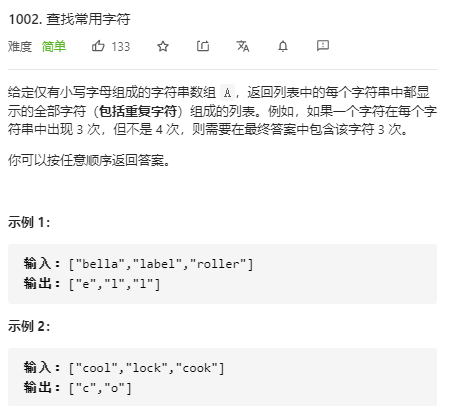
package com.example.lettcode.dailyexercises;
import java.util.*;
/**
* @Class CommonChars
* @Description 1002 查找常用字符串
* @Author
* @Date 2020/10/14
**/
public class CommonChars {
// 只有小写字母,可以利用一个长度位26的数组
public static List<String> commonChars(String[] A) {
int[] minFreq = new int[26];
// 用于保存常用的字符串
Arrays.fill(minFreq, Integer.MAX_VALUE);
List<String> ans = new ArrayList<>();
for (int i = 0; i < A.length; i++) {
int[] freq = new int[26];
// 统计每个字符串中每个字符出现的次数
for (int j = 0; j < A[i].length(); j++) {
++freq[A[i].charAt(j) - 'a'];
}
// 到当前字符串为止,所能保留的常用字符串
for (int j = 0; j < 26; j++) {
minFreq[j] = Math.min(minFreq[j], freq[j]);
}
}
// 统计常用字符串
for (int i = 0; i < 26; i++) {
// 同一个字符可能会被重复使用多次
for (int j = 0; j < minFreq[i]; j++) {
ans.add(String.valueOf((char) (i+ 'a')));
}
}
return ans;
}
public static void main(String[] args) {
String[] A = new String[]{"bella", "label", "roller"};
List<String> ans = commonChars(A);
System.out.println("CommonChars demo01 result:");
for (String s : ans) {
System.out.print(s + ',');
}
System.out.println();
A = new String[]{"cool", "lock", "cook"};
ans = commonChars(A);
System.out.println("CommonChars demo02 result:");
for (String s : ans) {
System.out.print(s + ',');
}
}
}