在js中用到的getshopinitinfo还未实现,现在实现这个方法
var initUrl = '/o2o/shopadmin/getshopinitinfo';//获取店铺的初始信息,还未定义
1 店铺类别
1.1 dao层
package com.csj2018.o2o.dao;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import com.csj2018.o2o.entity.ShopCategory;
public interface ShopCategoryDao {
List<ShopCategory> queryShopCategory(@Param("shopCategoryCondition") ShopCategory shopCategoryCondition);
List<ShopCategory> queryAllShopCategory();
}
1.2 店铺类别mapper
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org/DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.csj2018.o2o.dao.ShopCategoryDao">
<select id="queryShopCategory" resultType="com.csj2018.o2o.entity.ShopCategory">
select shop_category_id,
shop_category_name,
shop_category_desc,
shop_category_img,
priority,
create_time,
last_edit_time,
parent_id
from tb_shop_category
<where>
<if test="shopCategoryCondition.parent != null">
and parent_id = #{shopCategoryCondition.parent.shopCategoryId}
</if>
</where>
order by priority desc
</select>
<select id="queryAllShopCategory" resultType="com.csj2018.o2o.entity.ShopCategory">
select shop_category_id,
shop_category_name,
shop_category_desc,
shop_category_img,
priority,
create_time,
last_edit_time,
parent_id
from tb_shop_category order by priority desc
</select>
</mapper>
1.3 店铺类别验证Dao
package com.csj2018.o2o.dao;
import java.util.List;
import org.junit.Ignore;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import com.csj2018.o2o.BaseTest;
import com.csj2018.o2o.entity.ShopCategory;
public class ShopCatetoryDaoTest extends BaseTest{
@Autowired
private ShopCategoryDao shopCategoryDao;
@Test
public void testQueryShopCategory() {
List<ShopCategory> shopCategoryList = shopCategoryDao.queryShopCategory(new ShopCategory());
System.out.println(shopCategoryList.size());
ShopCategory parent = new ShopCategory();
ShopCategory son = new ShopCategory();
parent.setShopCategoryId(1L);
son.setParent(parent);
List<ShopCategory> shopCategoryList2 = shopCategoryDao.queryShopCategory(son);
}
@Test
@Ignore
public void testAllCategory() {
List<ShopCategory> shopCategoryList = shopCategoryDao.queryAllShopCategory();
}
}
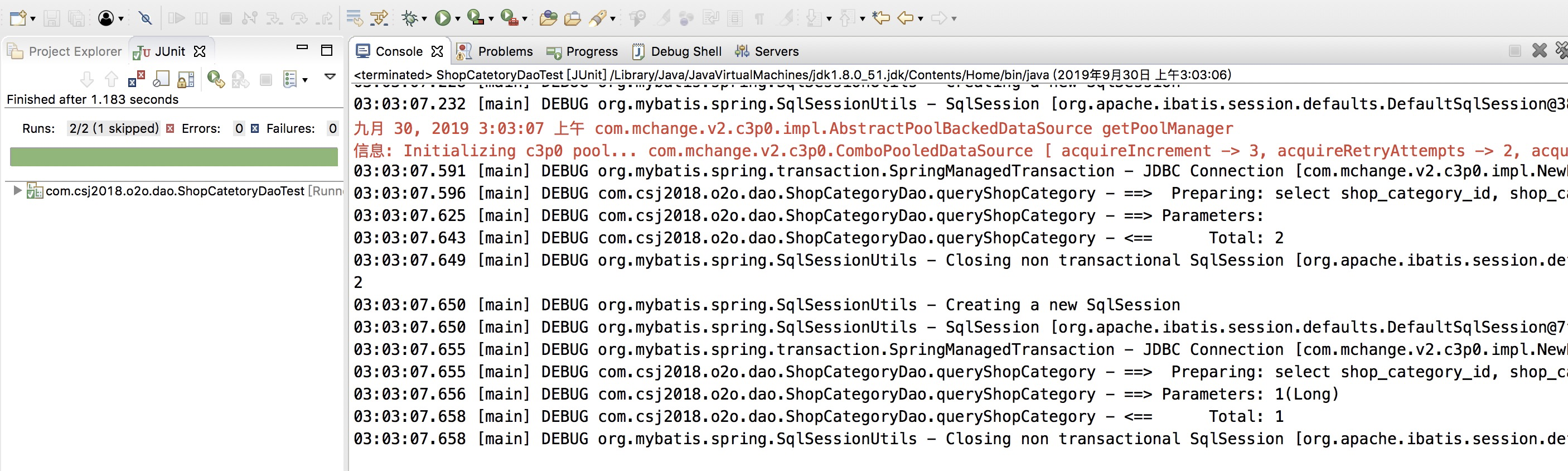
import java.util.List;
import org.apache.ibatis.annotations.Param;
import com.csj2018.o2o.entity.ShopCategory;
public interface ShopCategoryService {
List
}
### 1.5 Service实现层
```#java
package com.csj2018.o2o.service.impl;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.csj2018.o2o.dao.ShopCategoryDao;
import com.csj2018.o2o.entity.ShopCategory;
