1、实验目的与要求
(1) 掌握GUI布局管理器用法;
(2) 掌握各类Java Swing组件用途及常用API;
2、实验内容和步骤
实验1: 导入第12章示例程序,测试程序并进行组内讨论。
测试程序1
l 在elipse IDE中运行教材479页程序12-1,结合运行结果理解程序;
l 掌握各种布局管理器的用法;
l 理解GUI界面中事件处理技术的用途。
l 在布局管理应用代码处添加注释;
代码:
package calculator;
import javax.swing.*;
/**
* A frame with a calculator panel.
*/
public class CalculatorFrame extends JFrame
{
public CalculatorFrame()
{
add(new CalculatorPanel());
pack();
}
}
CalculatorFrame
package calculator;
import java.awt.*;
import javax.swing.*;
/**
* @version 1.34 2015-06-12
* @author Cay Horstmann
*/
public class Calculator
{
public static void main(String[] args)
{
EventQueue.invokeLater(() -> {
CalculatorFrame frame = new CalculatorFrame();
frame.setTitle("Calculator");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
});
}
}
Calculator
package calculator;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/**
* 具有计算器按钮和结果显示的面板
*/
public class CalculatorPanel extends JPanel {
private JButton display;
private JPanel panel;
private double result;
private String lastCommand;
private boolean start;
public CalculatorPanel() {
setLayout(new BorderLayout());
result = 0;
lastCommand = "=";
start = true;
// 添加display
display = new JButton("0");
display.setEnabled(false);
add(display, BorderLayout.NORTH);
ActionListener insert = new InsertAction();
ActionListener command = new CommandAction();
// 在4×4网格中添加按钮
panel = new JPanel();
panel.setLayout(new GridLayout(4, 4));
addButton("7", insert);
addButton("8", insert);
addButton("9", insert);
addButton("/", command);
addButton("4", insert);
addButton("5", insert);
addButton("6", insert);
addButton("*", command);
addButton("1", insert);
addButton("2", insert);
addButton("3", insert);
addButton("-", command);
addButton("0", insert);
addButton(".", insert);
addButton("=", command);
addButton("+", command);
add(panel, BorderLayout.CENTER);// 把面板添加在边框布局的中央
}
/**
* 向中心面板添加一个按钮
*
* @param label the button label
* @param listener the button listener
*/
private void addButton(String label, ActionListener listener) {
JButton button = new JButton(label);
button.addActionListener(listener);
panel.add(button);
}
/**
* 此操作将按钮操作字符串插入到显示文本的末尾
*/
private class InsertAction implements ActionListener {
public void actionPerformed(ActionEvent event) {
String input = event.getActionCommand();// 标识此事件命令的字符串
if (start) {
display.setText("");
start = false;
}
display.setText(display.getText() + input);
}
}
/**
* 此操作执行按钮操作字符串所表示的命令
*/
private class CommandAction implements ActionListener {
public void actionPerformed(ActionEvent event) {
String command = event.getActionCommand();
if (start) {
if (command.equals("-"))// 负数的情况
{
display.setText(command);
start = false;
} else
lastCommand = command;
} else {
calculate(Double.parseDouble(display.getText()));// 把display中的string转换为double
lastCommand = command;
start = true;
}
}
}
/**
* 执行即将发生的计算
*
* @param x值与先前结果一起累积。
*/
public void calculate(double x) {
if (lastCommand.equals("+"))
result += x;
else if (lastCommand.equals("-"))
result -= x;
else if (lastCommand.equals("*"))
result *= x;
else if (lastCommand.equals("/"))
result /= x;
else if (lastCommand.equals("="))
result = x;
display.setText("" + result);// 在double前添加""可转换为string
}
}
CalculatorPanel
结果:
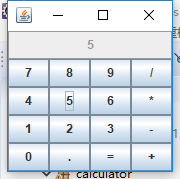
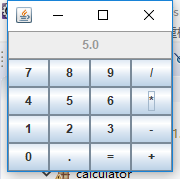
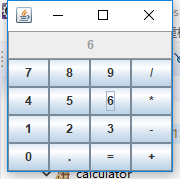
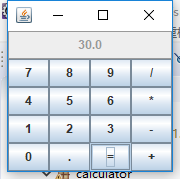
测试程序2
l 在elipse IDE中调试运行教材486页程序12-2,结合运行结果理解程序;
l 掌握各种文本组件的用法;
l 记录示例代码阅读理解中存在的问题与疑惑。
package Forth;
import java.awt.BorderLayout;
import java.awt.GridLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
/**
* A frame with sample text components.
*/
public class TextComponentFrame extends JFrame
{
public static final int TEXTAREA_ROWS = 8;
public static final int TEXTAREA_COLUMNS = 20;
public TextComponentFrame()
{
//本窗口有文本域和密码域
JTextField textField = new JTextField();
JPasswordField passwordField = new JPasswordField();
//定义一个Panel,设置了表格布局管理器并指定行与列
JPanel northPanel = new JPanel();
northPanel.setLayout(new GridLayout(2, 2));
// 添加文本域的标签
northPanel.add(new JLabel("User name: ", SwingConstants.RIGHT));
northPanel.add(textField);//将文本域添加到panel
northPanel.add(new JLabel("Password: ", SwingConstants.RIGHT));
northPanel.add(passwordField);
add(northPanel, BorderLayout.NORTH);//将pannel添加到frame
JTextArea textArea = new JTextArea(TEXTAREA_ROWS, TEXTAREA_COLUMNS);
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane, BorderLayout.CENTER);
// 添加按钮以将文本附加到文本区域
JPanel southPanel = new JPanel();
//定义一个按钮,添加到frame下方,并定义监听事件,点击按钮,文本区显示用户名与密码
JButton insertButton = new JButton("Insert");
southPanel.add(insertButton);
insertButton.addActionListener(event ->
textArea.append("User name: " + textField.getText() + " Password: "
+ new String(passwordField.getPassword()) + "
"));
add(southPanel, BorderLayout.SOUTH);
pack();
}
}
TextComponentFrame
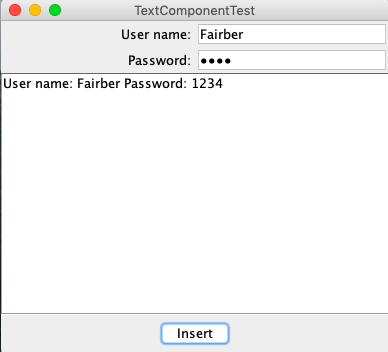
测试程序3
l 在elipse IDE中调试运行教材489页程序12-3,结合运行结果理解程序;
l 掌握复选框组件的用法;
l 记录示例代码阅读理解中存在的问题与疑惑。
package practise;
import java.awt.*;
import javax.swing.*;
/**
* @version 1.34 2015-06-12
* @author Cay Horstmann
*/
public class CheckBoxTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(() -> {
JFrame frame = new CheckBoxFrame();
frame.setTitle("CheckBoxTest");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
});
}
}
CheckBoxTest
package practise;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/**
* A frame with a sample text label and check boxes for selecting font
* attributes.
*/
public class CheckBoxFrame extends JFrame
{
private JLabel label;
private JCheckBox bold;
private JCheckBox italic;
private static final int FONTSIZE = 24;
public CheckBoxFrame()
{
// add the sample text label
//添加示例文本标签;
label = new JLabel("The quick brown fox jumps over the lazy dog.");
label.setFont(new Font("Serif", Font.BOLD, FONTSIZE));
add(label, BorderLayout.CENTER);
//此侦听器将标签的字体属性设置为复选框状态;
ActionListener listener = event -> {
int mode = 0;
if (bold.isSelected()) mode += Font.BOLD;
if (italic.isSelected()) mode += Font.ITALIC;
label.setFont(new Font("Serif", mode, FONTSIZE));
};
// 添加复选框
JPanel buttonPanel = new JPanel();
bold = new JCheckBox("Bold");
bold.addActionListener(listener);
bold.setSelected(true);
buttonPanel.add(bold);
italic = new JCheckBox("Italic");
italic.addActionListener(listener);
buttonPanel.add(italic);
add(buttonPanel, BorderLayout.SOUTH);
pack();
}
}
CheckBoxFrame
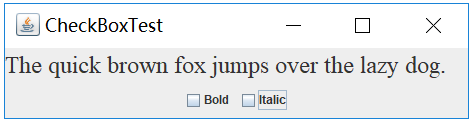
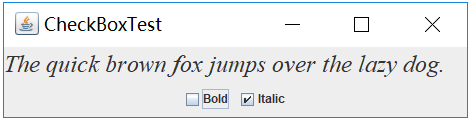
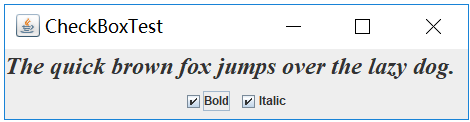
测试程序4
l 在elipse IDE中调试运行教材491页程序12-4,运行结果理解程序;
l 掌握单选按钮组件的用法;
l 记录示例代码阅读理解中存在的问题与疑惑。
package Second;
import java.awt.*;
import javax.swing.*;
/**
* @version 1.34 2015-06-12
* @author Cay Horstmann
*/
public class RadioButtonTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(() -> {
JFrame frame = new RadioButtonFrame();
frame.setTitle("RadioButtonTest");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
});
}
}
RadioButtonTest
package Second;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/**
* A frame with a sample text label and radio buttons for selecting font sizes.
