本文体验datagrid的排序。
□ 思路
当点击datagrid的标题,视图传递给Controller的Form Data类似这样:page=1&rows=10&sort=CustomerID&order=asc。为了应对变化,把关于分页的封装成基类,其他关于排序或搜索的封装成继承该基类的子类。再把这些子类对象实例传递给服务层方法。
相关Model
展开 //显示表相关 public class Customer { public int CustomerID { get; set; } public string CompanyName { get; set; } public string ContactName { get; set; } public string ContactTitle { get; set; } public string Address { get; set; } public string City { get; set; } public string Region { get; set; } public string Country { get; set; } public string Phone { get; set; } public string Fax { get; set; } } //关于分页封装成基类 public class PageParam { public int PageSize { get; set; } public int PageIndex { get; set; } } //关于搜索或排序封装成子类 public class CustomerParam : PageParam { public string SortProperty { get; set; } public bool Order { get; set; } }
服务层根据CustomerParam返回Customer集合,并返回一个输出总记录数
展开using System; using System.Collections; using System.Linq; using DataGridInMVC2.Models; using System.Collections.Generic; namespace DataGridInMVC2.Helpers { public class Service { //获取Customer列表 public IEnumerable<Customer> LoadPageCustomers(CustomerParam param, out int total) { var customers = InitializeCustomers(); total = customers.Count(); //考虑排序 //CustomerID,CompanyName,ContactName,ContactTitle,Address,Fax,Phone,City switch (param.SortProperty) { case "CustomerID": return customers.OrderByWithDirection(c => c.CustomerID, param.Order) .Skip(param.PageSize * (param.PageIndex - 1)) .Take(param.PageSize); break; case "CompanyName": return customers.OrderByWithDirection(c => c.CompanyName, param.Order) .Skip(param.PageSize * (param.PageIndex - 1)) .Take(param.PageSize); break; case "ContactName": return customers.OrderByWithDirection(c => c.ContactName, param.Order) .Skip(param.PageSize * (param.PageIndex - 1)) .Take(param.PageSize); break; case "ContactTitle": return customers.OrderByWithDirection(c => c.ContactTitle, param.Order) .Skip(param.PageSize * (param.PageIndex - 1)) .Take(param.PageSize); break; case "Address": return customers.OrderByWithDirection(c => c.Address, param.Order) .Skip(param.PageSize * (param.PageIndex - 1)) .Take(param.PageSize); break; case "Fax": return customers.OrderByWithDirection(c => c.Fax, param.Order) .Skip(param.PageSize * (param.PageIndex - 1)) .Take(param.PageSize); break; case "Phone": return customers.OrderByWithDirection(c => c.Phone, param.Order) .Skip(param.PageSize * (param.PageIndex - 1)) .Take(param.PageSize); break; case "City": return customers.OrderByWithDirection(c => c.City, param.Order) .Skip(param.PageSize * (param.PageIndex - 1)) .Take(param.PageSize); break; default: return customers.OrderByWithDirection(c => c.CustomerID, param.Order) .Skip(param.PageSize * (param.PageIndex - 1)) .Take(param.PageSize); break; } } //初始化Customer public IEnumerable<Customer> InitializeCustomers() { var customers = new List<Customer>(); for (int i = 0; i < 35; i++) { customers.Add(new Customer() { CustomerID = i + 1, CompanyName = "Company" + Convert.ToString(i + 1), ContactName = "ContactName" + Convert.ToString(i + 1), ContactTitle = "ContactTitle" + Convert.ToString(i + 1), Address = "Address" + Convert.ToString(i + 1), City = "City" + Convert.ToString(i + 1), Country = "China", Fax = "010-878789" + Convert.ToString(i + 1), Phone = "010-989876" + Convert.ToString(i + 1), Region = "Qingdao" }); } return customers; } } }
