GUI英文全称是Graphical User Interface,中文为图形用户接口。
tkinter是一个开放源码的图形接口开发工具,在安装Python时,就已经同时安装此模块了,在使用前只需要导入即可。
import tkinter print(tkinter.TkVersion)
8.6
1. 建立窗口
from tkinter import * root = Tk() root.mainloop()
通常将使用Tk()方法建立的窗口称为根窗口,默认名称是tk,之后可以在此根窗口中建立许多控件,也可以在此根窗口中建立上层窗口。mainloop()方法让程序继续执行,同时进入等待与处理窗口事件,单击窗口的"关闭"按钮,此程序才会结束。
与窗口相关的方法:
方法 | 说明 |
title() | 可以设置窗口的标题 |
geometry("widthxheight+x+y") | 设置窗口宽width与高height,单位是像素,设定窗口位置 |
maxsize(width,height) | 拖曳时窗口最大的宽和高 |
minsize(width,height) | 拖曳时窗口最小的宽和高 |
configure(bg="color") | 设置窗口的背景颜色 |
resizable(True,Ture) | 可设置是否可更改窗口大小,第一个参数是宽,第二个参数是高,如果要固定窗口宽与高,可以使用resizable(0,0) |
state("zoomed") | 最大化窗口 |
iconify() | 最小化窗口 |
iconbitmap("xx.ico") | 更改默认窗口图标 |
root = Tk() root.title("MyWindow") root.geometry("300x160+400+200") root.configure(bg="#33ff33") root.iconbitmap("mystar.ico") root.mainloop()
geometry中width和height用x分隔,表示窗口的宽和高,+x是窗口左边距离屏幕左边的距离,如果是-x则是表示窗口右边距离屏幕右边的距离,同理,+y或-y表示窗口上边(下边)距离屏幕上边(下边)的距离。
在tkinter模块中可以使用下列方法获得屏幕的宽度和高度:
- winfo_screenwidth():屏幕宽度;
- winfo_screenheight():屏幕高度。
下面的程序将窗口显示在屏幕中央。
root = Tk() screenWidth = root.winfo_screenwidth() screenHeight = root.winfo_screenheight() w = 300 h = 160 x = (screenWidth - w) / 2 y = (screenHeight -h) / 2 root.geometry("%dx%d+%d+%d" % (w,h,x,y)) root.mainloop()
2. tkinter的Widget
Widget可以翻译为控件或组件或部件。窗口建立完成后,下一步就是在窗口内建立控件。
控件
- Button:按钮;
- Canvas:画布;
- Checkbutton:多选按钮;
- Entry:文本框;
- Frame:框架;
- Label:标签;
- LabelFrame:标签框架;
- Listbox:列表框;
- Menu:菜单;
- Message:消息;
- OptionMenu:下拉菜单;
- PanedWindow:面板;
- Radiobutton:单选按钮;
- Scale:尺度;
- Scrolbar:滚动条;
- Spinbox:可微调输入控件;
- Text:文字区域;
- Toplevel:上层窗口。
加强版模块tkinter.ttk中新增的Widget:
- Combobox;
- Notebook;
- Progressbat;
- Separator;
- Sizegrip;
- Treeview。
Widget的共同属性:
- Dimensions:大小;
- Colors:颜色;
- Fonts:字体;
- Anchor:锚(位置参考点);
- Relief styles:属性边框;
- Bitmaps:显示位图;
- Cursors:鼠标外形。
Widget的共同方法:
- Configuration
- config(option=value):Widget属性可以在建立时设置,也可以在程序执行时使用config()重新设置;
- cget("option"):取得option参数值;
- keys():可以用此方法获得所有该Widget的参数。
- Event Processing
- mainloop():让程序继续执行,同时进入等待与处理窗口事件;
- quit():Python Shell窗口结束,但是所建立的窗口继续执行;
- update():更新窗口画面。
- Event callbacks
- bind(event,callback):事件绑定;
- unbind(event):接触绑定。
- Alarm handlers
- after(time,callback):间隔指定时间后调用callback()方法。
3. 标签
Label()方法用于在窗口内建立文字或图像标签。
Label(父对象,options,…)
常用options参数:
- anchor:如果空间大于所需时,控制标签的位置,默认是CENTER(居中);√ 3.2
- bg或background:背景颜色;√ 3.1
- bitmap:使用默认图标当做标签内容;√ 3.6
- borderwidth或bd:标签边界宽度,默认为1;
- compound:可以设置标签内含图形和文字时,彼此的位置关系;√ 3.7
- cursor:当鼠标光标在标签上方时的外形;√ 3.12
- fg或foreground:前景颜色;√ 3.1
- font:可选择字形、字形样式与大小;√ 3.4
- height:标签高度,单位是字符;√ 3.1
- image:标签以图形形式呈现;√ 3.10
