Description
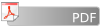
Run Length Encoding(RLE) is a simple form of compression. RLE consists of the process for searching for a repeated runs of a single character in a string to be compressed, and replacing them by a single instance of the character and a run count. For example, a stringabcccddddddefgggggggggghijk is encoded into a string ab3c6def10ghijk by RLE.
A new compression method similar to RLE is devised and the rule of the method is as follows: if a substring S<tex2html_verbatim_mark> is repeated k<tex2html_verbatim_mark> times, replace k<tex2html_verbatim_mark> copies of S<tex2html_verbatim_mark> by k(S)<tex2html_verbatim_mark> . For example, letsgogogo is compressed into lets3(go). The length of letsgogogo is 10, and the length of lets3(go) is 9. In general, the length of k(S)<tex2html_verbatim_mark> is (number of digits in k<tex2html_verbatim_mark> ) + (length of S<tex2html_verbatim_mark> ) + 2 (for `(' and `)'). For example, the length of 123(abc) is 8. It is also possible to nest compression, so the substring S<tex2html_verbatim_mark> may itself be a compressed string. For example,nowletsgogogoletsgogogo could be compressed as a now2(lets3(go)), and nowletsgogogoletsgogogoandrunrunrun could be compressed as now2(lets3(go))and3(run).
Write a program that, for a given string, gives a shortest compressed string using the compression rules as described above.
Input
Your program is to read from standard input. The input consists of T<tex2html_verbatim_mark> test cases. The number of test cases T<tex2html_verbatim_mark> is given in the first line of the input. Each test case consists of a single line containing one string of no more than 200 characters drawn from a lower case alphabet. The length of shortest input string is 1.
Output
Your program is to write to standard output. Print exactly one line for each test case. For each test case, print the length of the shortest compressed string.
The following shows sample input and output for four test cases.
Sample Input
4 ababcd letsgogogo nowletsgogogoletsgogogo nowletsgogogoletsgogogoandrunrunrun
Sample Output
6 9 15 24

1 #include <stdio.h> 2 #include <string.h> 3 #include <algorithm> 4 using namespace std; 5 6 char s[205]; 7 int dp[205][205]; 8 9 int check(int l,int r,int k) 10 { 11 int i,j; 12 if(l>r) 13 return 0; 14 for(i=l;i<=r;i++) 15 { 16 for(j=0;i+j*k<=r;j++) 17 { 18 if(s[i]!=s[i+j*k]) 19 return 0; 20 } 21 } 22 return 1; 23 } 24 25 int digit(int a) 26 { 27 int s=0; 28 while(a) 29 { 30 s++; 31 a=a/10; 32 } 33 return s; 34 } 35 36 int main() 37 { 38 int T; 39 int i,j,k,p,len; 40 scanf("%d",&T); 41 while(T--) 42 { 43 scanf("%s",s); 44 len=strlen(s); 45 for(i=0;i<len;i++) 46 { 47 for(j=0;j<len;j++) 48 { 49 dp[i][j]=205; 50 } 51 } 52 for(i=0;i<len;i++) 53 dp[i][i]=1; 54 for(i=2;i<=len;i++) 55 { 56 for(j=0;j+i-1<len;j++) 57 { 58 int b=j,e=j+i-1; 59 for(k=b;k<e;k++) 60 { 61 dp[b][e]=min(dp[b][e],dp[b][k]+dp[k+1][e]); 62 } 63 for(p=1;p<=i/2;p++) 64 { 65 if(i%p==0) 66 { 67 if(check(b,e,p)) 68 { 69 dp[b][e]=min(dp[b][e],digit(i/p)+2+dp[b][b+p-1]); 70 } 71 } 72 } 73 } 74 } 75 printf("%d ",dp[0][len-1]); 76 } 77 return 0; 78 }