选择器控件UIPickerView:
@protocol UIPickerViewDataSource, UIPickerViewDelegate;
@interface UIPickerView : UIView <NSCoding,
@property(nonatomic,assign) id<UIPickerViewDataSource> dataSource; // default is nil,设置代理
@property(nonatomic,assign) id<UIPickerViewDelegate> delegate; // default is nil,设置代理
@property(nonatomic) BOOL showsSelectionIndicator; // default is NO
@property(nonatomic,readonly) NSInteger numberOfComponents;
二、协议UIPickerViewDataSource
@protocol UIPickerViewDataSource<NSObject>
@required //必须要实现的方法
// 返回的列显示的数量。
- (NSInteger)numberOfComponentsInPickerView:(UIPickerView *)pickerView;
//返回行数在每个组件(每一列)
- (NSInteger)pickerView:(UIPickerView *)pickerView numberOfRowsInComponent:(NSInteger)component;
@end
三、协议UIPickerViewDelegate
@protocol UIPickerViewDelegate<NSObject>
@optional //可以选择执行的方法
//每一列组件的列宽度
- (CGFloat)pickerView:(UIPickerView *)pickerView widthForComponent:(NSInteger)component;
//每一列组件的行高度
- (CGFloat)pickerView:(UIPickerView *)pickerView rowHeightForComponent:(NSInteger)component;
// 返回每一列组件的每一行的标题内容
- (NSString *)pickerView:(UIPickerView *)pickerView titleForRow:(NSInteger)row forComponent:(NSInteger)component;
// 返回每一列组件的每一行的标题内容的属性
- (NSAttributedString *)pickerView:(UIPickerView *)pickerView attributedTitleForRow:(NSInteger)row forComponent:(NSInteger)component;
// 返回每一列组件的每一行的视图显示
- (UIView *)pickerView:(UIPickerView *)pickerView viewForRow:(NSInteger)row forComponent:(NSInteger)component reusingView:(UIView *)view;
//执行选择某列某行的操作
- (void)pickerView:(UIPickerView *)pickerView didSelectRow:(NSInteger)row inComponent:(NSInteger)component;
@end
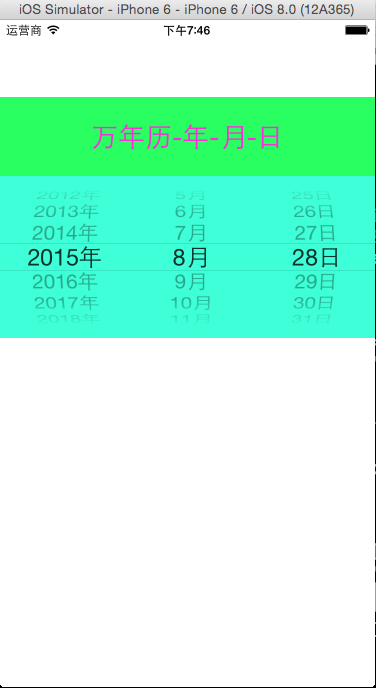
1 #import <UIKit/UIKit.h> 2 3 @interface ViewController : UIViewController<UIPickerViewDataSource,UIPickerViewDelegate> 4 @property(nonatomic,strong)NSArray *years; 5 @property(nonatomic,strong)NSArray *months; 6 @property(nonatomic,strong)NSArray *days; 7 8 @end
1 #import "ViewController.h" 2 3 @interface ViewController () 4 @property (weak, nonatomic) IBOutlet UIPickerView *pickerView; 5 6 @end 7 8 @implementation ViewController 9 10 - (void)viewDidLoad 11 { 12 [super viewDidLoad]; 13 //初始化数据 14 NSMutableArray *multYears = [NSMutableArray array];//年 15 for(int i=0; i<20; i++) 16 { 17 NSString *year = [NSString stringWithFormat:@"20%02d年",i+1]; 18 [multYears addObject:year]; 19 } 20 self.years = multYears; 21 22 NSMutableArray *multMonths = [NSMutableArray arrayWithCapacity:12];//月 23 for(int i=1; i<=12; i++) 24 { 25 NSString *month = [NSString stringWithFormat:@"%d月",i]; 26 [multMonths addObject:month]; 27 } 28 self.months = multMonths; 29 30 NSMutableArray *multDays = [NSMutableArray arrayWithCapacity:31];//日 31 for(int i=1; i<=31; i++) 32 { 33 NSString *day = [NSString stringWithFormat:@"%d日",i]; 34 [multDays addObject:day]; 35 } 36 self.days = multDays; 37 38 39 //设置pickerView的数据源和代理 40 self.pickerView.dataSource = self; 41 self.pickerView.delegate = self; 42 43 //显示当前日期 44 NSDate *now = [NSDate date]; 45 //分解日期 46 NSCalendar *calendar = [[NSCalendar alloc]initWithCalendarIdentifier:NSCalendarIdentifierGregorian]; 47 48 NSCalendarUnit unitFlags = NSCalendarUnitYear|NSCalendarUnitMonth|NSCalendarUnitDay; 49 NSDateComponents *components = [calendar components:unitFlags fromDate:now]; 50 51 52 //设置pickerView显示当前日期 53 NSInteger year = [components