- justify:存在多行文本时最后一行的对齐方式,可取left/center(默认)/right;√ 3.5
- padx/pady:标签文字与标签区间的间距,单位是像素;√ 3.9
- relief:默认为FLASE,可控制标签的外框;√ 3.8
- text:标签文字内容,使用 可输入多行;√ all
- textvariable:可以设置标签以变量的形式显示;
- underline:可以设置第几个文字有下划线,从0开始,默认为-1,表示无下划线;
- width:标签宽度,单位是字符;√ 3.1
- wraplength:文本到多少宽度后换行,单位是像素。√ 3.3
3.1 Color、Dimensions
Widget的共同属性。
bg、fg设置背景、前景色。
width、height的单位是字符。
root = Tk() root.title("Ex") root.geometry("200x80") label = Label(root, text = "I like tkinter", bg = "#EF72AA", fg = "white", height = 3, width = 20) label.pack() #包装与定位组件 root.mainloop()
3.2 Anchor
Widget的共同属性。
Anchor是指标签文字在标签区域的输出位置。
比如nw效果如下:
anchor的参数设置也可以使用NW、N、NE、W等大写常数,同时省略字符串的双引号。
3.3 Wraplength
wraplength = 35
效果如下:
3.4 Font
Widget的共同属性。
用于设置文字字形。
- family:字形,如Helvetica、Times等;
- size:字号,单位是像素;
- weight:如bold、normal;
- slant:如italic、roman;
- underline:True、False;
- overstrike:True、False。
root = Tk() root.title("Ex") label = Label(root, text = "I like tkinter", bg = "#EF72AA", fg = "white", height = 3, width = 20, font = "Helnetic 20 bold italic") # font = ("Helnetic", 20, "bold", "italic")) label.pack() #包装与定位组件 root.mainloop()
height和width都是字号联动的。
3.5 Justify
justify = "right"
3.6 Bitmaps
Widget的共同属性。
在标签位置放置内建位图。
error、hourglass、info、questhead、question、warning、gray12、gray25、gray50、gray75
label = Label(root, bitmap = "question")
3.7 Compound
图像与文字共存时,使用此参数定义文字与图像的位置关系。
- left:图像在左;
- right:图像在右;
- top:图像在上;
- bottom:图像在下;
- center:文字覆盖在图像上方。
label = Label(root, bitmap = "question", text = "Question",
compound = "left")
3.8 Relief
Widget的共同属性。
用于建立Widget的边框。
label = Label(root, text = "raised",
relief = "raised")
3.9 Padx/Pady
padx用于设置标签文字左右边界与标签区间的x轴间距,pady用于设置文字上下边界与标签取件单y轴间距。
与width和height作用相似,可以互相替代。
root.geometry("300x100")
label = Label(root, text = "raised",
bg = "lightyellow", relief = "raised",
padx = 5, pady = 10)
3.10 Image
图片可以应用在许多地方,例如标签、功能按钮、选项按钮文字区域等。在使用前可以使用PhotoImage()方法建立图像对象,然后再将此对象应用在其他窗口组件上。
imageobj = PhotoImage(file = "xxx.gif")
png也可。
image_obj = PhotoImage(file = "mystar.png")
label = Label(root, image = image_obj)
jpg无法直接解析:
需要借助PIL模块。
from tkinter import * from PIL import Image, ImageTk root = Tk() root.title("Ex") image_obj = Image.open("scenery.jpg") jpg_obj = ImageTk.PhotoImage(image_obj) label = Label(root, image = jpg_obj) label.pack() #包装与定位组件 root.mainloop()
注意:bitmap和image不能共存。
3.11 Cursors
Widget的共同属性。
表示光标形状,实际形状可能会因操作系统不同而有所差异。
3.12 keys()
Widget的共同方法。
可以以列表的形式传回Widget的所有参数。
root = Tk() root.title("Ex") label = Label(root, text = "tkinter") label.pack() print(label.keys())