import com.csj2018.o2o.service.ShopCategoryService;
@Service
public class ShopCategoryServiceImpl implements ShopCategoryService{
@Autowired
private ShopCategoryDao shopCategoryDao;
@Override
public List<ShopCategory> getShopCategoryList(ShopCategory shopCategoryCondition) {
// TODO Auto-generated method stub
return shopCategoryDao.queryShopCategory(shopCategoryCondition);
}
}
1.6 Service验证
package com.csj2018.o2o.service;
import static org.junit.Assert.assertEquals;
import java.util.List;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import com.csj2018.o2o.BaseTest;
import com.csj2018.o2o.entity.ShopCategory;
public class ShopCategoryServiceTest extends BaseTest{
@Autowired
private ShopCategoryService shopCategoryService;
@Test
public void testGetShopCategoryList() {
ShopCategory parent = new ShopCategory();
List<ShopCategory> shopCategoryList = shopCategoryService.getShopCategoryList(parent);
assertEquals(2,shopCategoryList.size());
ShopCategory son = new ShopCategory();
parent.setShopCategoryId(1L);
son.setParent(parent);
List<ShopCategory> shopCategoryList2 = shopCategoryService.getShopCategoryList(son);
assertEquals(1,shopCategoryList2.size());
}
}
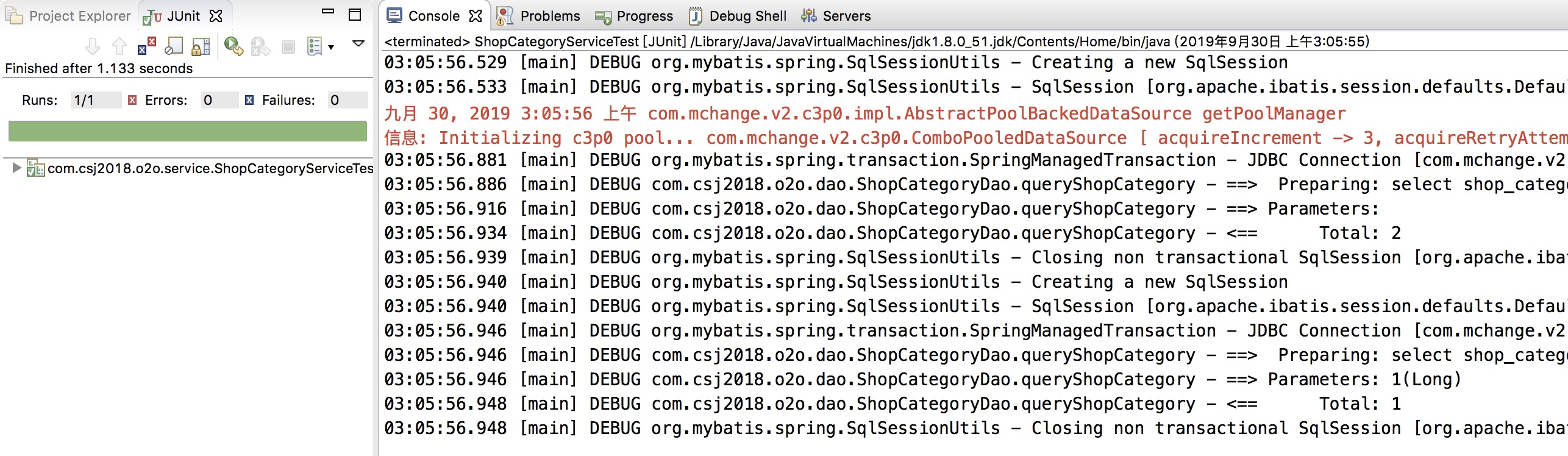
1.7 controller层
package com.csj2018.o2o.web.shopadmin;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import java.io.InputStream;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartHttpServletRequest;
import org.springframework.web.multipart.commons.CommonsMultipartFile;
import org.springframework.web.multipart.commons.CommonsMultipartResolver;
import com.csj2018.o2o.dto.ShopExecution;
import com.csj2018.o2o.entity.Area;
import com.csj2018.o2o.entity.PersonInfo;
import com.csj2018.o2o.entity.Shop;
import com.csj2018.o2o.entity.ShopCategory;
import com.csj2018.o2o.enums.ShopStateEnum;
import com.csj2018.o2o.service.AreaService;
import com.csj2018.o2o.service.ShopCategoryService;
import com.csj2018.o2o.service.ShopService;
import com.csj2018.o2o.util.CodeUtil;
import com.csj2018.o2o.util.HttpServletRequestUtil;
import com.csj2018.o2o.util.ImageUtil;
import com.csj2018.o2o.util.PathUtil;
import com.fasterxml.jackson.databind.ObjectMapper;
// 首先在Controller里面定义了SpringMVC相关的标签,这个标签包含了Controller的访问路径以及registerregisterShop方法的访问路径,
@Controller
@RequestMapping("/shopadmin")
public class ShopManagerController {
// 同时给它在执行的时候通过Spring容器注入之前实现好的ShopService实现类,用来提供addShop的服务。
@Autowired
private ShopService shopService;
@Autowired
private ShopCategoryService shopCategoryService;
