工厂模式:
.h文件:
#import <Foundation/Foundation.h>
typedef enum{
QFRed,
QFYellow,
QFBlue
}QFViewColor;
@interface QFview : UIView
+(id)viewWithColor:(QFViewColor)QFViewColorType;
@end
typedef enum{
QFRed,
QFYellow,
QFBlue
}QFViewColor;
@interface QFview : UIView
+(id)viewWithColor:(QFViewColor)QFViewColorType;
@end
.m文件:
+(id)viewWithColor:(QFViewColor)QFViewColorType{
UIView *view = [[UIView alloc] init];
switch (QFViewColorType) {
case QFRed:
view.backgroundColor=[UIColor redColor];
break;
case QFYellow:
view.backgroundColor=[UIColor yellowColor];
break;
case QFBlue:
view.backgroundColor=[UIColor blueColor];
break;
default:
view.backgroundColor=[UIColor whiteColor];
break;
}
return view;
}
UIView *view = [[UIView alloc] init];
switch (QFViewColorType) {
case QFRed:
view.backgroundColor=[UIColor redColor];
break;
case QFYellow:
view.backgroundColor=[UIColor yellowColor];
break;
case QFBlue:
view.backgroundColor=[UIColor blueColor];
break;
default:
view.backgroundColor=[UIColor whiteColor];
break;
}
return view;
}
利用工厂模式显示view视图
UIView *rootView = [[UIView alloc] initWithFrame:CGRectMake(50, 50, 300, 300)];
rootView.backgroundColor = [UIColor whiteColor];
rootView.clipsToBounds = YES;
//切割视图外部的东西
UIView *redView = [QFview viewWithColor:QFRed];
redView.frame = CGRectMake(0, 0, 200, 200);
[rootView addSubview:redView];
redView.alpha = 1;
UIView *yellowView = [QFview viewWithColor:QFYellow];
yellowView.frame = CGRectMake(50, 50, 150, 150);
yellowView.alpha = 1;
[rootView addSubview:yellowView];
UIView *blueView = [QFview viewWithColor:QFBlue];
blueView.frame = CGRectMake(100, 100, 100, 100);
[rootView addSubview:blueView];
rootView.backgroundColor = [UIColor whiteColor];
rootView.clipsToBounds = YES;
//切割视图外部的东西
UIView *redView = [QFview viewWithColor:QFRed];
redView.frame = CGRectMake(0, 0, 200, 200);
[rootView addSubview:redView];
redView.alpha = 1;
UIView *yellowView = [QFview viewWithColor:QFYellow];
yellowView.frame = CGRectMake(50, 50, 150, 150);
yellowView.alpha = 1;
[rootView addSubview:yellowView];
UIView *blueView = [QFview viewWithColor:QFBlue];
blueView.frame = CGRectMake(100, 100, 100, 100);
[rootView addSubview:blueView];
图片显示:
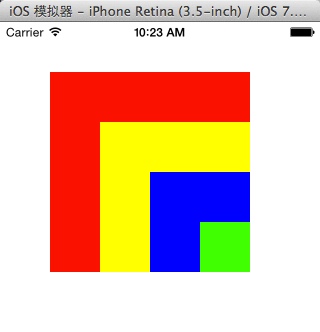
对视图进行层次化操作:
//[rootView bringSubviewToFront:redView];
//[rootView sendSubviewToBack:blueView];
NSArray *rootViewSubviews = rootView.subviews;
int yellowIndex = [rootViewSubviews indexOfObject:yellowView];
int redIndex = [rootViewSubviews indexOfObject:redView];
//[rootView exchangeSubviewAtIndex:yellowIndex withSubviewAtIndex:redIndex];
/* 将红色 黄色调换 */
UIView *greenView = [[UIView alloc] initWithFrame:CGRectMake(150, 150, 50, 50)];
greenView.backgroundColor = [UIColor greenColor];
[rootView insertSubview:greenView aboveSubview:blueView];
[self.window addSubview:rootView];
//[rootView sendSubviewToBack:blueView];
NSArray *rootViewSubviews = rootView.subviews;
int yellowIndex = [rootViewSubviews indexOfObject:yellowView];
int redIndex = [rootViewSubviews indexOfObject:redView];
//[rootView exchangeSubviewAtIndex:yellowIndex withSubviewAtIndex:redIndex];
/* 将红色 黄色调换 */
UIView *greenView = [[UIView alloc] initWithFrame:CGRectMake(150, 150, 50, 50)];
greenView.backgroundColor = [UIColor greenColor];
[rootView insertSubview:greenView aboveSubview:blueView];
[self.window addSubview:rootView];
设置视图内的按键不可用
UIButton *button = [UIButton buttonWithType:UIButtonTypeContactAdd];
button.frame = CGRectMake(50, 50, 50, 50);
button.enabled = NO; //设置按键不可用
