什么是CSS预处理器?
CSS预处理器定义了一种新的语言,其基本思想是,用一种专门的编程语言,为CSS增加了一些编程的特性,将CSS作为目标生成文件,然后开发者就只要使用这种语言进行编码工作。
什么是Sass?
Sass是一门高于CSS的元语言,它能用来清洗地、结构化地描述文件样式,有着比普通CSS更加强大的功能。Sass能够提供更简洁、更优雅的语法,同时提供多种功能来创建可维护和管理的样式表。
Sass的安装步骤:
第一步进入Ruby官网安装ruby(http://rubyinstaller.org/downloads),安装在C盘并且选中第二项;
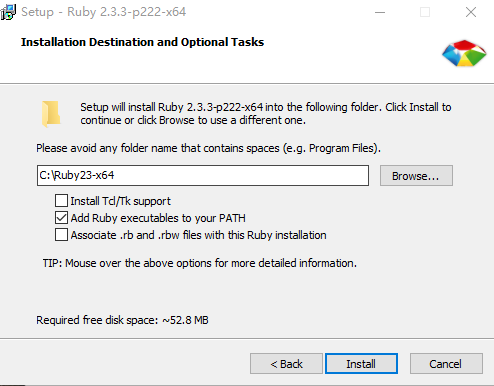
第二步在控制面板中找到Ruby,然后打开start command prompt with Ruby,输入gem install sass
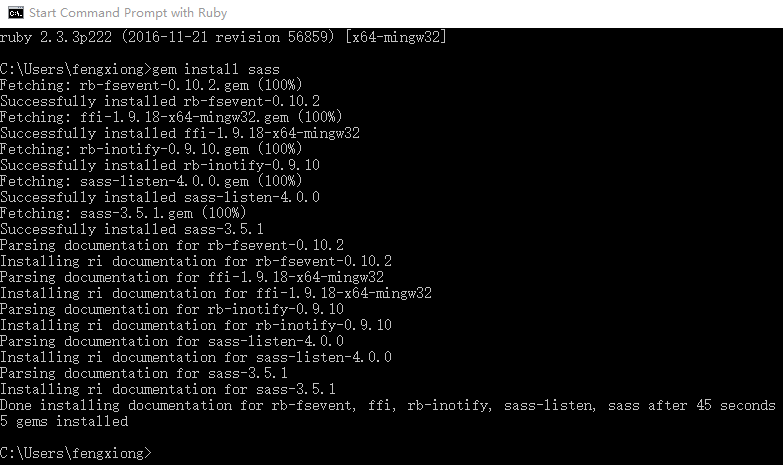
跟Sass有关的命令:
检查版本号(sass -v),也可以用来确认sass是否安装成功;
更新sass(gem update sass)
卸载sass(gem uninstall sass)
Sass语法格式:
老式的语法通过tab键控制缩进,不带任何的分号和大括号
$font-stack:"微软雅黑",sans-ser $primary-color:red body font-family:$font-stack color:$primary-color
新式语法又称scss,书写格式几乎和css一模一样
$font-stack:"微软雅黑",sans-ser; $primary-color:red; body{ font-family:$font-stack; color:$primary-color; }
注意:编译新式语法的时候文件后缀名得为".scss",而不是".sass"。不然编译会不通过
Sass的编译:
命令行单文件编译(一次编译,不能动态监测sass的变动)
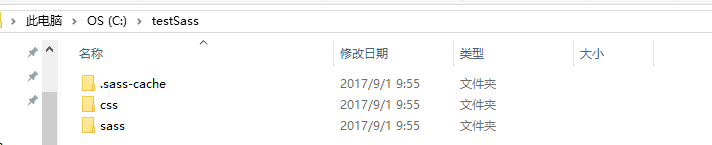
命令行单文件编译(动态监测)
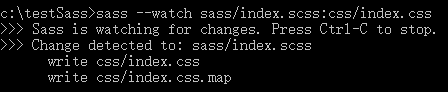
Sass界面编辑工具:Koala
(导入sass文件,在文件那里单击右键选择set output path可以编译成css文件)
Sass不同样式的输出方法
嵌套输出方式:sass --watch test.scss:test.css --style nested
展开输出方式:sass --watch test.scss:test.css --style expanded
紧凑输出方式:sass --watch test.scss:test.css --style compact
压缩输出方式:sass --watch test.scss:test.css --style compressed
Sass定义变量的语法
变量声明符 变量名称:变量值;
$300px;
Sass的全局变量和局部变量
$color: orange !default;//定义全局变量 .block { color: $color;//调用全局变量 } em { $color: red;//定义局部变量(全局变量 $color 的影子) a { color: $color;//调用局部变量 } }
Sass选择嵌套
nav { a { color: red; header & { color:green; } } }
编译后:
nav a {
color:red;
}
header nav a {
color:green;
}
Sass属性嵌套
.box {
border: {
top: 1px solid red;
bottom: 1px solid green;
}
}
编译后:
.box { border-top: 1px solid red; border-bottom: 1px solid green; }
Sass伪类嵌套
.clearfix{ &:before, &:after { content:""; display: table; } &:after { clear:both; overflow: hidden; } }
编译后:
clearfix:before, .clearfix:after { content: ""; display: table; } .clearfix:after { clear: both; overflow: hidden; }
Sass混合宏(声明)
//不带参数的混合宏 @mixin border-radius{ -webkit-border-radius: 5px; border-radius: 5px; }
//带参数的混合宏 @mixin border-radius($radius:5px){ -webkit-border-radius: $radius; border-radius: $radius; }
//复杂的混合宏 @mixin box-shadow($shadow...) { @if length($shadow) >= 1 { @include prefixer(box-shadow, $shadow); } @else{ $shadow:0 0 4px rgba(0,0,0,.3); @include prefixer(box-shadow, $shadow); } }
