第一、装饰者模式定义
装饰者模式是在不改变原有对象的基础之上,将功能附加到对象上,提供了比继承更有弹性的替代方案(扩展原有对象的功能),
属于结构型模式。
第二、装饰者的应用场景
1、用于扩展一个类的功能或者给一个类添加附加职责
2、动态的给一个类添加一个功能,这些功能可以再动态的撤销
第三、装饰者的优缺点
优点:
1、装饰者是继承的有力补充,比继承灵活,不改变原有对象的情况下动态地给一个对象
扩展功能,即插即用。
2、通过使用不同装饰类以及这些装饰类的排列组合,可以实现不同效果。
3、装饰者完全遵守开闭原则。
缺点:
1、会出现更多的代码,更多的类,增加程序复杂性。
2、动态装饰时,多层装饰时会更复杂。
第四、装饰者的类图
第五、装饰着模式的定义
(1)抽象组件:定义一个抽象接口,来规范准备附加功能的类
(2)具体组件:将要被附加功能的类,实现抽象构件角色接口
(3)抽象装饰者:持有对具体构件角色的引用并定义与抽象构件角色一致的接口
(4)具体装饰:实现抽象装饰者角色,负责对具体构件添加额外功能。
第六、代码实现
/**
* 定义一个抽象接口
*/
public abstract class GatewayComponent {
public abstract void service();
}
/**
* 定义一个抽象的装饰者
*/
public abstract class AbstractDecorator extends GatewayComponent{
private GatewayComponent gatewayComponent;
public AbstractDecorator(GatewayComponent gatewayComponent){
this.gatewayComponent = gatewayComponent;
}
@Override
public void service() {
this.gatewayComponent.service();
System.out.println("这个是抽象的装饰着类");
}
}
/**
* 被装饰着
*/
public class BaseGateway extends GatewayComponent{
@Override
public void service() {
System.out.println("被装饰着");
}
}
/**
* 日志装饰着
*/
public class LogDecorator extends AbstractDecorator {
public LogDecorator(GatewayComponent gatewayComponent) {
