在某些情况下希望处理一些图片,比如
给图片添加一般文字:
原图

程序处理成

给图片添加水印:
原图
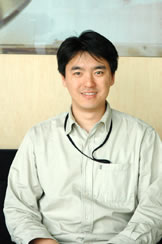
程序处理成

还有圆角效果:
原图

程序处理成

注:
(1)以下程序针对这个应用,也许这些对你没有用,但是基本的原理都差不多
(2)以上图为测试用,应该不算侵犯肖像权吧
程序界面:
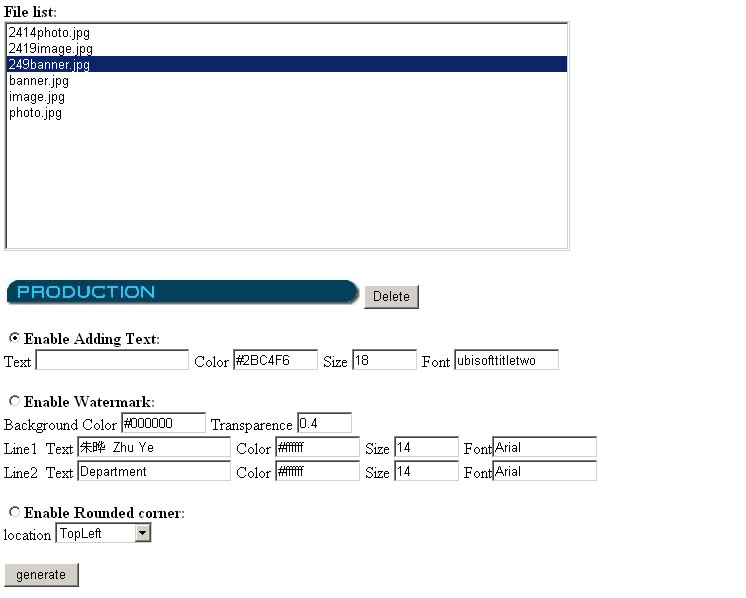
程序点击这里下载。
如果需要提高图片质量,把
image.Save(sDstFilePath, ImageFormat.Jpeg);
改成
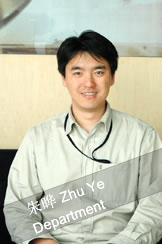
附一些关键代码:
给图片添加一般文字:
原图

