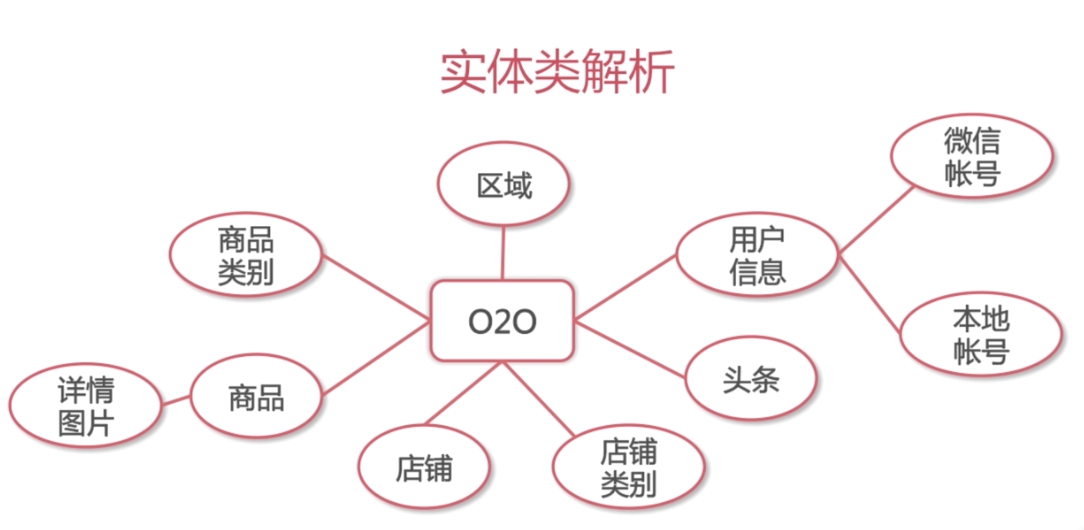
1. 区域
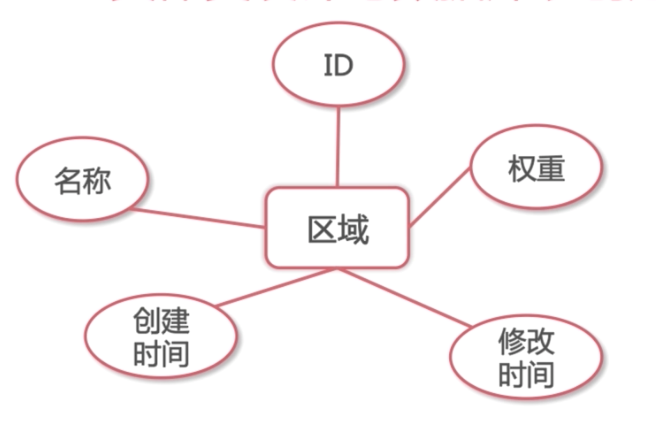
权重:显示优先级。权重大的,优先展示
1.1 创建Area类
在src/main/java下新建package名称为com.csj2018.o2o.entity,新建Area类
package com.csj2018.o2o.entity;
import java.util.Date;
public class Area {
private Integer areaId;//ID
private String areaName;//名称
private Integer priority;//权重
private Date createTime;//创建时间
private Date lastEditTime;//更新时间
//省略setter和getter方法
}
1.2 建立tb_area表
docker exec -it csjmysql bash
mysql -u root -p
create database if not exists o2o default character set = 'utf8';
drop table if EXISTS `tb_area`;
create table `tb_area` (
`area_id` int(2) NOT NULL auto_increment,
`area_name` varchar(200) NOT NULL,
`priority` int(2) NOT NULL DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
PRIMARY key(`area_id`),
UNIQUE key `UK_AREA`(`area_name`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
select * from tb_area;
2. 用户信息表
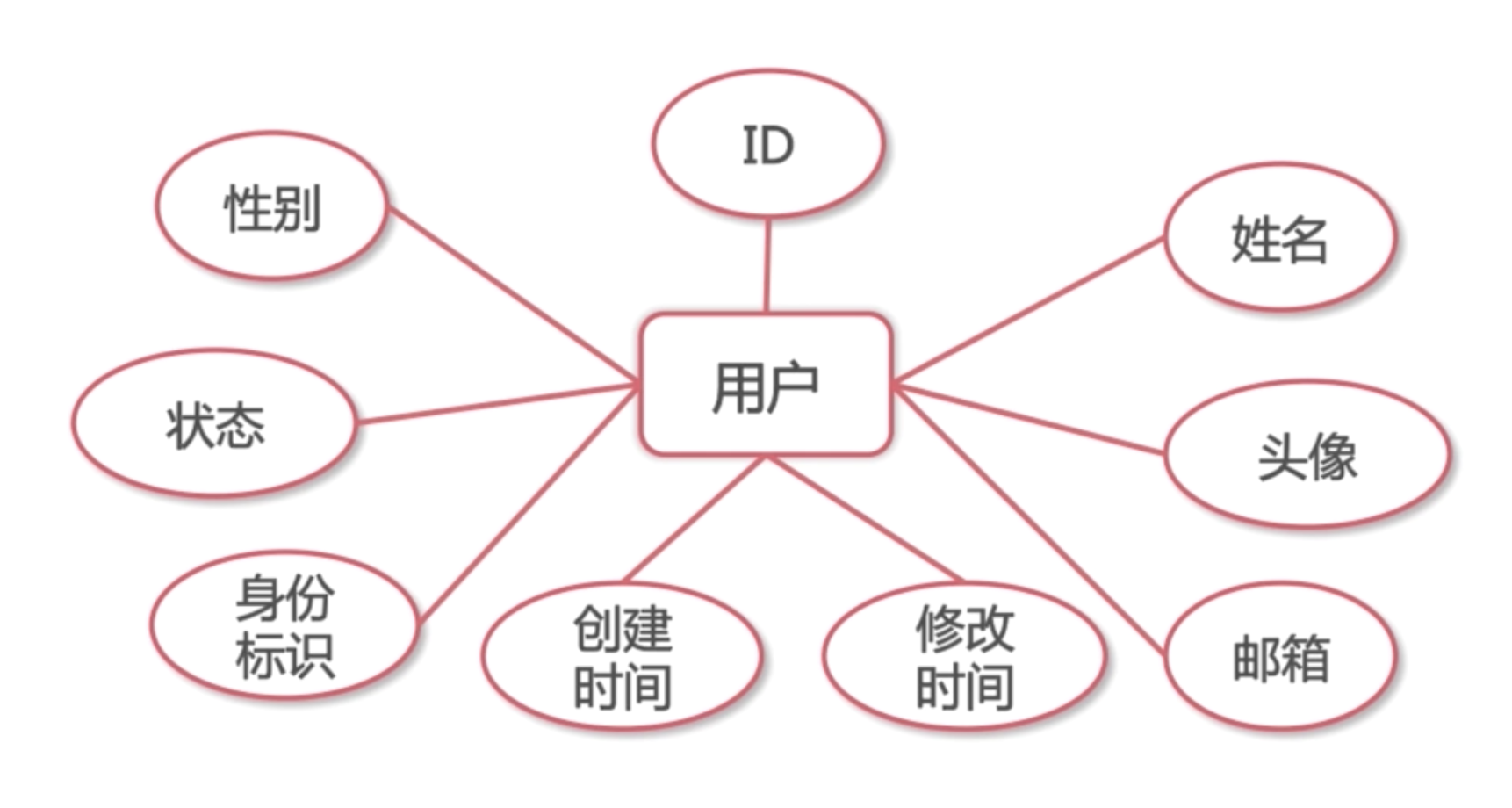
2.1 创建PersonInfo类
package com.csj2018.o2o.entity;
import java.util.Date;
public class PersonInfo {
private Long userId;
private String name;
private String profileImg;
private String email;
private String gender;
private Integer enableStatus;
//1顾客 2店家 3超级管理员
private Integer userType;
private Date createTime;
private Date lastEditTime;
//省略setter和getter方法
}
2.2 创建用户信息表
drop table if EXISTS `tb_person_info`;
create table `tb_person_info` (
`user_id` int(10) NOT NULL auto_increment,
`name` varchar(32) default NULL,
`profile_img` varchar(1024) DEFAULT NULL,
`email` varchar(1024) DEFAULT NULL,
`gender` varchar(2) DEFAULT NULL,
`enable_status` int(2) NOT NULL DEFAULT '0' COMMENT '0:禁止使用本商城,1:允许使用本商城',
