// R0 - The signal number. // R1 - Pointer to siginfo_t structure. // R2 - Pointer to ucontext structure. // TEXT ·sighandler(SB),NOSPLIT,$0 // si_signo should be sigill. MOVD SIGINFO_SIGNO(R1), R7 CMPW $4, R7 ----信号num是不是4 BNE fallback MOVD CONTEXT_PC(R2), R7 CMPW $0, R7 BEQ fallback MOVD R2, 8(RSP) BL ·bluepillHandler(SB) // Call the handler. RET fallback: // Jump to the previous signal handler. MOVD ·savedHandler(SB), R7 B (R7)
root@cloud:~/onlyGvisor/gvisor# grep '$4' -rn * pkg/sentry/platform/ring0/pagetables/pcids_aarch64.s:31: UBFX $ID_AA64MMFR0_ASIDBITS_SHIFT, R0, $4, R0 pkg/sentry/platform/ring0/entry_impl_arm64.s:347: BFI $48, R1, $16, R0; pkg/sentry/platform/ring0/entry_impl_arm64.s:357: BFI $48, R1, $16, R0; pkg/sentry/platform/ptrace/stub_arm64.s:45: CMN $4095, R0 pkg/sentry/platform/kvm/bluepill_arm64.s:78: CMPW $4, R7 pkg/sentry/time/muldiv_arm64.s:21:TEXT ·muldiv64(SB),NOSPLIT,$40-33 pkg/safecopy/memclr_arm64.s:38: LSR $4, R1, R2
pkg/sentry/platform/kvm/bluepill.go:90: if err := safecopy.ReplaceSignalHandler(bluepillSignal, reflect.ValueOf(sighandler).Pointer(), &savedHandler); err != nil { pkg/safecopy/safecopy.go:130: if err := ReplaceSignalHandler(syscall.SIGSEGV, reflect.ValueOf(signalHandler).Pointer(), &savedSigSegVHandler); err != nil { pkg/safecopy/safecopy.go:133: if err := ReplaceSignalHandler(syscall.SIGBUS, reflect.ValueOf(signalHandler).Pointer(), &savedSigBusHandler); err
SignalSetup
// SignalSetup implements Context.SignalSetup.
func (c *context64) SignalSetup(st *Stack, act *SignalAct, info *SignalInfo, alt *SignalStack, sigset linux.SignalSet) error {
sp := st.Bottom
if !(alt.IsEnabled() && sp == alt.Top()) {
sp -= 128
}
// Construct the UContext64 now since we need its size.
uc := &UContext64{
Flags: 0,
Stack: *alt,
MContext: SignalContext64{
Regs: c.Regs.Regs,
Sp: c.Regs.Sp,
Pc: c.Regs.Pc,
Pstate: c.Regs.Pstate,
},
Sigset: sigset,
}
ucSize := uc.SizeBytes()
// frameSize = ucSize + sizeof(siginfo).
// sizeof(siginfo) == 128.
// R30 stores the restorer address.
frameSize := ucSize + 128
frameBottom := (sp - usermem.Addr(frameSize)) & ^usermem.Addr(15)
sp = frameBottom + usermem.Addr(frameSize)
st.Bottom = sp
// Prior to proceeding, figure out if the frame will exhaust the range
// for the signal stack. This is not allowed, and should immediately
// force signal delivery (reverting to the default handler).
if act.IsOnStack() && alt.IsEnabled() && !alt.Contains(frameBottom) {
return syscall.EFAULT
}
// Adjust the code.
info.FixSignalCodeForUser()
// Set up the stack frame.
if _, err := info.CopyOut(st, StackBottomMagic); err != nil {
return err
}
infoAddr := st.Bottom
if _, err := uc.CopyOut(st, StackBottomMagic); err != nil {
return err
}
ucAddr := st.Bottom
// Set up registers.
c.Regs.Sp = uint64(st.Bottom)
c.Regs.Pc = act.Handler
c.Regs.Regs[0] = uint64(info.Signo) // 信号num
c.Regs.Regs[1] = uint64(infoAddr)
c.Regs.Regs[2] = uint64(ucAddr)
c.Regs.Regs[30] = uint64(act.Restorer)
// Save the thread's floating point state.
c.sigFPState = append(c.sigFPState, c.aarch64FPState)
// Signal handler gets a clean floating point state.
c.aarch64FPState = newAarch64FPState()
return nil
}
runtime.sighandler
root@cloud:~# dlv attach 952917 Type 'help' for list of commands. (dlv) b sighandler Breakpoint 1 set at 0x58230 for runtime.sighandler() GOROOT/src/runtime/signal_unix.go:522 (dlv) c > runtime.sighandler() GOROOT/src/runtime/signal_unix.go:522 (hits goroutine(19):1 total:1) (PC: 0x58230) Warning: debugging optimized function (dlv) bt 0 0x0000000000058230 in runtime.sighandler at GOROOT/src/runtime/signal_unix.go:522 1 0x0000000000000000 in ??? at :0 error: NULL address (truncated) (dlv) c > runtime.sighandler() GOROOT/src/runtime/signal_unix.go:522 (hits goroutine(232):1 total:2) (PC: 0x58230) Warning: debugging optimized function (dlv) bt 0 0x0000000000058230 in runtime.sighandler at GOROOT/src/runtime/signal_unix.go:522 1 0x0000000000075978 in runtime.systemstack_switch at src/runtime/asm_arm64.s:180 2 0x000000000004d2d4 in runtime.newproc at GOROOT/src/runtime/proc.go:3527 3 0x00000000006b8db4 in gvisor.dev/gvisor/pkg/sentry/watchdog.(*Watchdog).runTurn at pkg/sentry/watchdog/watchdog.go:273 4 0x00000000006b8c6c in gvisor.dev/gvisor/pkg/sentry/watchdog.(*Watchdog).loop at pkg/sentry/watchdog/watchdog.go:261 5 0x0000000000077c84 in runtime.goexit at src/runtime/asm_arm64.s:1136 (dlv) c
// sighandler: see bluepill.go for documentation. // // The arguments are the following: // // R0 - The signal number. // R1 - Pointer to siginfo_t structure. // R2 - Pointer to ucontext structure. // TEXT ·sighandler(SB),NOSPLIT,$0 // si_signo should be sigill. MOVD SIGINFO_SIGNO(R1), R7 CMPW $4, R7 BNE fallback MOVD CONTEXT_PC(R2), R7 CMPW $0, R7 BEQ fallback MOVD R2, 8(RSP) BL ·bluepillHandler(SB) // Call the handler. RET fallback: // Jump to the previous signal handler. MOVD ·savedHandler(SB), R7 B (R7)
触发 sigill
bluepill()->cli->exception->bluepillHandler()->save cli location X context->KVM_RUN from location X on guest ring0.
// See bluepill.go. TEXT ·bluepill(SB),NOSPLIT,$0 begin: MOVD vcpu+0(FP), R8 MOVD $VCPU_CPU(R8), R9 ORR $0xffff000000000000, R9, R9 // Trigger sigill. // In ring0.Start(), the value of R8 will be stored into tpidr_el1. // When the context was loaded into vcpu successfully, // we will check if the value of R10 and R9 are the same. WORD $0xd538d08a // MRS TPIDR_EL1, R10 check_vcpu: CMP R10, R9 BEQ right_vCPU wrong_vcpu: CALL ·redpill(SB) B begin right_vCPU: RET
Thread ID registers
TPIDR_EL0和TPIDRRO_EL0寄存器是两个具有不同访问权限的线程ID寄存器。
查看syscall.SIGILL的信号编号
root@cloud:~/onlyGvisor# grep bluepillSignal -rn * gvisor/pkg/sentry/platform/kvm/bluepill.go:90: if err := safecopy.ReplaceSignalHandler(bluepillSignal, reflect.ValueOf(sighandler).Pointer(), &savedHandler); err != nil { gvisor/pkg/sentry/platform/kvm/bluepill.go:91: panic(fmt.Sprintf("Unable to set handler for signal %d: %v", bluepillSignal, err)) gvisor/pkg/sentry/platform/kvm/bluepill_amd64.go:27: // The action for bluepillSignal is changed by sigaction(). gvisor/pkg/sentry/platform/kvm/bluepill_amd64.go:28: bluepillSignal = syscall.SIGSEGV gvisor/pkg/sentry/platform/kvm/bluepill_arm64.go:27: // The action for bluepillSignal is changed by sigaction(). gvisor/pkg/sentry/platform/kvm/bluepill_arm64.go:28: bluepillSignal = syscall.SIGILL root@cloud:~/onlyGvisor# kill -l | grep -i SIGILL 1) SIGHUP 2) SIGINT 3) SIGQUIT 4) SIGILL 5) SIGTRAP root@cloud:~/onlyGvisor# kill -4 948796 root@cloud:~/onlyGvisor#
给bluepillHandler设置断点
KVM_RUN is called in bluepillhandler and bluepillhandler is registered as the siganl SIGSEGV handler, so only this signal will trigger the KVM_RUN?
