TensorFlow基础见前博客
上实例:
MNIST 数据集介绍
MNIST 是一个手写阿拉伯数字的数据集。
其中包含有
60000
个已经标注了的训练集,还有 10000
个用于测试的测试集。本次实验的任务就是通过手写数字的图片,识别出具体写的是 0-9 之中的哪个数字。
理论知识回顾
View Code
View Code
一个两层的深层神经网络结构如下:
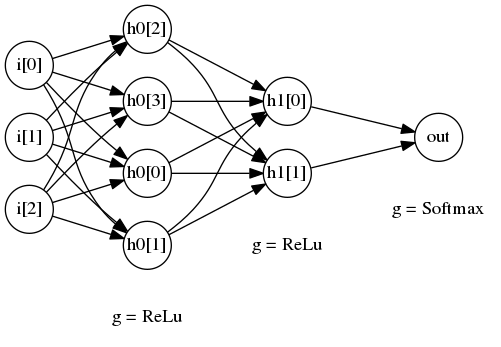
- 上图所示的是一个具有两层隐藏层的深层神经网络
- 第一个隐藏层有
4
个节点,对应的激活函数为ReLu
函数 - 第一个隐藏层有
2
个节点,对应的激活函数也是Relu
函数 - 最后,输出层使用 softmax 函数作为激活函数
激活函数定义(activation function):
模型设计
与上一个教程类似:
MNIST
数据一共有784
个输入,所以我们需要一个有784
个节点的输入层。MNIST
数据使用One-Hot
格式输出,有 0-910
个 label,分别对应是否为数字 0-9,所以我们在输出层有10
个节点,由于 0-9 的概率是互斥的,我们使用 Softmax 函数作为该层的激活函数。
不一样的是我们可以通过调整深度神经网络的层次来看看能不能达到不一样的效果。
训练模型
-
数据准备首先我们需要先下载
MNIST
的数据集。使用以下的命令进行下载:
wget https://devlab-1251520893.cos.ap-guangzhou.myqcloud.com/t10k-images-idx3-ubyte.gz
wget https://devlab-1251520893.cos.ap-guangzhou.myqcloud.com/t10k-labels-idx1-ubyte.gz
wget https://devlab-1251520893.cos.ap-guangzhou.myqcloud.com/train-images-idx3-ubyte.gz
wget https://devlab-1251520893.cos.ap-guangzhou.myqcloud.com/train-labels-idx1-ubyte.gz
实例代码:
#-*- encoding:utf-8 -*- #!/usr/local/env python import numpy as np import tensorflow as tf from tensorflow.examples.tutorials.mnist import input_data def add_layer(inputs, in_size, out_size, activation_function=None): W = tf.Variable(tf.random_normal([in_size, out_size])) b = tf.Variable(tf.zeros([1, out_size]) + 0.01) Z = tf.matmul(inputs, W) + b if activation_function is None: outputs = Z else: outputs = activation_function(Z) return outputs if __name__ == "__main__": MNIST = input_data.read_data_sets("mnist", one_hot=True) learning_rate = 0.01 batch_size = 128 n_epochs = 70 X = tf.placeholder(tf.float32, [batch_size, 784]) Y = tf.placeholder(tf.float32, [batch_size, 10]) layer_dims = [784, 500, 500, 10] layer_count = len(layer_dims)-1 # 不算输入层 layer_iter = X for l in range(1, layer_count): # layer [1,layer_count-1] is hidden layer layer_iter = add_layer(layer_iter, layer_dims[l-1], layer_dims[l], activation_function=tf.nn.relu) prediction = add_layer(layer_iter, layer_dims[layer_count-1], layer_dims[layer_count], activation_function=None) entropy = tf.nn.softmax_cross_entropy_with_logits(labels=Y, logits=prediction) loss = tf.reduce_mean(entropy) optimizer = tf.train.GradientDescentOptimizer(learning_rate).minimize(loss) init = tf.initialize_all_variables() with tf.Session() as sess: sess.run(init) n_batches = int(MNIST.test.num_examples/batch_size) for i in range(n_epochs): for j in range(n_batches): X_batch, Y_batch = MNIST.train.next_batch(batch_size) _, loss_ = sess.run([optimizer, loss], feed_dict={X: X_batch, Y: Y_batch}) if i % 10 == 5 and j == 0: print "Loss of epochs[{0}]: {1}".format(i, loss_) # test the model n_batches = int(MNIST.test.num_examples/batch_size) total_correct_preds = 0 for i in range(n_batches): X_batch, Y_batch = MNIST.test.next_batch(batch_size) preds = sess.run(prediction, feed_dict={X: X_batch, Y: Y_batch}) correct_preds = tf.equal(tf.argmax(preds, 1), tf.argmax(Y_batch, 1)) accuracy = tf.reduce_sum(tf.cast(correct_preds, tf.float32)) total_correct_preds += sess.run(accuracy) print "Accuracy {0}".format(total_correct_preds/MNIST.test.num_examples)
代码讲解
add_layer 函数
允许用户指定上一层的输出节点的个数作为
input_size
,本层的节点个数作为 output_size
,并指定激活函数 activation_function
可以看到我们调用的时候位神经网络添加了两个隐藏层
和输出层
for l in range(1, layer_count): # layer [1,layer_count-1] is hidden layer layer_iter = add_layer(layer_iter, layer_dims[l-1], layer_dims[l], activation_function=tf.nn.relu) prediction = add_layer(layer_iter, layer_dims[layer_count-1], layer_dims[layer_count], activation_function=None) entropy = tf.nn.softmax_cross_entropy_with_logits(labels=Y, logits=prediction) loss = tf.reduce_mean(entropy)
神经网络配置
注意这一行,我们配置了一个深度的神经网络,它包含
两个
隐藏层,一个输入层和一个输出层 隐藏层的节点数为 500
layer_dims = [784, 500, 500, 10]
执行结果:

Loss of epochs[5]: 19.5915279388 Loss of epochs[15]: 7.20698690414 ... Loss of epochs[65]: 0.24952724576 Accuracy 0.939
可以看到经过 70
轮的训练,准确度大约在 94%
左右
探索(play around)
你可以通过修改下面的这个代码片段来修改整个神经网络。
layer_dims = [784, 500, 500, 10]
比如你可以去掉一层隐藏层
,并将隐藏层的节点数改为 600
layer_dims = [784, 600, 10]
训练完的结果大概是这样:

Loss of epochs[5]: 13.1280488968 Loss of epochs[15]: 9.14239501953 Loss of epochs[25]: 3.60039186478 ... Loss of epochs[65]: 3.32054066658 Accuracy 0.9161
准确度大概 92%
的样子。have fun!