`user_type` int(2) NOT NULL DEFAULT '1' COMMENT '1:顾客,2:店家,3:超级管理员',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
PRIMARY KEY (`user_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
select * from tb_person_info;
3.微信账号和本地用户
微信账号和本地账号通过用户ID与用户信息表关联,
3.1 创建微信账号和本地用户类
package com.csj2018.o2o.entity;
import java.util.Date;
public class WechatAuth {
private Long wechatAuthId;
private String openId;
private Date createTime;
private PersonInfo personInfo;
//省略setter和getter方法
}
package com.csj2018.o2o.entity;
import java.util.Date;
public class LocalAuth {
private Long localAuthId;
private String username;
private String password;
private Date createTime;
private Date lastEditTime;
private PersonInfo personInfo;
//省略setter和getter方法
}
3.2 创建微信账号和本地用户表
drop table if EXISTS `tb_wechat_auth`;
create table `tb_wechat_auth` (
`wechat_auth_id` int(10) NOT NULL auto_increment,
`user_id` int(10) NOT NULL,
`open_id` varchar(1024) NOT NULL,
`create_time` datetime DEFAULT NULL,
PRIMARY KEY(`wechat_auth_id`),
CONSTRAINT `fk_wechatauth_profile` FOREIGN KEY(`user_id`) REFERENCES `tb_person_info`(`user_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
alter table tb_wechat_auth add UNIQUE INDEX(`open_id`);
select * from tb_wechat_auth;
drop table if EXISTS `tb_local_auth`;
create table `tb_local_auth`(
`local_auth_id` int(10) NOT NULL auto_increment,
`user_id` int(10) NOT NULL,
`username` varchar(128) NOT NULL,
`password` varchar(128) NOT NULL,
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
PRIMARY KEY(`local_auth_id`),
UNIQUE KEY `uk_local_profile`(`username`),