bluepillHandler's KVM_EXIT_HLT handler copies the registers at the point of SYSCALL to ucontext so that on sigreturn we'll return to the SYSCALL instructio
root@cloud:~# dlv attach 948796 Type 'help' for list of commands. (dlv) b bluepillHandler Breakpoint 1 set at 0x87b300 for gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (dlv) c > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (hits goroutine(257):1 total:1) (PC: 0x87b300) Warning: debugging optimized function (dlv) bt 0 0x000000000087b300 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler at pkg/sentry/platform/kvm/bluepill_unsafe.go:91 1 0x0000000000881bec in ??? at ?:-1 2 0x0000ffffa1ad1598 in ??? at ?:-1 3 0x000000000003f4a8 in runtime.futexsleep at GOROOT/src/runtime/os_linux.go:45 4 0x0000000000c11a8c in ??? at ?:-1 5 0x000000000001ac8c in runtime.notetsleep_internal at GOROOT/src/runtime/lock_futex.go:182 6 0x00000000015377e0 in ??? at ?:-1 7 0x0000000000074354 in os/signal.signal_recv at GOROOT/src/runtime/sigqueue.go:147 8 0x00000000015377e0 in ??? at ?:-1 9 0x0000000000077c84 in runtime.goexit at src/runtime/asm_arm64.s:1136 error: Undefined return address at 0x77c84 (truncated) (dlv)
// //go:nosplit func bluepillGuestExit(c *vCPU, context unsafe.Pointer) { // Increment our counter. atomic.AddUint64(&c.guestExits, 1) // Copy out registers. bluepillArchExit(c, bluepillArchContext(context)) // Return to the vCPUReady state; notify any waiters. user := atomic.LoadUint32(&c.state) & vCPUUser switch atomic.SwapUint32(&c.state, user) { case user | vCPUGuest: // Expected case. case user | vCPUGuest | vCPUWaiter: c.notify() default: throw("invalid state") } }
unc bluepillArchExit(c *vCPU, context *arch.SignalContext64) { regs := c.CPU.Registers() context.R8 = regs.R8 context.R9 = regs.R9 context.R10 = regs.R10 context.R11 = regs.R11 context.R12 = regs.R12 context.R13 = regs.R13 context.R14 = regs.R14 context.R15 = regs.R15 context.Rdi = regs.Rdi context.Rsi = regs.Rsi context.Rbp = regs.Rbp context.Rbx = regs.Rbx context.Rdx = regs.Rdx context.Rax = regs.Rax context.Rcx = regs.Rcx context.Rsp = regs.Rsp context.Rip = regs.Rip context.Eflags = regs.Eflags // Set the context pointer to the saved floating point state. This is // where the guest data has been serialized, the kernel will restore // from this new pointer value. context.Fpstate = uint64(uintptrValue((*byte)(c.floatingPointState))) }
发送 syscall.SIGILL
root@cloud:~/onlyGvisor# kill -4 948796
demo2
执行 docker rm -f test
(dlv) > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (hits goroutine(250):1 total:2) (PC: 0x87b300) Warning: debugging optimized function (dlv) > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (hits goroutine(250):2 total:3) (PC: 0x87b300) Warning: debugging optimized function (dlv) bt 0 0x000000000087b300 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler at pkg/sentry/platform/kvm/bluepill_unsafe.go:91 1 0x0000000000881bec in ??? at ?:-1 2 0x0000ffffa1ad1598 in ??? at ?:-1 3 0x000000000087a858 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.(*allocator).FreePTEs at pkg/sentry/platform/kvm/bluepill_allocator.go:91 调用bluepill 4 0x0000004000782480 in ??? at ?:-1 5 0x00000000008774e8 in gvisor.dev/gvisor/pkg/sentry/platform/ring0/pagetables.(*unmapWalker).iterateRangeCanonical at bazel-out/aarch64-dbg-ST-4c64f0b3d5c7/bin/pkg/sentry/platform/ring0/pagetables/walker_unmap_arm64.go:247 6 0x00000040005ca000 in ??? at ?:-1 7 0x0000000000874dd0 in gvisor.dev/gvisor/pkg/sentry/platform/ring0/pagetables.(*PageTables).Unmap at pkg/sentry/platform/ring0/pagetables/pagetables.go:226 8 0x000000400068fd78 in ??? at ?:-1 9 0x000000000087a204 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.(*addressSpace).Release at pkg/sentry/platform/kvm/address_space.go:243 10 0x0000004000124230 in ??? at ?:-1 11 0x00000000003dd7b0 in gvisor.dev/gvisor/pkg/sentry/mm.(*MemoryManager).DecUsers at pkg/sentry/mm/lifecycle.go:273 12 0x000000400000c100 in ??? at ?:-1 13 0x0000000000517d9c in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).run at pkg/sentry/kernel/task_run.go:97 14 0x0000004000367000 in ??? at ?:-1 error: input/output error
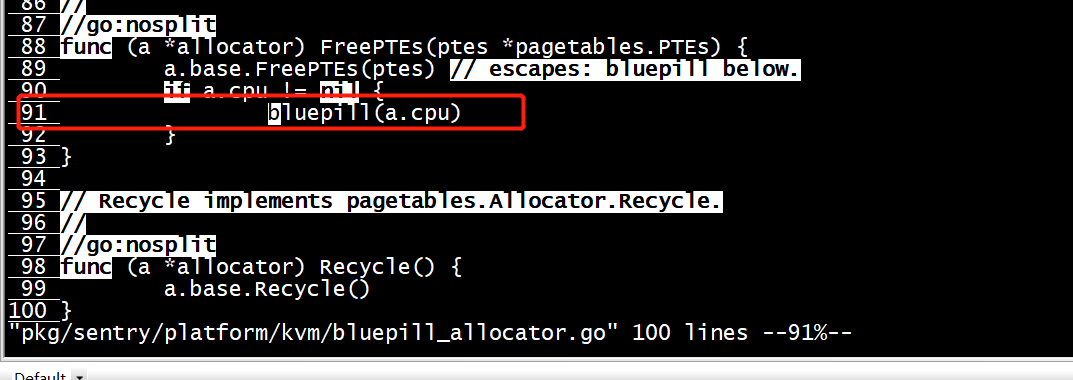
demo3
1、b bluepillHandler
2、docker exec -it test ping 8.8.8.8
(dlv) b bluepillHandler Breakpoint 2 set at 0x87b300 for gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (dlv) c > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (hits goroutine(230):1 total:1) (PC: 0x87b300) Warning: debugging optimized function (dlv) bt 0 0x000000000087b300 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler at pkg/sentry/platform/kvm/bluepill_unsafe.go:91 1 0x0000000000881bec in ??? at ?:-1 2 0x0000ffffa4a94598 in ??? at ?:-1 3 0x000000000087f514 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.(*vCPU).SwitchToUser at pkg/sentry/platform/kvm/machine_arm64_unsafe.go:249 4 0x0000004000475000 in ??? at ?:-1 5 0x000000000087f514 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.(*vCPU).SwitchToUser at pkg/sentry/platform/kvm/machine_arm64_unsafe.go:249 6 0x000000400062ccd8 in ??? at ?:-1 7 0x00000000005186d0 in gvisor.dev/gvisor/pkg/sentry/kernel.(*runApp).execute at pkg/sentry/kernel/task_run.go:271 8 0x000000400022c000 in ??? at ?:-1 9 0x0000000000077c84 in runtime.goexit at src/runtime/asm_arm64.s:1136 error: Undefined return address at 0x77c84 (truncated) (dlv)
和发送sigill的信号的调用栈不一样
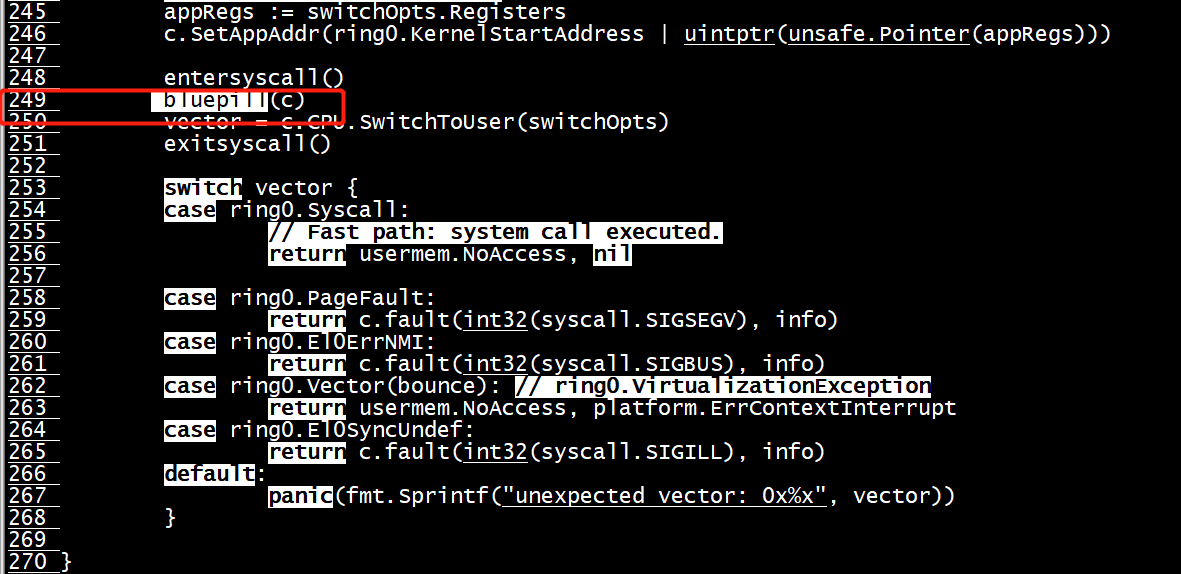
// See bluepill.go. TEXT ·bluepill(SB),NOSPLIT,$0 begin: MOVD vcpu+0(FP), R8 MOVD $VCPU_CPU(R8), R9 ORR $0xffff000000000000, R9, R9 // Trigger sigill. 触发sigill // In ring0.Start(), the value of R8 will be stored into tpidr_el1. // When the context was loaded into vcpu successfully, // we will check if the value of R10 and R9 are the same. WORD $0xd538d08a // MRS TPIDR_EL1, R10 check_vcpu: CMP R10, R9 BEQ right_vCPU wrong_vcpu: CALL ·redpill(SB) B begin right_vCPU: RET
runtime.sigreturn
https://github.com/google/gvisor/issues/25
runtime: newstack at runtime.printlock+0x76 sp=0xc4204cd9f8 stack=[0xc420051000, 0xc420052000] morebuf={pc:0x4293d3 sp:0xc4204cda00 lr:0x0} sched={pc:0x429d66 sp:0xc4204cd9f8 lr:0x0 ctxt:0x0} runtime.throw(0xb2537f, 0x8) GOROOT/src/runtime/panic.go:610 +0x13 fp=0xc4204cda20 sp=0xc4204cda00 pc=0x4293d3 gvisor.googlesource.com/gvisor/pkg/sentry/platform/kvm.bluepillHandler(0xc4204cdac0) pkg/sentry/platform/kvm/bluepill_unsafe.go:168 +0x38a fp=0xc4204cdab0 sp=0xc4204cda20 pc=0x81e79a gvisor.googlesource.com/gvisor/pkg/sentry/platform/kvm.sighandler(0x7, 0x0, 0xc4204c6000, 0x0, 0x8000, 0xc42008c418, 0xc42008c498, 0x1, 0xc42008c410, 0xc42008c410, ...) pkg/sentry/platform/kvm/bluepill_amd64.s:79 +0x24 fp=0xc4204cdac0 sp=0xc4204cdab0 pc=0x82c254 runtime: unexpected return pc for runtime.sigreturn called from 0x7 stack: frame={sp:0xc4204cdac0, fp:0xc4204cdac8} stack=[0xc420051000,0xc420052000) runtime.sigreturn(0x0, 0xc4204c6000, 0x0, 0x8000, 0xc42008c418, 0xc42008c498, 0x1, 0xc42008c410, 0xc42008c410, 0x0, ...) bazel-out/k8-fastbuild/bin/external/io_bazel_rules_go/linux_amd64_pure_stripped/stdlib~/src/runtime/sys_linux_amd64.s:444 fp=0xc4204cdac8 sp=0xc4204cdac0 pc=0x457340 created by gvisor.googlesource.com/gvisor/pkg/sentry/kernel.(*Task).Start pkg/sentry/kernel/task_start.go:251 +0x100 fatal error: runtime: stack split at bad time runtime stack: runtime.throw(0xb35d4b, 0x20) GOROOT/src/runtime/panic.go:616 +0x81 fp=0xc4204b3e48 sp=0xc4204b3e28 pc=0x429441 runtime.newstack() GOROOT/src/runtime/stack.go:954 +0xb61 fp=0xc4204b3fd8 sp=0xc4204b3e48 pc=0x442821 runtime.morestack() bazel-out/k8-fastbuild/bin/external/io_bazel_rules_go/linux_amd64_pure_stripped/stdlib~/src/runtime/asm_amd64.s:480 +0x89 fp=0xc4204b3fe0 sp=0xc4204b3fd8 pc=0x453669 goroutine 46 [syscall, locked to thread]: runtime.throw(0xb2537f, 0x8) GOROOT/src/runtime/panic.go:610 +0x13 fp=0xc4204cda20 sp=0xc4204cda00 pc=0x4293d3 gvisor.googlesource.com/gvisor/pkg/sentry/platform/kvm.bluepillHandler(0xc4204cdac0) pkg/sentry/platform/kvm/bluepill_unsafe.go:168 +0x38a fp=0xc4204cdab0 sp=0xc4204cda20 pc=0x81e79a gvisor.googlesource.com/gvisor/pkg/sentry/platform/kvm.sighandler(0x7, 0x0, 0xc4204c6000, 0x0, 0x8000, 0xc42008c418, 0xc42008c498, 0x1, 0xc42008c410, 0xc42008c410, ...) pkg/sentry/platform/kvm/bluepill_amd64.s:79 +0x24 fp=0xc4204cdac0 sp=0xc4204cdab0 pc=0x82c254 runtime: unexpected return pc for runtime.sigreturn called from 0x7 stack: frame={sp:0xc4204cdac0, fp:0xc4204cdac8} stack=[0xc420051000,0xc420052000) runtime.sigreturn(0x0, 0xc4204c6000, 0x0, 0x8000, 0xc42008c418, 0xc42008c498, 0x1, 0xc42008c410, 0xc42008c410, 0x0, ...) bazel-out/k8-fastbuild/bin/external/io_bazel_rules_go/linux_amd64_pure_stripped/stdlib~/src/runtime/sys_linux_amd64.s:444 fp=0xc4204cdac8 sp=0xc4204cdac0 pc=0x457340 created by gvisor.googlesource.com/gvisor/pkg/sentry/kernel.(*Task).Start pkg/sentry/kernel/task_start.go:251 +0x100
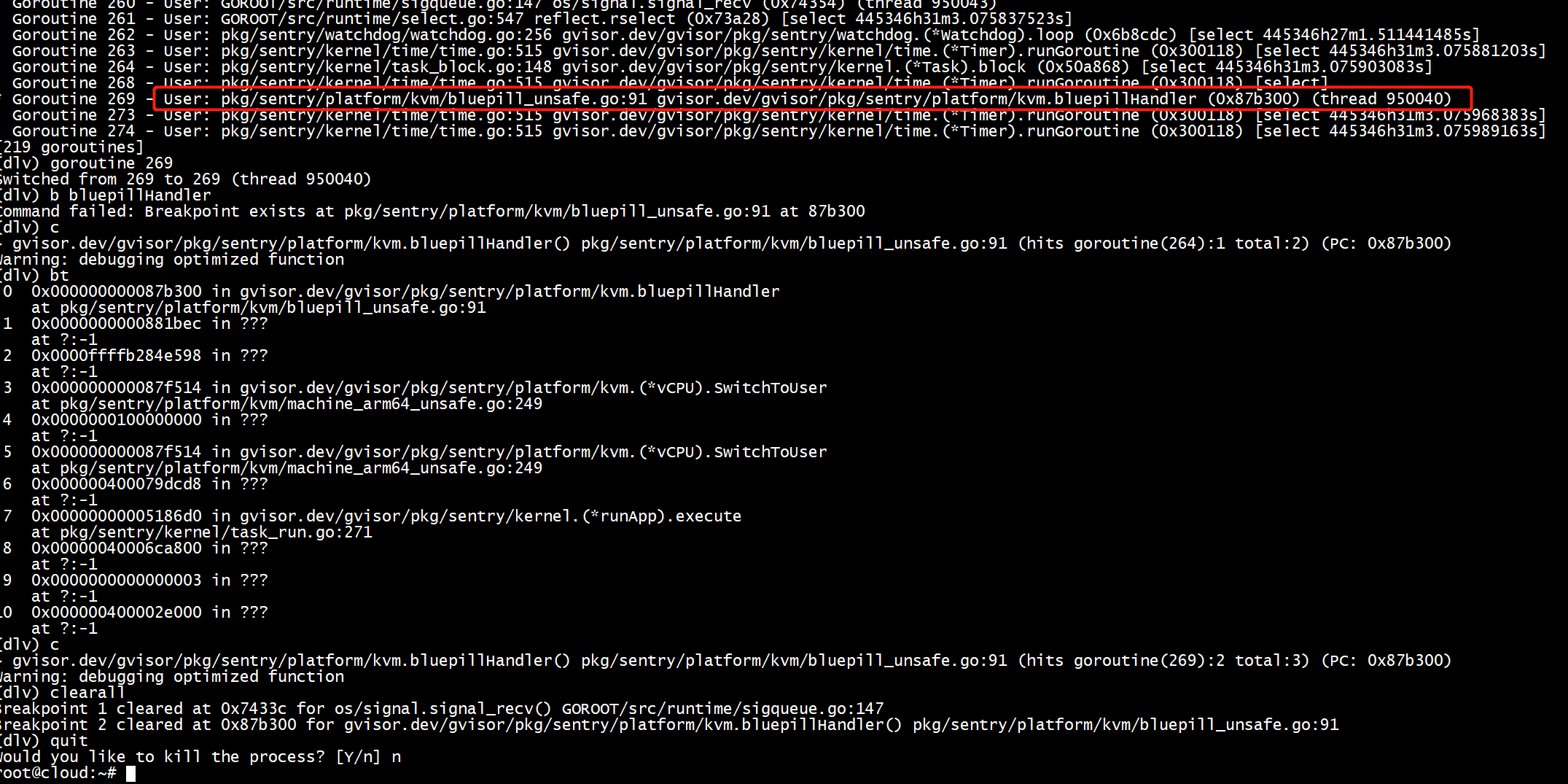
Goroutine bluepillHandler
* Goroutine 269 - User: pkg/sentry/platform/kvm/bluepill_unsafe.go:91 gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler (0x87b300) (thread 950040)
启动容器,没有bluepillHandler
docker run --runtime=runsc-kvm --rm --name=test -d alpine sleep 1000
