前序遍历,后序遍历,广度遍历,深度遍历,遍历一级节点.以及按钮如何响应点击事件。
import java.awt.*;
import java.awt.event.*;
import java.util.*;
import javax.swing.*;
import javax.swing.tree.*;
public class Tree_03 extends JFrame{
private DefaultMutableTreeNode root; //定义根节点.
//主方法.
public static void main(String[] args){
//实例化本类.
Tree_03 frame=new Tree_03();
frame.setVisible(true);
}
//构造方法.
public Tree_03(){
super(); //继承父类.
setTitle("遍历节点方法"); //设置窗口标题.
setBounds(100,100,300,300); //设置绝对位置.
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //设置窗口关闭方法.
root=new DefaultMutableTreeNode("ROOT"); //设置根节点名.
DefaultMutableTreeNode noteA=new DefaultMutableTreeNode("FirstA"); //定义及设置第一个子节点.
root.add(noteA); //将第一节点添加到树根上.
//给noteA增加两个子节点.
noteA.add(new DefaultMutableTreeNode("SecondAA"));
noteA.add(new DefaultMutableTreeNode("SecondAB"));
DefaultMutableTreeNode noteB=new DefaultMutableTreeNode("FirstB");//定义及设置第二个子节点.
root.add(noteB);
DefaultMutableTreeNode noteC=new DefaultMutableTreeNode("FirstC");//定义及设置第三个子节点.
root.add(noteC);
noteC.add(new DefaultMutableTreeNode("SecondCA"));//为noteC增加子节点.
DefaultMutableTreeNode noteSecondCB=new DefaultMutableTreeNode("SecondCB");//为noteC增加子节点.
noteC.add(noteSecondCB);//将noteSecondCB添加到noteC子节点下.
//给noteSecondCB增加子节点.
noteSecondCB.add(new DefaultMutableTreeNode("SecondCBA"));
noteSecondCB.add(new DefaultMutableTreeNode("SecondCBB"));
//给noteC增加SecondCC子节点.
noteC.add(new DefaultMutableTreeNode("SecondCC"));
JTree tree=new JTree(root);//根据根节点创建树.
getContentPane().add(tree,BorderLayout.CENTER);
//开始操作节点.
//定义Panel.
final JPanel panel=new JPanel();
panel.setLayout(new GridLayout(0,1)); //设置panel为网格布局.
getContentPane().add(panel,BorderLayout.EAST); //将panel放置到窗口右边.
final JButton button_01=new JButton("按前序遍历节点"); //定义及设置第一个按钮.
button_01.addActionListener(new ButtonActionListener("按前序遍历节点"));
panel.add(button_01); //将按钮放置到panel里.
final JButton button_02=new JButton("按后序遍历节点"); //定义及设置第二个按钮.
button_02.addActionListener(new ButtonActionListener("按后序遍历节点"));
panel.add(button_02); //将按钮放置到panel里.
final JButton button_03=new JButton("以广度遍历节点"); //定义及设置第三个按钮.
button_03.addActionListener(new ButtonActionListener("以广度遍历节点"));
panel.add(button_03); //将按钮放置到panel里.
final JButton button_04=new JButton("以深度遍历节点"); //定义及设置第四个按钮.
button_04.addActionListener(new ButtonActionListener("以深度遍历节点"));
panel.add(button_04); //将按钮放置到panel里.
final JButton button_05=new JButton("遍历直属子节点"); //定义及设置第五个按钮.
button_05.addActionListener(new ButtonActionListener("遍历直属子节点"));
panel.add(button_05); //将按钮放置到panel里.
}
//定义按钮点击事件.
private class ButtonActionListener implements ActionListener{
private String mode;//定义mode变量.
//构造方法.
public ButtonActionListener(String mode){
this.mode=mode;
}
//定义激活方法.
public void actionPerformed(ActionEvent e){
//声明节点枚举对象.
Enumeration<?> enumeration;
if(mode.equals("按前序遍历节点")){
//按前序遍历根节点.
enumeration=root.preorderEnumeration();
}else if(mode.equals("按后序遍历节点")){
enumeration=root.postorderEnumeration();
}else if(mode.equals("以广度遍历节点")){
enumeration=root.breadthFirstEnumeration();
}else if(mode.equals("以深度遍历节点")){
enumeration=root.depthFirstEnumeration();
}else{
enumeration=root.children();//遍历该节点的所有子节点.
}
//知道了遍历方式,开始遍历.
while(enumeration.hasMoreElements()){ //遍历枚举对象.
//先定义一个节点变量.
DefaultMutableTreeNode node;
node=(DefaultMutableTreeNode) enumeration.nextElement();//将节点名称给node.
//根据级别输出占位符.
for(int l=0;l<node.getLevel();l++){
System.out.print("---");
}
System.out.println(node.getUserObject());//输入节点标签.
}
System.out.println();
System.out.println();
}
}
}
运行结果:
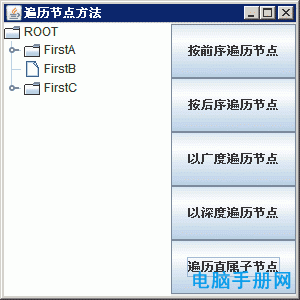
ROOT
---FirstA
------SecondAA
------SecondAB
---FirstB
---FirstC
------SecondCA
------SecondCB
---------SecondCBA
---------SecondCBB
------SecondCC
------SecondAA
------SecondAB
---FirstA
---FirstB
------SecondCA
---------SecondCBA
---------SecondCBB
------SecondCB
------SecondCC
---FirstC
ROOT
ROOT
---FirstA
---FirstB
---FirstC
------SecondAA
------SecondAB
------SecondCA
------SecondCB
------SecondCC
---------SecondCBA
---------SecondCBB
------SecondAA
------SecondAB
---FirstA
---FirstB
------SecondCA
---------SecondCBA
---------SecondCBB
------SecondCB
------SecondCC
---FirstC
ROOT
---FirstA
---FirstB
---FirstC