['activebackground', 'activeforeground', 'anchor', 'background', 'bd', 'bg', 'bitmap', 'borderwidth', 'compound', 'cursor', 'disabledforeground', 'fg', 'font', 'foreground', 'height', 'highlightbackground', 'highlightcolor', 'highlightthickness', 'image', 'justify', 'padx', 'pady', 'relief', 'state', 'takefocus', 'text', 'textvariable', 'underline', 'width', 'wraplength']
3.13 config()
Widget的共同方法。
Widget控件在建立时可以直接设置对象属性,若是部分属性未建立,未来在程序执行时可以使用config()方法建立或更改属性。此方法内属性设置的参数用法与建立时相同。
计数器:
from tkinter import * counter = 0 def run_counter(digit): def counting(): global counter counter += 1 digit.config(text=str(counter)) digit.after(1000,counting) counting() root = Tk() root.title("Ex") digit = Label(root, bg = "yellow", height = 3, width = 10, font = "Helvetic 20 bold") digit.pack() run_counter(digit) root.mainloop()
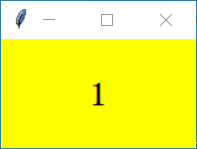
3.14 Separator
用于添加分隔线。
Separator(父对象,options)
options为HORIZONTAL表示建立水平分隔线,VERTICAL表示建立垂直分隔线。
from tkinter import * from tkinter.ttk import Separator root = Tk() root.title("Ex") myTitle = "我喜欢Tkinter" myContent = """今天,我开始学习Tkinter。 Tkinter 是 Python 的标准 GUI 库, Python 使用 Tkinter 可以快速的创建 GUI 应用程序。""" label1 = Label(root, text = myTitle, font = "Helvetic 20 bold") label1.pack(padx = 10, pady = 10) sep = Separator(root, orient = HORIZONTAL) sep.pack(fill = X, padx = 5) label2 = Label(root, text = myContent) label2.pack(padx = 10, pady = 10) root.mainloop()
4. 窗口控件配置管理员
4.1 简介
Widget Layout Manager用于将多个Widget控件配置到容器或窗口内,有3中方法:
- pack;
- grid;
- place。
4.2 pack
最常用的控件配置管理员,使用相对位置类处理控件,至于控件的正确位置则是由pack方法自动完成的。
pack(options,…)
-after, -anchor, -before, -expand, -fill, -in, -ipadx, -ipady, -padx, -pady, -side
1. side
用于水平或垂直配置控件。
- TOP:默认值,从上到下排列;
- BOTTOM:从下到上排列;
- LEFT:从左到右排列;
- RIGHT:从右到左排列。
root = Tk() root.title("Ex") label1 = Label(root, text = "哈尔滨工业大学", bg = "lightyellow", width = 30) label2 = Label(root, text = "哈尔滨工程大学", bg = "lightgreen", width = 15) label3 = Label(root, text = "东北林业大学", bg = "lightblue", width = 15) label1.pack(side = BOTTOM) label2.pack(side = RIGHT) label3.pack(side = LEFT) root.mainloop()
列出所有的relief属性:
root = Tk() root.title("Ex") Reliefs = ["flat", "groove", "raised" ,"ridge", "solid", "sunken"] for Relief in Reliefs: Label(root, text = Relief, relief = Relief, fg="darkgreen", font = "Times 20 bold").pack(side = LEFT, padx = 5) root.mainloop()
列出所有的位图:
root = Tk() root.title("Ex") bitMaps = ["error", "hourglass", "info", "questhead", "question", "warning", "gray12", "gray25", "gray50", "gray75"] for bitMap in bitMaps: Label(root, bitmap = bitMap).pack(side = LEFT, padx = 5) root.mainloop()
2. padx/pady
设定控件边界与容器或其他控件边界之间的距离。默认为1。
3. ipadx/ipady
控制文字与标签容器的x/y轴间距。
4. anchor
设定Widget控件在窗口中的位置