(dlv) goroutines Goroutine 1 - User: GOROOT/src/runtime/sema.go:56 sync.runtime_Semacquire (0x73ac8) [semacquire] Goroutine 2 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [force gc (idle)] Goroutine 3 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC sweep wait] Goroutine 4 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC scavenge wait] Goroutine 5 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select] Goroutine 6 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select] Goroutine 17 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [finalizer wait] Goroutine 18 - User: GOROOT/src/runtime/proc.go:312 sync.runtime_notifyListWait (0x73d7c) [sync.Cond.Wait] Goroutine 19 - User: pkg/sentry/kernel/timekeeper.go:218 gvisor.dev/gvisor/pkg/sentry/kernel.(*Timekeeper).startUpdater.func1 (0x53c204) [select] Goroutine 20 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 21 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 22 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 23 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 24 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 25 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 26 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 27 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 28 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 29 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 30 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 31 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 32 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 33 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 34 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 35 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 36 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 37 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 38 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 39 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 40 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 41 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 42 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 43 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 44 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 45 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 46 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 47 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 48 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 49 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 50 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 51 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 52 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 53 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 54 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 55 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 56 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 57 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 58 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 59 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 60 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 61 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 62 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 63 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 64 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 65 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 66 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 67 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 68 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 69 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 70 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 71 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 72 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 73 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 74 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 75 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 76 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 77 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 78 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 79 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 80 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 81 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 82 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 83 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 84 - User: pkg/sentry/watchdog/watchdog.go:237 gvisor.dev/gvisor/pkg/sentry/watchdog.(*Watchdog).waitForStart (0x6b8aac) [chan receive] * Goroutine 85 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950342) Goroutine 87 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 88 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 89 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 90 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 91 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 92 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 93 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 94 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 95 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 96 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 97 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 98 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 99 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 100 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 101 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 102 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 103 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 104 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 105 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 106 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 107 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 108 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 109 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 110 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 111 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 112 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 113 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 114 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 115 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 116 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 117 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 118 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 119 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 120 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 121 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 122 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 123 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 124 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 125 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 126 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 127 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 128 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 129 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 130 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 131 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 132 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 133 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 134 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 135 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 136 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 137 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 138 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 139 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 140 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 141 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 142 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 143 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 144 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 145 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 146 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 147 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 148 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 149 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 150 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 151 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 152 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 153 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 154 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 155 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 156 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 157 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 158 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 159 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 160 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 161 - User: pkg/tcpip/link/rawfile/rawfile_unsafe.go:164 gvisor.dev/gvisor/pkg/tcpip/link/rawfile.BlockingRecvMMsg (0x86b784) (thread 950362) [GC assist marking] Goroutine 162 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 163 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 164 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 165 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 166 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 167 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 168 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 169 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 170 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 171 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 172 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 173 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 174 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 175 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950363) Goroutine 176 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select] Goroutine 177 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 178 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 179 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 180 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 181 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 182 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 183 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 184 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 185 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 186 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 187 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 188 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 189 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 190 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 191 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 192 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 193 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 194 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 195 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 196 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 197 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 198 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 199 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 200 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 201 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 202 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 203 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 204 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 205 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 206 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 209 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 210 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 211 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 212 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 213 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 214 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 215 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 216 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 217 - User: GOROOT/src/runtime/select.go:547 reflect.rselect (0x73a28) [select] Goroutine 218 - User: pkg/sentry/watchdog/watchdog.go:256 gvisor.dev/gvisor/pkg/sentry/watchdog.