1.异常测试
对可能抛出的异常进行测试:
- 异常本身是方法签名的一部分:
* public static int parseInt(String s) throws NumberFormatException - 测试错误的输入是否导致特定的异常:
* Integer.parseInt(null)
* Integer.parseInt("")
* Integer.parseInt("xyz")
2.异常测试的方法:
- 使用try..catch (Exception e)捕获异常,但这样会编写许多try..catch代码
- expected测试异常,推荐
2.1示例1
package com.testList;
import org.junit.*;
import static org.junit.Assert.fail;
public class SequenceTest {
//使用try...catch
@Test
public void test1() throws Exception{
try {
Integer.parseInt(null);
fail("should throw java.lang.NumberFormatException");
}catch (NumberFormatException e){
System.out.println("pass");
}
}
//使用expected捕获异常
@Test(expected = NumberFormatException.class)
public void test2() throws Exception{
Integer.parseInt("p");
}
}
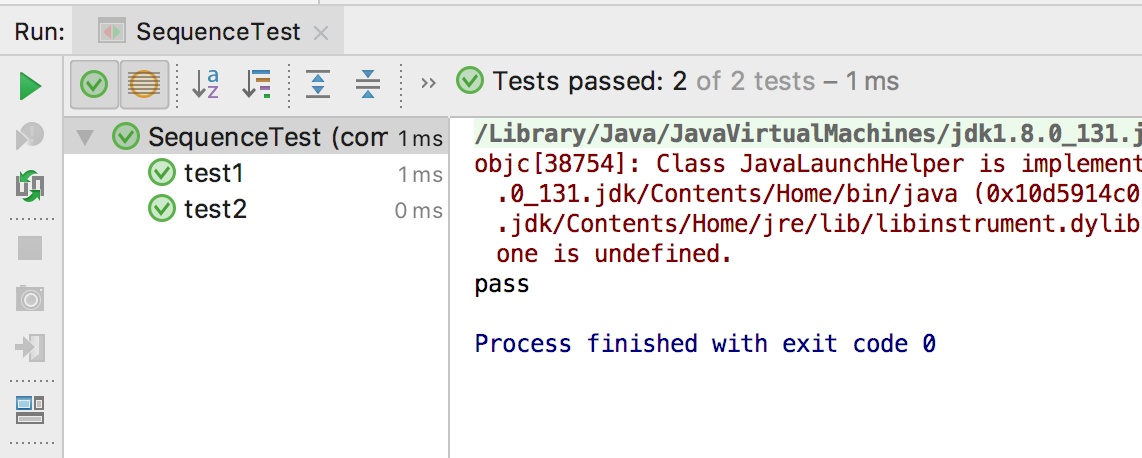
public class Calculator {
public int calculator(String expression){
if (expression == null){
throw new NumberFormatException();
}
String[] ss = expression.split("+");
int sum = 0;
for(String s:ss){
sum = sum + Integer.parseInt(s.trim());
}
return sum;
}
}
CalculatorTest.java
```#java
package com.testList;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import java.util.Calendar;
import static org.junit.Assert.*;
public class CalculatorTest {
Calculator calc;
@Before
public void setUp(){
calc = new Calculator();
}
@Test(expected = NumberFormatException.class)
public void testCalcWithEmptyString(){
int r = calc.calculator("");
}
@Test(expected =NumberFormatException.class)
public void testCalcAdd3Numbers(){
int r = calc.calculator(null);
}
@After
public void tearDown(){
calc = null;
}
}
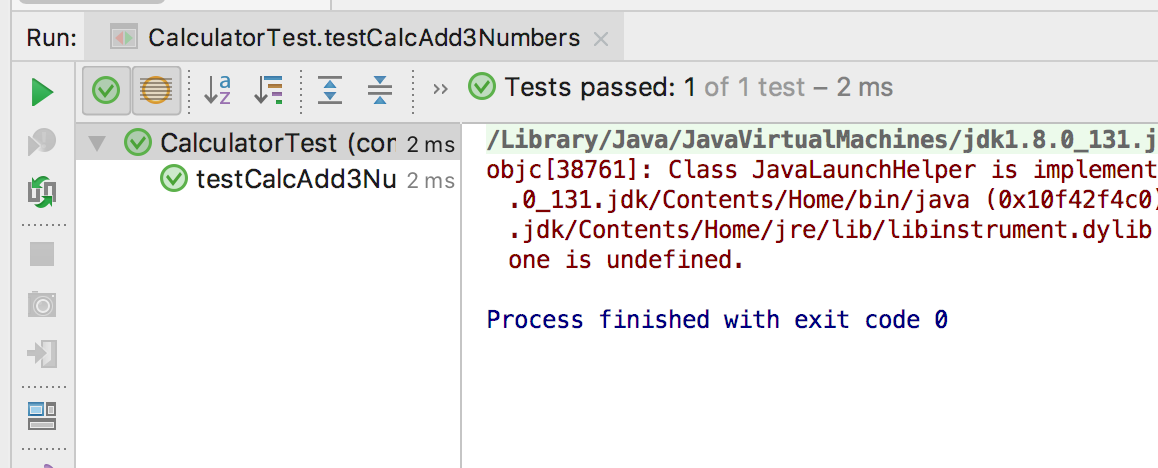
3.总结
- 测试异常可以使用@Test(expected = Exception.class)
- 对可能发生的每种类型的异常进行测试