CONSTRAINT `fk_localauth_profile` FOREIGN KEY(`user_id`) REFERENCES `tb_person_info`(`user_id`)
)ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
select * from tb_local_auth;
4. 头条
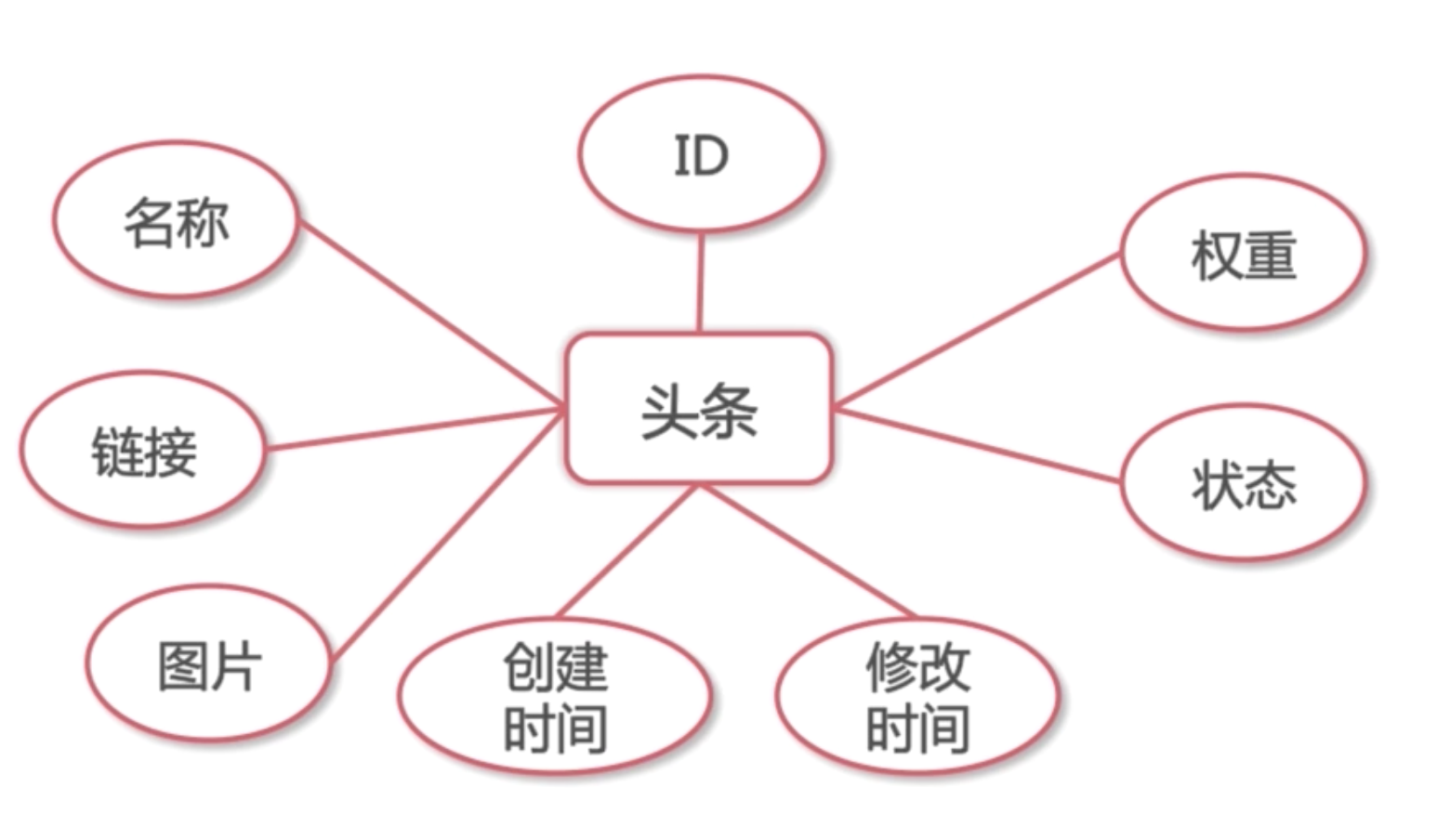
4.1 创建头条类
package com.csj2018.o2o.entity;
import java.util.Date;
public class HeadLine {
private Long lineId;
private String lineName;
private String lineLink;
private String lineImg;
private Integer priority;
//0不可用 1可用
private Integer enableStatus;
private Date createTime;
private Date lastEditTime;
//省略setter和getter方法
}
4.2 创建头条广告
drop table if EXISTS `tb_head_line`;
create table `tb_head_line` (
`line_id` int(100) NOT NULL auto_increment,
`line_name` varchar(1000) DEFAULT NULL,
`line_link` varchar(2000) NOT NULL,
`line_img` varchar(2000) NOT NULL,
`priority` int(2) NOT NULL DEFAULT '0',
`enable_status` int(2) DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
PRIMARY KEY(`line_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
select * from tb_head_line;
5. 店铺类别
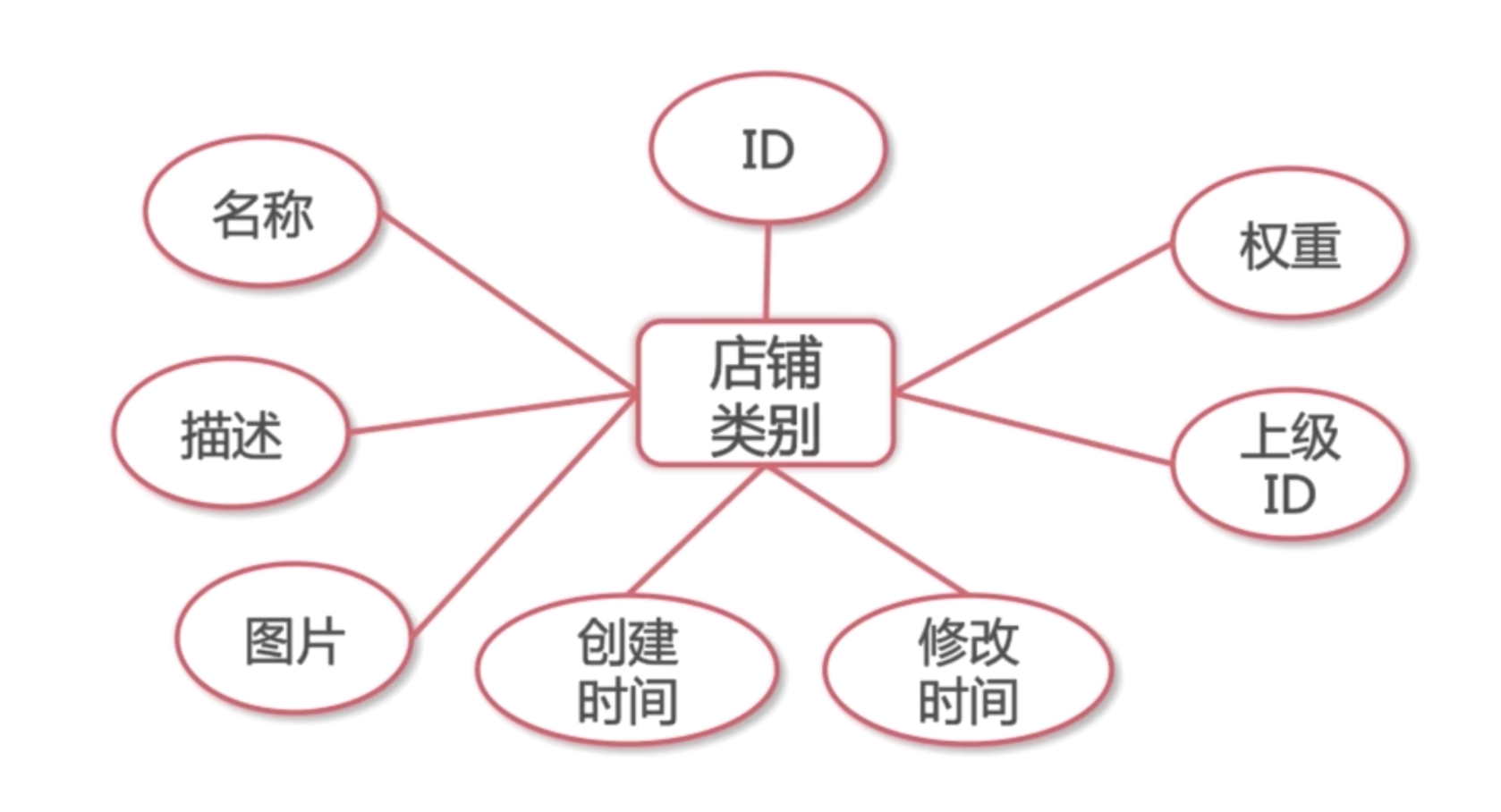
5.1 创建店铺类别类
package com.csj2018.o2o.entity;
import java.util.Date;
public class ShopCategory {
private Long shopCategoryId;
private String shopCategoryName;
private String shopCategoryDesc;
private String shopCategoryImg;
private Integer priority;
private Date createTime;
private Date lastEditTime;
private ShopCategory parent;
//省略setter和getter方法
}
5.2 创建店铺类别表
drop table if EXISTS `tb_shop_category`;
create table `tb_shop_category` (
`shop_category_id` int(11) NOT NULL auto_increment,
`shop_category_name` varchar(100) NOT NULL DEFAULT '',
`shop_category_desc` varchar(1000) DEFAULT '',
`shop_category_img` varchar(2000) DEFAULT NULL,