super(gatewayComponent);
}
@Override
public void service() {
//调用装饰着模式
super.service();
System.out.println("日志收集");
}
}
/**
* 限流
*/
public class LimitDecorator extends AbstractDecorator {
public LimitDecorator(GatewayComponent gatewayComponent) {
super(gatewayComponent);
}
@Override
public void service() {
super.service();
System.out.println("限流操作");
}
}
/**
* 获取工厂数据
*/
public class DecoratorFactory {
public static GatewayComponent getGatewayComponent(){
GatewayComponent gatewayComponent = new BaseGateway();
gatewayComponent = new LogDecorator(gatewayComponent);
gatewayComponent = new LimitDecorator(gatewayComponent);
return gatewayComponent;
}
}
/**
* 测试类
*/
public class Test001 {
public static void main(String[] args) {
GatewayComponent gatewayComponent = DecoratorFactory.getGatewayComponent();
gatewayComponent.service();
}
}
第七、装饰着在源码中应用
装饰器模式在源码中也应用得非常多,在 JDK 中体现最明显的类就是 IO 相关的类,如
BufferedReader、InputStream、OutputStream,看一下常用的 InputStream 的类结
构图:
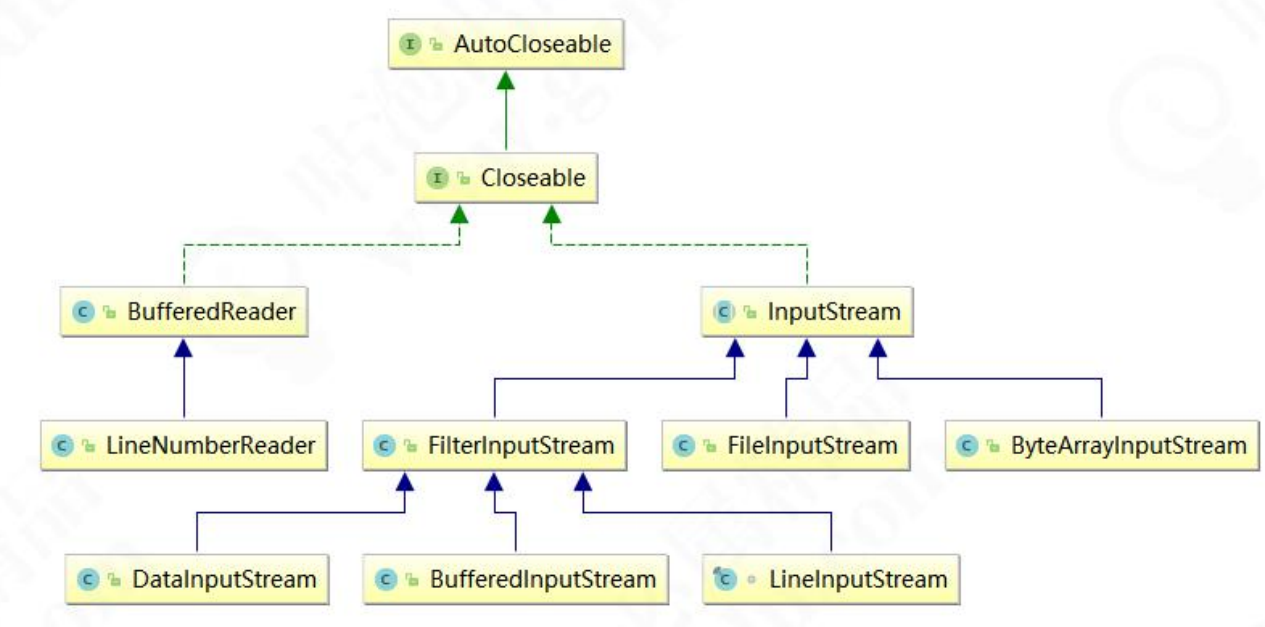
看看 MyBatis 中的一段处理缓存的设计 org.apache.ibatis.cache.Cache 类,找到它的包定位:
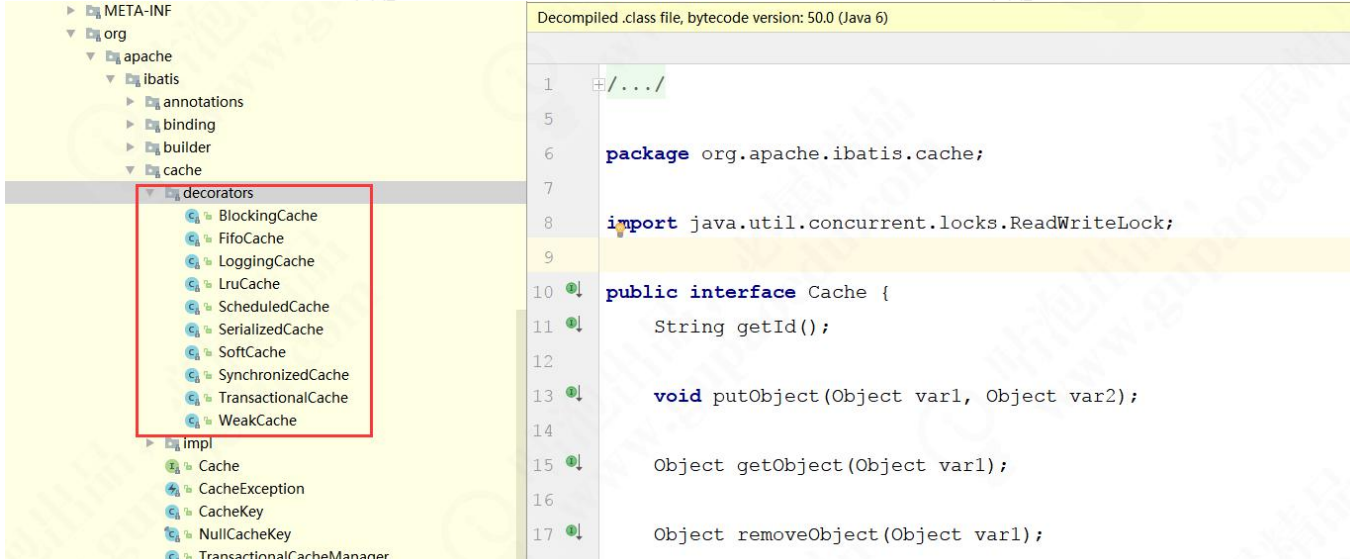