flexmage现在是rails中上传图片与处理图片的首选,就算是paperclip也比不上它。它对Rmagick做了一层很人性化的封装,让我们处理图片更加便捷。比起paperclip,它原生就支持远程URL上传图片和删除硬盘上的附件。使用fleximage需要先安装RMagick。
ruby script/generate scaffold Picture user :belongs_to is_avatar :boolean |
这个和原来的Photo模块没有什么两样,这样我们就可以比较一下,flexmage能把上传简化到什么地步了!
安装flexmage
修改视图
新建_form.html.erb
<% form_for @picture, :html => { :multipart => true } do |f| %>
<%= f.error_messages %>
<% if logged_in? %>
<%= f.hidden_field :user_id,:value => current_user.id %>
<% end %>
<% if action_name == "new" %>
<p>
<%= f.label :is_avatar,"是否作为头像" %>
<%= f.check_box :is_avatar %>
</p>
<% end %>
<p>
<%= f.file_field :image_file %><br />
或者通过URL<%= f.text_field :image_file_url %>
</p>
<p>
<%= f.hidden_field :image_file_temp %>
<b>Uploaded Image:</b><br />
<%= embedded_image_tag(@picture.operate { |img| img.resize 100 }) if @picture.has_image? %>
</p>
<p>
<button type="submit"><%= button_name %></button>
</p>
<% end %>
修改new.html.erb
<% title "上传图片" %>
<%= render :partial => 'form',:locals => {:button_name => "上传"} %>
<%= link_to 'Back', pictures_path,:class => "button" %>
<% title "编辑图片" %>
<%= render :partial => 'form',:locals => {:button_name => "更新"} %>
<%= link_to '大图', @picture,:class => "button" %>
<%= link_to '返回', pictures_path,:class => "button" %>
<div class="figure">
<%= embedded_image_tag(@picture) if @picture.has_image? %>
<div class="legend">所有人:<%=h @picture.user.login rescue nil%>;是否为头像:<%= @picture.is_avatar %></div>
</div>
<%= debug @picture %>
<%= link_to '编辑', [:edit,@picture],:class => "button" %>
<%= link_to '返回', pictures_path,:class => "button" %>
<h1>图片列表</h1>
<table>
<tr>
<th>所有人</th>
<th>是否作为头像</th>
<th>预览</th>
</tr>
<% @pictures.each do |picture| %>
<tr>
<td><%=h picture.user.login rescue nil %></td>
<td><%= picture.is_avatar ? "是" : "否" %></td>
<td><%= embedded_image_tag(picture.operate { |img| img.resize 100 }) if picture.has_image? %></td>
<td><%= link_to 'Show', picture %></td>
<td><%= link_to 'Edit', edit_picture_path(picture) %></td>
<td><%= link_to 'Destroy', picture, :confirm => 'Are you sure?', :method => :delete %></td>
</tr>
<% end %>
</table>
<br />
<%= link_to '上传图片', new_picture_path %>
class Picture < ActiveRecord::Base
belongs_to :user
validates_presence_of :user_id
#image_directory是必填参数,没有默认值
acts_as_fleximage :image_directory => 'public/images/uploaded_photos'
end
当我们在模型声明了acts_as_fleximage后,插件就为我们的Picture实例添加了image_file ,image_file_url与image_file_temp三个虚拟属性。image_file是用于本地上传,image_file_url是用于远程URL上传,image_file_temp是用来保存一个副本,而这个副本有什么用?我们在提交表单的时候,有时会由于网络等问题导致提交失败,让我们被逼重新填写所有字段,文件也当然要重新上传。image_file_temp就是为应对这情形而开发的,当我们上传文件,rails会把文件上传一个目录,但这时它不会立即把它们传送到目标目录中,就像电驴一样,会放到一个临时目录中,待到完成后才把它复制到目标目录。因此当我们提交失败后,rails就用不着重新上传,而是从临时目录中拿就是,速度就快多!这是个很贴心的功能。
再看看embedded_image_tag方法,它比image_tag强大了,它能显示尚未保存的模型的图片,而且能对图片进行实时编辑。但要注意了,它会在页面中生成大量base64编码,不但几何级地增大页面的体积,而且这编码是交由javascript解释器来渲染生成图片,这效率当然是慢一个字,特别是在IE中。因此图片的渲染还是交给ruby解析器吧,这样我们就得动一动控制器了。修改pictures_controller的show action:
def show
@picture = Picture.find(params[:id])
respond_to do |format|
format.html # show.html.erb
format.png { render :inline => "@picture.operate{}", :type => :flexi}
format.gif { render :inline => "@picture.operate{}", :type => :flexi}
format.jpg { render :inline => "@picture.operate{}", :type => :flexi}
format.xml { render :xml => @picture }
end
end
修改对应视图
<div class="figure">
<%= image_tag picture_path(@picture, :format => :jpg) %>
<div class="legend">所有人:<%=h @picture.user.login rescue nil%>;是否为头像:<%= @picture.is_avatar %></div>