`priority` int(2) NOT NULL DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
`parent_id` int(11) DEFAULT NULL,
PRIMARY KEY(`shop_category_id`),
CONSTRAINT `fk_shop_category_self` FOREIGN KEY(`parent_id`) REFERENCES `tb_shop_category`(`shop_category_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
select * from tb_shop_category;
6. 店铺
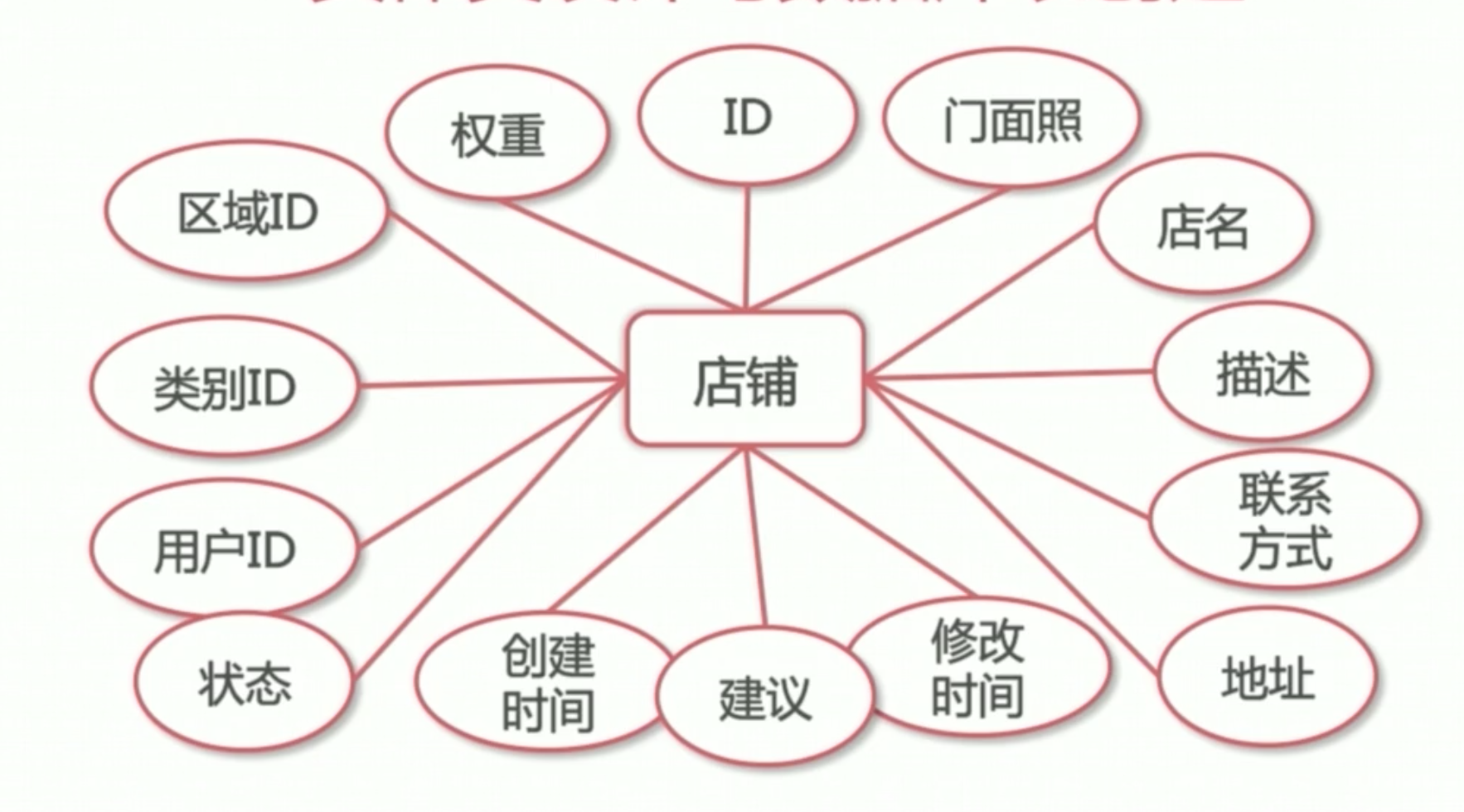
6.1 店铺类
package com.csj2018.o2o.entity;
import java.util.Date;
public class Shop {
private Area area;
private PersonInfo owner;
private ShopCategory shopCategory;
private Long shopId;
private String shopName;
private String shopDesc;
private String shopAddr;
private String phone;
private String shopImg;
private Integer priority;
private Date createTime;
private Date lastEditTime;
//-1不可用 0审核中 1可用
private Integer enableStatus;
//超级管理员给店家的提醒
private String advice;
}
//省略setter和getter方法
}
6.2 店铺表
drop table if EXISTS `tb_shop`;
create table `tb_shop` (
`shop_id` int(10) NOT NULL auto_increment,
`owner_id` int(10) NOT NULL COMMENT '店铺创建人',
`area_id` int(5) DEFAULT NULL,
`shop_category_id` int(11) DEFAULT NULL,
`shop_name` varchar(256) NOT NULL,
`shop_desc` varchar(1024) DEFAULT NULL,
`shop_addr` varchar(200) DEFAULT NULL,
`phone` varchar(128) DEFAULT NULL,
`shop_img` varchar(1024) DEFAULT NULL,
`priority` int(3) DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
`enable_status` int(2) NOT NULL DEFAULT '0',
`advice` varchar(255) DEFAULT NULL,
PRIMARY KEY(`shop_id`),
CONSTRAINT `fk_shop_area` FOREIGN KEY(`area_id`) REFERENCES `tb_area`(`area_id`),
CONSTRAINT `fk_shop_profile` FOREIGN KEY(`owner_id`) REFERENCES `tb_person_info`(`user_id`),
