记录一次简易集成Redis缓存 自定义Redisconfig配置 自定义序列化操作 加深印像
整合前提工具环境准备:
1、redis官网 https://redis.io/download
下载安装redis
运行成功
注意事项:
1,开启远程服务 放行防火墙 6379 端口 具体操作百度
2,配置
本地连接ip 就是127.0.0.1
如果在服务器端 要注释这行
3.配置 密码
还是在这这个文件redis.windows.conf 里面查找
requirepass
4.
再把protected-mode=yes
的yes
改为no
,
下载Redis视图软件 http://www.pc6.com/softview/SoftView_450180.html#download
springboot整合redis开始
第一步配置导包
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> </dependency>
第二步
实体类的配置
Redis读取数据都需要经过反序列化 不然读取失败 出错
要使用redis,实体类必须实现序列化接口
implements Serializable
否则会抛java.io.NotSerializableException
异常。
反序列化读取Json数据
@JsonIgnoreProperties(ignoreUnknown = true)
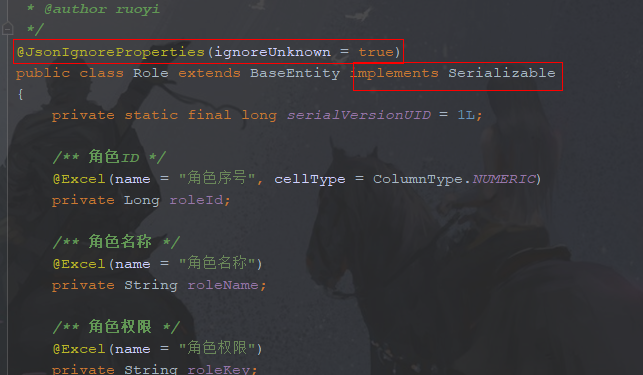
配置反序列化类
FastJson2JsonRedisSerializer
代码如下
package com.ruoyi.project.system.role.controller; import com.alibaba.fastjson.JSON; import com.alibaba.fastjson.parser.ParserConfig; import com.alibaba.fastjson.serializer.SerializerFeature; import com.fasterxml.jackson.databind.JavaType; import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.databind.type.TypeFactory; import org.apache.shiro.util.Assert; import org.springframework.data.redis.serializer.RedisSerializer; import org.springframework.data.redis.serializer.SerializationException; import java.nio.charset.Charset; public class FastJson2JsonRedisSerializer<T> implements RedisSerializer<T> { private ObjectMapper objectMapper = new ObjectMapper(); public static final Charset DEFAULT_CHARSET = Charset.forName("UTF-8"); private Class<T> clazz; static { ParserConfig.getGlobalInstance().setAutoTypeSupport(true); ParserConfig.getGlobalInstance().addAccept("com.openailab.oascloud"); } public FastJson2JsonRedisSerializer(Class<T> clazz) { super(); this.clazz = clazz; } @Override public byte[] serialize(T t) throws SerializationException { if (t == null) { return new byte[0]; } return JSON.toJSONString(t, SerializerFeature.WriteClassName).getBytes(DEFAULT_CHARSET); } @Override public T deserialize(byte[] bytes) throws SerializationException { if (bytes == null || bytes.length <= 0) { return null; } String str = new String(bytes, DEFAULT_CHARSET); return JSON.parseObject(str, clazz); } public void setObjectMapper(ObjectMapper objectMapper) { Assert.notNull(objectMapper, "'objectMapper' must not be null"); this.objectMapper = objectMapper; } protected JavaType getJavaType(Class<?> clazz) { return TypeFactory.defaultInstance().constructType(clazz); } }
配置application.yml
spring: redis: host: 127.0.0.1 port: 6379 password: root #如果没设置密码留空 jedis: pool: max-active: 8 max-wait: -1 max-idle: 500 min-idle: 0 lettuce: shutdown-timeout: 0
配置RedisConfig
* 1) RedisTemplate(或StringRedisTemplate)虽然已经自动配置,但是不灵活(第一没有序列化,第二泛型为<Object, Object>不是我们想要的类型)
* 所以自己实现RedisTemplate或StringRedisTemplate)
* 2) 采用RedisCacheManager作为缓存管理器
package com.ruoyi.project.system.role.controller; import com.fasterxml.jackson.annotation.JsonAutoDetect; import com.fasterxml.jackson.annotation.PropertyAccessor; import com.fasterxml.jackson.databind.ObjectMapper; import org.springframework.cache.CacheManager; import org.springframework.cache.annotation.CachingConfigurerSupport; import org.springframework.cache.annotation.EnableCaching; import org.springframework.cache.interceptor.KeyGenerator; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.data.redis.cache.RedisCacheConfiguration; import org.springframework.data.redis.cache.RedisCacheManager; import org.springframework.data.redis.cache.RedisCacheWriter; import org.springframework.data.redis.connection.RedisConnectionFactory; import org.springframework.data.redis.core.RedisTemplate; import org.springframework.data.redis.serializer.