(*Watchdog).loop (0x6b8cdc) [select] Goroutine 219 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select] Goroutine 220 - User: pkg/sentry/kernel/task_block.go:148 gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).block (0x50a868) [select] Goroutine 225 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 226 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 227 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 228 - User: src/syscall/asm_linux_arm64.s:44 syscall.Syscall6 (0x8dcd0) (thread 950414) Goroutine 229 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950422) Goroutine 230 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950423) Goroutine 231 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950419) Goroutine 232 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950361) Goroutine 241 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [select] Goroutine 242 - User: GOROOT/src/runtime/sigqueue.go:147 os/signal.signal_recv (0x74354) (thread 950420) [217 goroutines] (dlv)
执行新的命令,有bluepillHandler 协程
Croot@cloud:~# docker exec -it test ping 8.8.8.8 PING 8.8.8.8 (8.8.8.8): 56 data bytes 64 bytes from 8.8.8.8: seq=0 ttl=42 time=35.091 ms 64 bytes from 8.8.8.8: seq=1 ttl=42 time=32.483 ms 64 bytes from 8.8.8.8: seq=2 ttl=42 time=12.273 ms 64 bytes from 8.8.8.8: seq=3 ttl=42 time=11.812 ms 64 bytes from 8.8.8.8: seq=4 ttl=42 time=26.824 ms 64 bytes from 8.8.8.8: seq=5 ttl=42 time=31.979 ms 64 bytes from 8.8.8.8: seq=6 ttl=42 time=31.998 ms 64 bytes from 8.8.8.8: seq=7 ttl=42 time=13.570 ms
[217 goroutines] (dlv) c received SIGINT, stopping process (will not forward signal) > syscall.Syscall6() src/syscall/asm_linux_arm64.s:43 (PC: 0x8dccc) Warning: debugging optimized function (dlv) goroutines Goroutine 1 - User: GOROOT/src/runtime/sema.go:56 sync.runtime_Semacquire (0x73ac8) [semacquire 445346h14m21.09190664s] Goroutine 2 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [force gc (idle) 445346h14m21.09193764s] Goroutine 3 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC sweep wait] Goroutine 4 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC scavenge wait] Goroutine 5 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select 445346h14m21.091993521s] Goroutine 6 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select 445346h14m21.092013481s] Goroutine 8 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select] Goroutine 9 - User: pkg/sentry/kernel/task_block.go:148 gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).block (0x50a868) [select] Goroutine 17 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [finalizer wait 445346h14m21.092066901s] Goroutine 18 - User: GOROOT/src/runtime/proc.go:312 sync.runtime_notifyListWait (0x73d7c) [sync.Cond.Wait 445346h14m21.092086181s] Goroutine 19 - User: pkg/sentry/kernel/timekeeper.go:218 gvisor.dev/gvisor/pkg/sentry/kernel.(*Timekeeper).startUpdater.func1 (0x53c204) [select] Goroutine 20 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092122161s] Goroutine 21 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092141081s] Goroutine 22 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092159501s] Goroutine 23 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092179061s] Goroutine 24 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092197861s] Goroutine 25 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092216761s] Goroutine 26 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092235281s] Goroutine 27 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092254681s] Goroutine 28 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092273201s] Goroutine 29 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092292102s] Goroutine 30 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092310782s] Goroutine 31 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092329322s] Goroutine 32 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092347862s] Goroutine 33 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092366142s] Goroutine 34 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092384162s] Goroutine 35 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092407902s] Goroutine 36 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092426242s] Goroutine 37 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092444802s] Goroutine 38 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092463742s] Goroutine 39 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092483822s] Goroutine 40 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092502322s] Goroutine 41 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092521002s] Goroutine 42 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092539342s] Goroutine 43 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092558042s] Goroutine 44 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092576682s] Goroutine 45 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092594962s] Goroutine 46 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092614382s] Goroutine 47 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092633103s] Goroutine 48 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092651903s] Goroutine 49 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092670083s] Goroutine 50 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092688203s] Goroutine 51 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092706643s] Goroutine 52 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092725243s] Goroutine 53 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092743763s] Goroutine 54 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092762503s] Goroutine 55 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092780763s] Goroutine 56 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092799563s] Goroutine 57 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092817803s] Goroutine 58 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092836003s] Goroutine 59 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092854563s] Goroutine 60 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092873963s] Goroutine 61 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092893003s] Goroutine 62 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092911283s] Goroutine 63 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092931883s] Goroutine 64 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092950703s] Goroutine 65 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092968844s] Goroutine 66 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.092988444s] Goroutine 67 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093008344s] Goroutine 68 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093027284s] Goroutine 69 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093045944s] Goroutine 70 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093064544s] Goroutine 71 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093096624s] Goroutine 72 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093115604s] Goroutine 73 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093134224s] Goroutine 74 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093159604s] Goroutine 75 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093179004s] Goroutine 76 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093197464s] Goroutine 77 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093215944s] Goroutine 78 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093234844s] Goroutine 79 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093253464s] Goroutine 80 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093272324s] Goroutine 81 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093290745s] Goroutine 82 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093309265s] Goroutine 83 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093327465s] * Goroutine 85 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950342) Goroutine 87 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.093363145s] Goroutine 88 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.093384465s] Goroutine 89 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.093403605s] Goroutine 90 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.093422865s] Goroutine 91 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.093441085s] Goroutine 92 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.093460105s] Goroutine 93 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.093478765s] Goroutine 94 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.093497525s] Goroutine 95 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.093515885s] Goroutine 96 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.093534485s] Goroutine 97 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 98 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093568465s] Goroutine 99 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093587185s] Goroutine 100 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093606065s] Goroutine 101 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093624666s] Goroutine 102 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093643586s] Goroutine 103 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093662386s] Goroutine 104 