在网站开发中,经常会遇到要生成二维码的情况,比如要使用微信支付、网页登录等,本文分享一个Spring Boot生成二维码的例子,这里用到了google的zxing工具类。
本文目录
一、二维码简介二、编写代码生成二维码1.引入jar包2.编写工具类3.编写控制层代码4.运行并查看效果
一、二维码简介
二维码又称为QR Code,QR全称是Quick Response,是一个近几年来移动设备上超流行的一种编码方式。
二维码是用某种特定的几何图形按一定规律在平面(二维方向上)分布的黑白相间的图形记录数据符号信息的。
主要应用场景如下:
- 信息获取(名片、地图、WIFI密码、资料)
- 网站跳转(跳转到微博、手机网站、网站)
- 广告推送(用户扫码,直接浏览商家推送的视频、音频广告)
- 手机电商(用户扫码、手机直接购物下单)
- 防伪溯源(用户扫码、即可查看生产地;同时后台可以获取最终消费地)
- 优惠促销(用户扫码,下载电子优惠券,抽奖)
- 会员管理(用户手机上获取电子会员信息、VIP服务)
- 手机支付(扫描商品二维码,通过银行或第三方支付提供的手机端通道完成支付)
- 账号登录(扫描二维码进行各个网站或软件的登录)
二、编写代码生成二维码
1.引入jar包
pom.xml中引入zxing的jar包。
<!-- 二维码支持包 -->
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.2.0</version>
</dependency>
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>javase</artifactId>
<version>3.2.0</version>
</dependency>
2.编写工具类
QRCodeUtil.java代码如下:
/**
* QRCodeUtil 生成二维码工具类
*/
public class QRCodeUtil {
private static final String CHARSET = "utf-8";
private static final String FORMAT_NAME = "JPG";
// 二维码尺寸
private static final int QRCODE_SIZE = 300;
// LOGO宽度
private static final int WIDTH = 60;
// LOGO高度
private static final int HEIGHT = 60;
private static BufferedImage createImage(String content, String imgPath, boolean needCompress) throws Exception {
Hashtable hints = new Hashtable();
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
hints.put(EncodeHintType.CHARACTER_SET, CHARSET);
hints.put(EncodeHintType.MARGIN, 1);
BitMatrix bitMatrix = new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE, QRCODE_SIZE, QRCODE_SIZE,
hints);
int width = bitMatrix.getWidth();
int height = bitMatrix.getHeight();
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
image.setRGB(x, y, bitMatrix.get(x, y) ? 0xFF000000 : 0xFFFFFFFF);
}
}
if (imgPath == null || "".equals(imgPath)) {
return image;
}
// 插入图片
QRCodeUtil.insertImage(image, imgPath, needCompress);
return image;
}