CONSTRAINT `fk_shop_shopcate` FOREIGN KEY(`shop_category_id`) REFERENCES `tb_shop_category`(`shop_category_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
select * from tb_shop;
7. 商品类别
7.1 商品类别类
package com.csj2018.o2o.entity;
import java.util.Date;
public class ProductCategory {
private Long productCategoryId;
private Long shopId;
private String productCategoryName;
private Integer priority;
private Date createTime;
//省略setter和getter方法
}
7.2 商品类别表
drop table if EXISTS `tb_product_category`;
create table `tb_product_category`(
`product_category_id` int(11) NOT NULL auto_increment,
`product_category_name` varchar(100) NOT NULL,
`priority` int(2) DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`shop_id` int(20) NOT NULL DEFAULT '0',
PRIMARY KEY (`product_category_id`),
CONSTRAINT `fk_procate_shop` FOREIGN KEY(`shop_id`) REFERENCES `tb_shop`(`shop_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
select * from tb_product_category;
8. 商品
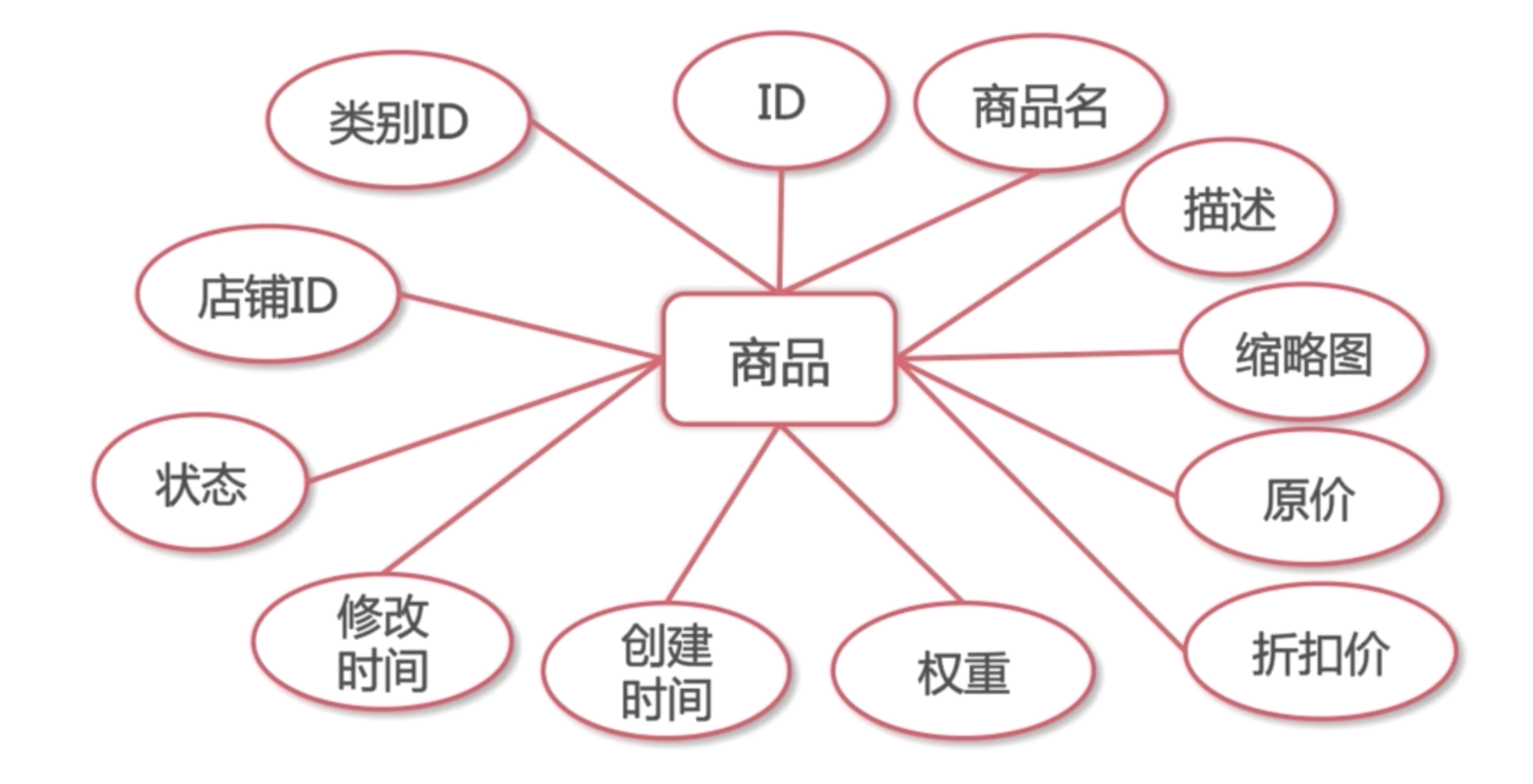
8.1 商品类
package com.csj2018.o2o.entity;
import java.util.Date;
import java.util.List;
public class Product {
private Long productId;
private String productName;
private String productDesc;
//缩略图
private String imgAddr;
private String normalPrice; //原价
private String promotionPrice; //促销价
private Integer priority; //展示权重
private Date createTime;
private Date lastEditTime;
//0下架 1在前端展示
private Integer enableStatus;
private List<ProductImg> productImgList;//商品图片列表
private ProductCategory productCategory; //属于那个商品类别
private Shop shop; //属于那家店铺
//忽略setter和getter方法
}
8.2 商品表
drop table if EXISTS `tb_product`;