*/
public class RadioButtonFrame extends JFrame
{
private JPanel buttonPanel;
private ButtonGroup group;
private JLabel label;
private static final int DEFAULT_SIZE = 36;
public RadioButtonFrame()
{
// 添加示例文本标签
label = new JLabel("The quick brown fox jumps over the lazy dog.");
label.setFont(new Font("Serif", Font.PLAIN, DEFAULT_SIZE));
add(label, BorderLayout.CENTER);
// 添加单选按钮;
buttonPanel = new JPanel();
group = new ButtonGroup();
addRadioButton("Small", 8);
addRadioButton("Medium", 12);
addRadioButton("Large", 18);
addRadioButton("Extra large", 36);
add(buttonPanel, BorderLayout.SOUTH);
pack();
}
/**
* Adds a radio button that sets the font size of the sample text.
* @param name the string to appear on the button
* @param size the font size that this button sets
*/
public void addRadioButton(String name, int size)
{
boolean selected = size == DEFAULT_SIZE;
JRadioButton button = new JRadioButton(name, selected);
group.add(button);
buttonPanel.add(button);
// 此监听器设定标签字型大小
ActionListener listener = event -> label.setFont(new Font("Serif", Font.PLAIN, size));
button.addActionListener(listener);
}
}
RadioButtonFrame
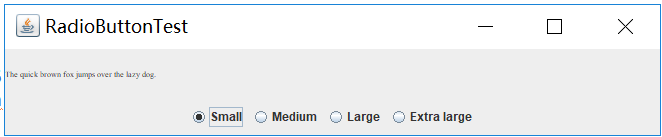
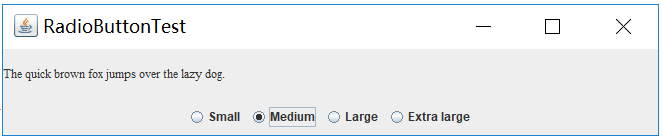
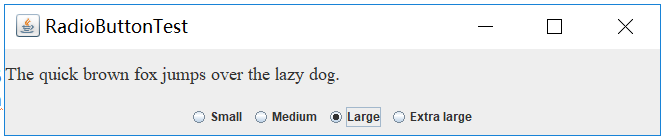
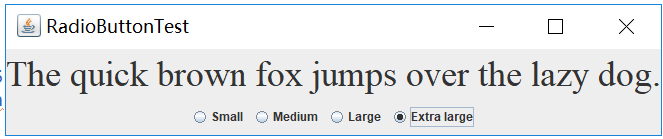
测试程序5
l 在elipse IDE中调试运行教材494页程序12-5,结合运行结果理解程序;
l 掌握边框的用法;
l 记录示例代码阅读理解中存在的问题与疑惑。
package Third;
import java.awt.*;
import javax.swing.*;
/**
* @version 1.34 2015-06-13
* @author Cay Horstmann
*/
public class BorderTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(() -> {
JFrame frame = new BorderFrame();
frame.setTitle("BorderTest");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
});
}
}
BorderTest
package Third;
import java.awt.*;
import javax.swing.*;
import javax.swing.border.*;
/**
* A frame with radio buttons to pick a border style.
*/
public class BorderFrame extends JFrame
{
private JPanel demoPanel;
private JPanel buttonPanel;
private ButtonGroup group;
public BorderFrame()
{
demoPanel = new JPanel();
buttonPanel = new JPanel();
group = new ButtonGroup();
addRadioButton("Lowered bevel", BorderFactory.createLoweredBevelBorder());//创建一个具有凹面效果的边框;
addRadioButton("Raised bevel", BorderFactory.createRaisedBevelBorder());//创建一个具有凸面效果的边框;
addRadioButton("Etched", BorderFactory.createEtchedBorder());//创建一个具有3D效果的直线边框;
addRadioButton("Line", BorderFactory.createLineBorder(Color.BLUE));//创建一个具有3D效果的蓝色直线边框;
addRadioButton("Matte", BorderFactory.createMatteBorder(10, 10, 10, 10, Color.BLUE));//创建一个用蓝色填充的粗边框;
addRadioButton("Empty", BorderFactory.createEmptyBorder());//创建一个空边框;
//将一个带有标题的蚀刻边框添加到面板;
Border etched = BorderFactory.createEtchedBorder();
Border titled = BorderFactory.createTitledBorder(etched, "Border types");
buttonPanel.setBorder(titled);
setLayout(new GridLayout(2, 1));//设置了表格布局管理器并指定行与列
add(buttonPanel);
add(demoPanel);
pack();
}
public void addRadioButton(String buttonName, Border b)
{
JRadioButton button = new JRadioButton(buttonName);
button.addActionListener(event -> demoPanel.setBorder(b));
group.add(button);
buttonPanel.add(button);
}
}
BorderFrame
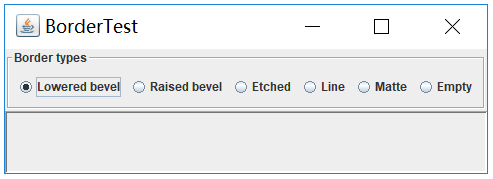
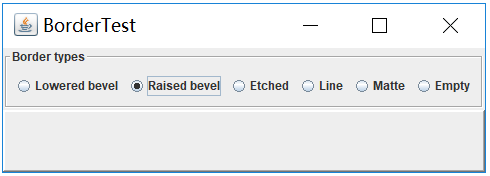
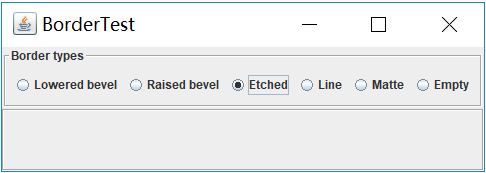
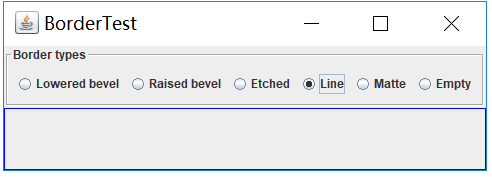
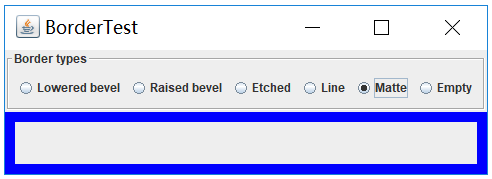
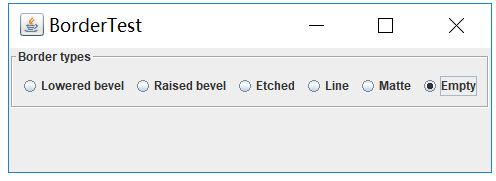
测试程序6
l 在elipse IDE中调试运行教材498页程序12-6,结合运行结果理解程序;
l 掌握组合框组件的用法;
l 记录示例代码阅读理解中存在的问题与疑惑。
package comboBox;
import java.awt.*;
import javax.swing.*;
/**
* @version 1.35 2015-06-12
* @author Cay Horstmann
*/
public class ComboBoxTest
{
public static void main(String[] args)
{
//lambda表达式
EventQueue.invokeLater(() -> {
//构造frame框架对象
JFrame frame = new ComboBoxFrame();
//设置标题
frame.setTitle("ComboBoxTest");
//设置用户在此窗体上发起 "close" 时默认执行的操作。
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//设置框架是否可见
frame.setVisible(true);
});
}
}
ComboBoxTest
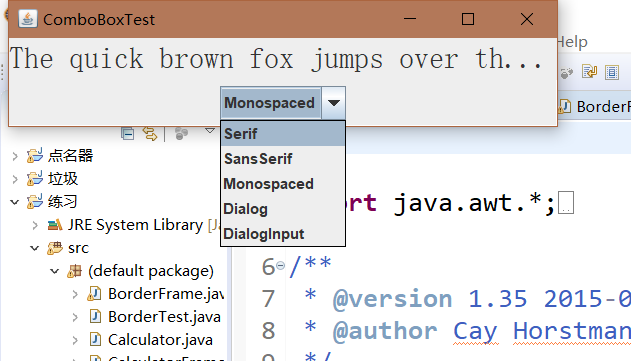
测试程序7
l 在elipse IDE中调试运行教材501页程序12-7,结合运行结果理解程序;
l 掌握滑动条组件的用法;
l 记录示例代码阅读理解中存在的问题与疑惑。
package comboBox;
import java.awt.BorderLayout;
import java.awt.Font;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
/**
* 具有示例文本标签和用于选择字体外观的组合框的框架。
* 组合框:将按钮或可编辑字段与下拉列表组合的组件。
* 用户可以从下拉列表中选择值,下拉列表在用户请求时显示。
* 如果使组合框处于可编辑状态,则组合框将包括用户可在其中键入值的可编辑字段。
*/
//ComboBoxFrame继承于JFrame类
public class ComboBoxFrame extends JFrame
{
//设置ComboBoxFrame的私有属性
private JComboBox<String> faceCombo;
private JLabel label;
private static final int DEFAULT_SIZE = 24;
public ComboBoxFrame()
{
// 添加示例文本标签
label = new JLabel("The quick brown fox jumps over the lazy dog.");
//设置字体
label.setFont(new Font("Serif", Font.PLAIN, DEFAULT_SIZE));
//添加到边框布局管理器的中间
add(label, BorderLayout.CENTER);
// 创建一个组合框对象并添加项目名称
faceCombo = new JComboBox<>();
//把一个选项添加到选项列表中,共五种选项
faceCombo.addItem("Serif");
faceCombo.addItem("SansSerif");
faceCombo.addItem("Monospaced");
faceCombo.addItem("Dialog");
faceCombo.addItem("DialogInput");
// 组合框监听器将标签字体更改为所选的名称(添加监听器,使用lambda表达式)
faceCombo.addActionListener(event ->
//设置标签的字体
label.setFont(
//getItemAt用于返回指定索引处的列表项;getSelectedIndex用于返回当前选择的选项
new Font(faceCombo.getItemAt(faceCombo.getSelectedIndex()),
Font.PLAIN, DEFAULT_SIZE)));
// 将组合框添加到框架南部边界的面板
JPanel comboPanel = new JPanel();
comboPanel.add(faceCombo);
add(comboPanel, BorderLayout.SOUTH);
pack();
}
}
ComboBoxFrame
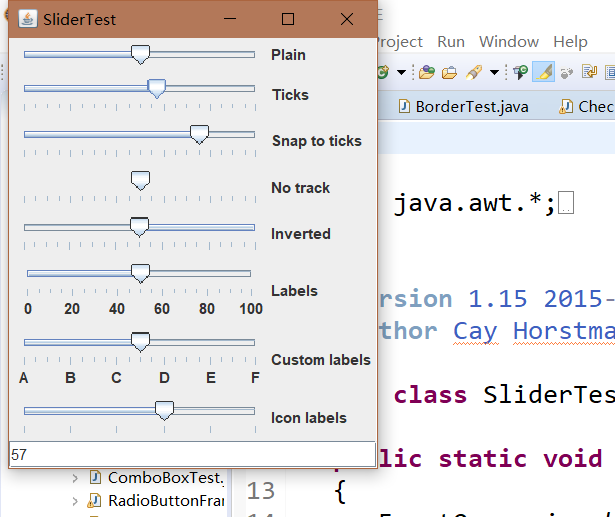
测试程序8
l 在elipse IDE中调试运行教材512页程序12-8,结合运行结果理解程序;
l 掌握菜单的创建、菜单事件监听器、复选框和单选按钮菜单项、弹出菜单以及快捷键和加速器的用法。
l 记录示例代码阅读理解中存在的问题与疑惑。
package menu;
import java.awt.event.*;
import javax.swing.*;
/**
* A frame with a sample menu bar.
