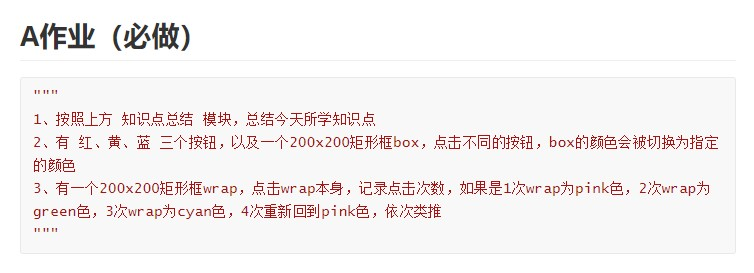
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>exercise</title>
<style>
.abc{
200px;
height: 200px;
}
.green{
background-color: green;
}
.red{
background-color: red;
}
.blue{
background-color: blue;
}
</style>
</head>
<body>
<div id="id1">
<div>
<input type="button" class="btn1 " @click="change_color1">
</div>
<div>
<input type="button" class="btn2 " @click="change_color2">
</div>
<div>
<input type="button" class="btn3 " @click="change_color3">
</div>
<div :style="myStyle1" id="id2">box</div>
</div>
</body>
<script src="vue.js"></script>
<script>
new Vue({
el:'#id1',
data:{
myStyle1: {
'200px',
height: '200px',
backgroundColor: 'orange'
},
},
methods:{
change_color1(){
this.myStyle1.backgroundColor = 'green'
},
change_color2(){
this.myStyle1.backgroundColor = 'red'
},
change_color3(){
this.myStyle1.backgroundColor = 'blue'
},
}
})
</script>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>exercise</title>
</head>
<body>
<div id="id1">
<div>
<input type="button" class="btn1 " @click="change_color1">
</div>
<div :style="myStyle1" id="id2" >{{ num }}</div>
</div>
</body>
<script src="vue.js"></script>
<script>
new Vue({
el:'#id1',
data:{
num:0,
color:['pink','cyan','green'],
myStyle1: {
'200px',
height: '200px',
backgroundColor: 'orange'
},
},
methods:{
change_color1(){
this.num += 1;
color1 = this.num%3;
console.log(color1);
this.myStyle1.backgroundColor = this.color[color1];
}
}
})
</script>
</html>
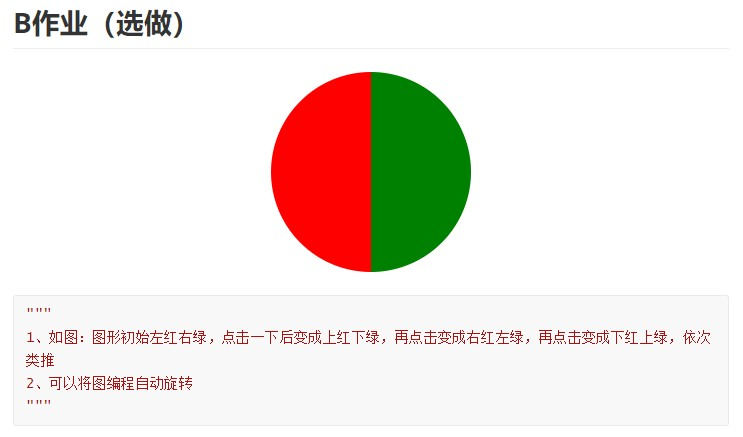
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>exercise</title>
<style>
.abc{
200px;
height: 200px;
}
.green{
background-color: green;
}
.red{
background-color: red;
}
.blue{
background-color: blue;
}
</style>
</head>
<body>
<div id="id1">
<div>
<input type="button" class="btn1 " @click="change_color1">
</div>
<div>
<input type="button" class="btn2 " @click="change_color2">
</div>
<div>
<input type="button" class="btn3 " @click="change_color3">
</div>
<div :style="myStyle1" id="id2">box</div>
</div>
</body>
<script src="vue.js"></script>
<script>
new Vue({
el:'#id1',
data:{
myStyle1: {
'200px',
height: '200px',
backgroundColor: 'orange'
},
},
methods:{
change_color1(){
this.myStyle1.backgroundColor = 'green'
},
change_color2(){
this.myStyle1.backgroundColor = 'red'
},
change_color3(){
this.myStyle1.backgroundColor = 'blue'
},
}
})
</script>
</html>