1.编写一个程序,指定一个文件夹,能自动计算出其总容量
package filesoperation;
import java.io.File;
import java.io.IOException;
import java.nio.file.FileVisitResult;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.SimpleFileVisitor;
import java.nio.file.attribute.BasicFileAttributes;
public class FolderTotalSize extends SimpleFileVisitor<Path> {
private static long size = 0;
public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException {
File f = new File(file.toString());
size += f.length();
return FileVisitResult.CONTINUE;
}
public static void main(String[] args) {
Path fileDirPath=Paths.get("F:\学习\作业");
FolderTotalSize visitor=new FolderTotalSize();
try {
Files.walkFileTree(fileDirPath, visitor);
System.out.println("文件夹"F:\学习\作业"总大小为" + size);
} catch (IOException e) {
e.printStackTrace();
}
}
}

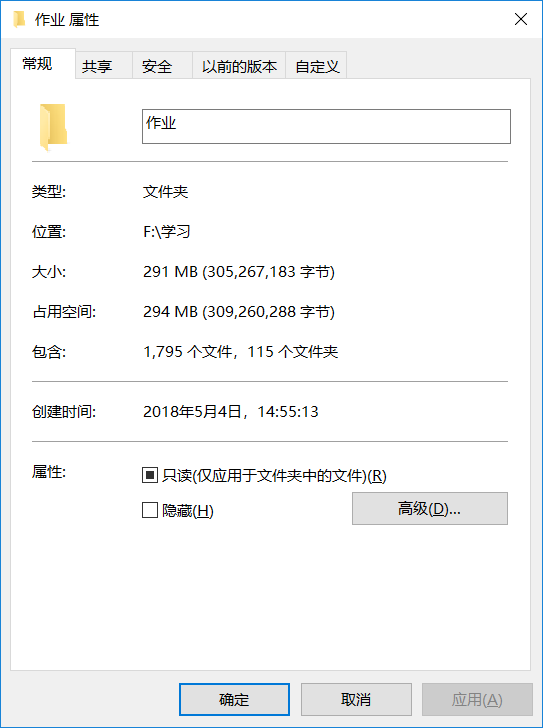
2.编写一个文件加解密程序,通过命令行完成加解密工作
package filesoperation;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class FileEncryption {
public static void encryption() throws IOException {
BufferedInputStream bis = new BufferedInputStream(new FileInputStream("未加密.txt"));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream("已加密.txt"));
int b;
while((b = bis.read()) != -1) {
bos.write(b ^ 123);
}
bis.close();
bos.close();
}
public static void main(String[] args) throws IOException {
encryption();
}
}
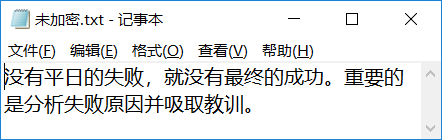
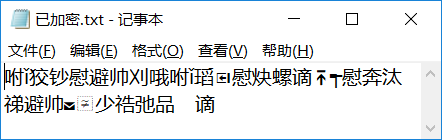
3.编写一个文件分割工具,能把一个大文件分割成多个小的文件。并且能再次把它们合并起来得到完整的文件。
package filesoperation;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.file.FileVisitResult;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.SimpleFileVisitor;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.ArrayList;
public class CutFile extends SimpleFileVisitor<Path>{
public static ArrayList<Path> smallfiles = new ArrayList<>();
public static void main(String[] args) {
cut("E:\test.txt", "E:\test", 1024 * 1024 * 1);
paste("E:\test", "E:\test1", 1024 * 1024 * 1);
}
public static void cut(String filepath, String endpath, int sizeofsmallfile) { //原文件,切割后小文件路径,小文件最大容量
FileInputStream fis = null;
File file = null;
try {
fis = new FileInputStream(filepath);
file = new File(filepath);
byte[] b = new byte[sizeofsmallfile];
int read = 0;
int numberofsmallfile = 1;
while ((read = fis.read(b)) != -1) {
String nameoflargefile = file.getName(); //获取大文件全名(带扩展名)
String nameofsmallfile = nameoflargefile.substring(0, nameoflargefile.lastIndexOf(".")); //获取小文件名(不带扩展名)
String extension = nameoflargefile.substring(nameoflargefile.lastIndexOf("."), nameoflargefile.length()); //获取扩展名
FileOutputStream fos = new FileOutputStream(endpath + "\" + nameofsmallfile + "-" + numberofsmallfile + extension); //创建小文件
fos.write(b, 0, read); //写入
fos.close();
numberofsmallfile++;
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null) {
fis.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
public static void paste(String smallfilespath, String endpath, int sizeofsmallfile) { //小文件路径,生成的大文件路径,小文件最大容量
Path fileDirPath = Paths.get(smallfilespath);
CutFile visitor = new CutFile();
try {
Files.walkFileTree(fileDirPath, visitor);
File file = new File(smallfiles.get(0).toString());
String nameofsmallfile = file.getName(); //获取第一个小文件全名(带扩展名)
String nameoflargefile = nameofsmallfile.substring(0, nameofsmallfile.lastIndexOf("-")); //获取大文件名(不带扩展名)
String extension = nameofsmallfile.substring(nameofsmallfile.lastIndexOf("."), nameofsmallfile.length()); //获取扩展名
FileOutputStream fos = new FileOutputStream(endpath + "\" + nameoflargefile + extension); //创建大文件
byte[] b = new byte[sizeofsmallfile];
for(Path p:smallfiles) {
FileInputStream fis = new FileInputStream(p.toString());
int read = fis.read(b);
fis.close();
fos.write(b, 0, read); //写入
}
fos.close();
}catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException {
if(attrs.isRegularFile()){
smallfiles.add(file);
}
return FileVisitResult.CONTINUE;
}
}

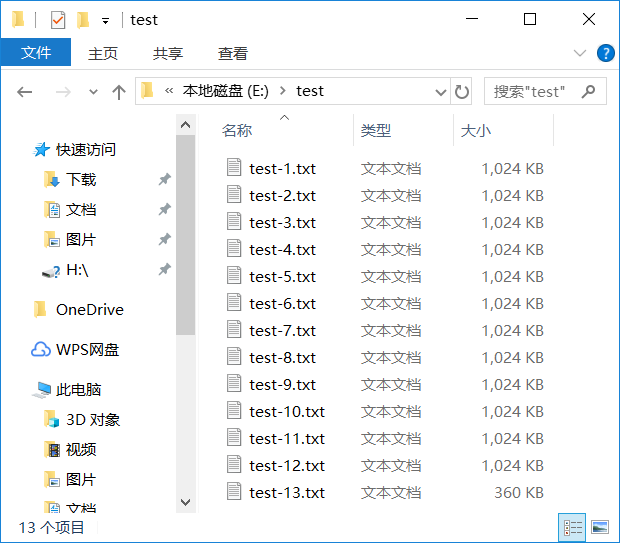
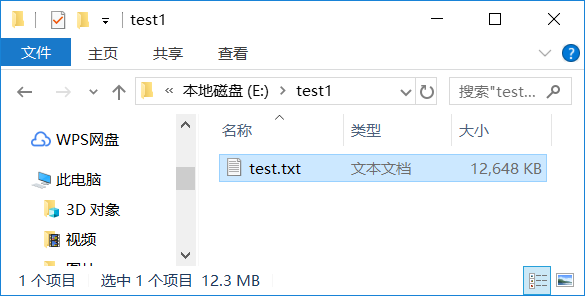