前端展示系统用来对店铺信息和商品信息进行展示,是顾客了解商品的门户。
首页开发:
- 1.头条读取以及滚动播放
- 2.一级类别列表的获取,之前只是二级类别的获取
1.头条Dao层
1.1 Dao接口
package com.csj2018.o2o.dao;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import com.csj2018.o2o.entity.HeadLine;
public interface HeadLineDao {
/**
* 9-1根据传入的查询条件返回头角
* @param headLineCondition
* @return
*/
List<HeadLine> queryHeadLine(@Param("headLineCondition") HeadLine headLineCondition);
}
1.2 mapper实现
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.csj2018.o2o.dao.HeadLineDao">
<!-- 9-1 -->
<select id="queryHeadLine" resultType="com.csj2018.o2o.entity.HeadLine">
select
line_id,line_name,line_link,line_img,priority,enable_status,create_time,last_edit_time
from tb_head_line
<where>
<if test="headLineCondition.enableStatus!=null">
and enable_status = #{headLineCondition.enableStatus}
</if>
</where>
order by priority desc
</select>
</mapper>
1.3 Dao单元测试
package com.csj2018.o2o.dao;
import static org.junit.Assert.assertEquals;
import java.util.List;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import com.csj2018.o2o.BaseTest;
import com.csj2018.o2o.entity.HeadLine;
public class HeadLineTest extends BaseTest{
@Autowired
private HeadLineDao headLineDao;
@Test
public void testQueryHeadLine() {
List<HeadLine> headLineList = headLineDao.queryHeadLine(new HeadLine());
assertEquals(headLineList.size(),0);
}
}
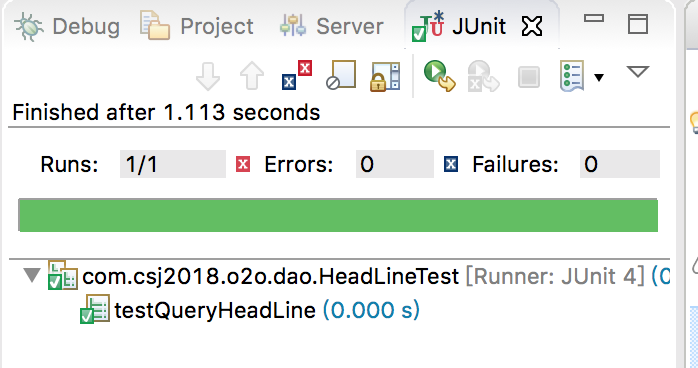
2.商品类别Dao层
商品列别queryShopCategory只支持二级类别,现在改写支持一级类别
2.1 mapper实现
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.csj2018.o2o.dao.ShopCategoryDao">
<!-- 9-1 -->
<select id="queryShopCategory" resultType="com.csj2018.o2o.entity.ShopCategory" >
select
shop_category_id,
shop_category_name,
shop_category_desc,
shop_category_img,
priority,create_time,
last_edit_time,
parent_id
from tb_shop_category
<where>
<if test="shopCategoryCondition == null">
and parent_id is null
</if>
<if test="shopCategoryCondition != null">
and parent_id is not null
</if>
<if test="shopCategoryCondition != null and shopCategoryCondition.parent != null and shopCategoryCondition.parent.shopCategoryId != null">
and parent_id = #{shopCategoryCondition.parent.shopCategoryId}
</if>
</where>
order by priority desc;
</select>
<select id="queryAllShopCategory" resultType="com.csj2018.o2o.entity.ShopCategory">
select shop_category_id,
shop_category_name,
shop_category_desc,
shop_category_img,
priority,
create_time,
last_edit_time,
parent_id
from tb_shop_category order by priority desc
</select>
</mapper>
2.2 单元测试
package com.csj2018.o2o.dao;
import static org.junit.Assert.assertEquals;
import java.util.List;
import org.junit.Ignore;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import com.csj2018.o2o.BaseTest;
import com.csj2018.o2o.entity.ShopCategory;
public class ShopCategoryDaoTest extends BaseTest {
@Autowired
private ShopCategoryDao shopCategoryDao;
@Test
@Ignore
public void testQueryShopCategory() {
//parent_id为1
ShopCategory sonCategory = new ShopCategory();
ShopCategory parentCategory = new ShopCategory();
parentCategory.setShopCategoryId(1L);
sonCategory.setParent(parentCategory);
List<ShopCategory>shopCategoryList = shopCategoryDao.queryShopCategory(sonCategory);
System.out.println(shopCategoryList.get(0).getShopCategoryName());
assertEquals(1,shopCategoryList.size());