private static void insertImage(BufferedImage source, String imgPath, boolean needCompress) throws Exception {
File file = new File(imgPath);
if (!file.exists()) {
System.err.println("" + imgPath + " 该文件不存在!");
return;
}
Image src = ImageIO.read(new File(imgPath));
int width = src.getWidth(null);
int height = src.getHeight(null);
if (needCompress) { // 压缩LOGO
if (width > WIDTH) {
width = WIDTH;
}
if (height > HEIGHT) {
height = HEIGHT;
}
Image image = src.getScaledInstance(width, height, Image.SCALE_SMOOTH);
BufferedImage tag = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics g = tag.getGraphics();
g.drawImage(image, 0, 0, null); // 绘制缩小后的图
g.dispose();
src = image;
}
// 插入LOGO
Graphics2D graph = source.createGraphics();
int x = (QRCODE_SIZE - width) / 2;
int y = (QRCODE_SIZE - height) / 2;
graph.drawImage(src, x, y, width, height, null);
Shape shape = new RoundRectangle2D.Float(x, y, width, width, 6, 6);
graph.setStroke(new BasicStroke(3f));
graph.draw(shape);
graph.dispose();
}
public static void encode(String content, String imgPath, String destPath, boolean needCompress) throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath, needCompress);
mkdirs(destPath);
ImageIO.write(image, FORMAT_NAME, new File(destPath));
}
public static BufferedImage encode(String content, String imgPath, boolean needCompress) throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath, needCompress);
return image;
}
public static void mkdirs(String destPath) {
File file = new File(destPath);
// 当文件夹不存在时,mkdirs会自动创建多层目录,区别于mkdir.(mkdir如果父目录不存在则会抛出异常)
if (!file.exists() && !file.isDirectory()) {
file.mkdirs();
}
}
public static void encode(String content, String imgPath, OutputStream output, boolean needCompress)
throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath, needCompress);
ImageIO.write(image, FORMAT_NAME, output);
}
public static void encode(String content, OutputStream output) throws Exception {
