有一个字符串如下。如下,也是通过jackson把list转换成的json字符串,我想把它转过来,看网上的内容都不尽人如意,都是片断的内容。估计只有写的知道怎么使用,所以就直接看了jackson的官网,知道了使用方法。
用的类主要是
import org.codehaus.jackson.type.TypeReference; import org.codehaus.jackson.map.ObjectMapper;
要转的字符串如下:
[{"id":"36CD0224C1ED25F5E0538A3B0B7A8190","catgId":null,"matcAmont":50000,"lendPoolId":"36CD0224C1C225F5E0538A3B0B7A8190","balance":50000,"matchFlag":1,"isLock":1,"createTime":1467576000000,"userId":0,"mltCustLendPool":{"id":"36CD0224C1C225F5E0538A3B0B7A8190","userId":157020,"state":1,"isLock":1,"balance":50000,"curTotaAmt":50000,"syncDate":1467561600000,"inviFlag":2,"investTime":1467595065000,"deadline":1499131065000,"billDate":1467681465000,"billDay":5,"productId":"6","productName":"xxx","investAmt":50000,"prodOrderId":38662,"userTelephone":"15922166933","userName":"张三","idcardNum":"1111111111111","projectNum":null,"matchModelCode":null,"creditProduct":{"id":"290AA19B1134838EE053A716C0769130","swldid":"6","prodName":"xxx","prodType":null,"prodRate":0.13,"synFeeRate":null,"payCapitalType":null,"freezeTime":12,"prodCode":6,"prodCatgory":1,"unit":1},"subject":null},"orderFlag":null,"subject":0,"projectNum":null,"matchModeCode":"1"},{"id":"36DA5B50E54E2790E0538A3B0B7A2261","catgId":null,"matcAmont":50000,"lendPoolId":"36CD0224C1C225F5E0538A3B0B7A8190","balance":50000,"matchFlag":1,"isLock":1,"createTime":1467748800000,"userId":0,"mltCustLendPool":{"id":"36CD0224C1C225F5E0538A3B0B7A8190","userId":157020,"state":1,"isLock":1,"balance":50000,"curTotaAmt":50000,"syncDate":1467561600000,"inviFlag":2,"investTime":1467595065000,"deadline":1499131065000,"billDate":1467681465000,"billDay":5,"productId":"6","productName":"xxx","investAmt":50000,"prodOrderId":38662,"userTelephone":"15922166933","userName":"王dd","idcardNum":"2222222222222","projectNum":null,"matchModelCode":null,"creditProduct":{"id":"290AA19B1134838EE053A716C0769130","swldid":"6","prodName":"dfdfd","prodType":null,"prodRate":0.13,"synFeeRate":null,"payCapitalType":null,"freezeTime":12,"prodCode":6,"prodCatgory":1,"unit":1},"subject":null},"orderFlag":null,"subject":0,"projectNum":null,"matchModeCode":"1"}]
主要代码如下:
ObjectMapper mapper = new ObjectMapper(); List<MltWaitLendReco> lendReco = mapper.readValue(listStr,new TypeReference<List<MltWaitLendReco>>() { }); System.out.println(lendReco.get(0).getId());
这样就可以把json字符串转换成想要的List.