*/
public class MenuFrame extends JFrame
{
private static final int DEFAULT_WIDTH = 300;
private static final int DEFAULT_HEIGHT = 200;
private Action saveAction;
private Action saveAsAction;
private JCheckBoxMenuItem readonlyItem;
private JPopupMenu popup;
/**
* A sample action that prints the action name to System.out
*/
class TestAction extends AbstractAction
{
public TestAction(String name)
{
super(name);
}
public void actionPerformed(ActionEvent event)
{
System.out.println(getValue(Action.NAME) + " selected.");
}
}
public MenuFrame()
{
setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT);
JMenu fileMenu = new JMenu("File");
fileMenu.add(new TestAction("New"));
// demonstrate accelerators
JMenuItem openItem = fileMenu.add(new TestAction("Open"));
openItem.setAccelerator(KeyStroke.getKeyStroke("ctrl O"));
fileMenu.addSeparator();
saveAction = new TestAction("Save");
JMenuItem saveItem = fileMenu.add(saveAction);
saveItem.setAccelerator(KeyStroke.getKeyStroke("ctrl S"));
saveAsAction = new TestAction("Save As");
fileMenu.add(saveAsAction);
fileMenu.addSeparator();
fileMenu.add(new AbstractAction("Exit")
{
public void actionPerformed(ActionEvent event)
{
System.exit(0);
}
});
// demonstrate checkbox and radio button menus
readonlyItem = new JCheckBoxMenuItem("Read-only");
readonlyItem.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
boolean saveOk = !readonlyItem.isSelected();
saveAction.setEnabled(saveOk);
saveAsAction.setEnabled(saveOk);
}
});
ButtonGroup group = new ButtonGroup();
JRadioButtonMenuItem insertItem = new JRadioButtonMenuItem("Insert");
insertItem.setSelected(true);
JRadioButtonMenuItem overtypeItem = new JRadioButtonMenuItem("Overtype");
group.add(insertItem);
group.add(overtypeItem);
// demonstrate icons
Action cutAction = new TestAction("Cut");
cutAction.putValue(Action.SMALL_ICON, new ImageIcon("cut.gif"));
Action copyAction = new TestAction("Copy");
copyAction.putValue(Action.SMALL_ICON, new ImageIcon("copy.gif"));
Action pasteAction = new TestAction("Paste");
pasteAction.putValue(Action.SMALL_ICON, new ImageIcon("paste.gif"));
JMenu editMenu = new JMenu("Edit");
editMenu.add(cutAction);
editMenu.add(copyAction);
editMenu.add(pasteAction);
// demonstrate nested menus
JMenu optionMenu = new JMenu("Options");
optionMenu.add(readonlyItem);
optionMenu.addSeparator();
optionMenu.add(insertItem);
optionMenu.add(overtypeItem);
editMenu.addSeparator();
editMenu.add(optionMenu);
// demonstrate mnemonics
JMenu helpMenu = new JMenu("Help");
helpMenu.setMnemonic('H');
JMenuItem indexItem = new JMenuItem("Index");
indexItem.setMnemonic('I');
helpMenu.add(indexItem);
// you can also add the mnemonic key to an action
Action aboutAction = new TestAction("About");
aboutAction.putValue(Action.MNEMONIC_KEY, new Integer('A'));
helpMenu.add(aboutAction);
// add all top-level menus to menu bar
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
menuBar.add(fileMenu);
menuBar.add(editMenu);
menuBar.add(helpMenu);
// demonstrate pop-ups
popup = new JPopupMenu();
popup.add(cutAction);
popup.add(copyAction);
popup.add(pasteAction);
JPanel panel = new JPanel();
panel.setComponentPopupMenu(popup);
add(panel);
}
}
package menu;
import java.awt.*;
import javax.swing.*;
/**
* @version 1.24 2012-06-12
* @author Cay Horstmann
*/
public class MenuTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(() -> {
JFrame frame = new MenuFrame();
frame.setTitle("MenuTest");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
});
}
}
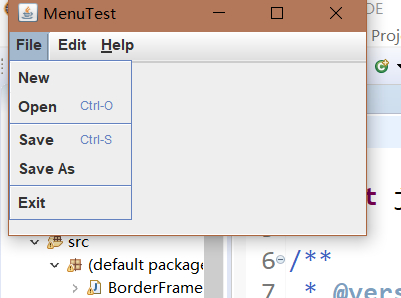
测试程序9
l 在elipse IDE中调试运行教材517页程序12-9,结合运行结果理解程序;
l 掌握工具栏和工具提示的用法;
l 记录示例代码阅读理解中存在的问题与疑惑。
package toolBar;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/**
* A frame with a toolbar and menu for color changes.
*/
public class ToolBarFrame extends JFrame
{ //定义两个私有属性
private static final int DEFAULT_WIDTH = 300;
private static final int DEFAULT_HEIGHT = 200;
private JPanel panel;
public ToolBarFrame() //定义工具提示类
{
setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT);
// add a panel for color change
panel = new JPanel();//创建新的JPanel
add(panel, BorderLayout.CENTER);
// set up actions
//建立动作
Action blueAction = new ColorAction("Blue", new ImageIcon("blue-ball.gif"), Color.BLUE);
Action yellowAction = new ColorAction("Yellow", new ImageIcon("yellow-ball.gif"),
Color.YELLOW);
Action redAction = new ColorAction("Red", new ImageIcon("red-ball.gif"), Color.RED);
Action exitAction = new AbstractAction("Exit", new ImageIcon("exit.gif"))
{
public void actionPerformed(ActionEvent event)
{
System.exit(0);
}
};
exitAction.putValue(Action.SHORT_DESCRIPTION, "Exit");
// populate toolbar
JToolBar bar = new JToolBar();
bar.add(blueAction);//用Action对象填充工具栏
bar.add(yellowAction);
bar.add(redAction);
bar.addSeparator();//用分隔符将按钮分组
bar.add(exitAction);
add(bar, BorderLayout.NORTH);
// populate menu
JMenu menu = new JMenu("Color");//显示颜色的菜单
menu.add(yellowAction);//在菜单添加颜色动作
menu.add(blueAction);
menu.add(redAction);
menu.add(exitAction);
JMenuBar menuBar = new JMenuBar();
menuBar.add(menu);
setJMenuBar(menuBar);
}
/**
* The color action sets the background of the frame to a given color.
*/
class ColorAction extends AbstractAction
{
public ColorAction(String name, Icon icon, Color c)
{
putValue(Action.NAME, name);//动作名称,显示在按钮和菜单
putValue(Action.SMALL_ICON, icon);//存储小图标的地方;显示在按钮、菜单项或工具栏中
putValue(Action.SHORT_DESCRIPTION, name + " background");
putValue("Color", c);
}
public void actionPerformed(ActionEvent event)
{
Color c = (Color) getValue("Color");
panel.setBackground(c);
}
}
}
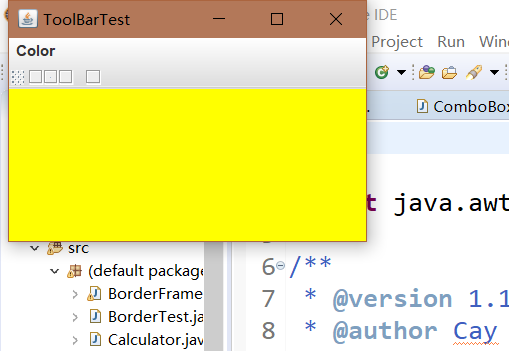
测试程序10
l 在elipse IDE中调试运行教材524页程序12-10、12-11,结合运行结果理解程序,了解GridbagLayout的用法。
l 在elipse IDE中调试运行教材533页程序12-12,结合程序运行结果理解程序,了解GroupLayout的用法。
l 记录示例代码阅读理解中存在的问题与疑惑。
import java.awt.Font;
import java.awt.GridBagLayout;
import java.awt.event.ActionListener;
import javax.swing.BorderFactory;
import javax.swing.JCheckBox;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextArea;
/**
* A frame that uses a grid bag layout to arrange font selection components.
