Flying to the Mars
Time Limit: 5000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 6271 Accepted Submission(s): 2053
Problem Description
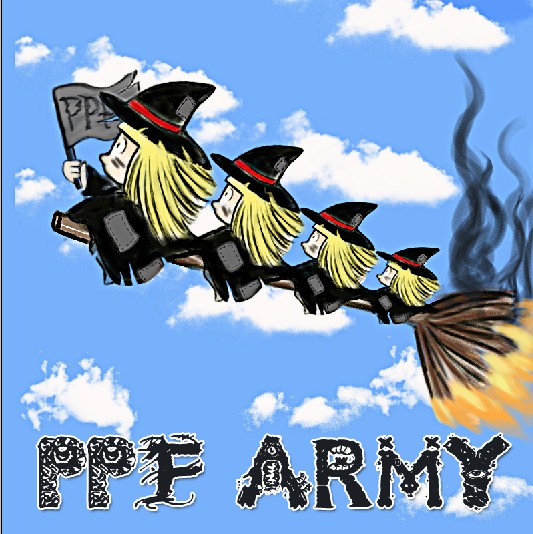
In the year 8888, the Earth is ruled by the PPF Empire . As the population growing , PPF needs to find more land for the newborns . Finally , PPF decides to attack Kscinow who ruling the Mars . Here the problem comes! How can the soldiers reach the Mars ? PPF convokes his soldiers and asks for their suggestions . “Rush … ” one soldier answers. “Shut up ! Do I have to remind you that there isn’t any road to the Mars from here!” PPF replies. “Fly !” another answers. PPF smiles :“Clever guy ! Although we haven’t got wings , I can buy some magic broomsticks from HARRY POTTER to help you .” Now , it’s time to learn to fly on a broomstick ! we assume that one soldier has one level number indicating his degree. The soldier who has a higher level could teach the lower , that is to say the former’s level > the latter’s . But the lower can’t teach the higher. One soldier can have only one teacher at most , certainly , having no teacher is also legal. Similarly one soldier can have only one student at most while having no student is also possible. Teacher can teach his student on the same broomstick .Certainly , all the soldier must have practiced on the broomstick before they fly to the Mars! Magic broomstick is expensive !So , can you help PPF to calculate the minimum number of the broomstick needed .
For example :
There are 5 soldiers (A B C D E)with level numbers : 2 4 5 6 4;
One method :
C could teach B; B could teach A; So , A B C are eligible to study on the same broomstick.
D could teach E;So D E are eligible to study on the same broomstick;
Using this method , we need 2 broomsticks.
Another method:
D could teach A; So A D are eligible to study on the same broomstick.
C could teach B; So B C are eligible to study on the same broomstick.
E with no teacher or student are eligible to study on one broomstick.
Using the method ,we need 3 broomsticks.
……
After checking up all possible method, we found that 2 is the minimum number of broomsticks needed.
Input
Input file contains multiple test cases.
In a test case,the first line contains a single positive number N indicating the number of soldiers.(0<=N<=3000)
Next N lines :There is only one nonnegative integer on each line , indicating the level number for each soldier.( less than 30 digits);
In a test case,the first line contains a single positive number N indicating the number of soldiers.(0<=N<=3000)
Next N lines :There is only one nonnegative integer on each line , indicating the level number for each soldier.( less than 30 digits);
Output
For each case, output the minimum number of broomsticks on a single line.
