二、Creating Custom Routes
In this tutorial, you learn how to add a custom route to an ASP.NET MVC application. You learn how to modify the default route table in the Global.asax file with a custom route.
For many simple ASP.NET MVC applications, the default route table will work just fine. However, you might discover that you have specialized routing needs. In that case, you can create a custom route.
Imagine, for example, that you are building a blog application. You might want to handle incoming requests that look like this:
/Archive/12-25-2009
When a user enters this request, you want to return the blog entry that corresponds to the date 12/25/2009. In order to handle this type of request, you need to create a custom route.
The Global.asax file in Listing 1 contains a new custom route, named Blog, which handles requests that look like /Archive/entry date.
Listing 1 - Global.asax (with custom route)
using System.Web.Mvc; using System.Web.Routing; namespace MvcApplication1 { public class MvcApplication : System.Web.HttpApplication { public static void RegisterRoutes(RouteCollection routes) { routes.IgnoreRoute("{resource}.axd/{*pathInfo}"); routes.MapRoute( "Blog", // Route name "Archive/{entryDate}", // URL with parameters new { controller = "Archive", action = "Entry" } // Parameter defaults ); routes.MapRoute( "Default", // Route name "{controller}/{action}/{id}", // URL with parameters new { controller = "Home", action = "Index", id = "" } // Parameter defaults ); } protected void Application_Start() { RegisterRoutes(RouteTable.Routes); } } }
The order of the routes that you add to the route table is important. Our new custom Blog route is added before the existing Default route. If you reversed the order, then the Default route always will get called instead of the custom route.
The custom Blog route matches any request that starts with /Archive/. So, it matches all of the following URLs:
/Archive/12-25-2009
/Archive/10-6-2004
/Archive/apple
The custom route maps the incoming request to a controller named Archive and invokes the Entry() action. When the Entry() method is called, the entry date is passed as a parameter named entryDate.
You can use the Blog custom route with the controller in Listing 2.
Listing 2 - ArchiveController.cs
using System; using System.Web.Mvc; namespace MvcApplication1.Controllers { public class ArchiveController : Controller { public string Entry(DateTime entryDate) { return "You requested the entry from " + entryDate.ToString(); } } }
Notice that the Entry() method in Listing 2 accepts a parameter of type DateTime. The MVC framework is smart enough to convert the entry date from the URL into a DateTime value automatically. If the entry date parameter from the URL cannot be converted to a DateTime, an error is raised (see Figure 1).
Figure 1 - Error from converting parameter
Summary
The goal of this tutorial was to demonstrate how you can create a custom route. You learned how to add a custom route to the route table in the Global.asax file that represents blog entries. We discussed how to map requests for blog entries to a controller named ArchiveController and a controller action named Entry().
三、Creating a Route Constraint
In this tutorial, Stephen Walther demonstrates how you can control how browser requests match routes by creating route constraints with regular expressions.
You use route constraints to restrict the browser requests that match a particular route. You can use a regular expression to specify a route constraint.
For example, imagine that you have defined the route in Listing 1 in your Global.asax file.
Listing 1 - Global.asax.cs
routes.MapRoute( "Product", "Product/{productId}", new {controller="Product", action="Details"} );
Listing 1 contains a route named Product.
You can use the Product route to map browser requests to the ProductController contained in Listing 2.
Listing 2 - ControllersProductController.cs
using System.Web.Mvc; namespace MvcApplication1.Controllers { public class ProductController : Controller { public ActionResult Details(int productId) { return View(); } } }
Notice that the Details() action exposed by the Product controller accepts a single parameter named productId. This parameter is an integer parameter.
The route defined in Listing 1 will match any of the following URLs:
- /Product/23
- /Product/7
Unfortunately, the route will also match the following URLs:
- /Product/blah
- /Product/apple
Because the Details() action expects an integer parameter, making a request that contains something other than an integer value will cause an error. For example, if you type the URL /Product/apple into your browser then you will get the error page in Figure 1.