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093681226s] Goroutine 105 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093699986s] Goroutine 106 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093718386s] Goroutine 107 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093736566s] Goroutine 108 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093756066s] Goroutine 109 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093774646s] Goroutine 110 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093792866s] Goroutine 111 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093812126s] Goroutine 112 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093830946s] Goroutine 113 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093849886s] Goroutine 114 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093868066s] Goroutine 115 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093886466s] Goroutine 116 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093904746s] Goroutine 117 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093923126s] Goroutine 118 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093941626s] Goroutine 119 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093960147s] Goroutine 120 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.093978447s] Goroutine 121 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094003587s] Goroutine 122 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094021847s] Goroutine 123 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094040247s] Goroutine 124 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094059727s] Goroutine 125 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094078107s] Goroutine 126 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094096527s] Goroutine 127 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094114647s] Goroutine 128 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094133807s] Goroutine 129 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094153507s] Goroutine 130 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094172247s] Goroutine 131 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094190927s] Goroutine 132 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094209687s] Goroutine 133 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094228187s] Goroutine 134 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094246747s] Goroutine 135 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094265507s] Goroutine 136 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094283787s] Goroutine 137 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094302008s] Goroutine 138 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094320768s] Goroutine 139 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094339648s] Goroutine 140 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094359328s] Goroutine 141 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094377948s] Goroutine 142 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094396268s] Goroutine 143 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094414508s] Goroutine 144 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094433088s] Goroutine 145 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094451528s] Goroutine 146 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094470088s] Goroutine 147 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094488188s] Goroutine 148 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094507008s] Goroutine 149 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094525168s] Goroutine 150 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094543568s] Goroutine 151 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094561948s] Goroutine 152 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094580528s] Goroutine 153 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094599008s] Goroutine 154 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094618048s] Goroutine 155 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094636509s] Goroutine 156 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094655129s] Goroutine 157 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094677869s] Goroutine 158 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094696609s] Goroutine 159 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094715969s] Goroutine 160 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h14m21.094734529s] Goroutine 161 - User: pkg/tcpip/link/rawfile/rawfile_unsafe.go:164 gvisor.dev/gvisor/pkg/tcpip/link/rawfile.BlockingRecvMMsg (0x86b784) (thread 950362) [GC assist marking] Goroutine 162 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 163 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 164 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.094803369s] Goroutine 165 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.094821929s] Goroutine 166 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.094840349s] Goroutine 167 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.094858649s] Goroutine 168 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.094877789s] Goroutine 169 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.094900769s] Goroutine 170 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.094920029s] Goroutine 171 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.094938329s] Goroutine 172 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.094956609s] Goroutine 173 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.09497595s] Goroutine 174 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.09499475s] Goroutine 175 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950363) Goroutine 176 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select 445346h14m21.09502917s] Goroutine 177 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 178 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 179 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 180 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 181 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.09511243s] Goroutine 182 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.09513073s] Goroutine 183 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.09514911s] Goroutine 184 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.09516729s] Goroutine 185 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.09518533s] Goroutine 186 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.09520367s] Goroutine 187 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.09522169s] Goroutine 188 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.09523957s] Goroutine 189 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.09525883s] Goroutine 190 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.09527749s] Goroutine 191 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095297191s] Goroutine 192 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095316531s] Goroutine 193 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095335091s] Goroutine 194 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 195 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 196 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 197 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095401611s] Goroutine 198 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095420251s] Goroutine 199 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095439491s] Goroutine 200 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095457911s] Goroutine 201 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095476211s] Goroutine 202 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095494891s] Goroutine 203 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095512911s] Goroutine 204 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095531031s] Goroutine 205 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095549671s] Goroutine 206 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095567791s] Goroutine 209 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095585891s] Goroutine 210 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095604091s] Goroutine 211 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095624231s] Goroutine 212 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095642812s] Goroutine 213 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095662332s] Goroutine 214 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095680652s] Goroutine 215 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095698952s] Goroutine 216 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095717532s] Goroutine 217 - User: GOROOT/src/runtime/select.go:547 reflect.rselect (0x73a28) [select 445346h14m21.095735692s] Goroutine 218 - User: pkg/sentry/watchdog/watchdog.go:256 gvisor.dev/gvisor/pkg/sentry/watchdog.(*Watchdog).loop (0x6b8cdc) [select 445346h14m21.095754592s] Goroutine 219 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select] Goroutine 220 - User: pkg/sentry/kernel/task_block.go:148 gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).block (0x50a868) [select 445346h14m21.095789512s] Goroutine 225 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095807992s] Goroutine 226 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095827212s] Goroutine 227 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h14m21.095845732s] Goroutine 228 - User: src/syscall/asm_linux_arm64.s:44 syscall.Syscall6 (0x8dcd0) (thread 950442) Goroutine 229 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950422) Goroutine 230 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950423) Goroutine 231 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950419) Goroutine 232 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950361) Goroutine 235 - User: GOROOT/src/runtime/sema.go:56 sync.runtime_Semacquire (0x73ac8) [semacquire] Goroutine 241 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [select 445346h14m21.095964793s] Goroutine 242 - User: GOROOT/src/runtime/sigqueue.go:147 os/signal.signal_recv (0x74354) (thread 950420) Goroutine 243 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select] [220 goroutines] (dlv)

中断命令, bluepillHandler 协程结束