[rootView addSubview:button];
rootView.userInteractionEnabled = NO;
//设置按键无法按下
button.frame = CGRectMake(50, 50, 50, 50);
button.enabled = NO; //设置按键不可用
[rootView addSubview:button];
rootView.userInteractionEnabled = NO;
//设置按键无法按下
制作动画:
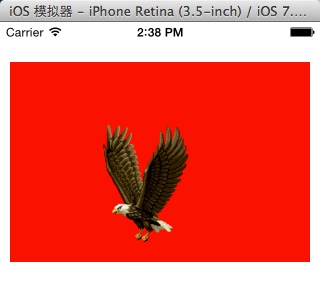
代码如下:
UIImageView *imageView = [[UIImageView alloc] initWithFrame:CGRectMake(10, 40, 300, 200)];
imageView.backgroundColor = [UIColor redColor];
//imageView.contentMode = UIViewContentModeScaleToFill;
imageView.contentMode = UIViewContentModeScaleAspectFill;
imageView.animationDuration = 1.5;
//速度多少秒
NSMutableArray *images = [NSMutableArray array];
for (int i=1; i<=13; ++i) {
[images addObject:[UIImage imageNamed:[NSString stringWithFormat:@"png%d", i]]];
}
imageView.animationImages = images;
//imageView.animationRepeatCount = 2;
//图片重复多少次
[imageView startAnimating];
[self.window addSubview:imageView];
imageView.backgroundColor = [UIColor redColor];
//imageView.contentMode = UIViewContentModeScaleToFill;
imageView.contentMode = UIViewContentModeScaleAspectFill;
imageView.animationDuration = 1.5;
//速度多少秒
NSMutableArray *images = [NSMutableArray array];
for (int i=1; i<=13; ++i) {
[images addObject:[UIImage imageNamed:[NSString stringWithFormat:@"png%d", i]]];
}
imageView.animationImages = images;
//imageView.animationRepeatCount = 2;
//图片重复多少次
[imageView startAnimating];
[self.window addSubview:imageView];
按钮可以换界面了、做四个界面每,按不同按钮切换不同界面:
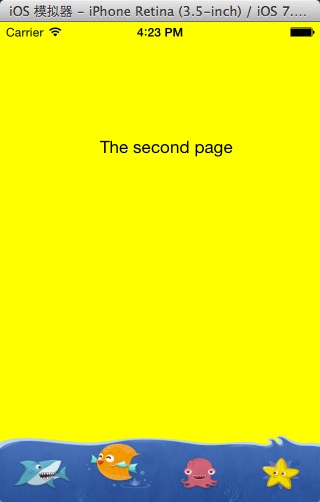
代码:
#import "Xib.h"
@interface Xib ()
@property (weak, nonatomic) IBOutlet UIButton *button1;
@property (weak, nonatomic) IBOutlet UIButton *button2;
@property (weak, nonatomic) IBOutlet UIButton *button3;
@property (weak, nonatomic) IBOutlet UIButton *button4;
@property (weak, nonatomic) IBOutlet UIView *menuView;
-(void)showRedView;
-(void)showYellowView;
-(void)showBlueView;
-(void)showGreenView;
@end
@implementation Xib
{
UIView *redView;
UIView *yellowView;
UIView *blueView;
UIView *greenView;
}
- (IBAction)selected_one:(id)sender {
_button1.selected = NO;
_button2.selected = NO;
_button3.selected = NO;
_button4.selected = NO;
UIButton *btn = sender;
NSLog(@"点击:%d",btn.tag);
btn.selected = YES;
switch (btn.tag) {
case 0:
[self showRedView];
break;
case 1:
[self showYellowView];
break;
case 2:
[self showBlueView];
break;
case 3:
[self showGreenView];
break;
}
}
-(void)showRedView{
if (redView == nil) {
redView = [[UIView alloc] initWithFrame:self.view.frame];
redView.backgroundColor = [UIColor redColor];
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(100, 100, 200, 50)];
label.text = @"The first page";
[redView addSubview:label];
[self.view addSubview:redView];
}
[self.view bringSubviewToFront:redView];
[self.view bringSubviewToFront:self.menuView];
}
-(void)showYellowView
{
if (yellowView == nil) {
yellowView = [[UIView alloc] initWithFrame:self.view.frame];
yellowView.backgroundColor = [UIColor yellowColor];
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(100, 100, 200, 50)];
label.text = @"The second page";
[yellowView addSubview:label];
[self.view addSubview:yellowView];
}
[self.view bringSubviewToFront:yellowView];
[self.view bringSubviewToFront:self.menuView];
}
-(void)showBlueView
{
if (blueView == nil) {
blueView = [[UIView alloc] initWithFrame:self.view.frame];
blueView.backgroundColor = [UIColor blueColor];
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(100, 100, 200, 50)];
label.text = @"The third page";