*/
public class FontFrame extends JFrame
{
public static final int TEXT_ROWS = 10;
public static final int TEXT_COLUMNS = 20;
private JComboBox<String> face;
private JComboBox<Integer> size;
private JCheckBox bold;
private JCheckBox italic;
private JTextArea sample;
public FontFrame()
{
GridBagLayout layout = new GridBagLayout();
setLayout(layout);
ActionListener listener = event -> updateSample();
// construct components
JLabel faceLabel = new JLabel("Face: ");
face = new JComboBox<>(new String[] { "Serif", "SansSerif", "Monospaced",
"Dialog", "DialogInput" });
face.addActionListener(listener);
JLabel sizeLabel = new JLabel("Size: ");
size = new JComboBox<>(new Integer[] { 8, 10, 12, 15, 18, 24, 36, 48 });
size.addActionListener(listener);
bold = new JCheckBox("Bold");
bold.addActionListener(listener);
italic = new JCheckBox("Italic");
italic.addActionListener(listener);
sample = new JTextArea(TEXT_ROWS, TEXT_COLUMNS);
sample.setText("The quick brown fox jumps over the lazy dog");
sample.setEditable(false);
sample.setLineWrap(true);
sample.setBorder(BorderFactory.createEtchedBorder());
// add components to grid, using GBC convenience class
add(faceLabel, new GBC(0, 0).setAnchor(GBC.EAST));
add(face, new GBC(1, 0).setFill(GBC.HORIZONTAL).setWeight(100, 0)
.setInsets(1));
add(sizeLabel, new GBC(0, 1).setAnchor(GBC.EAST));
add(size, new GBC(1, 1).setFill(GBC.HORIZONTAL).setWeight(100, 0)
.setInsets(1));
add(bold, new GBC(0, 2, 2, 1).setAnchor(GBC.CENTER).setWeight(100, 100));
add(italic, new GBC(0, 3, 2, 1).setAnchor(GBC.CENTER).setWeight(100, 100));
add(sample, new GBC(2, 0, 1, 4).setFill(GBC.BOTH).setWeight(100, 100));
pack();
updateSample();
}
public void updateSample()
{
String fontFace = (String) face.getSelectedItem();
int fontStyle = (bold.isSelected() ? Font.BOLD : 0)
+ (italic.isSelected() ? Font.ITALIC : 0);
int fontSize = size.getItemAt(size.getSelectedIndex());
Font font = new Font(fontFace, fontStyle, fontSize);
sample.setFont(font);
sample.repaint();
}
}
import java.awt.*;
/**
* This class simplifies the use of the GridBagConstraints class.
* @version 1.01 2004-05-06
* @author Cay Horstmann
*/
public class GBC extends GridBagConstraints
{
/**
* Constructs a GBC with a given gridx and gridy position and all other grid
* bag constraint values set to the default.
* @param gridx the gridx position
* @param gridy the gridy position
*/
public GBC(int gridx, int gridy)
{
this.gridx = gridx;
this.gridy = gridy;
}
/**
* Constructs a GBC with given gridx, gridy, gridwidth, gridheight and all
* other grid bag constraint values set to the default.
* @param gridx the gridx position
* @param gridy the gridy position
* @param gridwidth the cell span in x-direction
* @param gridheight the cell span in y-direction
*/
public GBC(int gridx, int gridy, int gridwidth, int gridheight)
{
this.gridx = gridx;
this.gridy = gridy;
this.gridwidth = gridwidth;
this.gridheight = gridheight;
}
/**
* Sets the anchor.
* @param anchor the anchor value
* @return this object for further modification
*/
public GBC setAnchor(int anchor)
{
this.anchor = anchor;
return this;
}
/**
* Sets the fill direction.
* @param fill the fill direction
* @return this object for further modification
*/
public GBC setFill(int fill)
{
this.fill = fill;
return this;
}
/**
* Sets the cell weights.
* @param weightx the cell weight in x-direction
* @param weighty the cell weight in y-direction
* @return this object for further modification
*/
public GBC setWeight(double weightx, double weighty)
{
this.weightx = weightx;
this.weighty = weighty;
return this;
}
/**
* Sets the insets of this cell.
* @param distance the spacing to use in all directions
* @return this object for further modification
*/
public GBC setInsets(int distance)
{
this.insets = new Insets(distance, distance, distance, distance);
return this;
}
/**
* Sets the insets of this cell.
* @param top the spacing to use on top
* @param left the spacing to use to the left
* @param bottom the spacing to use on the bottom
* @param right the spacing to use to the right
* @return this object for further modification
*/
public GBC setInsets(int top, int left, int bottom, int right)
{
this.insets = new Insets(top, left, bottom, right);
return this;
}
/**
* Sets the internal padding
* @param ipadx the internal padding in x-direction
* @param ipady the internal padding in y-direction
* @return this object for further modification
*/
public GBC setIpad(int ipadx, int ipady)
{
this.ipadx = ipadx;
this.ipady = ipady;
return this;
}
}
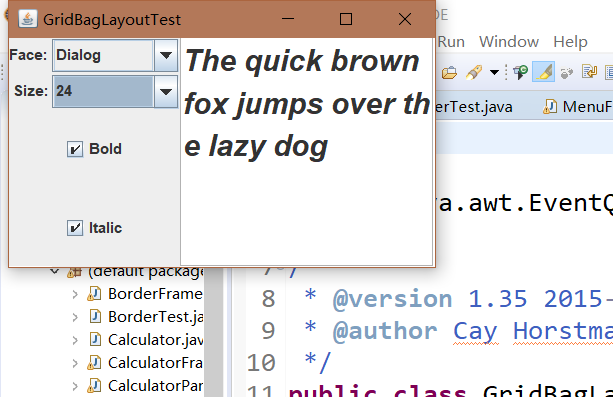
测试程序11
l 在elipse IDE中调试运行教材539页程序12-13、12-14,结合运行结果理解程序;
l 掌握定制布局管理器的用法。
l 记录示例代码阅读理解中存在的问题与疑惑。
测试程序12
l 在elipse IDE中调试运行教材544页程序12-15、12-16,结合运行结果理解程序;
l 掌握选项对话框的用法。
l 记录示例代码阅读理解中存在的问题与疑惑。
package optionDialog;
import java.awt.*;
import java.awt.event.*;
import java.awt.geom.*;
import java.util.*;
import javax.swing.*;
/**
* A frame that contains settings for selecting various option dialogs.
*/
public class OptionDialogFrame extends JFrame//对话框
{ //定义属性
private ButtonPanel typePanel;
private ButtonPanel messagePanel;
private ButtonPanel messageTypePanel;
private ButtonPanel optionTypePanel;
private ButtonPanel optionsPanel;
private ButtonPanel inputPanel;
private String messageString = "Message";
private Icon messageIcon = new ImageIcon("blue-ball.gif");
private Object messageObject = new Date();
private Component messageComponent = new SampleComponent();
public OptionDialogFrame()
{
JPanel gridPanel = new JPanel();
gridPanel.setLayout(new GridLayout(2, 3));
typePanel = new ButtonPanel("Type", "Message", "Confirm", "Option", "Input");
messageTypePanel = new ButtonPanel("Message Type", "ERROR_MESSAGE", "INFORMATION_MESSAGE",
"WARNING_MESSAGE", "QUESTION_MESSAGE", "PLAIN_MESSAGE");
messagePanel = new ButtonPanel("Message", "String", "Icon", "Component", "Other",
"Object[]");
optionTypePanel = new ButtonPanel("Confirm", "DEFAULT_OPTION", "YES_NO_OPTION",
"YES_NO_CANCEL_OPTION", "OK_CANCEL_OPTION");
optionsPanel = new ButtonPanel("Option", "String[]", "Icon[]", "Object[]");
inputPanel = new ButtonPanel("Input", "Text field", "Combo box");
gridPanel.add(typePanel);
gridPanel.add(messageTypePanel);
gridPanel.add(messagePanel);
gridPanel.add(optionTypePanel);
gridPanel.add(optionsPanel);
gridPanel.add(inputPanel);
// add a panel with a Show button
JPanel showPanel = new JPanel();
JButton showButton = new JButton("Show");
showButton.addActionListener(new ShowAction());
showPanel.add(showButton);
add(gridPanel, BorderLayout.CENTER);
add(showPanel, BorderLayout.SOUTH);
pack();
}
/**
* Gets the currently selected message.
* @return a string, icon, component, or object array, depending on the Message panel selection
*/
public Object getMessage()
{
String s = messagePanel.getSelection();
if (s.equals("String")) return messageString;
else if (s.equals("Icon")) return messageIcon;
else if (s.equals("Component")) return messageComponent;
else if (s.equals("Object[]")) return new Object[] { messageString, messageIcon,
messageComponent, messageObject };
else if (s.equals("Other")) return messageObject;
else return null;
}
/**
* Gets the currently selected options.
* @return an array of strings, icons, or objects, depending on the Option panel selection
*/
public Object[] getOptions()
{
String s = optionsPanel.getSelection();
if (s.equals("String[]")) return new String[] { "Yellow", "Blue", "Red" };
else if (s.equals("Icon[]")) return new Icon[] { new ImageIcon("yellow-ball.gif"),
new ImageIcon("blue-ball.gif"), new ImageIcon("red-ball.gif") };
else if (s.equals("Object[]")) return new Object[] { messageString, messageIcon,
messageComponent, messageObject };
else return null;
}
/**
* Gets the selected message or option type
* @param panel the Message Type or Confirm panel
* @return the selected XXX_MESSAGE or XXX_OPTION constant from the JOptionPane class
*/
public int getType(ButtonPanel panel)
{
String s = panel.getSelection();
try
{
return JOptionPane.class.getField(s).getInt(null);
}
catch (Exception e)
{
return -1;
}
}
/**
* The action listener for the Show button shows a Confirm, Input, Message, or Option dialog
* depending on the Type panel selection.