这个 box-shadow 的混合宏,带有多个参数,这个时候可以使用“ … ”来替代。简单的解释一下,当 $shadow 的参数数量值大于或等于“ 1 ”时,表示有多个阴影值,反之调用默认的参数值“ 0 0 4px rgba(0,0,0,.3) ”。
Sass混合宏(调用)
//声明混合宏 @mixin border-radius{ -webkit-border-radius: 3px; border-radius: 3px; } //调用混合宏 button { @include border-radius; } //编译后 button { -webkit-border-radius: 3px; border-radius: 3px; }
Sass混合宏(传参)
//传参不带默认值 @mixin border-radius($radius){ -webkit-border-radius: $radius; border-radius: $radius; } //调用 .box { @include border-radius(3px); }
//传参带默认值 @mixin border-radius($radius:3px){ -webkit-border-radius: $radius; border-radius: $radius; } //调用 .btn { @include border-radius; }
//传多个参数 @mixin center($width,$height){ $width; height: $height; position: absolute; top: 50%; left: 50%; margin-top: -($height) / 2; margin-left: -($width) / 2; } //调用 .box-center { @include center(500px,300px); }
//传非常多参数 @mixin box-shadow($shadows...){ @if length($shadows) >= 1 { -webkit-box-shadow: $shadows; box-shadow: $shadows; } @else { $shadows: 0 0 2px rgba(#000,.25); -webkit-box-shadow: $shadow; box-shadow: $shadow; } } //调用 .box { @include box-shadow(0 0 1px rgba(#000,.5),0 0 2px rgba(#000,.2)); }
Sass混合宏的不足
在调用相同的混合宏时,并不能智能的将相同的样式代码块合并在一起。
Sass的继承
.btn { border: 1px solid #ccc; padding: 6px 10px; font-size: 14px; } .btn-primary { color: #fff; @extend .btn; } .btn-second { color: #fff; @extend .btn; } //编译后 .btn, .btn-primary, .btn-second { border: 1px solid #ccc; padding: 6px 10px; font-size: 14px; } .btn-primary { color: #fff; } .btn-second { background-clor: orange; color: #fff; }
Sass占位符
Sass 中的占位符 %placeholder 功能是一个很强大,很实用的一个功能。他可以取代以前 CSS 中的基类造成的代码冗余的情形。因为 %placeholder 声明的代码,如果不被 @extend 调用的话,不会产生任何代码。来看一个演示:
//这段代码没有被 @extend 调用,他并没有产生任何代码块,只是静静的躺在你 //的某个 SCSS 文件中。只有通过 @extend 调用才会产生代码: %mt5 { margin-top: 5px; } %pt5{ padding-top: 5px; } //调用 %mt5 { margin-top: 5px; } %pt5{ padding-top: 5px; } .btn { @extend %mt5; @extend %pt5; } .block { @extend %mt5; span { @extend %pt5; } } //编译后 .btn, .block { margin-top: 5px; } .btn, .block span { padding-top: 5px; }
Sass混合宏、继承和占位符的使用场景
1、如果你的代码块中涉及到变量,建议使用混合宏来创建相同的代码块;
2、如果你的代码块不需要专任何变量参数,而且有一个基类已在文件中存在,那么建议使用 Sass 的继承;
3、占位符和继承的主要区别的,“占位符是独立定义,不调用的时候是不会在 CSS 中产生任何代码;继承是首先有一个基类存在,不管调用与不调用,基类的样式都将会出现在编译出来的 CSS 代码中”。
Sass插值
使用 CSS 预处理器语言的一个主要原因是想使用 Sass 获得一个更好的结构体系。比如说你想写更干净的、高效的和面向对象的 CSS。Sass 中的插值(Interpolation)就是重要的一部分。让我们看一下下面的例子:
$properties: (margin, padding); @mixin set-value($side, $value) { @each $prop in $properties { #{$prop}-#{$side}: $value; } } .login-box { @include set-value(top, 14px); } //编译后(它可以让变量和属性工作的很完美) .login-box { margin-top: 14px; padding-top: 14px; }
当你想设置属性值的时候你可以使用字符串插入进来。另一个有用的用法是构建一个选择器。可以这样使用:
@mixin generate-sizes($class, $small, $medium, $big) { .#{$class}-small { font-size: $small; } .#{$class}-medium { font-size: $medium; } .#{$class}-big { font-size: $big; } } @include generate-sizes("header-text", 12px, 20px, 40px); //编译后 .header-text-small { font-size: 12px; } .header-text-medium { font-size: 20px; } .header-text-big { font-size: 40px; }
在Sass混合宏中无法使用插值,在继承中倒是能够使用
%updated-status { margin-top: 20px; background: #F00; } .selected-status { font-weight: bold; } $flag: "status"; .navigation { @extend %updated-#{$flag}; @extend .selected-#{$flag}; }
Sass运算符
能够进行加减乘除
//加法,不同类型的单位在计算时会出错
.box { 20px + 8in; }
//减法,不同类型的单位在计算时会出错 $full- 960px; $sidebar- 200px; .content { $full-width - $sidebar-width; }
//乘法,不同类型的单位在计算时会出错 .box { 10px * 2; }
//除法,不同类型的单位在计算时会出错 .box { (100px / 2); }
Sass中的@if
@mixin blockOrHidden($boolean:true) { @if $boolean { @debug "$boolean is #{$boolean}"; display: block; } @else { @debug "$boolean is #{$boolean}"; display: none; } } .block { @include blockOrHidden; } .hidden{ @include blockOrHidden(false); } //编译后 .block { display: block; } .hidden { display: none; }
Sass中的@for
1、@for $i from <start>through<end>
//$i表示变量,start表示起始值,end表示结束值 @for $i from 1 through 3 { .item-#{$i} { 2em * $i; } } //编译后 .item-1 { 2em; } .item-2 { 4em; } .item-3 { 6em; }
2、@for $i from <start>to<end>
@for $i from 1 to 3 { .item-#{$i} { 2em * $i; } } 编译后 .item-1 { 2em; } .item-2 { 4em; }
3、@for进阶
$grid-prefix: span !default; $grid- 60px !default; $grid-gutter: 20px !default; %grid { float: left; margin-left: $grid-gutter / 2; margin-right: $grid-gutter / 2; } @for $i from 1 through 12 { .#{$grid-prefix}#{$i}{ $grid-width * $i + $grid-gutter * ($i - 1); @extend %grid; } }
//检测网址
Sass中@while
$types: 4; $type- 20px; @while $types > 0 { .while-#{$types} { $type-width + $types; } $types: $types - 1; } //编译后 .while-4 { 24px; } .while-3 { 23px; } .while-2 { 22px; } .while-1 { 21px; }
Sass中@each循环
$list: adam john wynn mason kuroir;//$list 就是一个列表 @mixin author-images { @each $author in $list { .photo-#{$author} { background: url("/images/avatars/#{$author}.png") no-repeat; } } } .author-bio { @include author-images; }