[blueView addSubview:label];
[self.view addSubview:blueView];
}
[self.view bringSubviewToFront:blueView];
[self.view bringSubviewToFront:self.menuView];
}
-(void)showGreenView
{
if (greenView == nil) {
greenView = [[UIView alloc] initWithFrame:self.view.frame];
greenView.backgroundColor = [UIColor greenColor];
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(100, 100, 200, 50)];
label.text = @"The forth page";
[greenView addSubview:label];
[self.view addSubview:greenView];
}
[self.view bringSubviewToFront:greenView];
[self.view bringSubviewToFront:self.menuView];
}
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
self.menuView.backgroundColor=[UIColor clearColor];
// Do any additional setup after loading the view from its nib.
}
@interface Xib ()
@property (weak, nonatomic) IBOutlet UIButton *button1;
@property (weak, nonatomic) IBOutlet UIButton *button2;
@property (weak, nonatomic) IBOutlet UIButton *button3;
@property (weak, nonatomic) IBOutlet UIButton *button4;
@property (weak, nonatomic) IBOutlet UIView *menuView;
-(void)showRedView;
-(void)showYellowView;
-(void)showBlueView;
-(void)showGreenView;
@end
@implementation Xib
{
UIView *redView;
UIView *yellowView;
UIView *blueView;
UIView *greenView;
}
- (IBAction)selected_one:(id)sender {
_button1.selected = NO;
_button2.selected = NO;
_button3.selected = NO;
_button4.selected = NO;
UIButton *btn = sender;
NSLog(@"点击:%d",btn.tag);
btn.selected = YES;
switch (btn.tag) {
case 0:
[self showRedView];
break;
case 1:
[self showYellowView];
break;
case 2:
[self showBlueView];
break;
case 3:
[self showGreenView];
break;
}
}
-(void)showRedView{
if (redView == nil) {
redView = [[UIView alloc] initWithFrame:self.view.frame];
redView.backgroundColor = [UIColor redColor];
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(100, 100, 200, 50)];
label.text = @"The first page";
[redView addSubview:label];
[self.view addSubview:redView];
}
[self.view bringSubviewToFront:redView];
[self.view bringSubviewToFront:self.menuView];
}
-(void)showYellowView
{
if (yellowView == nil) {
yellowView = [[UIView alloc] initWithFrame:self.view.frame];
yellowView.backgroundColor = [UIColor yellowColor];
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(100, 100, 200, 50)];
label.text = @"The second page";
[yellowView addSubview:label];
[self.view addSubview:yellowView];
}
[self.view bringSubviewToFront:yellowView];
[self.view bringSubviewToFront:self.menuView];
}
-(void)showBlueView
{
if (blueView == nil) {
blueView = [[UIView alloc] initWithFrame:self.view.frame];
blueView.backgroundColor = [UIColor blueColor];
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(100, 100, 200, 50)];
label.text = @"The third page";
[blueView addSubview:label];
[self.view addSubview:blueView];
}
[self.view bringSubviewToFront:blueView];
[self.view bringSubviewToFront:self.menuView];
}
-(void)showGreenView
{
if (greenView == nil) {
greenView = [[UIView alloc] initWithFrame:self.view.frame];
greenView.backgroundColor = [UIColor greenColor];
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(100, 100, 200, 50)];
label.text = @"The forth page";
[greenView addSubview:label];
[self.view addSubview:greenView];
}
[self.view bringSubviewToFront:greenView];
[self.view bringSubviewToFront:self.menuView];
}
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
self.menuView.backgroundColor=[UIColor clearColor];
// Do any additional setup after loading the view from its nib.
}