Given an array of integers, return indices of the two numbers such that they add up to a specific target.
You may assume that each input would have exactly one solution.
版本1:O(n^2) 【暴力 原始版本】O(1)
class Solution(object): def twoSum(self, nums, target): length = len(nums) for i in range(length): for j in range(i+1,length)://游标后移 if nums[i] + nums[j] == target: return [i,j]
版本2:O(n^2) 【暴力 枚举函数增强】O(1)
class Solution(object): def twoSum(self, nums, target): for index , item in enumerate(nums): for past_index , past_item in enumerate(nums[index+1:],index+1): if item + past_item == target: return [index, past_index]
版本3:O(n) 【双程hash table】O(n)
class Solution(object): def twoSum(self, nums, target): dd = dict() for index , value in enumerate(nums): dd[value] = index for index , value in enumerate(nums): tt = target - value if dd.has_key(tt) and dd[tt] != index: return [index , dd[tt]]
版本4:O(n) 【单程hash table】O(n)
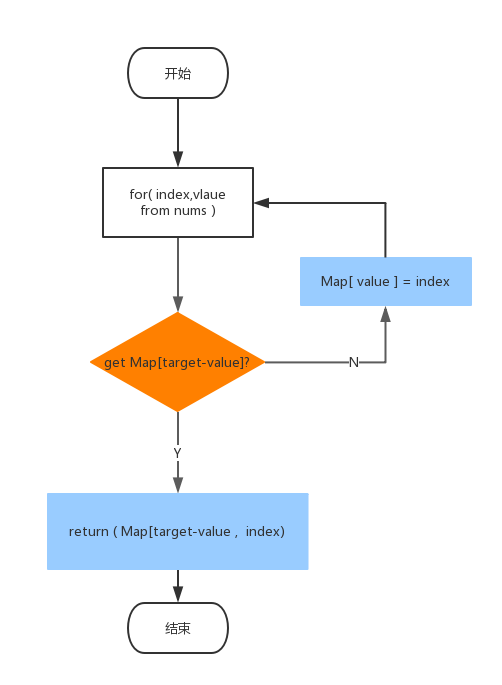
Python
class Solution(object): def twoSum(self, nums, target): dd = dict() for index , value in enumerate(nums): tt = target - value if dd.has_key(tt) and dd[tt] != index: return [dd[tt],index] else: dd[value] = index
Java
public int[] twoSum(int[] nums, int target) { Map<Integer,Integer> map = new HashMap<Integer,Integer>(); for ( int i=0;i<nums.length;i++ ){ if (!map.containsKey(target - nums[i]) ){ map.put(nums[i], i ); }else{ int[] rs = { map.get(target-nums[i]) , i }; return rs; } } return nums; }
1.enumerate内置函数的使用(枚举函数)---用于既要遍历索引又要遍历元素;可以接收第二个参数用于指定索引起始值。