一、什么是Hibernate?
Hibernate是一个轻量级的ORMapping框架
ORMapping原理(Object Relational Mapping
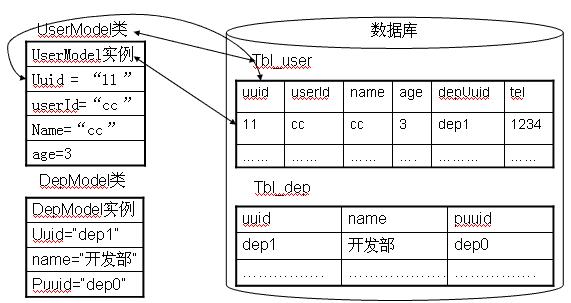
ORMapping基本对应规则:
1:类跟表相对应
2:类的属性跟表的字段相对应
3:类的实例与表中具体的一条记录相对应
4:一个类可以对应多个表,一个表也可以对应对个类
5:DB中的表可以没有主键,但是Object中必须设置主键字段
6:DB中表与表之间的关系(如:外键)映射成为Object之间的关系
7:Object中属性的个数和名称可以和表中定义的字段个数和名称不一样
二、Hibernate能干什么:
Hibernate主要用来实现Java对象和表之间的映射,除此之外还提供还提供数据查询和获取数据的方法,可以大幅度减少开发时人工使用SQL和JDBC处理数据的时间。
Hibernate的目标是对于开发者通常的数据持久化相关的编程任务,解放其中的95%。对于以数据为中心的程序来说,它们往往只在数据库中使用存储过程来实现商业逻辑,Hibernate可能不是最好的解决方案;对于那些在基于Java的中间层应用中,它们实现面向对象的业务模型和商业逻辑的应用,Hibernate是最有用的。
Hibernate可以帮助你消除或者包装那些针对特定厂商的SQL代码,并且帮你把结果集从表格式的表示形式转换到一系列的对象去。
(三)Hibernate中的对象
SessionFactory (org.hibernate.SessionFactory)
针对单个数据库映射关系经过编译后的内存镜像,是线程安全的(不可变)。 它是生成的工厂,本身要用到。
Session (org.hibernate.Session)
表示应用程序与持久储存层之间交互操作的一个单线程对象,此对象生存期很短,隐藏了连接,也是的工厂。
Transaction (org.hibernate.Transaction)
应用程序用来指定原子操作单元范围的对象,它是单线程的,生命周期很短。它通过抽象将应用从底层具体的、以及事务隔离开。
ConnectionProvider (org.hibernate.connection.ConnectionProvider)
生成连接的工厂(有连接池的作用)。它通过抽象将应用从底层的或隔离开。仅供开发者扩展/实现用,并不暴露给应用程序使用。
TransactionFactory (org.hibernate.TransactionFactory)
生成对象实例的工厂。仅供开发者扩展/实现用,并不暴露给应用程序使用。
示例如下:
数据库表:Student.sql
create table student ( studo varchar2(20), stuname varchar2(20), STUPASS varchar2(20), STUSEX VARCHAR2(2), MOBILE VARCHAR2(2), EMAIL VARCHAR2(20), ADDRESS VARCHAR2(50), STUAGE NUMBER );
配置文件:hibernate.cfg.xml 注意配置文件要写在src下 否则会加载不到
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- 数据库URL --> <property name = "connection.url">jdbc:oracle:thin:@10.103.1.204:1521:orcl</property> <!-- jdbc驱动 --> <property name ="conneciton.driver_class">oracle.jdbc.driver.OracleDriver</property> <!-- 账号 --> <property name = "connection.username">ybt</property> <!-- 密码 --> <property name = "connection.password">ybt!123</property> <!-- 每个数据库都有对应的Dialect以匹配其平台特性 --> <property name = "dialect">org.hibernate.dialect.Oracle10gDialect</property> <!-- 指定当前session范围和上下文 --> <property name = "current_session_context_class">thread</property> <!-- 指定运行期生成的SQL输出到日志以供调试 --> <property name = "show_sql">true</property> <!-- 是否格式化SQL --> <property name = "format_sql">true</property> <!-- 映射文件 --> <mapping resource="student.hbm.xml" /> </session-factory> </hibernate-configuration>
映射文件:Student.hbm.xml
?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name ="entity.student" table ="STUDENT"> <id name = "studo" type = "java.lang.String" column = "studo"> <!--generator的class类型 assigned:主键的状态 assigned表示程序生成 sequence:Oracle中的序列 identity:Sql中的自动编号 increment:先查询最大的编号再增1 uuid:生成32位长的字符串 native:根据数据库自动生成 --> <generator class="assigned"/> </id> <!-- 直接使用property属性设置 --> <property name = "stuname" type = "java.lang.String" column="stuname" length="50" not-null="true"/> <property name="stupass" type ="java.lang.String" column ="STUPASS"> <!-- 使用column设置 --> <!--测试发现colum在上层元素使用之后再使用会报错 <column name="STUPASS" length="50" not-null="true"/> --> </property> <property name="stusex" type ="java.lang.String" column ="STUSEX"/> <property name="stuage" type ="java.lang.Integer" column ="STUAGE"/> <property name="mobile" type ="java.lang.String" column ="MOBILE"/> <property name="email" type ="java.lang.String" column ="EMAIL"/> <property name="address" type ="java.lang.String" column ="ADDRESS"/> </class> </hibernate-mapping>