(dlv) goroutines Goroutine 1 - User: GOROOT/src/runtime/sema.go:56 sync.runtime_Semacquire (0x73ac8) [semacquire 445346h19m17.141844536s] Goroutine 2 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [force gc (idle) 445346h17m7.359959572s] Goroutine 3 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC sweep wait] Goroutine 4 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC scavenge wait] Goroutine 5 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select 445346h19m17.141942457s] Goroutine 6 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select 445346h19m17.141966877s] Goroutine 17 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [finalizer wait 445346h19m17.141989097s] Goroutine 18 - User: GOROOT/src/runtime/proc.go:312 sync.runtime_notifyListWait (0x73d7c) [sync.Cond.Wait] Goroutine 19 - User: pkg/sentry/kernel/timekeeper.go:218 gvisor.dev/gvisor/pkg/sentry/kernel.(*Timekeeper).startUpdater.func1 (0x53c204) [select] Goroutine 20 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142051057s] Goroutine 21 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142074877s] Goroutine 22 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142095677s] Goroutine 23 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142119097s] Goroutine 24 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142139837s] Goroutine 25 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142160437s] Goroutine 26 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142180817s] Goroutine 27 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142201158s] Goroutine 28 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142222118s] Goroutine 29 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142243078s] Goroutine 30 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142262878s] Goroutine 31 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142282978s] Goroutine 32 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142303858s] Goroutine 33 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142324478s] Goroutine 34 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142345258s] Goroutine 35 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142365958s] Goroutine 36 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142386758s] Goroutine 37 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142409138s] Goroutine 38 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142430098s] Goroutine 39 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142450698s] Goroutine 40 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142470698s] Goroutine 41 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142490998s] Goroutine 42 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142510939s] Goroutine 43 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142535559s] Goroutine 44 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142556299s] Goroutine 45 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142604539s] Goroutine 46 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142625919s] Goroutine 47 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142646659s] Goroutine 48 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142667539s] Goroutine 49 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142687979s] Goroutine 50 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142708379s] Goroutine 51 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142730659s] Goroutine 52 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142751379s] Goroutine 53 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142772159s] Goroutine 54 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142798199s] Goroutine 55 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.142819039s] Goroutine 56 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.14283934s] Goroutine 57 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.14286014s] Goroutine 58 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.14288058s] Goroutine 59 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.14290146s] Goroutine 60 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.14292136s] Goroutine 61 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.1429418s] Goroutine 62 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.14296222s] Goroutine 63 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.14298504s] Goroutine 64 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.14300524s] Goroutine 65 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143026s] Goroutine 66 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.14304626s] Goroutine 67 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.14306676s] Goroutine 68 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.1430876s] Goroutine 69 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.14310784s] Goroutine 70 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.1431281s] Goroutine 71 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143149161s] Goroutine 72 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143169761s] Goroutine 73 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143189921s] Goroutine 74 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143210641s] Goroutine 75 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143230521s] Goroutine 76 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143253281s] Goroutine 77 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143273761s] Goroutine 78 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143293801s] Goroutine 79 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143313901s] Goroutine 80 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143334261s] Goroutine 81 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143355281s] Goroutine 82 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143377721s] Goroutine 83 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143398381s] * Goroutine 85 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950342) Goroutine 87 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.143476502s] Goroutine 88 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.143497442s] Goroutine 89 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.143517942s] Goroutine 90 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.143537702s] Goroutine 91 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.143558042s] Goroutine 92 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.143578742s] Goroutine 93 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.143599222s] Goroutine 94 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.143619922s] Goroutine 95 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.143639702s] Goroutine 96 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.143660402s] Goroutine 97 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select] Goroutine 98 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143697982s] Goroutine 99 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143718982s] Goroutine 100 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143739562s] Goroutine 101 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143760362s] Goroutine 102 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143780703s] Goroutine 103 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143800763s] Goroutine 104 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143828363s] Goroutine 105 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143848763s] Goroutine 106 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143870423s] Goroutine 107 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143890743s] Goroutine 108 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143911883s] Goroutine 109 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143931803s] Goroutine 110 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143951863s] Goroutine 111 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143972003s] Goroutine 112 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.143994043s] Goroutine 113 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144014123s] Goroutine 114 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144034483s] Goroutine 115 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144054743s] Goroutine 116 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144075563s] Goroutine 117 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144099524s] Goroutine 118 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144120804s] Goroutine 119 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144141084s] Goroutine 120 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144161604s] Goroutine 121 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144182864s] Goroutine 122 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144203204s] Goroutine 123 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144223864s] Goroutine 124 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144243924s] Goroutine 125 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144266324s] Goroutine 126 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144287064s] Goroutine 127 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144307624s] Goroutine 128 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144327784s] Goroutine 129 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144348184s] Goroutine 130 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144368364s] Goroutine 131 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144388684s] Goroutine 132 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144409184s] Goroutine 133 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144429285s] Goroutine 134 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144449805s] Goroutine 135 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144470625s] Goroutine 136 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144492425s] Goroutine 137 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144513005s] Goroutine 138 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144533345s] Goroutine 139 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144553725s] Goroutine 140 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144582625s] Goroutine 141 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144604265s] Goroutine 142 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144624605s] Goroutine 143 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144645245s] Goroutine 144 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144665465s] Goroutine 145 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144686125s] Goroutine 146 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144707685s] Goroutine 147 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144728105s] Goroutine 148 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144748646s] Goroutine 149 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144769626s] Goroutine 150 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144791346s] Goroutine 151 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144811886s] Goroutine 152 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144833626s] Goroutine 153 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144854146s] Goroutine 154 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144874826s] Goroutine 155 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144895206s] Goroutine 156 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144920046s] Goroutine 157 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144941126s] Goroutine 158 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144961266s] Goroutine 159 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.144981966s] Goroutine 160 - User: pkg/sync/runtime_unsafe.go:40 gvisor.dev/gvisor/pkg/sleep.