@Autowired
private AreaService areaService;
@RequestMapping(value = "getshopinitinfo",method = RequestMethod.GET)
@ResponseBody
private Map<String,Object> getShopInitInfo(HttpServletRequest request){
Map<String,Object> modelMap = new HashMap<String,Object>();
List<ShopCategory> shopCategoryList = new ArrayList<ShopCategory>();
List<Area> areaList = new ArrayList<Area>();
try {
shopCategoryList = shopCategoryService.getShopCategoryList(new ShopCategory());
areaList = areaService.getAreaList();
modelMap.put("shopCategoryList", shopCategoryList);
modelMap.put("areaList", areaList);
modelMap.put("success",true);
}catch(Exception e) {
modelMap.put("success",false);
modelMap.put("errMsg", e.getMessage());
}
return modelMap;
}
@RequestMapping(value = "/registershop", method = RequestMethod.POST)
@ResponseBody
private Map<String, Object> registerShop(HttpServletRequest request) {
// 先定义一个返回值
Map<String, Object> modelMap = new HashMap<String, Object>();
if(!CodeUtil.checkVerifyCode(request)) {
modelMap.put("success", "false");
modelMap.put("message", "输入了错误的验证码");
return modelMap;
}
// 1.接收并转换相应的参数,包括店铺信息以及图片信息
// 获取请求头的店铺信息
String shopStr = HttpServletRequestUtil.getString(request, "shopStr");
// 将json转换为Shop实例
ObjectMapper mapper = new ObjectMapper();
Shop shop = null;
try {
shop = mapper.readValue(shopStr, Shop.class);
} catch (Exception e) {
modelMap.put("success", false);
modelMap.put("errMeg", e.getMessage());
return modelMap;
}
// 将请求中的文件流剥离出来,通过CommonsMultipartFile去接收
CommonsMultipartFile shopImg = null;
CommonsMultipartResolver commonsMultipartResolver = new CommonsMultipartResolver(
request.getSession().getServletContext());
if (commonsMultipartResolver.isMultipart(request)) {
MultipartHttpServletRequest multipartHttpServletRequest = (MultipartHttpServletRequest) request;
shopImg = (CommonsMultipartFile) multipartHttpServletRequest.getFile("shopImg");
} else {
modelMap.put("success", false);
modelMap.put("errMsg", "上传图片不能为空");
return modelMap;
}
// 2.注册店铺
if (shop != null && shopImg != null) {
PersonInfo owner = new PersonInfo();
// 预期从Session获取,目前自定义,以后完善
owner.setUserId(1L);
shop.setOwner(owner);
// 由于addShop的第二个参数是File类型的,而传入的ShopImg是CommonsMultipartFile这样的一个类型,因此需要将CommonsMultipartFile转换成File类型
File shopImgFile = new File(PathUtil.getImgBasePath() + ImageUtil.getRandomFileName());
try {
shopImgFile.createNewFile();
} catch (IOException e) {
modelMap.put("success", false);
modelMap.put("errMsg", e.getMessage());
return modelMap;
}
try {