程序处理成

给图片添加水印:
原图
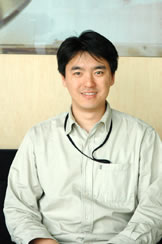
程序处理成

还有圆角效果:
原图

程序处理成

注:
(1)以下程序针对这个应用,也许这些对你没有用,但是基本的原理都差不多
(2)以上图为测试用,应该不算侵犯肖像权吧
程序界面:
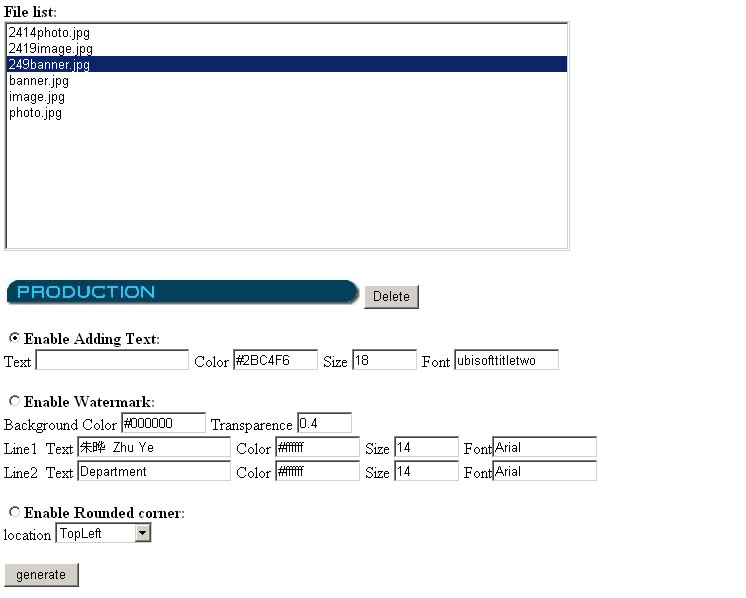
程序点击这里下载。
如果需要提高图片质量,把
image.Save(sDstFilePath, ImageFormat.Jpeg);
改成
= ImageCodecInfo.GetImageEncoders()[0];
myEncoder = Encoder.Quality;
myEncoderParameters = new EncoderParameters(1);
myEncoderParameter = new EncoderParameter(myEncoder, 100L); // 0-100
myEncoderParameters.Param[0] = myEncoderParameter;
image.Save(sDstFilePath, myImageCodecInfo, myEncoderParameters);
myEncoderParameter.Dispose();
myEncoderParameters.Dispose();
这是提高质量后的效果:myEncoder = Encoder.Quality;
myEncoderParameters = new EncoderParameters(1);
myEncoderParameter = new EncoderParameter(myEncoder, 100L); // 0-100
myEncoderParameters.Param[0] = myEncoderParameter;
image.Save(sDstFilePath, myImageCodecInfo, myEncoderParameters);
myEncoderParameter.Dispose();
myEncoderParameters.Dispose();
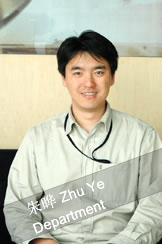
附一些关键代码:
using System; using System.Data; using System.Configuration; using System.Collections; using System.Web; using System.Web.Security; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web.UI.HtmlControls; using System.IO; using System.Drawing; using System.Drawing.Drawing2D; using System.Drawing.Imaging; public partial class Index : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { DirectoryInfo di = new DirectoryInfo(Server.MapPath(ConfigurationManager.AppSettings["uploadpath"])); this.lb_ImageList.DataSource = di.GetFiles("*.jpg"); this.lb_ImageList.DataBind(); } } protected void btn_upload_Click(object sender, EventArgs e) { string sFileName = this.FileUpload1.FileName; if (Path.GetExtension(sFileName).ToLower() != ".jpg") { ClientScriptManager csm = Page.ClientScript; csm.RegisterStartupScript(this.GetType(), "", "<script>alert('System can only handle jpg file!')</script>"); } else { string sFileFullPath = Server.MapPath(ConfigurationManager.AppSettings["uploadpath"] + sFileName); this.FileUpload1.SaveAs(sFileFullPath); this.lb_ImageList.Items.Add(sFileName); this.lb_ImageList.SelectedIndex = -1; this.lb_ImageList.Items.FindByValue(sFileName).Selected = true; this.img_Src.ImageUrl = ConfigurationManager.AppSettings["uploadpath"] + sFileName; } } protected void lb_ImageList_SelectedIndexChanged(object sender, EventArgs e) { this.img_Src.ImageUrl = ConfigurationManager.AppSettings["uploadpath"] + this.lb_ImageList.SelectedValue; ; } protected void btn_Generate_Click(object sender, EventArgs e) { string sFileName = this.lb_ImageList.SelectedValue; string prefix = DateTime.Now.Minute.ToString() + DateTime.Now.Second.ToString(); string sSrcFilePath = Server.MapPath(ConfigurationManager.AppSettings["uploadpath"] + sFileName); string sDstFilePath = Server.MapPath(ConfigurationManager.AppSettings["uploadpath"] + prefix + sFileName); ; if (this.rb_Text.Checked) { try { MyGDI.CreatePlainText(sSrcFilePath, sDstFilePath,this.tb_Text.Text,tb_Color.Text,tb_Size.Text,this.tb_Font.Text); this.lb_ImageList.Items.Add(prefix + sFileName); this.lb_ImageList.SelectedIndex = -1; this.lb_ImageList.Items.FindByValue(prefix + sFileName).Selected = true; this.img_Src.ImageUrl = ConfigurationManager.AppSettings["uploadpath"] + prefix+ sFileName; } catch (Exception ex) { Response.Write(ex.Message); } } else if (this.rb_Watermark.Checked) { try { MyGDI.CreateWatermark(sSrcFilePath, sDstFilePath, this.tb_Text1.Text, this.tb_Color1.Text, this.tb_Size1.Text, this.tb_Font1.Text, this.tb_Text2.Text, this.tb_Color2.Text, this.tb_Size2.Text, this.tb_Font2.Text, this.tb_bgcolor.Text, this.tb_transparence.Text); this.lb_ImageList.Items.Add(prefix + sFileName); this.lb_ImageList.SelectedIndex = -1; this.lb_ImageList.Items.FindByValue(prefix + sFileName).Selected = true; this.img_Src.ImageUrl = ConfigurationManager.AppSettings["uploadpath"] + prefix + sFileName; } catch (Exception ex) { Response.Write(ex.Message); } } else if (this.rb_Corner.Checked) { try { MyGDI.CreateRoundedCorner(sSrcFilePath, sDstFilePath, this.ddl_CornerLocation.SelectedValue); this.lb_ImageList.Items.Add(prefix + sFileName); this.lb_ImageList.SelectedIndex = -1; this.lb_ImageList.Items.FindByValue(prefix + sFileName).Selected = true; this.img_Src.ImageUrl = ConfigurationManager.AppSettings["uploadpath"] + prefix + sFileName; } catch(Exception ex) { Response.Write(ex.Message); } } } protected void btn_Delete1_Click(object sender, EventArgs e) { string