java代码:StudentService.java
/** * @author Administrator * */ package entity.dao; import java.util.List; import javax.transaction.HeuristicMixedException; import javax.transaction.HeuristicRollbackException; import javax.transaction.RollbackException; import javax.transaction.SystemException; import org.hibernate.HibernateException; import org.hibernate.Query; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.Transaction; import org.hibernate.cfg.Configuration; import entity.student; public class studentService{ private static Configuration conf; private static SessionFactory sf; private static Transaction tx; static{ try{ /** * Configuration()的configure()方法会默认在classpath下寻找hibernate.cnf.xml * 如果没有找到该文件,系统会打印如下信息并抛出HibernateException异常 * 其实不使用configure()方法也可以Configuration cfg =new Configuration(); * 这时hibernate会在classpath下面寻找hibernate.properties文件 * 如果没有找到该文件,系统会打印如下信息并抛出HibernateException异常。 * 使用hibernate.properties配置连接数据库时, * 还需要使用cfg.addClass()方法,把pojo类加载进来。 */ conf = new Configuration().configure(); //实例化hibernate.cnf.xml的配置 sf = conf.buildSessionFactory(); ////实例化一个session工程对象 } catch(HibernateException e){ e.printStackTrace(); } } public static Session getSession(){ return sf.openSession(); //过去老的方法,不需要使用事务 //return sf.getCurrentSession();// 新的方法,需要和事务一起使用,可以保证每个用户创建的session独立,需要在配置文件中配置 //<property name="current_session_context_class">thread</property> } /** * 获取所有学生列表 * @return */ public List GetAllStudent(){ List list = null; Session session = getSession(); if(session != null){ try{ String hql = "from student"; Query query = session.createQuery(hql); list = query.list(); }catch(HibernateException e){ e.printStackTrace(); }finally{ session.close(); } } return list; } /** * 获取单个学生信息 * @param studo * @return */ public student GetStudentBystudo(String studo){ student stu = null; Session session = getSession(); if(session != null){ try{ // get如果没有查询到数据,则返回null // stu = (Student) session.get(Student.class, stuNo); stu = (student) session.load(student.class, studo);// load如果没有查询到数据,则抛出异常 }catch(HibernateException e){ e.printStackTrace(); }finally{ session.close(); } } return stu; } /** * 添加一个学生 * @param stu * @throws SystemException * @throws HeuristicRollbackException * @throws HeuristicMixedException * @throws RollbackException * @throws IllegalStateException * @throws SecurityException */ public boolean AddStudent(student stu) throws SecurityException, IllegalStateException, RollbackException, HeuristicMixedException, HeuristicRollbackException, SystemException{ boolean result = false; Session session = getSession(); if(session != null){ try{ tx = (Transaction) session.beginTransaction(); //开启一个事务 session.save(stu); //保存 tx.commit(); //提交事务 result = true; }catch(HibernateException e){ e.printStackTrace(); tx.rollback(); //如果报错 则回滚 }finally{ session.close(); } } return result; } /** * 