(*Sleeper).nextWaker (0x5cfd44) [select 445346h19m17.145002466s] Goroutine 161 - User: pkg/tcpip/link/rawfile/rawfile_unsafe.go:164 gvisor.dev/gvisor/pkg/tcpip/link/rawfile.BlockingRecvMMsg (0x86b784) (thread 950362) [GC assist marking] Goroutine 162 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h17m7.363121701s] Goroutine 163 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h17m7.363141822s] Goroutine 164 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 165 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 166 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145121087s] Goroutine 167 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145141467s] Goroutine 168 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145175147s] Goroutine 169 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145196007s] Goroutine 170 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145216667s] Goroutine 171 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145236807s] Goroutine 172 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145257347s] Goroutine 173 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145278467s] Goroutine 174 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145298747s] Goroutine 175 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950363) Goroutine 176 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select 445346h19m17.145336507s] Goroutine 177 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h17m7.363439042s] Goroutine 178 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 179 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 180 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 181 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 182 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145456508s] Goroutine 183 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145478008s] Goroutine 184 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145498308s] Goroutine 185 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145519088s] Goroutine 186 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145541588s] Goroutine 187 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145561988s] Goroutine 188 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145582548s] Goroutine 189 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145602528s] Goroutine 190 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145622928s] Goroutine 191 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145645188s] Goroutine 192 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145666108s] Goroutine 193 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 194 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 195 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h17m7.363805904s] Goroutine 196 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle)] Goroutine 197 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145767389s] Goroutine 198 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145790209s] Goroutine 199 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145814309s] Goroutine 200 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145835249s] Goroutine 201 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145855649s] Goroutine 202 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145876569s] Goroutine 203 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145896389s] Goroutine 204 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145916869s] Goroutine 205 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145937169s] Goroutine 206 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145960669s] Goroutine 209 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.145981329s] Goroutine 210 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.146001569s] Goroutine 211 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.14602173s] Goroutine 212 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.14604311s] Goroutine 213 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.14606563s] Goroutine 214 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.14608671s] Goroutine 215 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.14610697s] Goroutine 216 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.14613021s] Goroutine 217 - User: GOROOT/src/runtime/select.go:547 reflect.rselect (0x73a28) [select 445346h19m17.14615035s] Goroutine 218 - User: pkg/sentry/watchdog/watchdog.go:256 gvisor.dev/gvisor/pkg/sentry/watchdog.(*Watchdog).loop (0x6b8cdc) [select] Goroutine 219 - User: pkg/sentry/kernel/time/time.go:515 gvisor.dev/gvisor/pkg/sentry/kernel/time.(*Timer).runGoroutine (0x300118) [select] Goroutine 220 - User: pkg/sentry/kernel/task_block.go:148 gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).block (0x50a868) [select 445346h19m17.14621065s] Goroutine 225 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.14623103s] Goroutine 226 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.14625335s] Goroutine 227 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [GC worker (idle) 445346h19m17.14627343s] Goroutine 228 - User: src/syscall/asm_linux_arm64.s:44 syscall.Syscall6 (0x8dcd0) (thread 950442) Goroutine 229 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950422) Goroutine 230 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950423) Goroutine 231 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950419) Goroutine 232 - User: src/syscall/asm_linux_arm64.s:43 syscall.Syscall6 (0x8dccc) (thread 950361) Goroutine 241 - User: GOROOT/src/runtime/proc.go:307 runtime.gopark (0x450c0) [select 445346h19m17.146382551s] Goroutine 242 - User: GOROOT/src/runtime/sigqueue.go:147 os/signal.signal_recv (0x74354) (thread 950420) [216 goroutines] (dlv)
b RtSigreturn
创建容器
root@cloud:~/gvisor/pkg# docker run --runtime=runsc-kvm --rm --name=test -d alpine sleep 1000 4ce4523d1c232ae331e00b87a183bb232c3b3bef989f86633c7fb78d3d4872fe root@cloud:~/gvisor/pkg#
设置断点
(dlv) b RtSigreturn Breakpoint 2 set at 0x586900 for gvisor.dev/gvisor/pkg/sentry/syscalls/linux.RtSigreturn() pkg/sentry/syscalls/linux/sys_signal.go:281 (dlv) c
在没有执行 docker exec -it test ping 8.8.8.8情况下,断点不会执行
(dlv) b SignalRestore Breakpoint 3 set at 0x281d54 for gvisor.dev/gvisor/pkg/sentry/arch.(*context64).SignalRestore() pkg/sentry/arch/signal_arm64.go:151 (dlv) c > gvisor.dev/gvisor/pkg/sentry/arch.(*context64).SignalRestore() pkg/sentry/arch/signal_arm64.go:151 (hits goroutine(248):1 total:1) (PC: 0x281d54) Warning: debugging optimized function (dlv) bt 0 0x0000000000281d54 in gvisor.dev/gvisor/pkg/sentry/arch.(*context64).SignalRestore at pkg/sentry/arch/signal_arm64.go:151 1 0x000000000051d138 in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).SignalReturn at pkg/sentry/kernel/task_signals.go:306 2 0x0000000000586920 in gvisor.dev/gvisor/pkg/sentry/syscalls/linux.RtSigreturn at pkg/sentry/syscalls/linux/sys_signal.go:282 3 0x0000000000522ea4 in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).executeSyscall at pkg/sentry/kernel/task_syscall.go:104 4 0x0000000000523c5c in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).doSyscallInvoke at pkg/sentry/kernel/task_syscall.go:239 5 0x00000000005238dc in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).doSyscallEnter at pkg/sentry/kernel/task_syscall.go:199 6 0x00000000005233e0 in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).doSyscall at pkg/sentry/kernel/task_syscall.go:174 7 0x0000000000518e00 in gvisor.dev/gvisor/pkg/sentry/kernel.(*runApp).execute at pkg/sentry/kernel/task_run.go:282 8 0x0000000000517d9c in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).run at pkg/sentry/kernel/task_run.go:97 9 0x0000000000077c84 in runtime.goexit at src/runtime/asm_arm64.s:1136 (dlv)
// SignalReturn implements sigreturn(2) (if rt is false) or rt_sigreturn(2) (if
// rt is true).
SignalRestore implements Context.SignalRestore. func (c *context64) SignalRestore(st *Stack, rt bool) (linux.SignalSet, SignalStack, error) { // Copy out the stack frame. var uc UContext64 if _, err := uc.CopyIn(st, StackBottomMagic); err != nil { return 0, SignalStack{}, err } var info SignalInfo if _, err := info.CopyIn(st, StackBottomMagic); err != nil { return 0, SignalStack{}, err } // Restore registers. c.Regs.Regs = uc.MContext.Regs c.Regs.Pc = uc.MContext.Pc c.Regs.Sp = uc.MContext.Sp c.Regs.Pstate = uc.MContext.Pstate // Restore floating point state. l := len(c.sigFPState) if l > 0 { c.aarch64FPState = c.sigFPState[l-1] // NOTE(cl/133042258): State save requires that any slice // elements from '[len:cap]' to be zero value. c.sigFPState[l-1] = nil c.sigFPState = c.sigFPState[0 : l-1] } else { // This might happen if sigreturn(2) calls are unbalanced with // respect to signal handler entries. This is not expected so // don't bother to do anything fancy with the floating point // state. log.Warningf("sigreturn unable to restore application fpstate") } return uc.Sigset, uc.Stack, nil }