</div>
<%= debug @picture %>
<%= link_to '编辑', [:edit,@picture],:class => "button" %>
<%= link_to '返回', pictures_path,:class => "button" %>
添加页面缓存
class PicturesController < ApplicationController
caches_page :show
# ...
def update
# ... standard update code
expire_picture(@picture)
end
def destroy
# ... standard destroy code
expire_picture(@picture)
end
private
def expire_picture(picture)
expire_page formatted_picture_path(picture, :jpg)
end
end
没什么好说,就是为了减少重复渲染页面,缩短响炒时间。由于直接给客户端发送静态页面,因此也免去读取数据库这一步了。
flexmage有个不好的地方,它并不是百分之一百复制原图片。它默认储存的图片格式为png,如果非png它会转换成png,并降低其画质,默认是其85%。我们得修改这些默认属性避免这问题。
acts_as_fleximage do
image_directory 'public/images/uploaded_photos'
image_storage_format :jpg
output_image_jpg_quality 100
end
我们也可以设置默认储存图片格式为gif,但是也改变了动态gif变成静态gif的命运……
不过有些东西我们还是能做到,如原图片的各字(连带其扩展名),长度与宽度。这些属性都是定死的,一定要那样命名(image_filename,image_width与image_height),flexmage才会在上传过来把这些信息抽取出来储存到数据库中。那么让我们为模型添加与这些属性同名的字段吧。
class AddColumnsToPictures < ActiveRecord::Migration
def self.up
add_column :pictures, :image_filename, :string
add_column :pictures, :image_width, :integer
add_column :pictures, :image_height, :integer
end
def self.down
remove_column :pictures, :image_height
remove_column :pictures, :image_width
remove_column :pictures, :image_filename
end
end
那样我们就可以在视图中显示它们了,如:
原名为:<%= @picture.image_filename %>
宽为:<%= @picture.image_width %>px
长为:<%= @picture.image_height %>px
为了防止图片失效,如目录更改了,我们可以像paperclip那样设置一个默认图片,如:
acts_as_fleximage do
image_directory 'public/images/uploaded_photos'
image_storage_format :jpg
output_image_jpg_quality 100
default_image_path 'public/images/rails.png'
end
添加其他参数
acts_as_fleximage do
image_directory 'public/images/uploaded_photos'
image_storage_format :jpg
output_image_jpg_quality 100
default_image_path 'public/images/rails.png'
use_creation_date_based_directories true
require_image true
missing_image_message 'is required'
invalid_image_message 'was not a readable image'
end
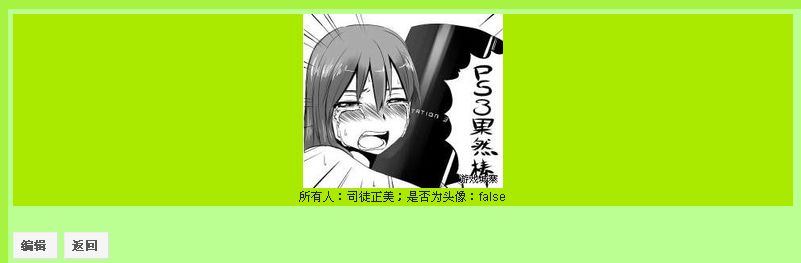
缩略图
fleximage的图片都是即时生成,不像paperclip导样在上传后我们设置了几种样式就生成几套图塞在硬盘中,这样对各自来说都有道理——fleximage说是节省空间,paperclip说是节省时间。在用户体验来说,fleximage是吃亏点,因此它才搞了个页面缓存。另一方面,原图肯定会经过处理,而且一定要经过特殊的渠道渲染出来,以前是要求show视图所在的目录建立一个flexi文件,如show.jpg.flexi,show.gif.flexi,不过我都是用"inline"方式实现而已,就像RJS那样,也有inline RJS的替代方案。为此,我们可以模拟了paperclop的@picture.image.url(:thumb)效果。
新建两个action与修改缓存。
class PicturesController < ApplicationController
caches_page :show,:thumb,:avatar
before_filter :find_picture, :only => [:show,:edit,:update,:destroy,:thumb,:avatar]
#==================其他actions==================
def thumb
render :inline => "@picture.operate {|p| p.resize '100x100'}", :type => :flexi
end
def avatar
render :inline => "@picture.operate {|p| p.resize '200x200'}", :type => :flexi
end
protected
def find_picture
@picture= Picture.find(params[:id])
end
def expire_picture(picture)
expire_page picture_path(picture, :format => :jpg)
expire_page thumb_picture_path(picture, :format => :jpg)
expire_page avatar_picture_path(picture, :format => :jpg)
end
end
map.resources :photos, :member => { :thumb => :get,:avatar => :get}
那么我们就可以在页面中使用缩略图了。
<%= image_tag thumb_picture_path(@picture, :format => :jpg) %>
<&#相当于 image_tag @picture.image.url(:thumb) %>
<%= image_tag avatar_picture_path(@picture, :format => :jpg) %>
<&#相当于 image_tag @picture.image.url(:avatar) %>