注意readValue()方法里的new TypeReference<List<MltWaitLendReco>>() { }写法很关键,你写成List.class是不行的,
还有一点,ObjectMapper类在com.fasterxml.jackson开头的包里也有,这里用的是org.codehaus.jackson
这里还有一个以前用的,对象和Json互转的工具类
import org.codehaus.jackson.JsonGenerationException; import org.codehaus.jackson.JsonParseException; import org.codehaus.jackson.JsonParser; import org.codehaus.jackson.map.DeserializationConfig; import org.codehaus.jackson.map.JsonMappingException; import org.codehaus.jackson.map.ObjectMapper; import org.codehaus.jackson.type.TypeReference; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import java.io.IOException; public class JsonUtils { /** * Logger for this class */ private static final Logger logger = LoggerFactory.getLogger(JsonUtils.class); private final static ObjectMapper objectMapper = new ObjectMapper(); static { objectMapper.configure(JsonParser.Feature.ALLOW_COMMENTS, true); objectMapper.configure(JsonParser.Feature.ALLOW_UNQUOTED_FIELD_NAMES, true); objectMapper.configure(JsonParser.Feature.ALLOW_SINGLE_QUOTES, true); objectMapper.configure(JsonParser.Feature.ALLOW_UNQUOTED_CONTROL_CHARS, true); objectMapper.configure(JsonParser.Feature.INTERN_FIELD_NAMES, true); objectMapper.configure(JsonParser.Feature.CANONICALIZE_FIELD_NAMES, true); objectMapper.configure(DeserializationConfig.Feature.FAIL_ON_UNKNOWN_PROPERTIES, false); } public static String encode(Object obj) { try { return objectMapper.writeValueAsString(obj); } catch (JsonGenerationException e) { logger.error("encode(Object)", e); //$NON-NLS-1$ } catch (JsonMappingException e) { logger.error("encode(Object)", e); //$NON-NLS-1$ } catch (IOException e) { logger.error("encode(Object)", e); //$NON-NLS-1$ } return null; } /** * 将json string反序列化成对象 * * @param json * @param valueType * @return */ public static <T> T decode(String json, Class<T> valueType) { try { return objectMapper.readValue(json, valueType); } catch (JsonParseException e) { logger.error("decode(String, Class<T>)", e); } catch (JsonMappingException e) { logger.error("decode(String, Class<T>)", e); } catch (IOException e) { logger.error("decode(String, Class<T>)", e); } return null; } /** * 将json array反序列化为对象 * * @param json * @param jsonTypeReference * @return */ public static <T> T decode(String json, TypeReference<T> jsonTypeReference) { try { return (T) objectMapper.readValue(json, jsonTypeReference); } catch (JsonParseException e) { logger.error("decode(String, JsonTypeReference<T>)", e); } catch (JsonMappingException e) { logger.error("decode(String, JsonTypeReference<T>)", e); } catch (IOException e) { logger.error("decode(String, JsonTypeReference<T>)", e); } return null; } }
插播个广告
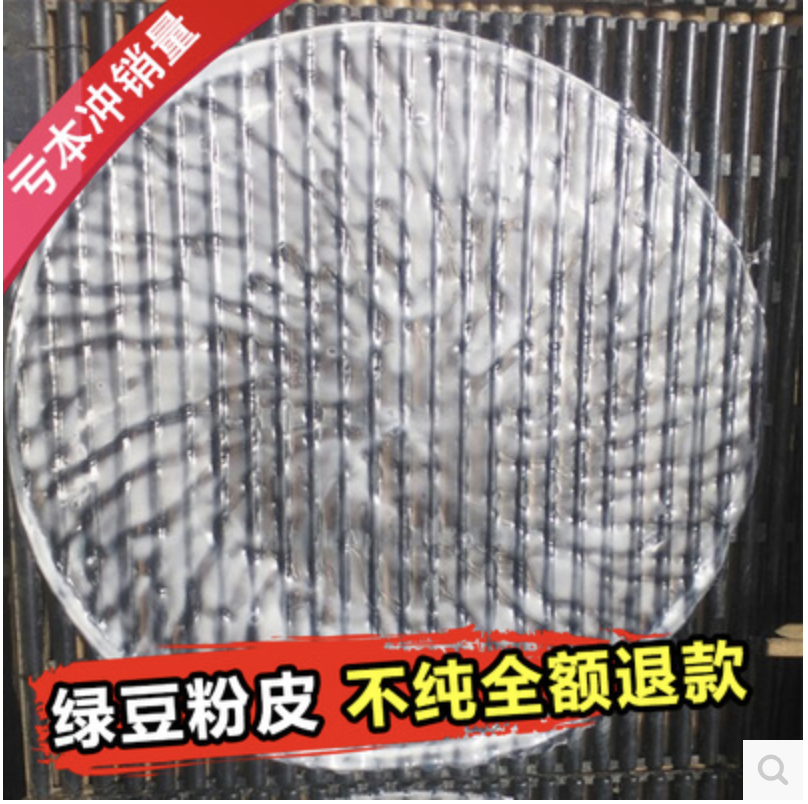
老丈人家的粉皮儿,农产品,没有乱七八糟的添加剂,欢迎惠顾