更新一个学生 这里只举一个更新手机号的例子 * @param stu * @throws SystemException * @throws HeuristicRollbackException * @throws HeuristicMixedException * @throws RollbackException * @throws IllegalStateException * @throws SecurityException */ public boolean UpdateStudent(String studo,String newMobile) throws SecurityException, IllegalStateException, RollbackException, HeuristicMixedException, HeuristicRollbackException, SystemException{ boolean result = false; Session session = getSession(); if(session != null){ try{ // 开启一个事务 tx = (Transaction) session.beginTransaction(); // 获取一个学生对象 student stu = (student) session.load(student.class, studo); // 更新某个属性 stu.setMobile(newMobile); // 提交事务 tx.commit(); result = true; }catch(HibernateException e){ e.printStackTrace(); tx.rollback(); //更新失败需要回滚 }finally{ session.close(); } } return result; } }
test.java
/** * @author Administrator * */ package text; import java.util.List; import javax.transaction.HeuristicMixedException; import javax.transaction.HeuristicRollbackException; import javax.transaction.RollbackException; import javax.transaction.SystemException; import entity.student; import entity.dao.studentService; public class textHibernateSQL{ public static void main(String []args) throws SecurityException, IllegalStateException, RollbackException, HeuristicMixedException, HeuristicRollbackException, SystemException{ //添加两个学生 //AddStudent(); 成功 //查出全部的学生 //getAllStudent(); 成功 //查询一个学生 getStudentById(); //更新一个学生 //UpdateStudent(); 成功 } private static void UpdateStudent() throws SecurityException, IllegalStateException, RollbackException, HeuristicMixedException, HeuristicRollbackException, SystemException { studentService ss = new studentService(); String studo = "201205457"; String newMobile = "00"; ss.UpdateStudent(studo, newMobile); } private static void getStudentById() { studentService ss = new studentService(); String no = "201205457"; student st = ss.GetStudentBystudo(no); System.out.println(st.getAddress()); } private static void getAllStudent() { studentService ss = new studentService(); List<student> list = ss.GetAllStudent(); for(student st: list){ System.out.println(st.getStuname()+" "+st.getStuage()); } } private static void AddStudent() throws SecurityException, IllegalStateException, RollbackException, HeuristicMixedException, HeuristicRollbackException, SystemException { studentService ss = new studentService(); student st = new student(); st.setStuname("大伟哥"); st.setAddress("天津南开"); st.setEmail("xxx@qq.com"); st.setMobile("12"); st.setStuage(24); st.setStudo("201205457"); st.setStupass("WHAT"); st.setStusex("1"); ss.AddStudent(st); } }
说明:
1:SessionFactory sf = new Configuration().configure().buildSessionFactory();这句话的意思是读取hibernate.cfg.xml,创建Session工厂,是线程安全的。
默认是”hibernate.cfg.xml”,不用写出来,如果文件名不是”hibernate.cfg.xml”,那么需要显示指定,如下:
SessionFactory sf = new Configuration(). configure( “javass.cfg.xml” ).buildSessionFactory();
2:Session是应用程序主要使用的Hibernate接口,约相当于JDBC的Connection+Statement/PreparedStatement的功能,是线程不安全的
3:这里使用的事务Transaction是Hibernate的Transaction,需要有,不能去掉。