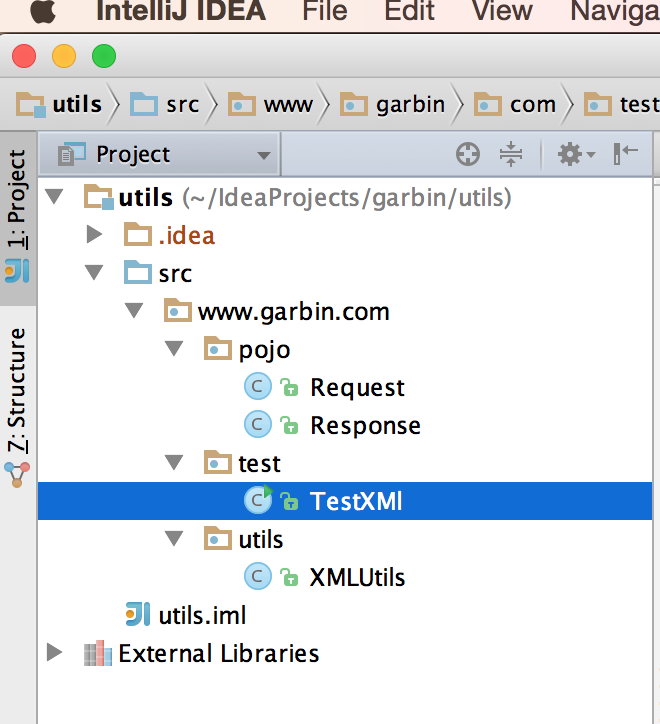
package www.garbin.com.utils;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import java.io.IOException;
import java.io.StringReader;
import java.io.StringWriter;
/**
* Created by linjiabin on 14/11/27.
*/
public class XMLUtils {
public static <T> T xml2Bean(Class<T> bean, String xmlStr) {
T s = null;
try {
JAXBContext context = JAXBContext.newInstance(bean);
Unmarshaller unmarshaller = context.createUnmarshaller();
s = (T)unmarshaller.unmarshal(new StringReader(xmlStr));
} catch (JAXBException e) {
e.printStackTrace();
}
return s;
}
public static <T> String bean2Xml(T bean) {
String xmlStr = null;
StringWriter writer = null;
try {
JAXBContext context = JAXBContext.newInstance(bean.getClass());
Marshaller marshaller = context.createMarshaller();
writer = new StringWriter();
marshaller.marshal(bean, writer);
xmlStr = writer.toString();
} catch (JAXBException e) {
e.printStackTrace();
} finally {
if (null != writer) {
try {
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return xmlStr;
}
}
package www.garbin.com.pojo;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
/**
* Created by linjiabin on 14/11/28.
*/
@XmlRootElement(name = "Request")
public class Request {
private String service;
private String lang;
private String head;
private Body body;
@XmlAttribute(name = "service")
public String getService() {
return service;
}
public void setService(String service) {
this.service = service;
}
@XmlAttribute(name = "lang")
public String getLang() {
return lang;
}
public void setLang(String lang) {
this.lang = lang;
}
@XmlElement(name = "Head")
public String getHead() {
return head;
}
public void setHead(String head) {
this.head = head;
}
@XmlElement(name = "Body")
public Body getBody() {
return body;
}
public void setBody(Body body) {
this.body = body;
}
public static class Body {
private Order order;
@XmlElement(name = "Order")
public Order getOrder() {
return order;
}
public void setOrder(Order order) {
this.order = order;
}
}
public static class Order {
private String orderId;
private String expressType;
private String jCompany;
private String jContact;
private String jTel;
private String jAddress;
private String dCompany;
private String dContact;
private String dTel;
private String dAddress;
private Integer parcelQuantity;
private String payMethod;
private OrderOption orderOption;
private Extra extra;
@XmlAttribute(name = "orderid")
public String getOrderId() {
return orderId;
}
public void setOrderId(String orderId) {
this.orderId = orderId;
}
@XmlAttribute(name = "express_type")
public String getExpressType() {
return expressType;
}
public void setExpressType(String expressType) {
this.expressType = expressType;
}
@XmlAttribute(name = "getj_company")
public String getjCompany() {
return jCompany;
}
public void setjCompany(String jCompany) {
this.jCompany = jCompany;
}
@XmlAttribute(name = "j_contact")
public String getjContact() {
return jContact;
}
public void setjContact(String jContact) {
this.jContact = jContact;
}
@XmlAttribute(name = "j_tel")
public String getjTel() {
return jTel;
}
public void setjTel(String jTel) {
this.jTel = jTel;
}
@XmlAttribute(name = "j_address")
public String getjAddress() {
return jAddress;
}
public void setjAddress(String jAddress) {
this.jAddress = jAddress;
}
@XmlAttribute(name = "j_company")
public String getdCompany() {
return dCompany;
}
public void setdCompany(String dCompany) {
this.dCompany = dCompany;
}
@XmlAttribute(name = "d_contact")
public String getdContact() {
return dContact;
}
public void setdContact(String dContact) {
this.dContact = dContact;
}
@XmlAttribute(name = "d_tel")
public String getdTel() {
return dTel;
}