*/
private class ShowAction implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
if (typePanel.getSelection().equals("Confirm")) JOptionPane.showConfirmDialog(
OptionDialogFrame.this, getMessage(), "Title", getType(optionTypePanel),
getType(messageTypePanel));
else if (typePanel.getSelection().equals("Input"))
{
if (inputPanel.getSelection().equals("Text field")) JOptionPane.showInputDialog(
OptionDialogFrame.this, getMessage(), "Title", getType(messageTypePanel));
else JOptionPane.showInputDialog(OptionDialogFrame.this, getMessage(), "Title",
getType(messageTypePanel), null, new String[] { "Yellow", "Blue", "Red" },
"Blue");
}
else if (typePanel.getSelection().equals("Message")) JOptionPane.showMessageDialog(
OptionDialogFrame.this, getMessage(), "Title", getType(messageTypePanel));
else if (typePanel.getSelection().equals("Option")) JOptionPane.showOptionDialog(
OptionDialogFrame.this, getMessage(), "Title", getType(optionTypePanel),
getType(messageTypePanel), null, getOptions(), getOptions()[0]);
}
}
}
/**
* A component with a painted surface
*/
class SampleComponent extends JComponent
{
public void paintComponent(Graphics g)
{
Graphics2D g2 = (Graphics2D) g;
Rectangle2D rect = new Rectangle2D.Double(0, 0, getWidth() - 1, getHeight() - 1);
g2.setPaint(Color.YELLOW);
g2.fill(rect);
g2.setPaint(Color.BLUE);
g2.draw(rect);
}
public Dimension getPreferredSize()
{
return new Dimension(10, 10);
}
}
package optionDialog;
import java.awt.*;
import javax.swing.*;
/**
* @version 1.34 2015-06-12
* @author Cay Horstmann
*/
public class OptionDialogTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(() -> {
JFrame frame = new OptionDialogFrame();
frame.setTitle("OptionDialogTest");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
});
}
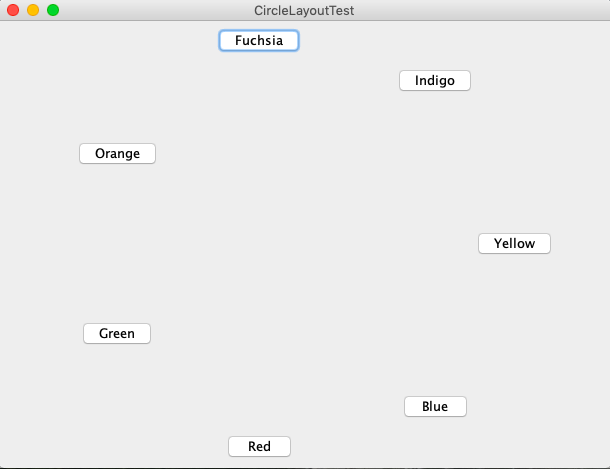
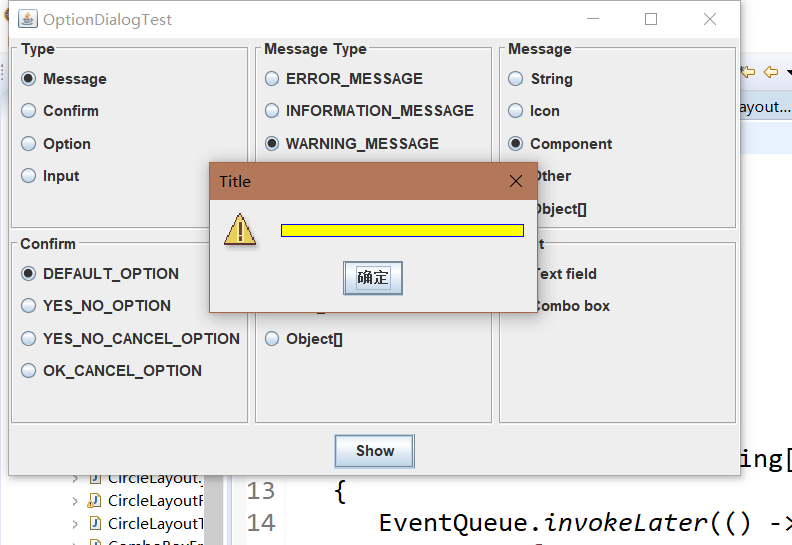
测试程序13
l 在elipse IDE中调试运行教材552页程序12-17、12-18,结合运行结果理解程序;
l 掌握对话框的创建方法;
l 记录示例代码阅读理解中存在的问题与疑惑。
package 课本示例13;
import java.awt.*;
import javax.swing.*;
/**
* @version 1.34 2015-06-12
* @author Cay Horstmann
*/
public class OptionDialogTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(() -> {
JFrame frame = new OptionDialogFrame();
frame.setTitle("OptionDialogTest");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
});
}
}
package 课本示例13;
import java.awt.*;
import java.awt.event.*;
import java.awt.geom.*;
import java.util.*;
import javax.swing.*;
/**
* 包含用于选择各种选项对话框的设置的框架。
*/
public class OptionDialogFrame extends JFrame
{
private ButtonPanel typePanel;
private ButtonPanel messagePanel;
private ButtonPanel messageTypePanel;
private ButtonPanel optionTypePanel;
private ButtonPanel optionsPanel;
private ButtonPanel inputPanel;
private String messageString = "Message";
private Icon messageIcon = new ImageIcon("blue-ball.gif");
private Object messageObject = new Date();
private Component messageComponent = new SampleComponent();
public OptionDialogFrame()
{
JPanel gridPanel = new JPanel();
gridPanel.setLayout(new GridLayout(2, 3));
typePanel = new ButtonPanel("Type", "Message", "Confirm", "Option", "Input");
messageTypePanel = new ButtonPanel("Message Type", "ERROR_MESSAGE", "INFORMATION_MESSAGE",
"WARNING_MESSAGE", "QUESTION_MESSAGE", "PLAIN_MESSAGE");
messagePanel = new ButtonPanel("Message", "String", "Icon", "Component", "Other",
"Object[]");
optionTypePanel = new ButtonPanel("Confirm", "DEFAULT_OPTION", "YES_NO_OPTION",
"YES_NO_CANCEL_OPTION", "OK_CANCEL_OPTION");
optionsPanel = new ButtonPanel("Option", "String[]", "Icon[]", "Object[]");
inputPanel = new ButtonPanel("Input", "Text field", "Combo box");
gridPanel.add(typePanel);
gridPanel.add(messageTypePanel);
gridPanel.add(messagePanel);
gridPanel.add(optionTypePanel);
gridPanel.add(optionsPanel);
gridPanel.add(inputPanel);
// add a panel with a Show button
JPanel showPanel = new JPanel();
JButton showButton = new JButton("Show");
showButton.addActionListener(new ShowAction());
showPanel.add(showButton);
add(gridPanel, BorderLayout.CENTER);
add(showPanel, BorderLayout.SOUTH);
pack();
}
/**
* Gets the currently selected message.
* @return a string, icon, component, or object array, depending on the Message panel selection
*/
public Object getMessage()
{
String s = messagePanel.getSelection();
if (s.equals("String")) return messageString;
else if (s.equals("Icon")) return messageIcon;
else if (s.equals("Component")) return messageComponent;
else if (s.equals("Object[]")) return new Object[] { messageString, messageIcon,
messageComponent, messageObject };
else if (s.equals("Other")) return messageObject;
else return null;
}
/**
* Gets the currently selected options.
* @return an array of strings, icons, or objects, depending on the Option panel selection
*/
public Object[] getOptions()
{
String s = optionsPanel.getSelection();
if (s.equals("String[]")) return new String[] { "Yellow", "Blue", "Red" };
else if (s.equals("Icon[]")) return new Icon[] { new ImageIcon("yellow-ball.gif"),
new ImageIcon("blue-ball.gif"), new ImageIcon("red-ball.gif") };
else if (s.equals("Object[]")) return new Object[] { messageString, messageIcon,
messageComponent, messageObject };
else return null;
}
/**
* 获取所选消息或选项类型
* @param面板消息类型或确认面板
* @return JOptionPane类中选定的XXX_MESSAGE或XXX_OPTION常量
*/
public int getType(ButtonPanel panel)
{
String s = panel.getSelection();
try
{
return JOptionPane.class.getField(s).getInt(null);
}
catch (Exception e)
{
return -1;
}
}
/**
* “显示”按钮的动作侦听器显示“确认”,“输入”,“消息”或“选项”对话框
*取决于“类型”面板选择。
*/
private class ShowAction implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
if (typePanel.getSelection().equals("Confirm")) JOptionPane.showConfirmDialog(
OptionDialogFrame.this, getMessage(), "Title", getType(optionTypePanel),
getType(messageTypePanel));
else if (typePanel.getSelection().equals("Input"))
{
if (inputPanel.getSelection().equals("Text field")) JOptionPane.showInputDialog(
OptionDialogFrame.this, getMessage(), "Title", getType(messageTypePanel));
else JOptionPane.showInputDialog(OptionDialogFrame.this, getMessage(), "Title",
getType(messageTypePanel), null, new String[] { "Yellow", "Blue", "Red" },
"Blue");
}
else if (typePanel.getSelection().equals("Message")) JOptionPane.showMessageDialog(
OptionDialogFrame.this, getMessage(), "Title", getType(messageTypePanel));
else if (typePanel.getSelection().equals("Option")) JOptionPane.showOptionDialog(
OptionDialogFrame.this, getMessage(), "Title", getType(optionTypePanel),
getType(messageTypePanel), null, getOptions(), getOptions()[0]);
}
}
}
/**
* 具有涂漆表面的组件
*/
class SampleComponent extends JComponent
{
public void paintComponent(Graphics g)
{
Graphics2D g2 = (Graphics2D) g;
Rectangle2D rect = new Rectangle2D.Double(0, 0, getWidth() - 1, getHeight() - 1);
g2.setPaint(Color.YELLOW);
g2.fill(rect);
g2.setPaint(Color.BLUE);
g2.draw(rect);
}
public Dimension getPreferredSize()
{
return new Dimension(10, 10);
}
}
package 课本示例13;
import javax.swing.*;
/**
* 带标题边框内的单选按钮的面板.
