数据库准备—创建db_login数据库 t_user表
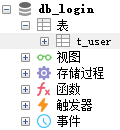

1、创建web工程
2、创建用户model user.java
1 package com.gxy.model;
2
3 public class User {
4 private int id;
5 private String userName;
6 private String password;
7
8 public User() {
9 super();
10 }
11
12
13 public User(String userName, String password) {
14 super();
15 this.userName = userName;
16 this.password = password;
17 }
18
19
20 public int getId() {
21 return id;
22 }
23 public void setId(int id) {
24 this.id = id;
25 }
26 public String getUserName() {
27 return userName;
28 }
29 public void setUserName(String userName) {
30 this.userName = userName;
31 }
32 public String getPassword() {
33 return password;
34 }
35 public void setPassword(String password) {
36 this.password = password;
37 }
38
39 }
3、创建util包 Dbutil.java
1 package com.gxy.util;
2
3 import java.sql.Connection;
4 import java.sql.DriverManager;
5
6 public class Dbutil {
7 private String dbUrl ="jdbc:mysql://localhost:3306/db_login";
8 private String jdbcName="com.mysql.jdbc.Driver";
9 private String dbUserName="root";
10 private String dbpassword="123456";
11
12 public Connection getcon() throws Exception{
13 Class.forName(jdbcName);
14 Connection con=DriverManager.getConnection(dbUrl,dbUserName,dbpassword);
15 return con;
16 }
17
18 public void closeCon(Connection con) throws Exception{
19 con.close();
20 }
21
22 }
4、创建dao包 UserDao.java
1 package com.gxy.dao;
2
3 import java.sql.Connection;
4 import java.sql.PreparedStatement;
5 import java.sql.ResultSet;
6
7 import com.gxy.model.User;
8
9 public class UserDao {
10 public User login(Connection con,User user) throws Exception{
11 User resultUser=null;
12 String sql="select * from t_user where userName=? and passWord=?";
13 PreparedStatement pst=con.prepareStatement(sql);
14 pst.setString(1, user.getUserName());
15 pst.setString(2,user.getPassword());
16 ResultSet rs=pst.executeQuery();
17 if(rs.next()){
18 resultUser=new User();
19 resultUser.setUserName(rs.getString("userName"));
20 resultUser.setPassword(rs.getString("passWord"));
21 }
22 return resultUser;
23 }
24
25 }
5、创建servlet包 loginServlet
1 package com.gxy.servlet;
2
3 import java.io.IOException;
4 import java.sql.Connection;
5
6 import javax.servlet.RequestDispatcher;
7 import javax.servlet.ServletException;
8 import javax.servlet.http.HttpServlet;
9 import javax.servlet.http.HttpServletRequest;
10 import javax.servlet.http.HttpServletResponse;
11 import javax.servlet.http.HttpSession;
12
13 import com.gxy.dao.UserDao;
14 import com.gxy.model.User;
15 import com.gxy.util.Dbutil;
16
17 public class loginServlet extends HttpServlet{
18
19 /**
20 *
21 */
22 private static final long serialVersionUID = 1L;
23
24 Dbutil dbutil=new Dbutil();
25 UserDao userDao=new UserDao();
26 @Override
27 protected void doGet(HttpServletRequest req, HttpServletResponse resp)
28 throws ServletException, IOException {
29 this.doPost(req, resp);
30 }
31
32 @Override
33 protected void doPost(HttpServletRequest req, HttpServletResponse resp)
34 throws ServletException, IOException {
35 String userName=req.getParameter("userName");
36 String passWord=req.getParameter("passWord");
37
38 Connection con=null;
39 try {
40 User user=new User(userName,passWord);
41 con=dbutil.getcon();
42 User resultUser=userDao.login(con, user);
43 if(resultUser==null){
44 System.out.println("no");
45 }else{
46 HttpSession session=req.getSession();
47 session.setAttribute("userName", resultUser.getUserName());
48 session.setAttribute("passWord", resultUser.getPassword());
49 resp.sendRedirect("target.jsp");
50 }
51 } catch (Exception e) {
52 // TODO Auto-generated catch block
53 e.printStackTrace();
54 }
55
56 }
57
58 }
6、用户登录界面 login.jsp
1 <%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
2 <%
3 String path = request.getContextPath();
4 String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
5 %>
6
7 <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
8 <html>
9 <head>
10 <base href="<%=basePath%>">
11
12 <title>My JSP 'login.jsp' starting page</title>
13
14 <meta http-equiv="pragma" content="no-cache">
15 <meta http-equiv="cache-control" content="no-cache">
16 <meta http-equiv="expires" content="0">
17 <meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
18 <meta http-equiv="description" content="This is my page">
19 <!--
20 <link rel="stylesheet" type="text/css" href="styles.css">
21 -->
22
23 </head>
24
25 <body>
26 <form action="login" method="post">
27 <table>
28 <tr>
29 <td>用户名:</td>
30 <td><input type="text" id="userName" name="userName"></td>
31 </tr>
32 <tr>
33 <td>密码:</td>
34 <td><input type="password" id="passWord" name="passWord"></td>
35 </tr>
36 <tr>
37 <td colspan="2"><input type="submit" value="提交" ></td>
38 </tr>
39 </table>
40 </form>
41 </body>
42 </html>
跳转界面 target.jsp
1 <%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
2 <%
3 String path = request.getContextPath();
4 String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
5 %>
6
7 <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
8 <html>
9 <head>
10 <base href="<%=basePath%>">
11
12 <title>My JSP 'target.jsp' starting page</title>
13
14 <meta http-equiv="pragma" content="no-cache">
15 <meta http-equiv="cache-control" content="no-cache">
16 <meta http-equiv="expires" content="0">
17 <meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
18 <meta http-equiv="description" content="This is my page">
19 <!--
20 <link rel="stylesheet" type="text/css" href="styles.css">
21 -->
22
23 </head>
24
25 <body>
26 <h1>用户名:<%=session.getAttribute("userName") %></h1>
27 <h1>密码:<%=session.getAttribute("passWord") %></h1>
28 </body>
29 </html>
7、运行结果