*; import java.lang.reflect.Method; import java.time.Duration; @Configuration @EnableCaching public class RedisConfig extends CachingConfigurerSupport { private RedisSerializer<String> keySerializer() { return new StringRedisSerializer(); } //使用Jackson序列化器 private RedisSerializer<Object> valueSerializer() { return new GenericJackson2JsonRedisSerializer(); } @Bean public CacheManager cacheManager(RedisConnectionFactory redisConnectionFactory) { //缓存配置对象 RedisCacheConfiguration redisCacheConfiguration = RedisCacheConfiguration.defaultCacheConfig(); redisCacheConfiguration = redisCacheConfiguration.entryTtl(Duration.ofMinutes(30L)) //设置缓存的默认超时时间:30分钟 .disableCachingNullValues() //如果是空值,不缓存 .serializeKeysWith(RedisSerializationContext.SerializationPair.fromSerializer(keySerializer())) //设置key序列化器 .serializeValuesWith(RedisSerializationContext.SerializationPair.fromSerializer((valueSerializer()))); //设置value序列化器 return RedisCacheManager .builder(RedisCacheWriter.nonLockingRedisCacheWriter(redisConnectionFactory)) .cacheDefaults(redisCacheConfiguration).build(); } @Override @Bean public KeyGenerator keyGenerator() { // TODO Auto-generated method stub return new KeyGenerator() { @Override public Object generate(Object object, Method method, Object... objects) { // TODO Auto-generated method stub StringBuilder sb = new StringBuilder(); sb.append(object.getClass().getName()); sb.append(method.getName()); for (Object obj : objects) { if (obj != null) { sb.append(obj.toString()); } } return sb.toString(); } }; } @Bean public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) { RedisTemplate<String, Object> redisTemplate = new RedisTemplate<String, Object>(); redisTemplate.setConnectionFactory(redisConnectionFactory); FastJson2JsonRedisSerializer fastJson2JsonRedisSerializer = new FastJson2JsonRedisSerializer(Object.class); ObjectMapper objectMapper = new ObjectMapper(); objectMapper.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY); objectMapper.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL); fastJson2JsonRedisSerializer.setObjectMapper(objectMapper); StringRedisSerializer stringRedisSerializer = new StringRedisSerializer(); // key采用String的序列化方式 redisTemplate.setKeySerializer(stringRedisSerializer); // string的value采用fastJson序列化方式 redisTemplate.setValueSerializer(fastJson2JsonRedisSerializer); // hash的key也采用String的序列化方式 redisTemplate.setHashKeySerializer(stringRedisSerializer); // hash的value采用fastJson序列化方式 redisTemplate.setHashValueSerializer(fastJson2JsonRedisSerializer); redisTemplate.afterPropertiesSet(); return redisTemplate; } }
在启动类上面加上redis缓存注解
在conroller使用redis
@Cacheable只是一种注解使用方法 更多的使用方法自行研究扩展
@Cacheable(cacheNames = "product", key = "123")
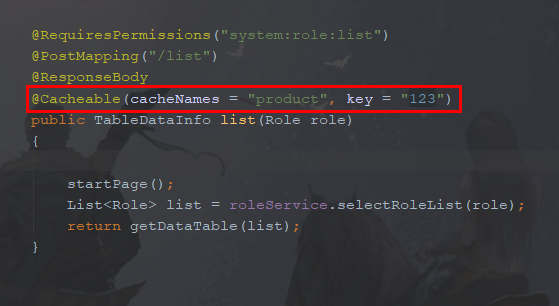
访问controler
第二次访问就会读取redis 记录一次简易集成
如何使用注解啦,这一步反而是最简单的.其实只用到了两个注解,@Cacheable和@CacheEvict.第一个注解代表从缓存中查询指定的key,如果有,从缓存中取,不再执行方法.如果没有则执
行方法,并且将方法的返回值和指定的key关联起来,放入到缓存中.而@CacheEvict则是从缓存中清除指定的key对应的数据.使用的代码如下:
@Cacheable(value="thisredis", key="'users_'+#id")
public User findUser(Integer id) {
User user = new User();
user.setUsername("hlhdidi");
user.setPassword("123");
user.setUid(id.longValue());
System.out.println("log4j2坏啦?");
logger.info("输入user,用户名:{},密码:{}",user.getUsername(),user.getPassword());
return user;
}
@CacheEvict(value="thisredis", key="'users_'+#id",condition="#id!=1")
public void delUser(Integer id) {
// 删除user
System.out.println("user删除");
}
可以看出,我们用@Cacheable的value属性指定具体缓存,并通过key将其放入缓存中.这里key非常灵活,支持spring的el表达式,可以通过方法参数产生可变的key(见findUser方法),也可以通过其指定在
什么情况下,使用/不使用缓存(见delUser方法).
更多的操作和优化 可自行研究