} shell getprop|grep "ro.product.brand"|awk -F ':' '{print $2}'` > ${path}/${i}.txt
echo "手机机型" `adb -s ${i} shell getprop|grep "ro.product.model"|awk -F ':' '{print $2}'` >> ${path}/${i}.txt
echo "手机系统" `adb -s ${i} shell getprop|grep "build.version.release"|awk -F ':' '{print $2}'` >> ${path}/${i}.txt
echo "屏幕分辨率:" `adb -s ${i} shell wm size| awk -F ':' '{print $2}'` >> ${path}/${i}.txt
echo ""
done
echo `date`
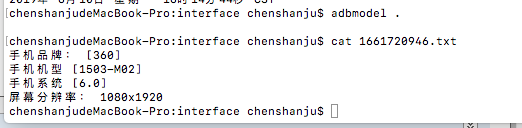
5.修改.bash_profile文件,每次可以直接输入名称执行脚本
alias adbscreenshot=/Users/csj/sh_script/screenshot.sh
alias adbrecordscreen=/Users/csj/sh_script/recordscreen.sh
alias adbmodel=/Users/csj/sh_script/model.sh
alias adbinput=/Users/csj/sh_script/input.sh
6.adb改造
#!/bin/bash
PLATFORM_TOOL="/usr/local/Libary/Android/android-sdk/PLATFORM_TOOL/adb"
echo "$@" >> /tmp/adb.log #会将执行的命令追加到/tmp/adb.log中
问题:
1.登陆页面截屏失败
原因:应用出于安全性考虑,会禁止在包含密码的页面进行截屏操作。