*/
public class ButtonPanel extends JPanel
{
private ButtonGroup group;
/**
* 构造一个按钮面板.
* @param标题边框中显示的标题
* @param选项一组单选按钮标签
*/
public ButtonPanel(String title, String... options)
{
setBorder(BorderFactory.createTitledBorder(BorderFactory.createEtchedBorder(), title));
setLayout(new BoxLayout(this, BoxLayout.Y_AXIS));
group = new ButtonGroup();
// make one radio button for each option
for (String option : options)
{
JRadioButton b = new JRadioButton(option);
b.setActionCommand(option);
add(b);
group.add(b);
b.setSelected(option == options[0]);
}
}
/**
* 获取当前选定的选项。
* @return当前所选单选按钮的标签。
*/
public String getSelection()
{
return group.getSelection().getActionCommand();
}
}
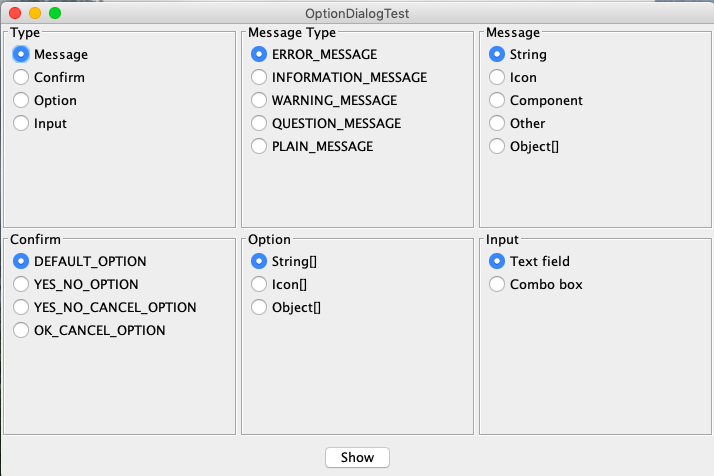
测试程序14
l 在elipse IDE中调试运行教材556页程序12-19、12-20,结合运行结果理解程序;
l 掌握对话框的数据交换用法;
l 记录示例代码阅读理解中存在的问题与疑惑。
package dataExchange;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/**
* A frame with a menu whose File->Connect action shows a password dialog.
*/
public class DataExchangeFrame extends JFrame
{
public static final int TEXT_ROWS = 20;
public static final int TEXT_COLUMNS = 40;
private PasswordChooser dialog = null;
private JTextArea textArea;
public DataExchangeFrame()
{
// construct a File menu
JMenuBar mbar = new JMenuBar();
setJMenuBar(mbar);
JMenu fileMenu = new JMenu("File");
mbar.add(fileMenu);
// add Connect and Exit menu items
JMenuItem connectItem = new JMenuItem("Connect");
connectItem.addActionListener(new ConnectAction());
fileMenu.add(connectItem);
// The Exit item exits the program
JMenuItem exitItem = new JMenuItem("Exit");
exitItem.addActionListener(event -> System.exit(0));
fileMenu.add(exitItem);
textArea = new JTextArea(TEXT_ROWS, TEXT_COLUMNS);
add(new JScrollPane(textArea), BorderLayout.CENTER);
pack();
}
/**
* The Connect action pops up the password dialog.
*/
private class ConnectAction implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
// if first time, construct dialog
if (dialog == null) dialog = new PasswordChooser();
// set default values
dialog.setUser(new User("yourname", null));
// pop up dialog
if (dialog.showDialog(DataExchangeFrame.this, "Connect"))
{
// if accepted, retrieve user input
User u = dialog.getUser();
textArea.append("user name = " + u.getName() + ", password = "
+ (new String(u.getPassword())) + "
");
}
}
}
}
测试程序15
l 在elipse IDE中调试运行教材556页程序12-21、12-2212-23,结合程序运行结果理解程序;
l 掌握文件对话框的用法;
l 记录示例代码阅读理解中存在的问题与疑惑。
package dataExchange;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/**
* A frame with a menu whose File->Connect action shows a password dialog.
*/
public class DataExchangeFrame extends JFrame
{
public static final int TEXT_ROWS = 20;
public static final int TEXT_COLUMNS = 40;
private PasswordChooser dialog = null;
private JTextArea textArea;
public DataExchangeFrame()
{
// construct a File menu
JMenuBar mbar = new JMenuBar();
setJMenuBar(mbar);
JMenu fileMenu = new JMenu("File");
mbar.add(fileMenu);
// add Connect and Exit menu items
JMenuItem connectItem = new JMenuItem("Connect");
connectItem.addActionListener(new ConnectAction());
fileMenu.add(connectItem);
// The Exit item exits the program
JMenuItem exitItem = new JMenuItem("Exit");
exitItem.addActionListener(event -> System.exit(0));
fileMenu.add(exitItem);
textArea = new JTextArea(TEXT_ROWS, TEXT_COLUMNS);
add(new JScrollPane(textArea), BorderLayout.CENTER);
pack();
}
/**
* The Connect action pops up the password dialog.
*/
private class ConnectAction implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
// if first time, construct dialog
if (dialog == null) dialog = new PasswordChooser();
// set default values
dialog.setUser(new User("yourname", null));
// pop up dialog
if (dialog.showDialog(DataExchangeFrame.this, "Connect"))
{
// if accepted, retrieve user input
User u = dialog.getUser();
textArea.append("user name = " + u.getName() + ", password = "
+ (new String(u.getPassword())) + "
");
}
}
}
}
package dataExchange;
import java.awt.BorderLayout;
import java.awt.Component;
import java.awt.Frame;
import java.awt.GridLayout;
import javax.swing.JButton;
import javax.swing.JDialog;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
import javax.swing.SwingUtilities;
/**
* A password chooser that is shown inside a dialog
*/
public class PasswordChooser extends JPanel
{
private JTextField username;
private JPasswordField password;
private JButton okButton;
private boolean ok;
private JDialog dialog;
public PasswordChooser()
{
setLayout(new BorderLayout());
// construct a panel with user name and password fields
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(2, 2));
panel.add(new JLabel("User name:"));
panel.add(username = new JTextField(""));
panel.add(new JLabel("Password:"));
panel.add(password = new JPasswordField(""));
add(panel, BorderLayout.CENTER);
// create Ok and Cancel buttons that terminate the dialog
okButton = new JButton("Ok");
okButton.addActionListener(event -> {
ok = true;
dialog.setVisible(false);
});
JButton cancelButton = new JButton("Cancel");
cancelButton.addActionListener(event -> dialog.setVisible(false));
// add buttons to southern border
JPanel buttonPanel = new JPanel();
buttonPanel.add(okButton);
buttonPanel.add(cancelButton);
add(buttonPanel, BorderLayout.SOUTH);
}
/**
* Sets the dialog defaults.
* @param u the default user information
*/
public void setUser(User u)
{
username.setText(u.getName());
}
/**
* Gets the dialog entries.
* @return a User object whose state represents the dialog entries
*/
public User getUser()
{
return new User(username.getText(), password.getPassword());
}
/**
* Show the chooser panel in a dialog
* @param parent a component in the owner frame or null
* @param title the dialog window title
*/
public boolean showDialog(Component parent, String title)
{
ok = false;
// locate the owner frame
Frame owner = null;
if (parent instanceof Frame)
owner = (Frame) parent;
else
owner = (Frame) SwingUtilities.getAncestorOfClass(Frame.class, parent);
// if first time, or if owner has changed, make new dialog
if (dialog == null || dialog.getOwner() != owner)
{
dialog = new JDialog(owner, true);
dialog.add(this);
dialog.getRootPane().setDefaultButton(okButton);
dialog.pack();
}
// set title and show dialog
dialog.setTitle(title);
dialog.setVisible(true);
return ok;
}
}
package dataExchange;
/**
* A user has a name and password. For security reasons, the password is stored as a char[], not a
* String.