root = Tk() root.title("Ex") root.geometry("300x180") label1 = Label(root, text = "Next", font = "Times 15 bold", fg = "white", bg = "blue") label2 = Label(root, text = "Previous", font = "Times 15 bold", fg = "white", bg = "red") label1.pack(anchor = S, side = RIGHT, padx = 10, pady = 10) label2.pack(anchor = S, side = RIGHT, padx = 10, pady = 10) root.mainloop()
5. fill
设置控件填满所分配容器的方式。
- fill = X:填满所分配空间的X轴不留白;
- fill = Y:填满Y轴不留白;
- fill = BOTH:填满X轴和Y轴;
- fill = NONE:默认值,保持原大小。
如果所分配容器区间已经满了,则使用fill参数不会有任何作用。
root = Tk() root.title("Ex") label1 = Label(root, text = "哈尔滨工业大学", bg = "lightyellow") label2 = Label(root, text = "哈尔滨工程大学", bg = "lightgreen") label3 = Label(root, text = "东北林业大学", bg = "lightblue") label1.pack(side = LEFT, fill = Y) label2.pack() label3.pack(fill = X) root.mainloop()
6. expend
设定Widget控件是否填满额外的父容器界面,默认为False。
label2.pack(fill = BOTH, expand = True)
7. pack的方法
pack其实在tkinter中也是一个类别,有以下方法可供使用:
- slaves():传回所有的Widget控件对象;
- info():传回pack选项的对应值;
- forget():隐藏Widget控件,可以用pack(opions,…)复原显示;
- location(x,y):传回此点是否在单元格,如果是传回坐标,如果不是传回(-1,-1);
- size():传回Widget的控件大小;
- propagate():参数是True表示父窗口大小由子控件决定,默认为True。
print(root.pack_slaves())
[<tkinter.Label object .!label>, <tkinter.Label object .!label2>, <tkinter.Label object .!label3>]
4.3 grid
这是一种以格状或者类似Excel表格方式包装和定位窗口组件的方法。
grid(opions,…)
-column, -columnspan, -in, -ipadx, -ipady, -padx, -pady, -row, -rowspan, -sticky
1. row、column
label1.grid(row = 0, column = 0)
label2.grid(row = 1, column = 2)
label3.grid(row = 2, column = 1)
2. rowspan、columnspan
可以设定row或column方向的合并数量。
3. padx/pady
与pack方法中的参数相同。
4. sticky
类似于anchor,只能设定N/S/W/E,即上/下/左/右对齐,但可以组合使用。
- N+W:左上角;
- N+E:右上角;
- S+W:左下角;
- S+E:右下角;
- N+S:拉长高度让控件在顶端和底端对齐;
- W+E:拉长宽度让控件在左边和右边对齐;
- N+S+W:顶端和底端对齐,切齐左边;
- N+S+E:顶端和底端对齐,切齐右边;
- W+E+N:左边和右边对齐,切齐顶端;
- W+E+S:左边和右边对齐,切齐底端;
- N+S+W+E:拉伸平铺。
5. rowconfigure()、columnconfigure()
用于设定某行或列在窗口缩放大小时的缩放比例。
rowconfigure(0, weight = 1) #row 0 的控件在窗口改变大小时缩放比是1
columnconfigure(0, weight = 1) #column 0 的控件在窗口改变大小时缩放比是1
root = Tk() root.title("Ex") root.rowconfigure(0,weight=1) root.columnconfigure(1,weight=1) label1 = Label(root, text = "哈尔滨工业大学", bg = "lightyellow") label2 = Label(root, text = "哈尔滨工程大学", bg = "lightgreen") label3 = Label(root, text = "东北林业大学", bg = "lightblue") label1.grid(row = 0, column = 0, sticky = N+S) label2.grid(row = 1, column = 0) label3.grid(row = 1, column = 1, sticky = W+E) root.mainloop()
6. grid方法
建立色彩标签:
root = Tk() root.title("Ex") Colors = ["red", "orange", "yellow", "green","cyan", "blue", "purple"] r = 0 for color in Colors: Label(root, text = color, relief = "groove", width = 20).grid(row = r, column = 0) Label(root, bg = color, relief = "ridge", width = 20).grid(row = r,column = 1) r += 1 root.mainloop()