Sample Input
4 10 20 30 04 5 2 3 4 3 4
Sample Output
1 2
1 /* 2 功能Function Description: HDOJ 1800 3 开发环境Environment: DEV C++ 4.9.9.1 4 技术特点Technique: 5 版本Version: 6 作者Author: 可笑痴狂 7 日期Date: 20120810 8 备注Notes: 9 题目意思: 10 有n个士兵每个人有一个水平值,水平高的的人可以教低的人,意思就是求最少的扫帚, 11 那么我们只要知道找到最大重复元素的次数即可,因为相同的人肯定不能共用一个, 12 所以求得最少即为最大的重复次数 13 注意:前置的0必须要去掉,例如数据 14 3 15 0 16 00 17 000 18 输出 3 19 20 这题和拦截导弹那题(动态规划求最长不降子序列)的区别是 导弹来的顺序是固定的不能改变,而这道题是可以随意变动的 21 */ 22 23 /* 24 //代码一:-------直接排序找最长平台(贪心算法) 25 #include<cstdio> 26 #include<cstring> 27 #include<algorithm> 28 using namespace std; 29 30 int main() 31 { 32 int n,i,max,t; 33 int a[3005]; 34 while(scanf("%d",&n)!=EOF) 35 { 36 for(i=0;i<n;++i) 37 scanf("%d",&a[i]); 38 sort(a,a+n); 39 max=1; 40 t=1; 41 for(i=1;i<n;++i) 42 { 43 if(a[i]!=a[i-1]) 44 t=1; 45 else 46 ++t; 47 if(t>max) 48 max=t; 49 } 50 printf("%d\n",max); 51 } 52 return 0; 53 } 54 55 56 //代码二:---------字典树 57 #include<cstdio> 58 #include<cstring> 59 #include<stdlib.h> 60 61 struct node 62 { 63 int sum; 64 node *next[26]; 65 }; 66 node memory[1000000]; 67 int k; 68 int max; 69 70 void insert(char *t,node *T) 71 { 72 int i,id,j; 73 node *p,*q; 74 p=T; 75 i=0; 76 while(t[i]) 77 { 78 id=t[i]-'0'; 79 if(p->next[id]==NULL) 80 { 81 // q=(node *)malloc(sizeof(node)); 82 q=&memory[k++]; 83 q->sum=0; 84 for(j=0;j<26;++j) 85 q->next[j]=NULL; 86 p->next[id]=q; 87 } 88 p=p->next[id]; 89 ++i; 90 } 91 p->sum++; 92 if(max<p->sum) 93 max=p->sum; 94 } 95 96 int main() 97 { 98 int n,i; 99 char s[35]; 100 node *T; 101 // T=(node *)malloc(sizeof(node)); 102 T=&memory[0]; 103 while(scanf("%d",&n)!=EOF) 104 { 105 max=0; 106 k=1; 107 T->sum=0; 108 for(i=0;i<26;++i) 109 T->next[i]=NULL; 110 while(n--) 111 { 112 scanf("%s",s); 113 i=0; 114 while(s[i++]=='0'); 115 insert(&s[i-1],T); 116 } 117 printf("%d\n",max); 118 } 119 return 0; 120 } 121 122 */ 123 124 //代码三: 125 126 //BST代码:----二分查找树/二叉排序树 127 128 #include <stdio.h> 129 #include <malloc.h> 130 struct node 131 { 132 int num; 133 node *lc; 134 node *rc; 135 int time; 136 }; 137 int max; 138 struct node *BST(struct node *root,int num) 139 { 140 if (root==NULL) //根节点为空 建立根节点 141 { 142 root=(struct node *)malloc(sizeof(struct node)); 143 root->lc=NULL; 144 root->rc=NULL; 145 root->num=num; 146 root->time=1; 147 } 148 else 149 { 150 if (root->num==num) // 根的name 和 s相同,次数+1 151 { 152 root->time++; 153 if (root->time>max) 154 max=root->time; 155 } 156 else if (root->num>num) // 根比s大,找左子树 157 root->lc=BST(root->lc,num); 158 else // 根比s小,找右子树 159 root->rc=BST(root->rc,num); 160 } 161 return root; 162 } 163 int main() 164 { 165 int n,i,num; 166 while (scanf("%d",&n)!=EOF) 167 { 168 struct node *root=NULL; 169 max=1; 170 for (i=0;i<n;i++) 171 { 172 scanf("%d",&num); 173 root=BST(root,num); 174 } 175 printf ("%d\n",max); 176 } 177 return 0; 178 }