QRCodeUtil.encode(content, null, output, false);
}
}
3.编写控制层代码
QrCodeController.java代码如下:
/**
* 根据 url 生成 普通二维码
*/
@RequestMapping(value = "/createCommonQRCode")
public void createCommonQRCode(HttpServletResponse response, String url) throws Exception {
ServletOutputStream stream = null;
try {
stream = response.getOutputStream();
//使用工具类生成二维码
QRCodeUtil.encode(url, stream);
} catch (Exception e) {
e.getStackTrace();
} finally {
if (stream != null) {
stream.flush();
stream.close();
}
}
}
/**
* 根据 url 生成 带有logo二维码
*/
@RequestMapping(value = "/createLogoQRCode")
public void createLogoQRCode(HttpServletResponse response, String url) throws Exception {
ServletOutputStream stream = null;
try {
stream = response.getOutputStream();
String logoPath = Thread.currentThread().getContextClassLoader().getResource("").getPath()
+ "templates" + File.separator + "logo.jpg";
//使用工具类生成二维码
QRCodeUtil.encode(url, logoPath, stream, true);
} catch (Exception e) {
e.getStackTrace();
} finally {
if (stream != null) {
stream.flush();
stream.close();
}
}
}
4.运行并查看效果
本项目中提供了生成普通二维码和带有logo二维码的两个接口,启动项目,我们来演示下生成下http://www.baidu.com这个url的二维码;
- 生成普通二维码
本地浏览器打开http://localhost:8080/createCommonQRCode?url=http://www.baidu.com,生成的二维码截图如下:
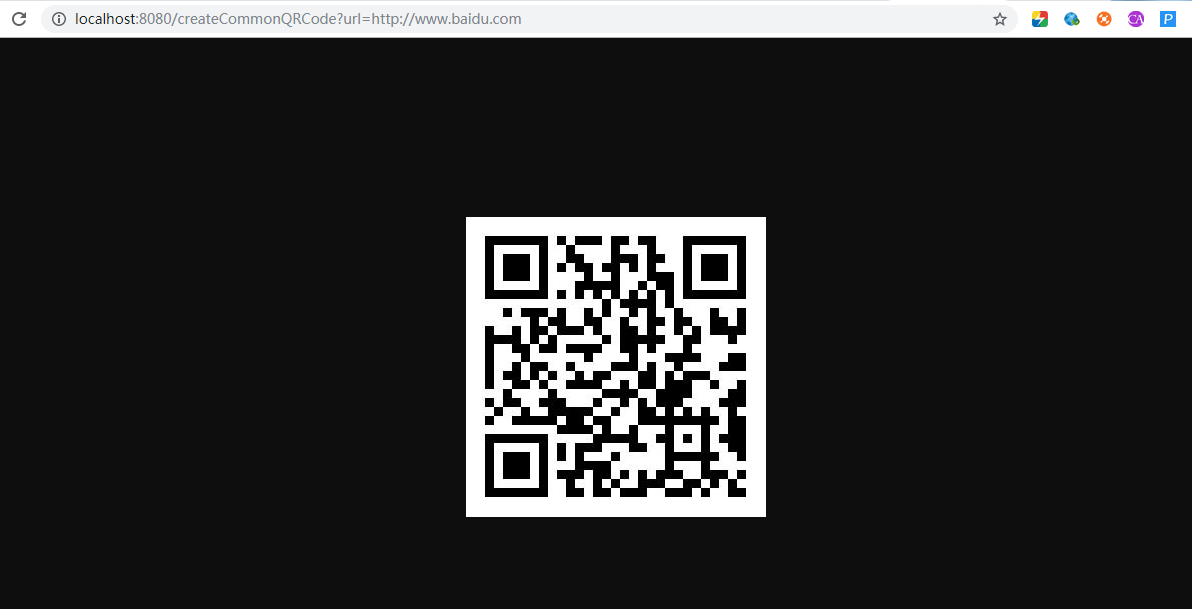
生成百度的普通二维码
- 生成带logo的二维码
本地浏览器打开http://localhost:8080/createLogoQRCode?url=http://www.baidu.com,生成的二维码截图如下:
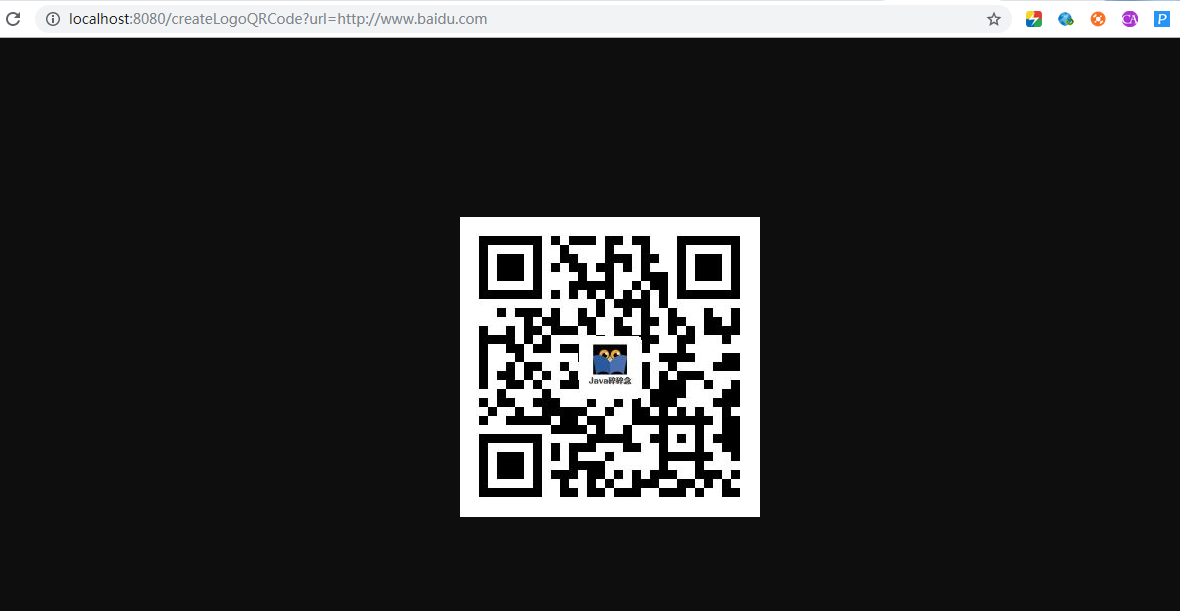
生成百度的带logo二维码
到此Spring Boot 2.X中利用ZXing生成二维码功能全部实现,有问题欢迎留言沟通哦!
完整源码地址: https://github.com/suisui2019/springboot-study
推荐阅读
1.从技术的角度分析下为什么不要在网上发“原图”
2.Spring Boot 2.X 如何快速整合jpa?
3.Spring Boot之Profile--快速搞定多环境使用与切换
4.Spring Boot 2.X整合Spring-cache,让你的网站速度飞起来
5.利用Spring Boot+WxJava实现网站集成微信登录功能
限时领取免费Java相关资料,涵盖了Java、Redis、MongoDB、MySQL、Zookeeper、Spring Cloud、Dubbo/Kafka、Hadoop、Hbase、Flink等高并发分布式、大数据、机器学习等技术。
关注下方公众号即可免费领取:
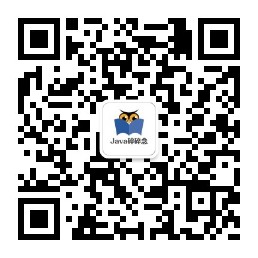