4.4 place
使用直接指定方式将Widget控件放在容器中的方法。
place(options,…)
1. x/y
直接设定控件左上角位置,单位是像素。
窗口区左上角是(x=0,y=0),x向右递增,y向下递增。
2. width/height
在插入图片的同时设定图片的大小。
from PIL import Image, ImageTk root = Tk() #root=Toplevel() root.title("Ex") root.geometry("520x520") flower = ImageTk.PhotoImage(Image.open("flower.jpg")) scenery = ImageTk.PhotoImage(Image.open("scenery.jpg")) p1 = Label(root, image = flower) p2 = Label(root, image = scenery) p1.place(x=20, y=20, width = 200, height = 200) p2.place(x=240, y=240, width = 260, height = 260) root.mainloop()
3. relx/rely、relwidth/relheight
relx/rely用于设置相对于父容器的位置,relwidth/relheight用于设置相对于父容器的大小,取值为0~1.0。
5. 功能按钮Button
在窗口组件中可以设计在单击功能按钮时,执行某一个特定的动作,这个动作也称为callback方法。
Button(父对象,options,…)
常用的options参数:
- borderwidth或bd:边界宽度,默认2像素;
- bg或background:背景色彩;
- command:单击功能按钮时,执行此方法;
- cursor:当鼠标光标移至按钮上时的形状;
- fg或foreground:前景色彩;
- font:字形;
- height:高,单位是字符;
- highlightbackground:当功能按钮取得焦点时的背景色彩;
- highlightcolor:当功能按钮取得焦点时的色彩;
- image:功能按钮上的图像;
- justify:当有多行文字时,最后一行文字的对齐方式;
- padx/pady:默认为1;
- relief:默认为FLAT;
- state:默认为NORMAL,若设置为DISABLED则以灰阶显示按钮表示无法使用;
- text:功能按钮文字;
- underline:设置第几个文字有下划线;
- width:宽,单位是字符;
- wraplength:限制每行的字符数。
5.1 示例
def msgShow(): label["text"] = "I love tkinter." label["bg"] = "lightyellow" label["fb"] = "blue" label.config(text = "I love tkinter.", bg = "lightyellow", fg = "blue") root = Tk() root.title("Ex") label = Label(root) btn1 = Button(root, text = "Show Message", width = 15, command = msgShow) btn2 = Button(root, text = "Exit", width = 15, command = root.destroy) label.pack() btn1.pack(side = LEFT) btn2.pack(side = LEFT) root.mainloop()
5.2 Lambda
def bColor(bgColor): root.config(bg = bgColor) root = Tk() root.title("Ex") root.geometry("300x200") #建立3个按钮 bluebtn = Button(root, text = "Blue", command = lambda:bColor("blue")) yellowbtn = Button(root, text = "Yellow", command = lambda:bColor("yellow")) exitbtn = Button(root, text = "Exit", command = root.destroy) exitbtn.pack(anchor = S, side = RIGHT, padx = 5, pady = 5) yellowbtn.pack(anchor = S, side = RIGHT, padx = 5, pady = 5) bluebtn.pack(anchor = S, side = RIGHT, padx = 5, pady = 5) root.mainloop()
6. 文本框Entry
通常是指单行文本框,是GUI设计中用于输入的最基本的Widget控件,可以用来输入单行字符串。
如果输入的字符串长度大于文本框的宽度,输入的文字会自动隐藏造成部分内容无法显示出来,此时可以使用箭头键移动鼠标光标到看不到的区域。
如果需要处理多行文字,则需要使用Text。
Entry(父对象,options,…)
常用的options参数:
- bg,bd,cursor,fg,font,height,highlightbackground,highlightcolor,justify,relief,width;
- command:当用户更改内容时,会自动执行此函数;
- exportselection:选取的字符串自动输出至剪切板,使用exportselection = 0来避免;
- selectbackground:被选取字符串时的背景色彩;
- selectborderwidth:被选取字符串时的边界宽度,默认为1;
- selectforeground:被选取字符串时的前景色彩;