//检测网址
Sass中的字符串函数之unquote()函数
unquote() 函数主要是用来删除一个字符串中的引号,如果这个字符串没有带有引号,将返回原始的字符串。简单的使用终端来测试这个函数的运行结果:
.test1 { content: unquote('Hello Sass!') ; } .test2 { ontent: unquote("'Hello Sass!"); } .test3 { content: unquote("I'm Web Designer"); } .test4 { content: unquote("'Hello Sass!'"); } .test5 { content: unquote('"Hello Sass!"'); } .test6 { content: unquote(Hello Sass); }
//检测网址
注意:从测试的效果中可以看出,unquote( ) 函数只能删除字符串最前和最后的引号(双引号或单引号),而无法删除字符串中间的引号。如果字符没有带引号,返回的将是字符串本身。
Sass中的字符串函数之quote()函数
quote() 函数刚好与 unquote() 函数功能相反,主要用来给字符串添加引号。如果字符串,自身带有引号会统一换成双引号 ""。如:
.test1 { content: quote('Hello Sass!'); } .test2 { content: quote("Hello Sass!"); } .test3 { content: quote(ImWebDesigner); } .test4 { content: quote(' '); }
//检测网址
注意:使用 quote() 函数只能给字符串增加双引号,而且字符串中间有单引号或者空格时,需要用单引号或双引号括起,否则编译的时候将会报错。同时 quote() 碰到特殊符号,比如: !、?、> 等,除中折号 - 和 下划线_ 都需要使用双引号括起,否则编译器在进行编译的时候同样会报错:
Sass中字符串函数之To-upper-case()和To-lower-case()
1、To-upper-case() 函数将字符串小写字母转换成大写字母。如:
.test { text: to-upper-case(aaaaa); text: to-upper-case(aA-aAAA-aaa); } //编译后 .test { text: AAAAA; text: AA-AAAA-AAA; }
2、To-lower-case() 函数将字符串小写字母转换成大写字母。如:
.test { text: to-lower-case(AAAAA); text: to-lower-case(aA-aAAA-aaa); } //编译后 .test { text: aaaaa; text: aa-aaaa-aaa; }
Sass数字函数之percentage()
percentage()函数主要是将一个不带单位的数字转换成百分比形式,语法有:
1、percentage(.2);2、percentage(2px / 10px);3、percentage(2em / 10em)
.footer{ width : percentage(.2) } //编译后 .footer{ width : 20% }
Sass数字函数之round()
round() 函数可以将一个数四舍五入为一个最接近的整数,在round() 函数中可以携带单位的任何数值:
.footer { round(12.3px) } //编译后 .footer { 12px; }
Sass数字函数之ceil()
ceil() 函数将一个数转换成最接近于自己的整数,会将一个大于自身的任何小数转换成大于本身 1 的整数。也就是只做入,不做舍的计算:
.footer { ceil(12.3px); } //编译后 .footer { 13px; }
Sass数字函数之floor()
floor() 函数刚好与 ceil() 函数功能相反,其主要将一个数去除其小数部分,并且不做任何的进位。也就是只做舍,不做入的计算:
.footer { floor(12.3px); } //编译后 .footer { 12px; }
Sass数字函数之abs()
abs( ) 函数会返回一个数的绝对值。
.footer { abs(-12.3px); } //编译后 .footer { 12.3px; }
Sass数字函数之min()
min() 函数功能主要是在多个数之中找到最小的一个,这个函数可以设置任意多个参数:
Sass数字函数之random()
random() 函数是用来获取一个随机数:
Sass中length()函数
length() 函数主要用来返回一个列表中有几个值,简单点说就是返回列表清单中有多少个值:
Sass中nth()函数
nth() 函数用来指定列表中某个位置的值。不过在 Sass 中,nth() 函数和其他语言不同,1 是指列表中的第一个标签值,2 是指列给中的第二个标签值,依此类推。如:
//语法 nth($list,$n) >> nth(10px 20px 30px,1) 10px >> nth((Helvetica,Arial,sans-serif),2) "Arial" >> nth((1px solid red) border-top green,1) (1px "solid" #ff0000)
Sass中join()函数
join() 函数是将两个列表连接合并成一个列表。