create table `tb_product` (
`product_id` int(100) NOT NULL auto_increment,
`product_name` varchar(100) NOT NULL,
`product_desc` varchar(2000) DEFAULT NULL,
`img_addr` varchar(2000) DEFAULT '',
`normal_price` varchar(100) DEFAULT NULL,
`promotion_price` varchar(100) DEFAULT NULL,
`priority` int(2) NOT NULL DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
`enable_status` int(2) NOT NULL DEFAULT '0' comment '0下架 1展示',
`product_category_id` int(11) DEFAULT NULL,
`shop_id` int(20) NOT NULL DEFAULT '0',
PRIMARY KEY(`product_id`),
CONSTRAINT `fk_product_procate` FOREIGN KEY(`product_category_id`) REFERENCES `tb_product_category`(`product_category_id`),
CONSTRAINT `fk_product_shop` FOREIGN KEY(`shop_id`) REFERENCES `tb_shop`(`shop_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
select * from tb_product;
9. 详情图片
9.1 商品图片类
package com.csj2018.o2o.entity;
import java.util.Date;
public class ProductImg {
private Long productImgId;
private String imgAddr;
private String imgDesc;
private Integer priority;
private Date createTime;
private Long productId;
//省略setter和getter方法
}
9.2 商品图片表
drop table if EXISTS `tb_product_img`;
create table `tb_product_img` (
`product_img_id` int(20) NOT NULL auto_increment,
`img_addr` varchar(2000) NOT NULL,
`img_desc` varchar(2000) DEFAULT NULL,
`priority` int(2) DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`product_id` int(20) DEFAULT NULL,
PRIMARY KEY(`product_img_id`),
CONSTRAINT `fk_proimg_product` FOREIGN KEY (`product_id`) REFERENCES `tb_product`(`product_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
select * from tb_product_img;
FAQ:
1.为什么成员变量都用的是引用类型,而不是基本类型?