sFileFullPath = Server.MapPath(ConfigurationManager.AppSettings["uploadpath"] + this.lb_ImageList.SelectedValue); FileInfo fi = new FileInfo(sFileFullPath); fi.Delete(); this.lb_ImageList.Items.Remove(this.lb_ImageList.SelectedItem); } } public class MyGDI { public static void CreateWatermark(string sSrcFilePath, string sDstFilePath, string sText1, string sColor1, string sSize1, string sFont1, string sText2, string sColor2, string sSize2, string sFont2, string sBgColor, string sTransparence) { System.Drawing.Image image = System.Drawing.Image.FromFile(sSrcFilePath); Graphics g = Graphics.FromImage(image); g.SmoothingMode = SmoothingMode.AntiAlias; g.InterpolationMode = InterpolationMode.HighQualityBicubic; g.CompositingQuality = CompositingQuality.HighQuality; g.TextRenderingHint = System.Drawing.Text.TextRenderingHint.AntiAlias; //文字抗锯齿 g.DrawImage(image, 0, 0, image.Width, image.Height); Font f1 = new Font(sFont1, float.Parse(sSize1)); Font f2 = new Font(sFont2, float.Parse(sSize2)); Brush brushfortext1 = new SolidBrush(ColorTranslator.FromHtml(sColor1)); Brush brushfortext2 = new SolidBrush(ColorTranslator.FromHtml(sColor2)); Brush brushforbg = new SolidBrush(Color.FromArgb(Convert.ToInt16(255 * float.Parse(sTransparence)), ColorTranslator.FromHtml(sBgColor))); g.RotateTransform(-20); Rectangle rect = new Rectangle(-image.Width/2-50, image.Height - 50, image.Width * 2, 40); g.DrawRectangle(new Pen(brushforbg), rect); g.FillRectangle(brushforbg, rect); Rectangle rectfortext1 = new Rectangle(-image.Width/2 + image.Width / 5, image.Height - 45, image.Width * 2, 60); for (int i = 0; i < 10; i++) g.DrawString(sText1, f1, brushfortext1, rectfortext1); Rectangle rectfortext2 = new Rectangle(-image.Width / 2 + image.Width / 5, image.Height -25, image.Width * 2, 60); for (int i = 0; i < 10; i++) g.DrawString(sText2, f2, brushfortext2, rectfortext2); image.Save(sDstFilePath, ImageFormat.Jpeg); image.Dispose(); } public static void CreateRoundedCorner(string sSrcFilePath, string sDstFilePath, string sCornerLocation) { System.Drawing.Image image = System.Drawing.Image.FromFile(sSrcFilePath); Graphics g = Graphics.FromImage(image); g.SmoothingMode = SmoothingMode.HighQuality; g.InterpolationMode = InterpolationMode.HighQualityBicubic; g.CompositingQuality = CompositingQuality.HighQuality; Rectangle rect = new Rectangle(0, 0, image.Width, image.Height); GraphicsPath rectPath = CreateRoundRectanglePath(rect, image.Width / 10, sCornerLocation); //构建圆角外部路径 Brush b = new SolidBrush(Color.White);//圆角背景白色 g.DrawPath(new Pen(b), rectPath); g.FillPath(b, rectPath); g.Dispose(); image.Save(sDstFilePath, ImageFormat.Jpeg); image.Dispose(); } public static void CreatePlainText(string sSrcFilePath, string sDstFilePath,string sText, string sColor, string sSize, string sFont) { System.Drawing.Image image = System.Drawing.Image.FromFile(sSrcFilePath); Graphics g = Graphics.FromImage(image); g.SmoothingMode = SmoothingMode.AntiAlias; g.InterpolationMode = InterpolationMode.HighQualityBicubic; g.CompositingQuality = CompositingQuality.HighQuality; g.DrawImage(image, 0, 0, image.Width, image.Height); g.TextRenderingHint = System.Drawing.Text.TextRenderingHint.AntiAlias; //文字抗锯齿 Font f = new Font(sFont,float.Parse(sSize)); Brush b = new SolidBrush(ColorTranslator.FromHtml(sColor)); Rectangle rect = new Rectangle(10, 5, image.Width, image.Height); //适当空开一段距离 for (int i = 0; i < 30; i++) //加强亮度 g.DrawString(sText, f, b, rect); image.Save(sDstFilePath, ImageFormat.Jpeg); image.Dispose(); } private static GraphicsPath CreateRoundRectanglePath(Rectangle rect, int radius, string sPosition) { GraphicsPath rectPath = new GraphicsPath(); switch (sPosition) { case "TopLeft": { rectPath.AddArc(rect.Left, rect.Top, radius * 2, radius * 2, 180, 90); rectPath.AddLine(rect.Left, rect.Top, rect.Left, rect.Top + radius); break; } case "TopRight": { rectPath.AddArc(rect.Right - radius * 2, rect.Top, radius * 2, radius * 2, 270, 90); rectPath.AddLine(rect.Right, rect.Top, rect.Right - radius, rect.Top); break; } case "BottomLeft": { rectPath.AddArc(rect.Left, rect.Bottom - radius * 2, radius * 2, radius * 2, 90, 90); rectPath.AddLine(rect.Left, rect.Bottom - radius, rect.Left, rect.Bottom); break; } case "BottomRight": { rectPath.AddArc(rect.Right - radius * 2, rect.Bottom - radius * 2, radius * 2, radius * 2, 0, 90); rectPath.AddLine(rect.Right - radius, rect.Bottom, rect.Right, rect.Bottom); break; } } return rectPath; } }