public void setdTel(String dTel) {
this.dTel = dTel;
}
@XmlAttribute(name = "d_address")
public String getdAddress() {
return dAddress;
}
public void setdAddress(String dAddress) {
this.dAddress = dAddress;
}
@XmlAttribute(name = "parcel_quantity")
public Integer getParcelQuantity() {
return parcelQuantity;
}
public void setParcelQuantity(Integer parcelQuantity) {
this.parcelQuantity = parcelQuantity;
}
@XmlAttribute(name = "pay_method")
public String getPayMethod() {
return payMethod;
}
public void setPayMethod(String payMethod) {
this.payMethod = payMethod;
}
@XmlElement(name = "OrderOption")
public OrderOption getOrderOption() {
return orderOption;
}
public void setOrderOption(OrderOption orderOption) {
this.orderOption = orderOption;
}
@XmlElement(name = "Extra")
public Extra getExtra() {
return extra;
}
public void setExtra(Extra extra) {
this.extra = extra;
}
}
public static class OrderOption {
private String custId;
private String jShippercode;
private String dDeliverycode;
private String cargo;
private Float cargoTotalWeight;
private String sendstarttime;
private String mailno;
private String remark;
private AddedService addedService;
@XmlAttribute(name = "custid")
public String getCustId() {
return custId;
}
public void setCustId(String custId) {
this.custId = custId;
}
@XmlAttribute(name = "j_shippercode")
public String getjShippercode() {
return jShippercode;
}
public void setjShippercode(String jShippercode) {
this.jShippercode = jShippercode;
}
@XmlAttribute(name = "d_deliverycode")
public String getdDeliverycode() {
return dDeliverycode;
}
public void setdDeliverycode(String dDeliverycode) {
this.dDeliverycode = dDeliverycode;
}
@XmlAttribute(name = "cargo")
public String getCargo() {
return cargo;
}
public void setCargo(String cargo) {
this.cargo = cargo;
}
@XmlAttribute(name = "cargo_total_weight")
public Float getCargoTotalWeight() {
return cargoTotalWeight;
}
public void setCargoTotalWeight(Float cargoTotalWeight) {
this.cargoTotalWeight = cargoTotalWeight;
}
@XmlAttribute(name = "sendstarttime")
public String getSendstarttime() {
return sendstarttime;
}
public void setSendstarttime(String sendstarttime) {
this.sendstarttime = sendstarttime;
}
@XmlAttribute(name = "mailno")
public String getMailno() {
return mailno;
}
public void setMailno(String mailno) {
this.mailno = mailno;
}
@XmlAttribute(name = "remark")
public String getRemark() {
return remark;
}
public void setRemark(String remark) {
this.remark = remark;
}
@XmlElement(name = "AddedService")
public AddedService getAddedService() {
return addedService;
}
public void setAddedService(AddedService addedService) {
this.addedService = addedService;
}
}
public static class AddedService {
private String name;
private String value;
private String value1;
private String value2;
private String value3;
@XmlAttribute(name = "name")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@XmlAttribute(name = "value")
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
@XmlAttribute(name = "value1")
public String getValue1() {
return value1;
}
public void setValue1(String value1) {
this.value1 = value1;
}
@XmlAttribute(name = "value2")
public String getValue2() {
return value2;
}
public void setValue2(String value2) {
this.value2 = value2;
}
@XmlAttribute(name = "value3")
public String getValue3() {
return value3;
}
public void setValue3(String value3) {
this.value3 = value3;
}
}
public static class Extra {
private String e1;
@XmlAttribute(name = "e1")
public String getE1() {
return e1;
}
public void setE1(String e1) {
this.e1 = e1;
}
}
}
package www.garbin.com.pojo;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlValue;
import java.io.Serializable;
/**
* Created by linjiabin on 14/11/28.