inputStreamToFile(shopImg.getInputStream(), shopImgFile);
} catch (Exception e) {
modelMap.put("success", false);
modelMap.put("errMsg", e.getMessage());
return modelMap;
}
// 注册店铺
ShopExecution se;
try {
se = shopService.addShop(shop, shopImg.getInputStream(), shopImg.getOriginalFilename());
if (se.getState() == ShopStateEnum.CHECK.getState()) {
modelMap.put("success", true);
} else {
modelMap.put("success", false);
modelMap.put("errMsg", se.getStateInfo());
return modelMap;
}
return modelMap;
} catch (IOException e) {
modelMap.put("success", false);
modelMap.put("errMsg", e.getMessage());
return modelMap;
}
} else {
modelMap.put("success", false);
modelMap.put("errMsg", "请输入店铺信息");
return modelMap;
}
}
private static void inputStreamToFile(InputStream inputStream, File file) {
FileOutputStream os = null;
try {
os = new FileOutputStream(file);
int bytesRead = 0;
byte[] buffer = new byte[1024];
while ((bytesRead = inputStream.read(buffer)) > 0) {
os.write(buffer, 0, bytesRead);
}
} catch (Exception e) {
throw new RuntimeException("调用inputStreamToFile产生异常:" + e.getMessage());
} finally {
try {
if (os != null) {
os.close();
}
if (inputStream != null) {
inputStream.close();
}
} catch (IOException e) {
throw new RuntimeException("调用inputStreamToFile产生异常:" + e.getMessage());
}
}
}
}
1.8验证controller层
浏览器打开地址:http://127.0.0.1:18080/o2o/shopadmin/getshopinitinfo
{"shopCategoryList":[{"shopCategoryId":2,"shopCategoryName":"咖啡","shopCategoryDesc":"咖啡奶茶有咖啡","shopCategoryImg":"kafei.png","priority":0,"createTime":null,"lastEditTime":null,"parent":null}],"success":true,"areaList":[{"areaId":2,"areaName":"南苑","priority":7,"createTime":null,"lastEditTime":null},{"areaId":3,"areaName":"北苑","priority":5,"createTime":null,"lastEditTime":null},{"areaId":1,"areaName":"东苑","priority":1,"createTime":null,"lastEditTime":null}]}
2.前端页面
3.修改店铺类别的mapper
对于店铺类别来说,我们希望所有的店铺都保存在二级类别下面。
主页是由一级类别组成,如二手市场、美容美发、美食饮品、休闲娱乐等
点击二级市场,展示二级类别,如二手车辆、二手书籍。店铺会存储在二级类别下面
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.csj2018.o2o.dao.ShopCategoryDao">
<select id="queryShopCategory" resultType="com.csj2018.o2o.entity.ShopCategory" >
select
shop_category_id,
shop_category_name,
shop_category_desc,
shop_category_img,
priority,create_time,
last_edit_time,
parent_id
from tb_shop_category
<where>
<if test="shopCategoryCondition != null">
and parent_id is not null
</if>
<if test="shopCategoryCondition.parent != null">
and parent_id = #{shopCategoryCondition.parent.shopCategoryId}
</if>
</where>
order by priority desc;
</select>
</mapper>