RtSigreturn
// SignalReturn implements sigreturn(2) (if rt is false) or rt_sigreturn(2) (if // rt is true). func (t *Task) SignalReturn(rt bool) (*SyscallControl, error) { st := t.Stack() sigset, alt, err := t.Arch().SignalRestore(st, rt) if err != nil { return nil, err } // Attempt to record the given signal stack. Note that we silently // ignore failures here, as does Linux. Only an EFAULT may be // generated, but SignalRestore has already deserialized the entire // frame successfully. t.SetSignalStack(alt) // Restore our signal mask. SIGKILL and SIGSTOP should not be blocked. t.SetSignalMask(sigset &^ UnblockableSignals) t.p.FullStateChanged() return ctrlResume, nil }
创建容器
root@cloud:~/gvisor/pkg# docker run --runtime=runsc-kvm --rm --name=test -d alpine sleep 1000 4ce4523d1c232ae331e00b87a183bb232c3b3bef989f86633c7fb78d3d4872fe root@cloud:~/gvisor/pkg#
设置断点
(dlv) b RtSigreturn Breakpoint 2 set at 0x586900 for gvisor.dev/gvisor/pkg/sentry/syscalls/linux.RtSigreturn() pkg/sentry/syscalls/linux/sys_signal.go:281 (dlv) c
没有执行docker exec -it test ping 8.8.8.8,断点不会被调用
执行docker exec -it test ping 8.8.8.8,断点被调用
(dlv) b RtSigreturn Breakpoint 2 set at 0x586900 for gvisor.dev/gvisor/pkg/sentry/syscalls/linux.RtSigreturn() pkg/sentry/syscalls/linux/sys_signal.go:281 (dlv) c > gvisor.dev/gvisor/pkg/sentry/syscalls/linux.RtSigreturn() pkg/sentry/syscalls/linux/sys_signal.go:281 (hits goroutine(248):1 total:1) (PC: 0x586900) Warning: debugging optimized function (dlv) bt 0 0x0000000000586900 in gvisor.dev/gvisor/pkg/sentry/syscalls/linux.RtSigreturn at pkg/sentry/syscalls/linux/sys_signal.go:281 1 0x0000000000522ea4 in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).executeSyscall at pkg/sentry/kernel/task_syscall.go:104 2 0x0000000000523c5c in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).doSyscallInvoke at pkg/sentry/kernel/task_syscall.go:239 3 0x00000000005238dc in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).doSyscallEnter at pkg/sentry/kernel/task_syscall.go:199 4 0x00000000005233e0 in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).doSyscall at pkg/sentry/kernel/task_syscall.go:174 5 0x0000000000518e00 in gvisor.dev/gvisor/pkg/sentry/kernel.(*runApp).execute at pkg/sentry/kernel/task_run.go:282 6 0x0000000000517d9c in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).run at pkg/sentry/kernel/task_run.go:97 7 0x0000000000077c84 in runtime.goexit at src/runtime/asm_arm64.s:1136 (dlv) c > gvisor.dev/gvisor/pkg/sentry/syscalls/linux.RtSigreturn() pkg/sentry/syscalls/linux/sys_signal.go:281 (hits goroutine(248):2 total:2) (PC: 0x586900) Warning: debugging optimized function (dlv) bt 0 0x0000000000586900 in gvisor.dev/gvisor/pkg/sentry/syscalls/linux.RtSigreturn at pkg/sentry/syscalls/linux/sys_signal.go:281 1 0x0000000000522ea4 in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).executeSyscall at pkg/sentry/kernel/task_syscall.go:104 2 0x0000000000523c5c in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).doSyscallInvoke at pkg/sentry/kernel/task_syscall.go:239 3 0x00000000005238dc in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).doSyscallEnter at pkg/sentry/kernel/task_syscall.go:199 4 0x00000000005233e0 in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).doSyscall at pkg/sentry/kernel/task_syscall.go:174 5 0x0000000000518e00 in gvisor.dev/gvisor/pkg/sentry/kernel.(*runApp).execute at pkg/sentry/kernel/task_run.go:282 6 0x0000000000517d9c in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).run at pkg/sentry/kernel/task_run.go:97 7 0x0000000000077c84 in runtime.goexit at src/runtime/asm_arm64.s:1136 (dlv) clear Command failed: not enough arguments (dlv) clearall Breakpoint 2 cleared at 0x586900 for gvisor.dev/gvisor/pkg/sentry/syscalls/linux.RtSigreturn() pkg/sentry/syscalls/linux/sys_signal.go:281 (dlv) b SignalRestore Breakpoint 3 set at 0x281d54 for gvisor.dev/gvisor/pkg/sentry/arch.(*context64).SignalRestore() pkg/sentry/arch/signal_arm64.go:151 (dlv) c > gvisor.dev/gvisor/pkg/sentry/arch.(*context64).SignalRestore() pkg/sentry/arch/signal_arm64.go:151 (hits goroutine(248):1 total:1) (PC: 0x281d54) Warning: debugging optimized function (dlv) bt 0 0x0000000000281d54 in gvisor.dev/gvisor/pkg/sentry/arch.(*context64).SignalRestore at pkg/sentry/arch/signal_arm64.go:151 1 0x000000000051d138 in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).SignalReturn at pkg/sentry/kernel/task_signals.go:306 2 0x0000000000586920 in gvisor.dev/gvisor/pkg/sentry/syscalls/linux.RtSigreturn at pkg/sentry/syscalls/linux/sys_signal.go:282 3 0x0000000000522ea4 in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).executeSyscall at pkg/sentry/kernel/task_syscall.go:104 4 0x0000000000523c5c in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).doSyscallInvoke at pkg/sentry/kernel/task_syscall.go:239 5 0x00000000005238dc in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).doSyscallEnter at pkg/sentry/kernel/task_syscall.go:199 6 0x00000000005233e0 in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).doSyscall at pkg/sentry/kernel/task_syscall.go:174 7 0x0000000000518e00 in gvisor.dev/gvisor/pkg/sentry/kernel.(*runApp).execute at pkg/sentry/kernel/task_run.go:282 8 0x0000000000517d9c in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).run at pkg/sentry/kernel/task_run.go:97 9 0x0000000000077c84 in runtime.goexit at src/runtime/asm_arm64.s:1136
(dlv) b bluepillHandler Breakpoint 3 set at 0x87b300 for gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (dlv) b SignalRestore Breakpoint 4 set at 0x281d54 for gvisor.dev/gvisor/pkg/sentry/arch.(*context64).SignalRestore() pkg/sentry/arch/signal_arm64.go:151 (dlv) c > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (hits goroutine(259):1 total:1) (PC: 0x87b300) Warning: debugging optimized function (dlv) c > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (hits goroutine(259):2 total:2) (PC: 0x87b300) Warning: debugging optimized function (dlv) c > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (hits goroutine(259):3 total:3) (PC: 0x87b300) Warning: debugging optimized function (dlv) c > gvisor.dev/gvisor/pkg/sentry/arch.(*context64).SignalRestore() pkg/sentry/arch/signal_arm64.go:151 (hits goroutine(259):1 total:1) (PC: 0x281d54) Warning: debugging optimized function (dlv) c > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (hits goroutine(259):4 total:4) (PC: 0x87b300) Warning: debugging optimized function (dlv) c > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (hits goroutine(259):5 total:5) (PC: 0x87b300) Warning: debugging optimized function (dlv) c > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (hits goroutine(259):6 total:6) (PC: 0x87b300) Warning: debugging optimized function (dlv) c > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (hits goroutine(259):7 total:7) (PC: 0x87b300) Warning: debugging optimized function (dlv) c > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler() pkg/sentry/platform/kvm/bluepill_unsafe.go:91 (hits goroutine(259):8 total:8) (PC: 0x87b300) Warning: debugging optimized function (dlv) c > gvisor.dev/gvisor/pkg/sentry/arch.(*context64).SignalRestore() pkg/sentry/arch/signal_arm64.go:151 (hits goroutine(259):2 total:2) (PC: 0x281d54) Warning: debugging optimized function (dlv) c
bluepillArchEnter bluepillArchExit
root@cloud:~# docker exec -it test ping 8.8.8.8 PING 8.8.8.8 (8.8.8.8): 56 data bytes 64 bytes from 8.8.8.8: seq=0 ttl=42 time=14.428 ms 64 bytes from 8.8.8.8: seq=1 ttl=42 time=24.014 ms 64 bytes from 8.8.8.8: seq=2 ttl=42 time=11.613 ms 64 bytes from 8.8.8.8: seq=3 ttl=42 time=11.333 ms 64 bytes from 8.8.8.8: seq=4 ttl=42 time=11.457 ms 64 bytes from 8.8.8.8: seq=5 ttl=42 time=24.010 ms 64 bytes from 8.8.8.8: seq=6 ttl=42 time=11.425 ms 64 bytes from 8.8.8.8: seq=7 ttl=42 time=11.745 ms 64 bytes from 8.8.8.8: seq=8 ttl=42 time=11.259 ms 64 bytes from 8.8.8.8: seq=9 ttl=42 time=11.278 ms
root@cloud:~# dlv attach 952413 Type 'help' for list of commands. (dlv) b bluepillArchEnter Breakpoint 1 set at 0x87aa00 for gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillArchEnter() pkg/sentry/platform/kvm/bluepill_arm64.go:67 (dlv) b bluepillArchExit Breakpoint 2 set at 0x87aa90 for gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillArchExit() pkg/sentry/platform/kvm/bluepill_arm64.go:84 (dlv) c > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillArchEnter() pkg/sentry/platform/kvm/bluepill_arm64.go:67 (hits goroutine(274):1 total:1) (PC: 0x87aa00) Warning: debugging optimized function (dlv) bt 0 0x000000000087aa00 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillArchEnter at pkg/sentry/platform/kvm/bluepill_arm64.go:67 1 0x000000000087b324 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler at pkg/sentry/platform/kvm/bluepill_unsafe.go:93 2 0x0000000000881bec in ??? at ?:-1 3 0x0000ffffb4459598 in ??? at ?:-1 4 0x000000000087f514 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.(*vCPU).SwitchToUser at pkg/sentry/platform/kvm/machine_arm64_unsafe.go:249 5 0x0000000000000d08 in ??? at ?:-1 6 0x000000000087bb1c in gvisor.dev/gvisor/pkg/sentry/platform/kvm.(*context).Switch at pkg/sentry/platform/kvm/context.go:75 7 0x00000040001aa000 in ??? at ?:-1 8 0x0000000000517d9c in gvisor.dev/gvisor/pkg/sentry/kernel.(*Task).run at pkg/sentry/kernel/task_run.go:97 9 0x00000040006373f0 in ??? at ?:-1 error: input/output error (truncated) (dlv) c > gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillArchExit() pkg/sentry/platform/kvm/bluepill_arm64.go:84 (hits goroutine(274):1 total:1) (PC: 0x87aa90) Warning: debugging optimized function (dlv) bt 0 0x000000000087aa90 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillArchExit at pkg/sentry/platform/kvm/bluepill_arm64.go:84 1 0x000000000087b284 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillGuestExit at pkg/sentry/platform/kvm/bluepill_unsafe.go:69 2 0x000000000087b61c in gvisor.dev/gvisor/pkg/sentry/platform/kvm.bluepillHandler at pkg/sentry/platform/kvm/bluepill_unsafe.go:183 3 0x0000000000881bec in ??? at ?:-1 4 0x0000ffffb4459598 in ??? at ?:-1 5 0x000000000087f514 in gvisor.dev/gvisor/pkg/sentry/platform/kvm.(*vCPU).SwitchToUser at pkg/sentry/platform/kvm/machine_arm64_unsafe.go:249 6 0x0000000000000d08 in ??? at ?:-1 7 0x000000400050f000 in ??? at ?:-1