>> join(10px 20px, 30px 40px) (10px 20px 30px 40px) >> join((blue,red),(#abc,#def)) (#0000ff, #ff0000, #aabbcc, #ddeeff) >> join((blue,red),(#abc #def)) (#0000ff, #ff0000, #aabbcc, #ddeeff)
不过 join() 只能将两个列表连接成一个列表,如果直接连接两个以上的列表将会报错:
>> join((blue red),(#abc, #def),(#dee #eff)) SyntaxError: $separator: (#ddeeee #eeffff) is not a string for `join'
但很多时候不只碰到两个列表连接成一个列表,这个时候就需要将多个 join() 函数合并在一起使用:
>> join((blue red), join((#abc #def),(#dee #eff)))
(#0000ff #ff0000 #aabbcc #ddeeff #ddeeee #eeffff)
Sass中append()函数
append() 函数是用来将某个值插入到列表中,并且处于最末位。
>> append(10px 20px ,30px) (10px 20px 30px) >> append((10px,20px),30px) (10px, 20px, 30px) >> append(green,red) (#008000 #ff0000) >> append(red,(green,blue)) (#ff0000 (#008000, #0000ff))
Sass中zip()函数
zip()函数将多个列表值转成一个多维的列表:
>> zip(1px 2px 3px,solid dashed dotted,green blue red)
((1px "solid" #008000), (2px "dashed" #0000ff), (3px "dotted" #ff0000))
Sass中index()函数
index() 函数类似于索引一样,主要让你找到某个值在列表中所处的位置。在 Sass 中,第一个值就是1,第二个值就是 2,依此类推:
>> index(1px solid red, 1px) 1 >> index(1px solid red, solid) 2 >> index(1px solid red, red) 3
Sass中type-of()函数
type-of() 函数主要用来判断一个值是属于什么类型:
>> type-of(100) "number" >> type-of(100px) "number" >> type-of("asdf") "string" >> type-of(asdf) "string" >> type-of(true) "bool" >> type-of(false) "bool" >> type-of(#fff) "color" >> type-of(blue) "color" >> type-of(1 / 2 = 1) "string"
Sass中unit()函数
unit() 函数主要是用来获取一个值所使用的单位,碰到复杂的计算时,其能根据运算得到一个“多单位组合”的值,不过只充许乘、除运算:
>> unit(100) "" >> unit(100px) "px" >> unit(20%) "%" >> unit(1em) "em" >> unit(10px * 3em) "em*px" >> unit(10px / 3em) "px/em" >> unit(10px * 2em / 3cm / 1rem) "em/rem"
Sass中unitless()函数
unitless() 函数相对来说简单明了些,只是用来判断一个值是否带有单位,如果不带单位返回的值为 true,带单位返回的值为 false:
>> unitless(100) true >> unitless(100px) false >> unitless(100em) false >> unitless(100%) false >> unitless(1 /2 ) true >> unitless(1 /2 + 2 ) true >> unitless(1px /2 + 2 ) false
Sass中comparable()函数
comparable() 函数主要是用来判断两个数是否可以进行“加,减”以及“合并”。如果可以返回的值为 true,如果不可以返回的值是 false:
comparable(2px,1px) true >> comparable(2px,1%) false >> comparable(2px,1em) false >> comparable(2rem,1em) false >> comparable(2px,1cm) true >> comparable(2px,1mm) true >>comparable(2px,1rem) false >> comparable(2cm,1mm) true
Sass中Miscellaneous函数
在这里把 Miscellaneous 函数称为三元条件函数,主要因为他和 JavaScript 中的三元判断非常的相似。他有两个值,当条件成立返回一种值,当条件不成立时返回另一种值:
>> if(true,1px,2px) 1px >> if(false,1px,2px) 2px
Sass中Map函数