year]; 54 [self.pickerView selectRow:year-2000-1 inComponent:0 animated:year]; 55 56 NSInteger month = [components month]; 57 [self.pickerView selectRow:month-1 inComponent:1 animated:month]; 58 59 NSInteger day = [components day]; 60 [self.pickerView selectRow:day-1 inComponent:2 animated:day]; 61 } 62 63 #pragma mark - pickerView的代理方法 64 -(NSInteger)numberOfComponentsInPickerView:(UIPickerView *)pickerView 65 { 66 return 3; 67 } 68 -(NSInteger)pickerView:(UIPickerView *)pickerView numberOfRowsInComponent:(NSInteger)component 69 { 70 NSInteger row = 0; 71 switch (component) 72 { 73 case 0: 74 row = self.years.count; 75 break; 76 case 1: 77 row = self.months.count; 78 break; 79 case 2: 80 row = self.days.count; 81 break; 82 } 83 return row; 84 } 85 86 -(NSString*)pickerView:(UIPickerView *)pickerView titleForRow:(NSInteger)row forComponent:(NSInteger)component 87 { 88 NSString *title; 89 switch (component) 90 { 91 case 0: 92 title = self.years[row]; 93 break; 94 case 1: 95 title = self.months[row]; 96 break; 97 case 2: 98 title = self.days[row]; 99 break; 100 } 101 return title; 102 } 103 -(void)pickerView:(UIPickerView *)pickerView didSelectRow:(NSInteger)row inComponent:(NSInteger)component 104 { 105 NSString *strDate = [NSString stringWithFormat:@"%@-%@-%@", 106 self.years[[pickerView selectedRowInComponent:0]], 107 self.months[[pickerView selectedRowInComponent:1]], 108 self.days[[pickerView selectedRowInComponent:2]]]; 109 NSLog(@"%@",strDate); 110 } 111 @end
演示二:制作简单的字体表,包括字体类型、大小、颜色
源码如下:
1 #import <UIKit/UIKit.h> 2 3 @interface ViewController : UIViewController<UIPickerViewDataSource,UIPickerViewDelegate> 4 @property(nonatomic,strong) NSArray *fontNames; 5 @property(nonatomic,strong) NSArray *fontSizes; 6 @property(nonatomic,strong) NSArray *fontColors; 7 @end
1 #import "ViewController.h" 2 3 @interface ViewController () 4 @property (weak, nonatomic) IBOutlet UILabel *label; 5 @property (weak, nonatomic) IBOutlet UIPickerView *pickerView; 6 7 8 @end 9 10 @implementation ViewController 11 12 - (void)viewDidLoad { 13 [super viewDidLoad]; 14 //初始化数据 15 self.fontNames = [UIFont familyNames];//字体名字 16 17 NSMutableArray *mutsize = [NSMutableArray arrayWithCapacity:20];//字体大小 18 for(int i=1; i<=30; i++) 19 { 20 [mutsize addObject:[NSString stringWithFormat:@"%d",10+i]]; 21 } 22 self.fontSizes = mutsize; 23 24 self.fontColors = @[ 25 @{@"name":@"红色",@"color":[UIColor redColor]}, 26 @{@"name":@"黑色",@"color":[UIColor blackColor]}, 27 @{@"name":@"蓝色",@"color":[UIColor blueColor]}, 28 @{@"name":@"黄色",@"color":[UIColor yellowColor]}, 29 @{@"name":@"绿色",@"color":[UIColor greenColor]} 30 ]; 31 //设置pickerView的代理和数据源 32 self.pickerView.dataSource = self; 33 self.pickerView.delegate = self; 34 35 //设置label的font 36 self.label.font = [UIFont fontWithName:self.fontNames[0] size:[self.fontSizes[0] integerValue]]; 37 38 self.label.textColor = [self.fontColors[0] objectForKey:@"color"]; 39 } 40 41 #pragma mark - pickerView的代理方法 42 -(NSInteger)numberOfComponentsInPickerView:(UIPickerView *)pickerView 43 { 44 return 3; 45 } 46 -(NSInteger)pickerView:(UIPickerView *)pickerView numberOfRowsInComponent:(NSInteger)component 47 { 48 NSInteger row = 0; 49 switch (component) 50 { 51 case 0: 52 row = self.fontNames.count; 53 break; 54 case 1: 55 row = self.fontSizes.count; 56 break; 57 case 2: 58 row = self.fontColors.count; 59 break; 60 } 61 return row; 62 } 63 -(NSString*)pickerView:(UIPickerView *)pickerView titleForRow:(NSInteger)row forComponent:(NSInteger)component 64 { 65 NSString *title; 66 switch (component) 67 { 68 case 0: 69 title = self.fontNames[row]; 70 break; 71 case 1: 72 title = self.fontSizes[row]; 73 break; 74 case 2: 75 title = [self.fontColors[row] objectForKey:@"name"]; 76 break; 77 } 78 return title; 79 } 80 //设置每一列的宽度 81 -(CGFloat)pickerView:(UIPickerView *)pickerView widthForComponent:(NSInteger)component 82 { 83 CGFloat width = 0.0f; 84 switch (component) 85 { 86 case 0: 87 width = pickerView.frame.size.width/2; 88 break; 89 case 1: 90 width = pickerView.frame.size.width/4; 91 break; 92 case 2: 93 width = pickerView.frame.size.width/4; 94 break; 95 } 96 return width; 97 } 98 99 -(void)pickerView:(UIPickerView *)pickerView didSelectRow:(NSInteger)row inComponent:(NSInteger)component 100 { 101 //设置label的font 102 self.label.font = [UIFont fontWithName:self.fontNames[[pickerView selectedRowInComponent:0]] size:[self.fontSizes[[pickerView selectedRowInComponent:1]] integerValue]]; 103 104 self.label.textColor = [self.fontColors[[pickerView selectedRowInComponent:2]] objectForKey:@"color"]; 105 } 106 @end
演示三:制作简单的图库浏览器
源码如下:
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController<UIPickerViewDelegate,UIPickerViewDataSource>
@end
1 #import "ViewController.h" 2 3 @interface ViewController () 4 @property (weak, nonatomic) IBOutlet UIPickerView *pickerView; 5 @property (strong,nonatomic)NSArray *imageNames; 6 @end 7 8 @implementation ViewController 9 10 - (void)viewDidLoad { 11 [super viewDidLoad]; 12 //初始化数据 13 NSMutableArray *mutImageNames = [NSMutableArray arrayWithCapacity:9]; 14 for(int i=0; i<9; i++) 15 { 16 [mutImageNames addObject:[NSString stringWithFormat:@"%d.png",i]]; 17 } 18 self.imageNames = mutImageNames; 19 20 //设置pickerView的数据源和代理 21 self.pickerView.dataSource = self; 22 self.pickerView.delegate = self; 23 24 } 25 #pragma mark - pickerview代理方法 26 -(NSInteger)numberOfComponentsInPickerView:(UIPickerView *)pickerView 27 { 28 return 1; 29 } 30 -(NSInteger)pickerView:(UIPickerView *)pickerView numberOfRowsInComponent:(NSInteger)component 31 { 32 return self.imageNames.count; 33 } 34 //设置行高 35 -(CGFloat)pickerView:(UIPickerView *)pickerView rowHeightForComponent:(NSInteger)component 36 { 37 UIImage *image = [UIImage imageNamed:self.imageNames[0]]; 38 return image.size.height; 39 } 40 //设置每行显示的视图 41 -(UIView *)pickerView:(UIPickerView *)pickerView viewForRow:(NSInteger)row forComponent:(NSInteger)component reusingView:(UIView *)view 42 { 43 UIImage *image = [UIImage imageNamed:self.imageNames[row]]; 44 //UIImageView *imageView = [[UIImageView alloc]initWithImage:image]; 45 //imageView.frame = CGRectMake(0, 0, image.size.width, image.size.height); 46 //return imageView; 47 48 UIButton *button = [[UIButton alloc]initWithFrame:CGRectMake(0, 0,image.size.width, image.size.height)]; 49 [button setBackgroundImage:image forState:UIControlStateNormal]; 50 [button addTarget:self action:@selector(buttonClicked:) forControlEvents:UIControlEventTouchUpInside]; 51 return button; 52 } 53 -(void)buttonClicked:(UIButton *)sender 54 { 55 NSLog(@"button clicked"); 56 } 57 @end