- show:显示输入字符,例如show = '*'表示显示星号,常用于输入密码;
- state:输入状态,默认NORMAL表示可以输入,DISABLE表示无法输入;
- textvariable:文字变量;
- xscrollcommand:在x轴使用滚动条。
6.1 示例
root = Tk() root.title("Ex") accountL = Label(root, text = "Account:") accountL.grid(row = 0) passwordL = Label(root, text = "Password:") passwordL.grid(row = 1) accountE = Entry(root) accountE.grid(row = 0,column = 1) passwordE = Entry(root, show = '*') passwordE.grid(row = 1, column = 1) root.mainloop()
6.2 get()
用于获取目前Entry的字符串内容。
def printInfo(): print("Account:%s Password:%s" % (accountE.get(), passwordE.get())) ... loginbtn = Button(root, text = "Login", command = printInfo) loginbtn.grid(row = 3) quitbtn = Button(root, text = "Quit", command = root.destroy) quitbtn.grid(row = 3, column = 1) root.mainloop()
Account:叮叮当当sunny
Password:123456789abcdefg
6.3 insert()
用于建立默认文字。
insert(index,s) #将字符串s插入在index位置
accountE.insert(0, "Kevin")
passwordE.insert(0, "pwd123")
6.4 delete()
用于删除文字。
delete(first,last = None) #删除从第first到第last-1字符之间的字符串(last = None则仅删除第first个字符)
delete(0,END) #删除文本框内整个字符串
6.5 eval()
用于将字符串转换为Python语句执行,可以直接计算数学表达式。
result = eval(expression) #expression是字符串
数学表达式计算:
from tkinter import * import numpy as np def cal(): out.configure(text = "结果:" + str(eval(equ.get()))) root = Tk() root.title("Ex") label = Label(root, text = "请输入数学表达式:") label.pack() equ = Entry(root) equ.pack(padx = 20, pady = 5) out = Label(root) out.pack() btn = Button(root, text = "计算", command = cal) btn.pack(pady = 5) root.mainloop()
7. 变量类型
要将Widget控件的参数以变量方式处理时,需要借助tkinter模块内的变量类别,这个类别有4个子类别,每一个类别其实是一个数据类型的构造方法,我们可以通过这4个子类别的数据类型将它们与Widget控件的相关参数结合。
- x = IntVar() #整型变量,默认是0
- x = DoubleVar() #浮点型变量,默认是0.0
- x = StringVar #字符串变量,默认是""
- x = BooleanVar() #布尔型变量
7.1 get()、set()
使用get()方法取得变量内容,使用set()方法设置变量内容。
msg_on = False def btn_hit(): global msg_on if msg_on == False: msg_on = True x.set("I like tkinter") else: msg_on = False x.set("") root = Tk() root.title("Ex") x = StringVar() label = Label(root, textvariable = x, fg = "blue", bg = "lightyellow", font = "Verdana 15 bold", width = 25, height = 2) label.pack() btn = Button(root, text = "Show", command = btn_hit) btn.pack(pady = 5) root.mainloop()
def btn_hit():
if x.get() == "":
x.set("I like tkinter")
else:
x.set("")
7.2 trace()
利用变量追踪Widget控件,当其内容更改时,让程序执行callback函数。
def callback(*args): print("data changed:",xE.get()) root = Tk() root.title("Ex") xE = StringVar() entry = Entry(root, textvariable = xE) entry.pack() xE.trace('w', callback) root.mainloop()
data changed: t
data changed: tk
data changed: tki
data changed: tkin
data changed: tkint
data changed: tkinte
data changed: tkinter
w表示当写入时,自动执行callback函数,这个动作称为变动追踪。