在进行分类的时候,用到了针对 IEnumerable<Customer>扩展方法OrderByWithDirection,如下:
using System.Linq;
namespace DataGridInMVC2.Helpers
{
public static class SortExtension
{
public static IOrderedEnumerable<TSource> OrderByWithDirection<TSource, TKey>(
this IEnumerable<TSource> source,
System.Func<TSource, TKey> keySelector,
bool descending)
{
return descending ? source.OrderByDescending(keySelector) : source.OrderBy(keySelector);
}
}
}
CustomerController
展开using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; using DataGridInMVC2.Helpers; using DataGridInMVC2.Models; namespace DataGridInMVC2.Controllers { public class CustomerController : Controller { public ActionResult Index() { return View(); } public ActionResult GetData() { //接收datagrid传来的参数 //page=1&rows=10&sort=CustomerID&order=asc int pageIndex = int.Parse(Request["page"]); int pageSize = int.Parse(Request["rows"]); string sortProperty = Request["sort"]; bool order = Request["order"] == "asc" ? false : true; //构建服务类方法所需要的参数实例 var temp = new CustomerParam() { PageIndex = pageIndex, PageSize = pageSize, SortProperty = sortProperty, Order = order }; var service = new Service(); int totalNum = 0; var customers = service.LoadPageCustomers(temp, out totalNum); var result = from customer in customers select new { customer.CustomerID, customer.CompanyName, customer.ContactName, customer.ContactTitle, customer.Country, customer.Region, customer.Address, customer.Fax, customer.Phone, customer.City }; var jsonResult = new {total = totalNum, rows = result}; //序列化成json字符串 string str = JsonSerializeHelper.SerializeToJson(jsonResult); return Content(str); } } }
Customer/Index 视图
展开@{ ViewBag.Title = "Index"; Layout = "~/Views/Shared/_Layout.cshtml"; } <link href="~/Content/themes/default/easyui.css" rel="stylesheet" /> <link href="~/Content/themes/icon.css" rel="stylesheet" /> <table id="tt"></table> @section scripts { <script src="~/Scripts/jquery.easyui.min.js"></script> <script src="~/Scripts/easyui-lang-zh_CN.js"></script> <script type="text/javascript"> $(function () { initData(); }); function initData(params) { $('#tt').datagrid({ url: '@Url.Action("GetData","Customer")', 730, height: 400, title: 'Customer列表', fitColumns: true, rownumbers: true, //是否加行号 pagination: true, //是否显式分页 pageSize: 15, //页容量,必须和pageList对应起来,否则会报错 pageNumber: 2, //默认显示第几页 pageList: [15, 30, 45],//分页中下拉选项的数值 columns: [[ //CustomerID,CompanyName,ContactName,ContactTitle,Country,Region,Address,Fax,Phone,City { field: 'CustomerID', title: '编号',sortable: true }, { field: 'CompanyName', title: '客户名称', sortable: true }, { field: 'ContactName', title: '联系人名称', sortable: true }, { field: 'ContactTitle', title: '职位', sortable: true }, { field: 'Address', title: '地址', sortable: true }, { field: 'City', title: '城市名称', sortable: true }, { field: 'Region', title: '区域' }, { field: 'Country', title: '国家' }, { field: 'Phone', title: '电话', sortable: true }, { field: 'Fax', title: '传真', sortable: true } ]], queryParams: params, //搜索json对象 sortName: 'CustomerID', //初始排序字段 sortOrder: 'asc' //初始排序方式 }); } </script> }
最终效果:
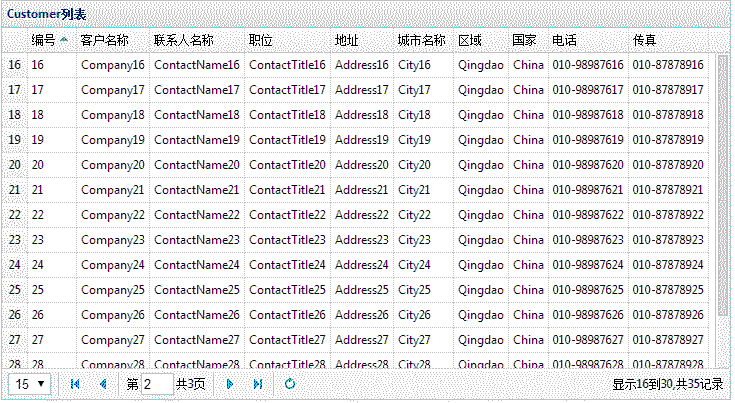