今日内容大纲
01 面试
1.技术 50% 2.谈吐,交朋友 50%
02 昨日知识回顾
03 作业讲解
04 类空间,对象空间,查询顺序
05 组合
06 预习博客内容
07 作业
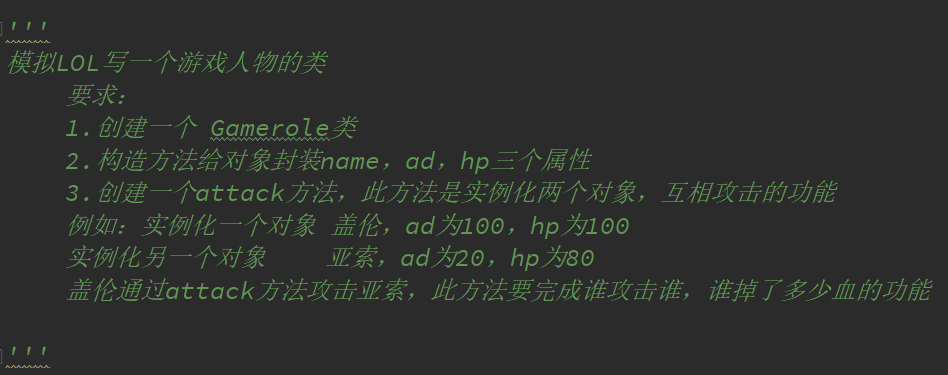
#版本一:代码不合理,人物利用武器攻击别人,动作发起者是人,而不是武器
class Game_role:
def __init__(self, name, ad, hp):
self.name = name
self.ad = ad
self.hp = hp
def attack(self, p):
p.hp = p.hp - self.ad
print('%s攻击%s,%s掉了%s血,%s还剩%s血' % (self.name, p.name, p.name, self.ad, p.name, p.hp))
p1 = Game_role('盖伦', 20, 500)
p2 = Game_role('亚索', 50, 200)
p1.attack(p2)
print(p2.hp)
class weapon:
def __init__(self,name,ad):
self.name=name
self.ad=ad
def fight(self,p1,p2):
p2.hp=p2.hp-self.ad
print('%s用%s攻击了%s,%s掉了%s血,还剩%s血'%(p1.name,self.name,p2.name,p2.name,self.ad,p2.hp))
p1 = Game_role('盖伦', 20, 500)
p2 = Game_role('亚索', 50, 200)
axe = weapon('斧子',60)
knife = weapon('小刀',20)
axe.fight(p1,p2)
axe.fight(p1,p2)
knife.fight(p1,p2)
knife.fight(p2,p1)
#版本二
class Game_role:
def __init__(self, name, ad, hp):
self.name = name
self.ad = ad
self.hp = hp
def attack(self, p):
p.hp = p.hp - self.ad
print('%s攻击%s,%s掉了%s血,%s还剩%s血' % (self.name, p.name, p.name, self.ad, p.name, p.hp))
def Armament_weapon(self,wea):
self.wea=wea
p1 = Game_role('盖伦', 20, 500)
p2 = Game_role('亚索', 50, 200)
p1.attack(p2)
print(p2.hp)
class weapon:
def __init__(self,name,ad):
self.name=name
self.ad=ad
def fight(self,p1,p2):
p2.hp=p2.hp-self.ad
print('%s用%s攻击了%s,%s掉了%s血,还剩%s血'%(p1.name,self.name,p2.name,p2.name,self.ad,p2.hp))
p1 = Game_role('盖伦', 20, 500)
p2 = Game_role('亚索', 50, 200)
axe = weapon('斧子',60)
knife = weapon('小刀',20)
print(axe)
p1.Armament_weapon(axe) #给p1装备了斧子
print(p1.wea)
p1.wea.fight(p1,p2)
组合:
给一个类的对象封装一个属性,这个属性是另一个类的对象
class Person:
animal = '高级动物'
soul = '有灵魂'
language = '语言'
def __init__(self, country, name, sex, age, hight):
self.country = country
self.name = name
self.sex = sex
self.age = age
self.hight = hight
def eat(self):
print('%s吃饭' % self.name)
def sleep(self):
print('睡觉')
def work(self):
print('工作')
Person.__dict__['sleep'](111)
p1 = Person('菲律宾','alex','未知',42,175)
p2 = Person('菲律宾','alex','未知',42,175)
p3 = Person('菲律宾','alex','未知',42,175)
p1.animal = '禽兽'
print(p1.animal)
print(Person.name)
p2 = Person('美国','武大','男',35,160)
查询顺序:
对象.属性 : 先从对象空间找,如果找不到,再从类空间找,再找不到,再从父类找....
类名.属性 : 先从本类空间找,如果找不到,再从父类找....
对象与对象之间是互相独立的.
计算一个类 实例化多少对象.
class Count:
count = 0
def __init__(self):
Count.count = self.count + 1
obj1 = Count()
obj2 = Count()
print(Count.count)
count = 0
def func():
print(count)
func()
class Count:
count = 0
def __init__(self):
pass
通过类名可以更改我的类中的静态变量值
Count.count = 6
print(Count.__dict__)
但是通过对象 不能改变只能引用类中的静态变量
obj1 = Count()
print(obj1.count)
obj1.count = 6