如果是基本类型,会为空值赋一个默认的值,比如priority,如果是基本类型int,当为空时,priority是0。但对于别的属性,比如areaName,我们并不希望有一个默认值'',空就是空。因此统一都用引用类型。
2.数据库初始化
-- create database if not exists o2o default character set = 'utf8';
-- 1.area
drop table if EXISTS `tb_area`;
create table `tb_area` (
`area_id` int(2) NOT NULL auto_increment,
`area_name` varchar(200) NOT NULL,
`priority` int(2) NOT NULL DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
PRIMARY key(`area_id`),
UNIQUE key `UK_AREA`(`area_name`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
-- 2.用户表
drop table if EXISTS `tb_person_info`;
create table `tb_person_info` (
`user_id` int(10) NOT NULL auto_increment,
`name` varchar(32) default NULL,
`profile_img` varchar(1024) DEFAULT NULL,
`email` varchar(1024) DEFAULT NULL,
`gender` varchar(2) DEFAULT NULL,
`enable_status` int(2) NOT NULL DEFAULT '0' COMMENT '0:禁止使用本商城,1:允许使用本商城',
`user_type` int(2) NOT NULL DEFAULT '1' COMMENT '1:顾客,2:店家,3:超级管理员',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
PRIMARY KEY (`user_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
-- 3微信账号
drop table if EXISTS `tb_wechat_auth`;
create table `tb_wechat_auth` (
`wechat_auth_id` int(10) NOT NULL auto_increment,
`user_id` int(10) NOT NULL,
`open_id` varchar(200) NOT NULL,
`create_time` datetime DEFAULT NULL,
PRIMARY KEY(`wechat_auth_id`),
CONSTRAINT `fk_wechatauth_profile` FOREIGN KEY(`user_id`) REFERENCES `tb_person_info`(`user_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
alter table tb_wechat_auth add UNIQUE INDEX(`open_id`);
-- 4本地账号
drop table if EXISTS `tb_local_auth`;
create table `tb_local_auth`(
`local_auth_id` int(10) NOT NULL auto_increment,
`user_id` int(10) NOT NULL,
`username` varchar(128) NOT NULL,
`password` varchar(128) NOT NULL,
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
PRIMARY KEY(`local_auth_id`),
UNIQUE KEY `uk_local_profile`(`username`),
CONSTRAINT `fk_localauth_profile` FOREIGN KEY(`user_id`) REFERENCES `tb_person_info`(`user_id`)
)ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
-- 5头条
drop table if EXISTS `tb_head_line`;
create table `tb_head_line` (
`line_id` int(100) NOT NULL auto_increment,
`line_name` varchar(1000) DEFAULT NULL,
`line_link` varchar(2000) NOT NULL,
`line_img` varchar(2000) NOT NULL,
`priority` int(2) NOT NULL DEFAULT '0',
`enable_status` int(2) DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
PRIMARY KEY(`line_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
-- 6.店铺类别
drop table if EXISTS `tb_shop_category`;
create table `tb_shop_category` (
`shop_category_id` int(11) NOT NULL auto_increment,
`shop_category_name` varchar(100) NOT NULL DEFAULT '',
`shop_category_desc` varchar(1000) DEFAULT '',
`shop_category_img` varchar(2000) DEFAULT NULL,
`priority` int(2) NOT NULL DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
`parent_id` int(11) DEFAULT NULL,
PRIMARY KEY(`shop_category_id`),
CONSTRAINT `fk_shop_category_self` FOREIGN KEY(`parent_id`) REFERENCES `tb_shop_category`(`shop_category_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
-- 7.店铺
drop table if EXISTS `tb_shop`;
create table `tb_shop` (
`shop_id` int(10) NOT NULL auto_increment,
`owner_id` int(10) NOT NULL COMMENT '店铺创建人',
`area_id` int(5) DEFAULT NULL,
`shop_category_id` int(11) DEFAULT NULL,
`shop_name` varchar(256) NOT NULL,
`shop_desc` varchar(1024) DEFAULT NULL,
`shop_addr` varchar(200) DEFAULT NULL,
`phone` varchar(128) DEFAULT NULL,
`shop_img` varchar(1024) DEFAULT NULL,
`priority` int(3) DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
`enable_status` int(2) NOT NULL DEFAULT '0',
`advice` varchar(255) DEFAULT NULL,
PRIMARY KEY(`shop_id`),
CONSTRAINT `fk_shop_area` FOREIGN KEY(`area_id`) REFERENCES `tb_area`(`area_id`),
CONSTRAINT `fk_shop_profile` FOREIGN KEY(`owner_id`) REFERENCES `tb_person_info`(`user_id`),
CONSTRAINT `fk_shop_shopcate` FOREIGN KEY(`shop_category_id`) REFERENCES `tb_shop_category`(`shop_category_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
-- 8.商品类别
drop table if EXISTS `tb_product_category`;