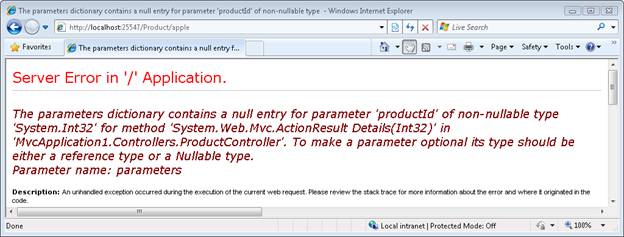
What you really want to do is only match URLs that contain a proper integer productId. You can use a constraint when defining a route to restrict the URLs that match the route. The modified Product route in Listing 3 contains a regular expression constraint that only matches integers.
Listing 3 - Global.asax.cs
routes.MapRoute( "Product", "Product/{productId}", new {controller="Product", action="Details"}, new {productId = @"d+" } );
The regular expression d+ matches one or more integers. This constraint causes the Product route to match the following URLs:
- /Product/3
- /Product/8999
But not the following URLs:
- /Product/apple
- /Product
These browser requests will be handled by another route or, if there are no matching routes, a The resource could not be found error will be returned.
四、Creating a Custom Route Constraint
Stephen Walther demonstrates how you can create a custom route constraint. We implement a simple custom constraint that prevents a route from being matched when a browser request is made from a remote computer.
The goal of this tutorial is to demonstrate how you can create a custom route constraint. A custom route constraint enables you to prevent a route from being matched unless some custom condition is matched.
In this tutorial, we create a Localhost route constraint. The Localhost route constraint only matches requests made from the local computer. Remote requests from across the Internet are not matched.
You implement a custom route constraint by implementing the IRouteConstraint interface. This is an extremely simple interface which describes a single method:
bool Match( HttpContextBase httpContext, Route route, string parameterName, RouteValueDictionary values, RouteDirection routeDirection )
The method returns a Boolean value. If you return false, the route associated with the constraint won’t match the browser request.The Localhost constraint is contained in Listing 1.
Listing 1 - LocalhostConstraint.cs
using System.Web; using System.Web.Routing; namespace MvcApplication1.Constraints { public class LocalhostConstraint : IRouteConstraint { public bool Match ( HttpContextBase httpContext, Route route, string parameterName, RouteValueDictionary values, RouteDirection routeDirection ) { return httpContext.Request.IsLocal; } } }
The constraint in Listing 1 takes advantage of the IsLocal property exposed by the HttpRequest class. This property returns true when the IP address of the request is either 127.0.0.1 or when the IP of the request is the same as the server’s IP address.
You use a custom constraint within a route defined in the Global.asax file. The Global.asax file in Listing 2 uses the Localhost constraint to prevent anyone from requesting an Admin page unless they make the request from the local server. For example, a request for /Admin/DeleteAll will fail when made from a remote server.
Listing 2 - Global.asax
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; using System.Web.Routing; using MvcApplication1.Constraints; namespace MvcApplication1 { public class MvcApplication : System.Web.HttpApplication { public static void RegisterRoutes(RouteCollection routes) { routes.IgnoreRoute("{resource}.axd/{*pathInfo}"); routes.MapRoute( "Admin", "Admin/{action}", new {controller="Admin"}, new {isLocal=new LocalhostConstraint()} ); //routes.MapRoute( // "Default", // Route name // "{controller}/{action}/{id}", // URL with parameters // new { controller = "Home", action = "Index", id = "" } // Parameter defaults //); } protected void Application_Start() { RegisterRoutes(RouteTable.Routes); } } }
The Localhost constraint is used in the definition of the Admin route. This route won’t be matched by a remote browser request. Realize, however, that other routes defined in Global.asax might match the same request. It is important to understand that a constraint prevents a particular route from matching a request and not all routes defined in the Global.asax file.
Notice that the Default route has been commented out from the Global.asax file in Listing 2. If you include the Default route, then the Default route would match requests for the Admin controller. In that case, remote users could still invoke actions of the Admin controller even though their requests wouldn’t match the Admin route.
原文:http://www.asp.net/mvc/tutorials/controllers-and-routing/asp-net-mvc-controller-overview-vb
译文:http://www.cnblogs.com/JimmyZhang/archive/2009/03/08/1406512.html