}
@Test
@Ignore
public void testAllCategory() {
//传入空的类
List<ShopCategory> shopCategoryList = shopCategoryDao.queryAllShopCategory();
assertEquals(2,shopCategoryList.size());
}
//9-1
@Test
public void testQueryShopCategory2() {
List<ShopCategory> shopCategoryList = shopCategoryDao.queryShopCategory(null);
assertEquals(shopCategoryList.size(),0);
}
}
3.Service层
3.1 Service接口
package com.csj2018.o2o.service;
import java.io.IOException;
import java.util.List;
import com.csj2018.o2o.entity.HeadLine;
public interface HeadLineService {
/**
* 9-1 根据传入的条件返回指定的头条列表
* @param headLineCondition
* @return
* @throws IOException
*/
List<HeadLine> getHeadLineList(HeadLine headLineCondition) throws IOException;
}
3.2 Service实现类
package com.csj2018.o2o.service.impl;
import java.io.IOException;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.csj2018.o2o.dao.HeadLineDao;
import com.csj2018.o2o.entity.HeadLine;
import com.csj2018.o2o.service.HeadLineService;
@Service
public class HeadLineServiceImpl implements HeadLineService{
@Autowired
private HeadLineDao headLineDao;
//9-1
@Override
public List<HeadLine> getHeadLineList(HeadLine headLineCondition) throws IOException{
return headLineDao.queryHeadLine(headLineCondition);
}
}
4. Controller层
4.1 Contrller层
package com.csj2018.o2o.web.frontend;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import com.csj2018.o2o.entity.HeadLine;
import com.csj2018.o2o.entity.ShopCategory;
import com.csj2018.o2o.service.HeadLineService;
import com.csj2018.o2o.service.ShopCategoryService;
@Controller
@RequestMapping(value="/frontend")
public class MainPageController {
@Autowired
private ShopCategoryService shopCategoryService;
@Autowired
private HeadLineService headLineService;
/**
* 9-1初始化前端展示系统的主页信息,包括获取一级店铺类别列表和头条列表
* @return
*/
@RequestMapping(value="/listmainpageinfo",method=RequestMethod.GET)
@ResponseBody
public Map<String,Object> listMainPageInfo(){
Map<String,Object> modelMap = new HashMap<String,Object>();
List<ShopCategory> shopCategoryList = new ArrayList<ShopCategory>();
try {
//获取一级店铺类别列表,即parentId为空的shopCategory
shopCategoryList = shopCategoryService.getShopCategoryList(null);
modelMap.put("shopCategoryList", shopCategoryList);
}catch (Exception e) {
modelMap.put("success", false);
modelMap.put("errMsg", e.getMessage());
return modelMap;
}
List<HeadLine> headLineList = new ArrayList<HeadLine>();
try {
//获取状态为可用1的头条列表
HeadLine headLineCondition = new HeadLine();
headLineCondition.setEnableStatus(1);
headLineList = headLineService.getHeadLineList(headLineCondition);
modelMap.put("headLineList",headLineList);
}catch (Exception e) {
modelMap.put("success", false);
modelMap.put("errMsg", e.getMessage());
return modelMap;
}
modelMap.put("success", true);
return modelMap;
}
}
4.2 controller验证
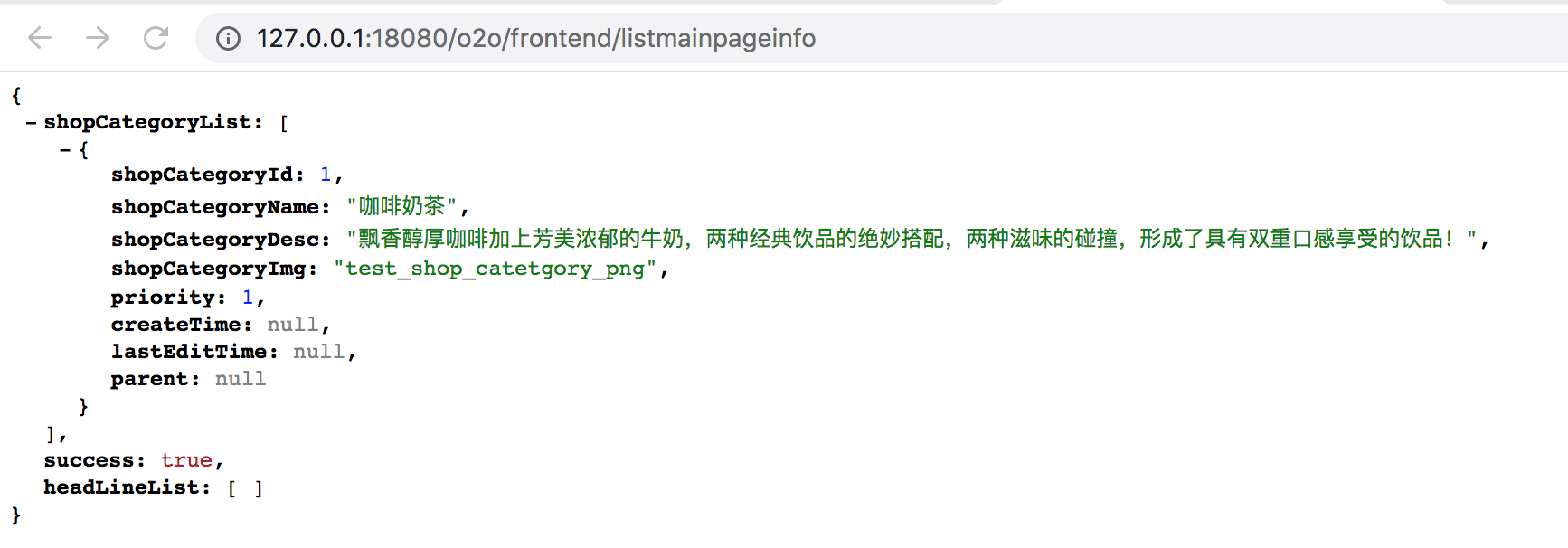
insert into tb_head_line(line_name,line_link,line_img,priority,enable_status)VALUES
('五菱之光','https://www.autohome.com.cn/2456/','/upload/item/headtitle/zhiguang.jpg',4,1),
('五菱宏光','https://www.autohome.com.cn/2139/','/upload/item/headtitle/hongguang.jpg',5,1),
('五零荣光','https://www.autohome.com.cn/2451/','/upload/item/headtitle/rongguang.jpg',7,1);
select line_name,line_link,line_img,priority,enable_status from tb_head_line;