*/
@XmlRootElement(name = "Response")
public class Response implements Serializable {
private String service;
private String head;
private Error error;
private Body body;
@XmlAttribute(name = "service")
public String getService() {
return service;
}
public void setService(String service) {
this.service = service;
}
@XmlElement(name = "Head")
public String getHead() {
return head;
}
public void setHead(String head) {
this.head = head;
}
@XmlElement(name = "ERROR")
public Error getError() {
return error;
}
public void setError(Error error) {
this.error = error;
}
@XmlElement(name = "Body")
public Body getBody() {
return body;
}
public void setBody(Body body) {
this.body = body;
}
public static class Error {
private String value;
private String code;
@XmlValue
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
@XmlAttribute(name = "code")
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
}
public static class Body {
private OrderResponse orderResponse;
@XmlElement(name = "OrderResponse")
public OrderResponse getOrderResponse() {
return orderResponse;
}
public void setOrderResponse(OrderResponse orderResponse) {
this.orderResponse = orderResponse;
}
}
public static class OrderResponse {
private String orderId;
private String mailno;
private String origincode;
private String destcode;
private String filterResut;
private String remark;
@XmlAttribute(name = "orderid")
public String getOrderId() {
return orderId;
}
public void setOrderId(String orderId) {
this.orderId = orderId;
}
@XmlAttribute(name = "mailno")
public String getMailno() {
return mailno;
}
public void setMailno(String mailno) {
this.mailno = mailno;
}
@XmlAttribute(name = "origincode")
public String getOrigincode() {
return origincode;
}
public void setOrigincode(String origincode) {
this.origincode = origincode;
}
@XmlAttribute(name = "destcode")
public String getDestcode() {
return destcode;
}
public void setDestcode(String destcode) {
this.destcode = destcode;
}
@XmlAttribute(name = "filter_result")
public String getFilterResut() {
return filterResut;
}
public void setFilterResut(String filterResut) {
this.filterResut = filterResut;
}
@XmlAttribute(name = "remark")
public String getRemark() {
return remark;
}
public void setRemark(String remark) {
this.remark = remark;
}
}
}
package www.garbin.com.test;
import www.garbin.com.pojo.Request;
import www.garbin.com.utils.XMLUtils;
import javax.xml.bind.JAXBException;
import java.rmi.RemoteException;
import java.util.Random;
/**
* Created by linjiabin on 14/11/28.
*/
public class TestXMl {
public static void main(String[] args) throws JAXBException, RemoteException {
Request request = new Request();
request.setLang("zh-CN");
request.setService("OrderService");
Request.Body body = new Request.Body();
Request.Order requestOrder = new Request.Order();
requestOrder.setOrderId("WSD_22222232" + "_" + new Random().nextInt());
requestOrder.setdAddress("福建厦门市思明区软件园二期望海路55号");
requestOrder.setjAddress("福建厦门市思明区软件园二期望海路55号");
requestOrder.setdTel("13506962224");
requestOrder.setjTel("13514087953");
requestOrder.setExpressType("2");
requestOrder.setParcelQuantity(1);
requestOrder.setPayMethod("1");
Request.OrderOption orderOption = new Request.OrderOption();
orderOption.setCustId("5555555555");
Request.AddedService addedService = new Request.AddedService();
addedService.setName("COD");
addedService.setValue("1111");
addedService.setValue1("5555555555");
orderOption.setAddedService(addedService);
requestOrder.setOrderOption(orderOption);
body.setOrder(requestOrder);
request.setBody(body);
request.setHead("SHFJ,Eta7siUxZX5zIfNO");
String xmlStr = XMLUtils.bean2Xml(request);
System.out.println(xmlStr);
}
}