HDU 1394
Minimum Inversion Number
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 11961 Accepted Submission(s): 7310
Problem Description
The inversion number of a given number sequence a1, a2, ..., an is the number of pairs (ai, aj) that satisfy i < j and ai > aj.
For a given sequence of numbers a1, a2, ..., an, if we move the first m >= 0 numbers to the end of the seqence, we will obtain another sequence. There are totally n such sequences as the following:
a1, a2, ..., an-1, an (where m = 0 - the initial seqence)
a2, a3, ..., an, a1 (where m = 1)
a3, a4, ..., an, a1, a2 (where m = 2)
...
an, a1, a2, ..., an-1 (where m = n-1)
You are asked to write a program to find the minimum inversion number out of the above sequences.
For a given sequence of numbers a1, a2, ..., an, if we move the first m >= 0 numbers to the end of the seqence, we will obtain another sequence. There are totally n such sequences as the following:
a1, a2, ..., an-1, an (where m = 0 - the initial seqence)
a2, a3, ..., an, a1 (where m = 1)
a3, a4, ..., an, a1, a2 (where m = 2)
...
an, a1, a2, ..., an-1 (where m = n-1)
You are asked to write a program to find the minimum inversion number out of the above sequences.
Input
The input consists of a number of test cases. Each case consists of two lines: the first line contains a positive integer n (n <= 5000); the next line contains a permutation of the n integers from 0 to n-1.
Output
For each case, output the minimum inversion number on a single line.
Sample Input
10
1 3 6 9 0 8 5 7 4 2
Sample Output
16
逆序数、改段求点
#include <iostream> #include <algorithm> #include <cstdio> #include <cstring> #include <queue> #include <cmath> using namespace std; #define INF 0x3f3f3f3f #define N 5010 int n; int a[N]; int c[N]; int lowbit(int x) { return x&(-x); } void update(int pos,int val) { while(pos>0) { c[pos]+=val; pos-=lowbit(pos); } } int query(int pos) { int res=0; while(pos<=n) { res+=c[pos]; pos+=lowbit(pos); } return res; } int main() { int res; while(scanf("%d",&n)!=EOF) { res=0; memset(c,0,sizeof(c)); for(int i=1;i<=n;i++) { scanf("%d",&a[i]); a[i]++; } for(int i=1;i<=n;i++) { update(a[i]-1,1); res+=query(a[i]); } //printf("逆序数:%d",res); int Min=INF; for(int i=1;i<=n;i++) { res+=n-2*a[i]+1; Min=min(res,Min); } printf("%d ",Min); } return 0; }
POJ 2299
Ultra-QuickSort
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 43824 | Accepted: 15983 |
Description
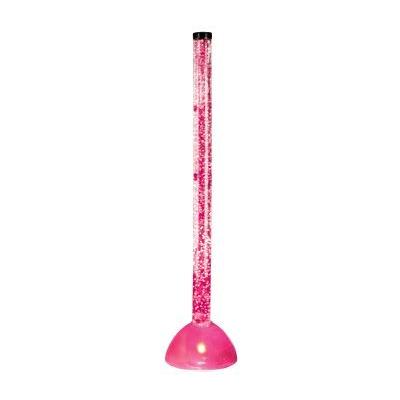
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
Source
Waterloo local 2005.02.05
#include <iostream> #include <algorithm> #include <cstdio> #include <cstring> #include <queue> #include <cmath> using namespace std; #define ll long long #define INF 0x3f3f3f3f #define N 500000 int n; int a[N]; int b[N]; int c[N]; int lowbit(int x) { return x&(-x); } void update(int pos,int val) { while(pos>0) { c[pos]+=val; pos-=lowbit(pos); } } int query(int pos) { int res=0; while(pos<=N) { res+=c[pos]; pos+=lowbit(pos); } return res; } int main() { while(scanf("%d",&n),n) { ll res=0; memset(c,0,sizeof(c)); for(int i=0;i<n;i++) { scanf("%d",&a[i]); b[i]=a[i]; } sort(b,b+n); for(int i=0;i<n;i++) { int pos=lower_bound(b,b+n,a[i])-b; pos++; update(pos-1,1); res+=query(pos); } printf("%lld ",res); } return 0; }