*/
public class User
{
private String name;
private char[] password;
public User(String aName, char[] aPassword)
{
name = aName;
password = aPassword;
}
public String getName()
{
return name;
}
public char[] getPassword()
{
return password;
}
public void setName(String aName)
{
name = aName;
}
public void setPassword(char[] aPassword)
{
password = aPassword;
}
}
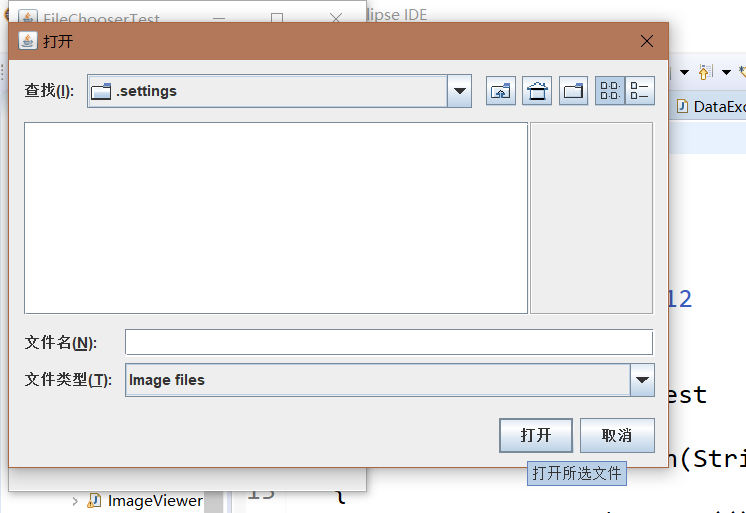
测试程序16
l 在elipse IDE中调试运行教材570页程序12-24,结合运行结果理解程序;
l 了解颜色选择器的用法。
记录示例代码阅读理解中存在的问题与疑惑。
package colorChooser;
import java.awt.Color;
import java.awt.Frame;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JColorChooser;
import javax.swing.JDialog;
import javax.swing.JPanel;
/**
* A panel with buttons to pop up three types of color choosers
*/
public class ColorChooserPanel extends JPanel
{
public ColorChooserPanel()//狗仔一个初始颜色的颜色选择器
{
JButton modalButton = new JButton("Modal");
modalButton.addActionListener(new ModalListener());
add(modalButton);
JButton modelessButton = new JButton("Modeless");
modelessButton.addActionListener(new ModelessListener());
add(modelessButton);
JButton immediateButton = new JButton("Immediate");
immediateButton.addActionListener(new ImmediateListener());
add(immediateButton);
}
/**
* This listener pops up a modal color chooser
*/
private class ModalListener implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
Color defaultColor = getBackground();
Color selected = JColorChooser.showDialog(ColorChooserPanel.this, "Set background",
defaultColor);
if (selected != null) setBackground(selected);
}
}
/**
* This listener pops up a modeless color chooser. The panel color is changed when the user
* clicks the OK button.
*/
private class ModelessListener implements ActionListener
{
private JDialog dialog;
private JColorChooser chooser;
public ModelessListener()
{
chooser = new JColorChooser();
dialog = JColorChooser.createDialog(ColorChooserPanel.this, "Background Color",
false /* not modal */, chooser,
event -> setBackground(chooser.getColor()),
null /* no Cancel button listener */);
}
public void actionPerformed(ActionEvent event)
{
chooser.setColor(getBackground());
dialog.setVisible(true);
}
}
/**
* This listener pops up a modeless color chooser. The panel color is changed immediately when
* the user picks a new color.
*/
private class ImmediateListener implements ActionListener
{
private JDialog dialog;
private JColorChooser chooser;
public ImmediateListener()
{
chooser = new JColorChooser();
chooser.getSelectionModel().addChangeListener(
event -> setBackground(chooser.getColor()));
dialog = new JDialog((Frame) null, false /* not modal */);
dialog.add(chooser);
dialog.pack();
}
public void actionPerformed(ActionEvent event)
{
chooser.setColor(getBackground());
dialog.setVisible(true);
}
}
}
package colorChooser;
import javax.swing.*;
/**
* A frame with a color chooser panel
*/
public class ColorChooserFrame extends JFrame
{//设置两个私有属性
private static final int DEFAULT_WIDTH = 300;
private static final int DEFAULT_HEIGHT = 200;
public ColorChooserFrame()//颜色选择器
{
setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT);
// add color chooser panel to frame
ColorChooserPanel panel = new ColorChooserPanel();
add(panel);
}
}
package colorChooser;
import java.awt.*;
import javax.swing.*;
/**
* @version 1.04 2015-06-12
* @author Cay Horstmann
*/
public class ColorChooserTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(() -> {
JFrame frame = new ColorChooserFrame();//生成ColorChooserFrame类
frame.setTitle("ColorChooserTest");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);//关闭界面操作
frame.setVisible(true);
});
}
}
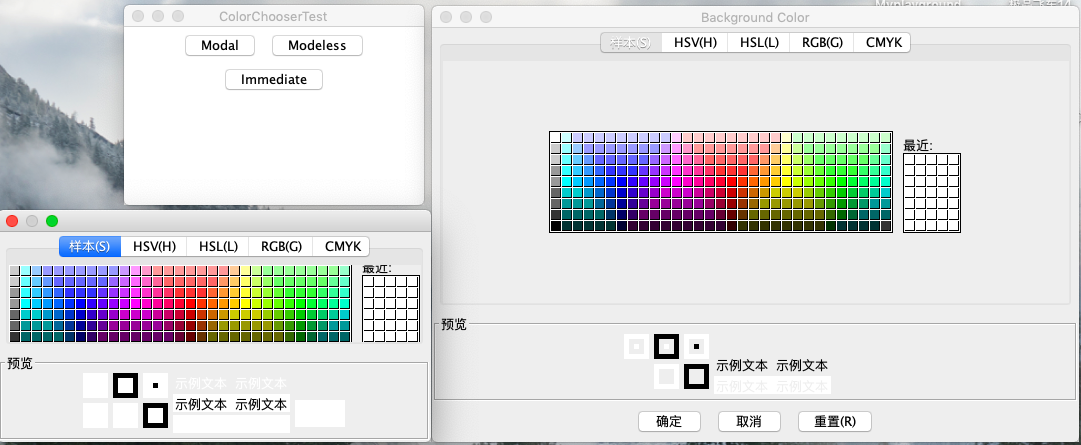
实验2:组内讨论反思本组负责程序,理解程序总体结构,梳理程序GUI设计中应用的相关组件,整理相关组件的API,对程序中组件应用的相关代码添加注释。
所添加注释:
package 课本示例13;
import java.awt.*;
import javax.swing.*;
/**
* @version 1.34 2015-06-12
* @author Cay Horstmann
*/
public class OptionDialogTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(() -> {
JFrame frame = new OptionDialogFrame();
frame.setTitle("OptionDialogTest");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
});
}
}
package 课本示例13;
import java.awt.*;
import java.awt.event.*;
import java.awt.geom.*;
import java.util.*;
import javax.swing.*;
/**
* 包含用于选择各种选项对话框的设置的框架。
*/
public class OptionDialogFrame extends JFrame
{
private ButtonPanel typePanel;
private ButtonPanel messagePanel;
private ButtonPanel messageTypePanel;
private ButtonPanel optionTypePanel;
private ButtonPanel optionsPanel;
private ButtonPanel inputPanel;
private String messageString = "Message";
private Icon messageIcon = new ImageIcon("blue-ball.gif");
private Object messageObject = new Date();
private Component messageComponent = new SampleComponent();
public OptionDialogFrame()
{
JPanel gridPanel = new JPanel();
gridPanel.setLayout(new GridLayout(2, 3));
typePanel = new ButtonPanel("Type", "Message", "Confirm", "Option", "Input");
messageTypePanel = new ButtonPanel("Message Type", "ERROR_MESSAGE", "INFORMATION_MESSAGE",
"WARNING_MESSAGE", "QUESTION_MESSAGE", "PLAIN_MESSAGE");
messagePanel = new ButtonPanel("Message", "String", "Icon", "Component", "Other",
"Object[]");
optionTypePanel = new ButtonPanel("Confirm", "DEFAULT_OPTION", "YES_NO_OPTION",
"YES_NO_CANCEL_OPTION", "OK_CANCEL_OPTION");
optionsPanel = new ButtonPanel("Option", "String[]", "Icon[]", "Object[]");
inputPanel = new ButtonPanel("Input", "Text field", "Combo box");
gridPanel.add(typePanel);
gridPanel.add(messageTypePanel);
gridPanel.add(messagePanel);
gridPanel.add(optionTypePanel);
gridPanel.add(optionsPanel);
gridPanel.add(inputPanel);
// add a panel with a Show button
JPanel showPanel = new JPanel();
JButton showButton = new JButton("Show");
showButton.addActionListener(new ShowAction());
showPanel.add(showButton);
add(gridPanel, BorderLayout.CENTER);
add(showPanel, BorderLayout.SOUTH);
pack();
}
/**
* Gets the currently selected message.
* @return a string, icon, component, or object array, depending on the Message panel selection
*/
public Object getMessage()
{
String s = messagePanel.getSelection();
if (s.equals("String")) return messageString;
else if (s.equals("Icon")) return messageIcon;
else if (s.equals("Component")) return messageComponent;
else if (s.equals("Object[]")) return new Object[] { messageString, messageIcon,
messageComponent, messageObject };
else if (s.equals("Other")) return messageObject;
else return null;
}
/**
* Gets the currently selected options.
* @return an array of strings, icons, or objects, depending on the Option panel selection
*/
public Object[] getOptions()
{
String s = optionsPanel.getSelection();
if (s.equals("String[]")) return new String[] { "Yellow", "Blue", "Red" };
else if (s.equals("Icon[]")) return new Icon[] { new ImageIcon("yellow-ball.gif"),
new ImageIcon("blue-ball.gif"), new ImageIcon("red-ball.gif") };
else if (s.equals("Object[]")) return new Object[] { messageString, messageIcon,
messageComponent, messageObject };
else return null;
}
/**
* 获取所选消息或选项类型
* @param面板消息类型或确认面板
* @return JOptionPane类中选定的XXX_MESSAGE或XXX_OPTION常量
*/
public int getType(ButtonPanel panel)
{
String s = panel.getSelection();
try
{
return JOptionPane.class.getField(s).getInt(null);
}
catch (Exception e)
{
return -1;
}
}
/**
* “显示”按钮的动作侦听器显示“确认”,“输入”,“消息”或“选项”对话框
*取决于“类型”面板选择。
*/
private class ShowAction implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
if (typePanel.getSelection().equals("Confirm")) JOptionPane.showConfirmDialog(
OptionDialogFrame.this, getMessage(), "Title", getType(optionTypePanel),
getType(messageTypePanel));
else if (typePanel.getSelection().equals("Input"))
{
if (inputPanel.getSelection().equals("Text field")) JOptionPane.showInputDialog(
OptionDialogFrame.this, getMessage(), "Title", getType(messageTypePanel));
else JOptionPane.showInputDialog(OptionDialogFrame.this, getMessage(), "Title",
getType(messageTypePanel), null, new String[] { "Yellow", "Blue", "Red" },
"Blue");
}
else if (typePanel.getSelection().equals("Message")) JOptionPane.showMessageDialog(
OptionDialogFrame.this, getMessage(), "Title", getType(messageTypePanel));
else if (typePanel.getSelection().equals("Option")) JOptionPane.showOptionDialog(
OptionDialogFrame.this, getMessage(), "Title", getType(optionTypePanel),
getType(messageTypePanel), null, getOptions(), getOptions()[0]);
}
}
}
/**
* 具有涂漆表面的组件
*/
class SampleComponent extends JComponent
{
public void paintComponent(Graphics g)
{
Graphics2D g2 = (Graphics2D) g;
Rectangle2D rect = new Rectangle2D.Double(0, 0, getWidth() - 1, getHeight() - 1);
g2.setPaint(Color.YELLOW);
g2.fill(rect);
g2.setPaint(Color.BLUE);
g2.draw(rect);
}
public Dimension getPreferredSize()
{
return new Dimension(10, 10);
}
}
package 课本示例13;
import javax.swing.*;
/**
* 带标题边框内的单选按钮的面板.