create table `tb_product_category`(
`product_category_id` int(11) NOT NULL auto_increment,
`product_category_name` varchar(100) NOT NULL,
`priority` int(2) DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`shop_id` int(20) NOT NULL DEFAULT '0',
PRIMARY KEY (`product_category_id`),
CONSTRAINT `fk_procate_shop` FOREIGN KEY(`shop_id`) REFERENCES `tb_shop`(`shop_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
-- 9.商品
drop table if EXISTS `tb_product`;
create table `tb_product` (
`product_id` int(100) NOT NULL auto_increment,
`product_name` varchar(100) NOT NULL,
`product_desc` varchar(2000) DEFAULT NULL,
`img_addr` varchar(2000) DEFAULT '',
`normal_price` varchar(100) DEFAULT NULL,
`promotion_price` varchar(100) DEFAULT NULL,
`priority` int(2) NOT NULL DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
`enable_status` int(2) NOT NULL DEFAULT '0' comment '0下架 1展示',
`product_category_id` int(11) DEFAULT NULL,
`shop_id` int(20) NOT NULL DEFAULT '0',
PRIMARY KEY(`product_id`),
CONSTRAINT `fk_product_procate` FOREIGN KEY(`product_category_id`) REFERENCES `tb_product_category`(`product_category_id`),
CONSTRAINT `fk_product_shop` FOREIGN KEY(`shop_id`) REFERENCES `tb_shop`(`shop_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
-- 10.商品图片
drop table if EXISTS `tb_product_img`;
create table `tb_product_img` (
`product_img_id` int(20) NOT NULL auto_increment,
`img_addr` varchar(2000) NOT NULL,
`img_desc` varchar(2000) DEFAULT NULL,
`priority` int(2) DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`product_id` int(20) DEFAULT NULL,
PRIMARY KEY(`product_img_id`),
CONSTRAINT `fk_proimg_product` FOREIGN KEY (`product_id`) REFERENCES `tb_product`(`product_id`)
) ENGINE=INNODB auto_increment=1 DEFAULT CHARSET=utf8;
-- 插入地区
insert into tb_area (area_name, priority) values('东苑', 1),('南苑', 7),('北苑', 5);
-- 插入用户
insert into tb_person_info(`name`,`profile_img`,`email`,`gender`,`enable_status`,`user_type`)
values ('测试','test_person_png',"test@126.com",1,1,2);
-- 插入店铺类别
insert into tb_shop_category(`shop_category_name`,`shop_category_desc`,`shop_category_img`,`priority`)
values('咖啡奶茶','飘香醇厚咖啡加上芳美浓郁的牛奶,两种经典饮品的绝妙搭配,两种滋味的碰撞,形成了具有双重口感享受的饮品!','test_shop_catetgory_png',1);
-- 插入店铺
set @userId=(select user_id from tb_person_info where `name` ='测试' limit 1);
set @areaId=(select area_id from tb_area where `area_name` = '北苑');
set @shopCategoryId=(select shop_category_id from tb_shop_category where shop_category_name = '咖啡奶茶');
select @userId,@areaId,@shopCategoryId;
insert into tb_shop(
owner_id, area_id, shop_category_id,
shop_name, shop_desc,shop_addr,phone,shop_img,priority,
create_time,
last_edit_time,
enable_status,advice)
values(
@userId, @areaId, @shopCategoryId,
'测试de店铺', '店铺描述', '测试路1号', '1234567890', '商铺图片',3,
str_to_date('yyyy-mm-dd hh24:mi:ss','2020-03-04b 21:51:55'),
str_to_date('yyyy-mm-dd hh24:mi:ss','2020-03-04b 21:51:55'),
1, '审核中');
insert into tb_shop(
`owner_id`, `area_id`,`shop_category_id`,`shop_name`,
`shop_desc`,`shop_addr`,`phone`,`priority`,`create_time`,
`last_edit_time`,`enable_status`)
values
(2,1,8,'美美','工商局调查员','通道北路220号','13811112224',10,
STR_TO_DATE('2020-03-20 21:45:26','%Y-%m-%d %H:%i:%s'),
STR_TO_DATE('2020-03-20 21:45:26','%Y-%m-%d %H:%i:%s'),1),
(2,1,8,'兰兰','地震局调查员','通道北路210号','13811112223',10,
STR_TO_DATE('2020-03-20 21:45:26','%Y-%m-%d %H:%i:%s'),
STR_TO_DATE('2020-03-20 21:45:26','%Y-%m-%d %H:%i:%s'),1);
-- 插入商品列别
set @shopId=(select `shop_id` from tb_shop where `shop_name`='测试de店铺' limit 1);
insert into tb_product_category(`product_category_name`,`priority`,`shop_id`)
values('店铺商品类别1',0,@shopId),('店铺商品类别2',20,@shopId),('店铺商品类别3',2,@shopId);
-- 插入商品
set @productCategoryId=(select product_category_id from tb_product_category where shop_id=@shopId limit 1);
select @shopId,@productCategoryId;
insert into tb_product(`product_name`,`product_desc`,`img_addr`,`normal_price`,`promotion_price`,`priority`,`enable_status`,`product_category_id`,`shop_id`)
values('美式咖啡','美式咖啡的描述','美式咖啡的缩略图',15,10,2,1,@productCategoryId,@shopId);