一、CountDownLatchDemo
package com.duchong.concurrent;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.CountDownLatch;
/*
* CountDownLatch :阻塞主线程,等子线程完成
*/
public class CountDownLatchDemo {
/**
* 存放子线程产生的结果
*/
private static ConcurrentHashMap<String,Integer> resultMap =new ConcurrentHashMap<>();
public static void main(String[] args) {
final CountDownLatch latch = new CountDownLatch(5);
SubThread subThread = new SubThread(latch);
for (int i = 0; i <=4; i++) {
new Thread(subThread).start();
}
try {
//阻塞主线程
latch.await();
}
catch (InterruptedException e) {
}
//计算总结果
int sum=0;
for(Map.Entry<String,Integer> subNumber:resultMap.entrySet()){
sum +=subNumber.getValue();
}
System.out.println("sum = "+sum);
}
/**
* 子线程
*/
static class SubThread implements Runnable {
private CountDownLatch latch;
public SubThread(CountDownLatch latch) {
this.latch = latch;
}
@Override
public void run() {
String name = Thread.currentThread().getName();
try {
int number = RandomUtil.getNumber();
System.out.println(name+"---number:"+number);
resultMap.put(name,number);
}
finally {
latch.countDown();
}
}
}
}
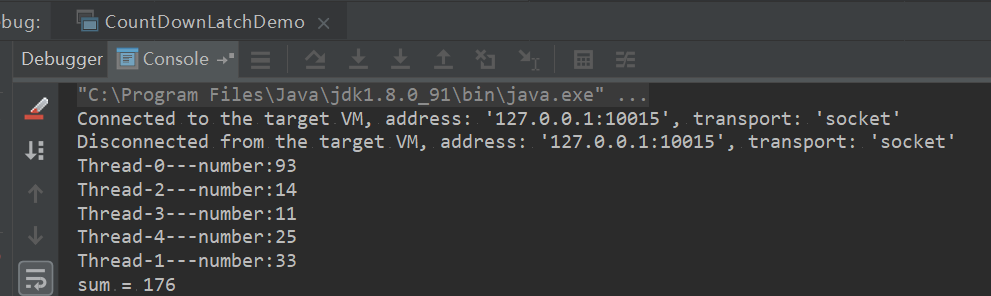
二、CyclicBarrierDemo
package com.duchong.concurrent;
import java.util.Map;
import java.util.concurrent.BrokenBarrierException;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.CyclicBarrier;
/*
* CyclicBarrier :阻塞子线程,当等待中的子线程数到达一定数量时,跳闸。
*/
public class CyclicBarrierDemo {
/**
* 屏障,初始5 当await()的线程数量达到5时,跳闸。
*/
static CyclicBarrier c = new CyclicBarrier(5,new SumThread());
/**
* 存放子线程产生的结果
*/
private static ConcurrentHashMap<String,Integer> resultMap =new ConcurrentHashMap<>();
public static void main(String[] args) {
//模拟四个子线产生随机数值
for(int i=0;i<=4;i++){
new Thread(new SubThread()).start();
}
}
/**
* 所有子线程等待数等于5时,执行
*/
private static class SumThread implements Runnable{
@Override
public void run() {
int result =0;
for(Map.Entry<String,Integer> workResult:resultMap.entrySet()){
result = result+workResult.getValue();
}
System.out.println("result = "+result);
}
}
/**
* 子线程
*/
static class SubThread implements Runnable{
@Override
public void run() {
String name = Thread.currentThread().getName();
int number = RandomUtil.getNumber();
System.out.println(name+"---number:"+number);
resultMap.put(name,number);
try {
//阻塞子线程
c.await();
} catch (InterruptedException e) {
e.printStackTrace();
} catch (BrokenBarrierException e) {
e.printStackTrace();
}
}
}
}
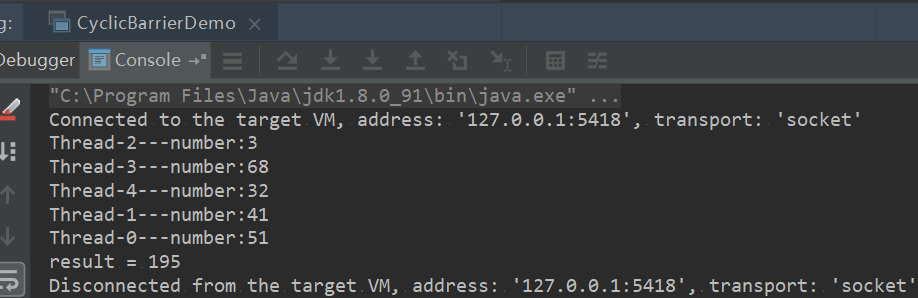