1 //冒泡排序 2 void BubbleSort(int array[], int n) 3 { 4 int i, j; 5 for (i = 0; i < n-1; ++i) 6 { 7 for (j = 0; j < n - i - 1; ++j) 8 { 9 if (array[j] > array[j + 1])//当前者大于后者为真时是从小到大排序,若前者小于后者为真时是从大到小排序 10 { 11 //swap(array[j],array[j+1];//位置交换使用swap()函数最好提前定义该函数; 12 int temp = array[j]; 13 array[j] = array[j + 1]; 14 array[j + 1] = temp; 15 } 16 } 17 } 18 }
快速排序动态图
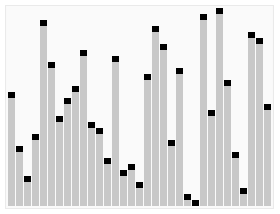
1 //快速排序01 2 void InsertSort(int array[], int left,int right) 3 { 4 int i = left; 5 int j = right; 6 int temp = array[left]; 7 if (left > right) 8 return; 9 while (i < j) 10 { 11 while (i < j && array[j] >= temp) 12 { 13 j--; 14 } 15 swap(array[i], array[j]);//将比枢轴移小的数据移至低端 16 while (i < j && array[i] <= temp) 17 { 18 i++; 19 } 20 swap(array[i], array[j]);//将比枢轴移大的数据移至搞端 21 } 22 InsertSort(array, left, i-1);//对低子表递归 23 InsertSort(array, i+1, right);//对高子表递归 24 }
1 //快速排序02 2 void InsertSort(int array[], int left,int right) 3 { 4 int i = left; 5 int j = right; 6 int temp = array[left]; 7 if (left > right) 8 return; 9 while (i < j) 10 { 11 while (i < j && array[j] >= temp) 12 { 13 --j; 14 } 15 while (i < j && array[i] <= temp) 16 { 17 ++i; 18 } 19 swap(array[i], array[j]);//左侧比枢轴大的数据与右侧比枢轴小的数据交换位置 20 } 21 swap(array[left], array[i]);//将左侧枢轴交换至中间合适位置 22 InsertSort(array, left, i-1);//对低子表递归 23 InsertSort(array, i+1, right);//对高子表递归 24 }
1 int main() 2 { 3 int ch[] = { 2,4,6,8,3,5,7,9,0,1 }; 4 InsertSort(ch, 0, 9); 5 int length = sizeof(ch) / sizeof(int); 6 for (int i = 0; i < length; ++i) 7 { 8 std::cout << ch[i] << " "; 9 } 10 return 0; 11 }
冒泡排序时间复杂度为o(n2);