一、默认进行懒观察(lazy observation)
在 2.x 版本里,不管数据多大,都会在一开始就为其创建观察者。当数据很大时,这可能会在页面载入时造成明显的性能压力。3.x 版本,只会对「被用于渲染初始可见部分的数据」创建观察者,而且 3.x 的观察者更高效。
二、更精准的变更通知。
举例来说:2.x 版本中,使用 Vue.set 来给对象新增一个属性时,这个对象的所有 watcher 都会重新运行;3.x 版本中,只有依赖那个属性的 watcher 才会重新运行。
三、3.0 新加入了 TypeScript 以及 PWA 的支持
四、部分命令发生了变化:
1.下载安装 npm install -g vue@cli
2.删除了vue list
3.创建项目 vue create
4.启动项目 npm run serve
五、默认项目目录结构也发生了变化:
移除了配置文件目录,config 和 build 文件夹
移除了 static 文件夹,新增 public 文件夹,并且 index.html 移动到 public 中
在 src 文件夹中新增了 views 文件夹,用于分类 视图组件 和 公共组件
六、使用上的变化
1.Data
export default { data(){ return{ } } }, /////////////////////// 取而代之是使用以下的方式去初始化数据: <template> <div class="hello"> 123 </div> <div>{{name.name}}</div> </template> import {reactive} from 'vue' export default { setup(){ const name = reactive({ name:'hello 番茄' }) return {name} } }
假设如果你不return出来,而直接去使用的话浏览器是会提醒你:
runtime-core.esm-bundler.js?5c40:37 [Vue warn]: Property "name" was accessed during render but is not defined on instance.
at <Anonymous>
at <Anonymous> (Root)
2.Method
<div class="hello"> <div>{{name.name}}</div> <div>{{count}}</div> <button @click="increamt">button</button> </div> </template> <script> import {reactive,ref} from 'vue' export default { setup(){ const name = reactive({ name:'王' }) const count=ref(0) const increamt=()=>{ count.value++ } return {name,count,increamt} } }
需要注意的时,在ref的函数中,如何你要去改变或者去引用它的值,ref的这个方法提供了一个value的返回值,对值进行操作。
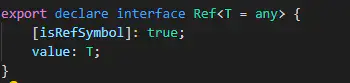
3.LifeCycle(Hooks) 3.0当中的生命周期与2.0的生命周期出现了很大的不同:
beforeCreate -> 请使用 setup()
created -> 请使用 setup()
beforeMount -> onBeforeMount
mounted -> onMounted
beforeUpdate -> onBeforeUpdate
updated -> onUpdated
beforeDestroy -> onBeforeUnmount
destroyed -> onUnmounted
errorCaptured -> onErrorCaptured
如果要想在页面中使用生命周期函数的,根据以往2.0的操作是直接在页面中写入生命周期,而现在是需要去引用的,这就是为什么3.0能够将代码压缩到更低的原因。
import {reactive, ref, onMounted} from 'vue'
export default {
setup(){
const name = reactive({
name:'王'
})
const count=ref(0)
const increamt=()=>{
count.value++
}
onMounted(()=>{
console.log('123')
})
return {name,count,increamt}
}
4.computed
<template>
<div class="hello">
<div>{{name.name}}</div>
<div>{{count}}</div>
<div>计算属性{{computeCount}}</div>
<button @click="increamt">button</button>
</div>
</template>
<script>
import {reactive, ref, onMounted,computed} from 'vue'
export default {
setup(){
const name = reactive({
name:'王'
})
const count=ref(0)
const increamt=()=>{
count.value++
}
const computeCount=computed(()=>count.value*10)//使用computed记得需要引入,这也是刚接触3.0容易忘记的事情
onMounted(()=>{
console.log('123')
})
return {name,count,increamt,computeCount}
}
}
</script>
5.组件使用上的区别
<template> <div id="app"> <HelloWorld msg="Welcome to Your Vue.js App"/> </div> </template> <script> import HelloWorld from './components/HelloWorld.vue' export default { name: 'App', components: { HelloWorld } } </script> 子组件 <template> <div class="hello"> <h1>{{ msg }}</h1> </div> </template> <script> export default { name: 'HelloWorld', props: { msg: String }, data(){ return{ } }, method:{ handleClick(){ this.$emit('childclick','123') } } } </script>
以上是最常见的父子组件之间的调用,但是在vue3.0中就存在差异。
父组件:
<template>
<div class="hello">
<div>123</div>
<NewComp :name="name" @childClick="parentClick"/>
</div>
</template>
<script>
import {reactive} from 'vue'
import NewComp from './newComp.vue'
export default {
components:{
NewComp
},
setup(){
const name=reactive({
name:'hello 番茄'
})
const parentClick=(e)=>{
console.log(e)
console.log('123')
}
return {name,parentClick}
}
}
</script>
子组件:
<template> <div> <button @click="handleClick">组件</button> </div> </template> <script> export default { setup(props,{emit} ){ const handleClick=()=>{ emit('childClick','hello') } return { props, handleClick } } } </script>
通过上面的vue3.0父子组件之间的调用,我们不难发现,父组件当中在调用子组件时,基本与2.0相同,而在子组件当中,要想获取到父组件传递过来的参数,我们是直接在setup()中直接获取到props值和emit事件。这是因为setup为我们提供了props以及context这两个属性,而在context中又包含了emit等事件。 为什么不用this.$emit的方法来向外触发子组件事件,因为在3.0当中已经不在使用this关键词。