链接:
Antenna Placement
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 5500 | Accepted: 2750 |
Description
The Global Aerial Research Centre has been allotted the task of building the fifth generation of mobile phone nets in Sweden. The most striking reason why they got the job, is their discovery of a new, highly noise resistant, antenna. It is called 4DAir, and
comes in four types. Each type can only transmit and receive signals in a direction aligned with a (slightly skewed) latitudinal and longitudinal grid, because of the interacting electromagnetic field of the earth. The four types correspond to antennas operating
in the directions north, west, south, and east, respectively. Below is an example picture of places of interest, depicted by twelve small rings, and nine 4DAir antennas depicted by ellipses covering them.
Obviously, it is desirable to use as few antennas as possible, but still provide coverage for each place of interest. We model the problem as follows: Let A be a rectangular matrix describing the surface of Sweden, where an entry of A either is a point of interest, which must be covered by at least one antenna, or empty space. Antennas can only be positioned at an entry in A. When an antenna is placed at row r and column c, this entry is considered covered, but also one of the neighbouring entries (c+1,r),(c,r+1),(c-1,r), or (c,r-1), is covered depending on the type chosen for this particular antenna. What is the least number of antennas for which there exists a placement in A such that all points of interest are covered?
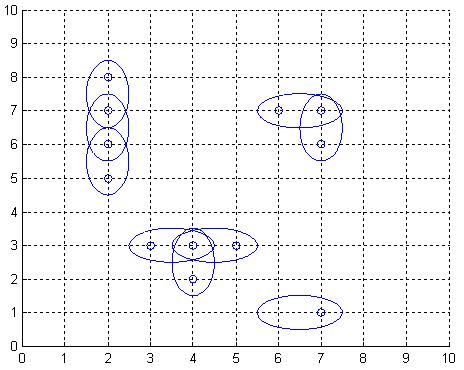
Obviously, it is desirable to use as few antennas as possible, but still provide coverage for each place of interest. We model the problem as follows: Let A be a rectangular matrix describing the surface of Sweden, where an entry of A either is a point of interest, which must be covered by at least one antenna, or empty space. Antennas can only be positioned at an entry in A. When an antenna is placed at row r and column c, this entry is considered covered, but also one of the neighbouring entries (c+1,r),(c,r+1),(c-1,r), or (c,r-1), is covered depending on the type chosen for this particular antenna. What is the least number of antennas for which there exists a placement in A such that all points of interest are covered?
Input
On the first row of input is a single positive integer n, specifying the number of scenarios that follow. Each scenario begins with a row containing two positive integers h and w, with 1 <= h <= 40 and 0 < w <= 10. Thereafter is a matrix presented, describing
the points of interest in Sweden in the form of h lines, each containing w characters from the set ['*','o']. A '*'-character symbolises a point of interest, whereas a 'o'-character represents open space.
Output
For each scenario, output the minimum number of antennas necessary to cover all '*'-entries in the scenario's matrix, on a row of its own.
Sample Input
2 7 9 ooo**oooo **oo*ooo* o*oo**o** ooooooooo *******oo o*o*oo*oo *******oo 10 1 * * * o * * * * * *
Sample Output
17 5
题意:
‘*’代表城市
'o'代表空地
要求你用最小的无线电雷达来覆盖所有的城市
雷达只可以建立在城市上
每一个雷达,只可以覆盖它自己所在的位置和与它相邻的一个位置
【上 || 下 || 左】
注意:也就是说一个雷达只可以覆盖两个城市
'o'代表空地
要求你用最小的无线电雷达来覆盖所有的城市
雷达只可以建立在城市上
每一个雷达,只可以覆盖它自己所在的位置和与它相邻的一个位置
【上 || 下 || 左】
注意:也就是说一个雷达只可以覆盖两个城市
英文题目太坑了。。。
算法:二分图的匹配 ———— 最小路径覆盖
最小路径覆盖=最小路径覆盖=|G|-最大匹配数
二分图的最大匹配总结
思路:
拆点,把每一个城市看成是两个点
具体分析还是看这篇博客学去吧,不能比她说的更好了Orz
http://blog.csdn.net/lyy289065406/article/details/6647040
具体分析还是看这篇博客学去吧,不能比她说的更好了Orz
http://blog.csdn.net/lyy289065406/article/details/6647040
PS:感觉还是分不怎么清什么时候用最小路径覆盖,什么时候用最大匹配
(╯▽╰)╭先记住直接匹配的就用最大匹配
要拆点的就用最小路径覆盖好了,水菜伤不起
code:
/********************************************************************* 题意:‘*’代表城市 'o'代表空地 要求你用最小的无线电雷达来覆盖所有的城市 雷达只可以建立在城市上 每一个雷达,只可以覆盖它自己所在的位置和与它相邻的一个位置 【上 || 下 || 左】 注意:也就是说一个雷达只可以覆盖两个城市 算法:二分图的匹配 ———— 最小路径覆盖 最小路径覆盖=最小路径覆盖=|G|-最大匹配数 思路:拆点,把每一个城市看成是两个点 具体分析还是看这篇博客学去吧,不能比她说的更好了Orz http://blog.csdn.net/lyy289065406/article/details/6647040 PS:感觉还是分不怎么清什么时候用最小路径覆盖,什么时候用最大匹配 ╮(╯▽╰)╭先记住直接匹配的就用最大匹配 要拆点的就用最小路径覆盖好了,水菜伤不起 **********************************************************************/ #include<stdio.h> #include<string.h> #include<algorithm> #include<iostream> using namespace std; const int maxn = 40*10+10; int map[45][15]; int g[maxn][maxn]; int linker[maxn]; int vis[maxn]; int uN,vN; bool dfs(int u) { for(int v = 1; v <= vN; v++) { if(!vis[v] && g[u][v]) { vis[v] = 1; if(linker[v] == -1 || dfs(linker[v])) { linker[v] = u; return true; } } } return false; } int hungary() { int sum = 0; memset(linker,-1,sizeof(linker)); for(int u = 1; u <= uN; u++) { memset(vis,0,sizeof(vis)); if(dfs(u)) sum++; } return sum; } int main() { int T; int row,col; scanf("%d", &T); while(T--) { scanf("%d%d%*c", &row,&col); char c; int num = 0; //记录有多少个点 memset(map,0,sizeof(map)); memset(g,0,sizeof(g)); for(int i = 1; i <= row; i++) //从 1 开始, map[][]周围加边 { for(int j = 1; j <= col; j++) { scanf("%c", &c); if(c == '*') map[i][j] = ++num; } getchar(); } uN = vN = num; for(int i = 1; i <= row; i++) //建图 { for(int j = 1; j <= col; j++) { if(map[i][j]) { if(map[i][j+1]) g[map[i][j]][map[i][j+1]] = 1; if(map[i][j-1]) g[map[i][j]][map[i][j-1]] = 1; if(map[i+1][j]) g[map[i][j]][map[i+1][j]] = 1; if(map[i-1][j]) g[map[i][j]][map[i-1][j]] = 1; } } } printf("%d ", num-hungary()/2); //最小路径覆盖数 } return 0; }