*/
public class ButtonPanel extends JPanel
{
private ButtonGroup group;
/**
* 构造一个按钮面板.
* @param标题边框中显示的标题
* @param选项一组单选按钮标签
*/
public ButtonPanel(String title, String... options)
{
setBorder(BorderFactory.createTitledBorder(BorderFactory.createEtchedBorder(), title));
setLayout(new BoxLayout(this, BoxLayout.Y_AXIS));
group = new ButtonGroup();
// make one radio button for each option
for (String option : options)
{
JRadioButton b = new JRadioButton(option);
b.setActionCommand(option);
add(b);
group.add(b);
b.setSelected(option == options[0]);
}
}
/**
* 获取当前选定的选项。
* @return当前所选单选按钮的标签。
*/
public String getSelection()
{
return group.getSelection().getActionCommand();
}
}
GUI相关组件:
GUI窗口
Jframe是窗体组件。创建该组件即创建了一个窗口。窗口是一个容器组件,所有内容均可以放入其中。
创建窗体对象:
JFrame frame = newJFrame(“标题”);
// 在这里需要简单设置属性:
frame.setSize(int 宽,int 高); //设置窗体尺寸
frame.setLocation(int x轴,int y轴); //设置窗体位置
frame. setVisible(true); //设置窗体可见
this.setResizable(false); //设置窗体大小固定
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// 关闭窗口默认操作
向窗体中添加其他组件:
frame.add(其他组件);
GUI布局
布局是指界面存放组件位置的方式。
如果组件凌乱地存放在容器当中不好看,通常可以采用对应布局方式,来规范组件的位置,Java也提供了常见的布局对象。
LayoutManager: 布局接口,各种布局类均为其子类
frame.setLayout(布局对象) //设置窗口布局
null:不使用布局,此时可以容器内的组件可以调用方法,通过坐标以及尺寸进行组件定位:
组件定位方法为void setBounds(int x轴,int y轴,int 宽度,int 高度)
BorderLayout:边界布局,将界面分为东西南北中5个方位,frame的默认布局
GUI面板
JPanel面板与JFrame窗体类似,属于容器组件,可以存放其他组件内容。通常用来划分区域,完成一个完整的功能。
通常的方式是: JFrame存放JPanel,JPanel存放其他组件。
GUI按钮
在容器内最终完成数据展示与交互的均为功能组件。组件众多,其他组件的使用方法均类似,这里不一一介绍。欢迎界面中使用到了Button组件。
Button btn = new Button();
通用步骤:
1、创建功能组件对象;
2、给功能组件绑定监听器;
3、将功能组件添加到容器组件中;
GUI监听器
页面中的各种组件均包含一些Java预设的用户操作(如鼠标点击,键盘按键等),如何在用户完成对应操作时,执行对应的代码,是事件监听机制完成的。
将发生预设事件的组件绑定监听器,监听器某个组件是否发生了用户操作,当用户进行对应的操作后,执行对应代码。
我们最常见的操作是鼠标点击按钮,是按钮的动作。这里介绍按钮的事件监听。
事件监听步骤:
① 被监听的组件调用方法与监听器关联
按钮组件关联监听器方法:addActionListener(监听器对象)
按钮动作监听器接口:ActionListener
② 在监听器中定义用户操作对应的执行代码
实验3:组间协同学习:在本班课程QQ群内,各位同学对实验1中存在的问题进行提问,提问时注明实验1中的测试程序编号,负责对应程序的小组需及时对群内提问进行回答。
实验总结:
1. Swing简介
Swing 是 Java 为图形界面应用开发提供的一组工具包,是 Java 基础类的一部分。
Swing 包含了构建图形界面(GUI)的各种组件,如: 窗口、标签、按钮、文本框等。
Swing 提供了许多比 AWT 更好的屏幕显示元素,使用纯 Java 实现,能够更好的兼容跨平台运行。
为了和AWT 组件区分,Swing 组件在javax.swing.*包下,类名均以 J 开头,例如: JFrame、JLabel、JButton等。
2. Swing组件
一个Java 的图形界面,由各种不同类型的“元素”组成,例如: 窗口、菜单栏、对话框、标签、按钮、文本框等等,这些“元素”统一被称为 组件(Component)。
组件按照不同的功能,可分为顶层容器、中间容器、基本组件。一个简单窗口的组成,如下层级结构所示:
顶层容器
菜单栏
中间容器
基本组件
基本组件
组件类型的继承关系:
顶层容器属于窗口类组件,继承自java.awt.Window;
中间容器和基本组件继承自javax.swing.JComponent。
(1)顶层容器
顶层容器属于窗口类组件,可以独立显示,一个图形界面至少需要一个窗口,例如:
#组件描述
1JFrame一个普通的窗口(绝大多数Swing 图形界面程序使用 JFrame 作为顶层容器)
2JDialog对话框
(2)中间容器
中间容器充当基本组件的载体,不可独立显示。中间容器可以添加若干基本组件(也可以嵌套添加中间容器),对容器内的组件进行管理,类似于给各种复杂的组件进行分组管理。最顶层的一个中间容器必须依托在顶层容器(窗口)内。
常用的中间容器(面板):
#组件描述
1JPanel一般轻量级面板容器组件
2JScrollPane带滚动条的,可以水平和垂直滚动的面板组件
3JSplitPane分隔面板
4JTabbedPane选项卡面板
5JLayeredPane层级面板
特殊的中间容器:
#组件描述
1JMenuBar菜单栏
2JToolBar工具栏
3JPopupMenu弹出菜单
4JInternalFrame内部窗口
(3)基本组件
基本组件是直接实现人机交互的组件。
常用的简单的基本组件:
#组件描述
1JLabel标签
2JButton按钮
3JRadioButton单选按钮
4JCheckBox复选框
5JToggleButton开关按钮
6JTextField文本框
7JPasswordField密码框
8JTextArea文本区域
9JComboBox下拉列表框
10JList列表
11JProgressBar进度条
12JSlider滑块
选取器组件:
#组件描述
1JFileChooser文件选取器
2JColorChooser颜色选取器
其他较为复杂的基本组件:
#组件描述
1JTable表格
2JTree树
3. 布局管理器
把Swing 的各种组件(JComponent)添加到面板容器中(JPanel),需要给面板容器指定布局管理器(LayoutManager),明确容器(Container)内的各个组件之间的排列布局方式。
常用的布局管理器:
#布局管理器描述
1FlowLayout流式布局,按组件加入的顺序,按水平方向排列,排满一行换下一行继续排列。
2GridLayout网格布局,把Container按指定行列数分隔出若干网格,每一个网格按顺序放置一个控件。
3GridBagLayout网格袋布局,按网格划分Container,每个组件可占用一个或多个网格,可将组件垂直、水平或沿它们的基线对齐。
4BoxLayout箱式布局,将Container中的多个组件按 水平 或 垂直 的方式排列。
5GroupLayout分组布局,将组件按层次分组(串行或并行),分别确定组件组在水平和垂直方向上的位置。
6CardLayout卡片布局,将Container中的每个组件看作一张卡片,一次只能显示一张卡片,默认显示第一张卡片。
7BorderLayout边界布局,把Container按方位分为 5 个区域(东、西、南、北、中),每个区域放置一个组件。
8SpringLayout弹性布局,通过定义组件四条边的坐标位置来实现布局。
9null绝对布局,通过设置组件在Container中的坐标位置来放置组件。
4. 代码实例: 一个简单的窗口程序
package com.xiets.swing;
import javax.swing.*;
public class Main {
public static void main(String[] args) {
// 1. 创建一个顶层容器(窗口)
JFrame jf = new JFrame("测试窗口"); // 创建窗口
jf.setSize(250, 250); // 设置窗口大小
jf.setLocationRelativeTo(null); // 把窗口位置设置到屏幕中心
jf.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); // 当点击窗口的关闭按钮时退出程序(没有这一句,程序不会退出)
// 2. 创建中间容器(面板容器)
JPanel panel = new JPanel(); // 创建面板容器,使用默认的布局管理器
// 3. 创建一个基本组件(按钮),并添加到 面板容器 中
JButton btn = new JButton("测试按钮");
panel.add(btn);
// 4. 把 面板容器 作为窗口的内容面板 设置到 窗口
jf.setContentPane(panel);
// 5. 显示窗口,前面创建的信息都在内存中,通过 jf.setVisible(true) 把内存中的窗口显示在屏幕上。
jf.setVisible(true);
}
}