r表示当控件内容被读取时,执行特定函数。
def callbackW(*args): xL.set(xE.get()) def callbackR(*args): print("Waring:数据被读取!") def hit(): print("读取数据:", xE.get()) root = Tk() root.title("Ex") xE = StringVar() entry = Entry(root, textvariable = xE) entry.pack(padx = 20, pady = 5) xE.trace('w', callbackW) xE.trace('r', callbackR) xL = StringVar() label = Label(root, textvariable = xL) xL.set("同步显示") label.pack(padx = 20, pady = 5) btn = Button(root, text = "读取", command = hit) btn.pack(padx = 20, pady = 5) root.mainloop()
Waring:数据被读取!
读取数据: tkinter
callback函数其实有3个参数:tk变量名称、index索引、mode模式。
7.3 计算器
def calculate(): r = re.findall(".+", equ.get()) result = eval(str(r[-1])) equ.set(equ.get() + " " + str(result)) def show(buttonString): content = equ.get() if content == "0": content = "" equ.set(content + buttonString) def backspace(): equ.set(str(equ.get()[:-1])) def clear(): equ.set("0") root = Tk() root.title("calculator") equ = StringVar() equ.set("0") #默认显示0 #显示区 label = Label(root, width = 25, height = 2, relief = "raised", anchor = SE, textvariable = equ) label.grid(row = 0, column = 0, columnspan = 4, padx = 5, pady = 5) #按钮设计 Button(root, text = "C", fg = "blue", width = 5, command = clear).grid(row = 1, column = 0) Button(root,text="DEL",width=5,command=backspace).grid(row=1,column=1) Button(root,text="%",width=5,command=lambda:show("%")).grid(row=1,column=2) Button(root,text="/",width=5,command=lambda:show("/")).grid(row=1,column=3) Button(root,text="7",width=5,command=lambda:show("7")).grid(row=2,column=0) Button(root,text="8",width=5,command=lambda:show("8")).grid(row=2,column=1) Button(root,text="9",width=5,command=lambda:show("9")).grid(row=2,column=2) Button(root,text="*",width=5,command=lambda:show("*")).grid(row=2,column=3) Button(root,text="4",width=5,command=lambda:show("4")).grid(row=3,column=0) Button(root,text="5",width=5,command=lambda:show("5")).grid(row=3,column=1) Button(root,text="6",width=5,command=lambda:show("6")).grid(row=3,column=2) Button(root,text="-",width=5,command=lambda:show("-")).grid(row=3,column=3) Button(root,text="1",width=5,command=lambda:show("1")).grid(row=4,column=0) Button(root,text="2",width=5,command=lambda:show("2")).grid(row=4,column=1) Button(root,text="3",width=5,command=lambda:show("3")).grid(row=4,column=2) Button(root,text="+",width=5,command=lambda:show("+")).grid(row=4,column=3) Button(root, text="0", width=12, command = lambda:show("0")).grid(row = 5, column = 0, columnspan = 2) Button(root, text=".", width=5, command = lambda:show(".")).grid(row = 5, column = 2) Button(root, text="=", bg = "lightyellow", width=5, command = calculate).grid(row = 5, column = 3) root.mainloop()