编写数据库初始化函数
package AppInit
import (
"github.com/jinzhu/gorm"
_ "github.com/jinzhu/gorm/dialects/mysql"
"log"
)
var db *gorm.DB
func init() {
var err error
db, err = gorm.Open("mysql",
"root:123456@tcp(localhost:3306)/micro?charset=utf8mb4&parseTime=True&loc=Local")
if err != nil {
log.Fatal(err)
}
db.DB().SetMaxIdleConns(10)
db.DB().SetMaxOpenConns(50)
}
func GetDB() *gorm.DB {
return db
}
编写Models.proto
syntax = "proto3";
package Services;
import "google/protobuf/timestamp.proto";
message UserModel {
int32 user_id = 1;
string user_name = 2;
string user_pwd = 3;
google.protobuf.Timestamp user_date=4;
}
编写Service.proto
syntax = "proto3";
package Services;
import "Models.proto";
message RegResponse {
string status = 1;
string message = 2;
}
service UserService{
rpc UserReg(UserModel) returns(RegResponse);
}
生成pb文件
protoc --go_out=../ Models.proto #models文件可以不加--micro_out
protoc --micro_out=../ --go_out=../ UserService.proto
protoc-go-inject-tag -input=../Models.pb.go
protoc-go-inject-tag -input=../UserService.pb.go
编写实例结构体
package ServicesImpl
import (
"context"
"micro/AppInit"
"micro/DBModels"
"micro/Services"
"time"
)
type UserService struct {
}
func (this *UserService) UserReg(ctx context.Context, req *Services.UserModel, rsp *Services.RegResponse) (err error) {
user := DBModels.Users{
UserName: req.UserName,
UserPwd: req.UserPwd,
UserDate: time.Now(),
}
if err = AppInit.GetDB().Create(&user).Error; err != nil {
rsp.Message = err.Error()
rsp.Status = "success"
}
return err
}
启动服务并且使用micro配置网关之后使用浏览器访问
package main
import (
"github.com/micro/go-micro"
"github.com/micro/go-micro/registry"
"github.com/micro/go-micro/registry/etcd"
_ "github.com/micro/go-plugins/registry/etcd"
"micro/Services"
"micro/ServicesImpl"
)
func main() {
//consulReg := consul.NewRegistry(registry.Addrs("localhost:8500"))
etcdReg := etcd.NewRegistry(registry.Addrs("106.12.72.181:23791"))
myservice := micro.NewService(
micro.Name("api.xiahualou.com.myapp"),
micro.Address(":8001"),
micro.Registry(etcdReg),
//micro.Registry(consulReg),
)
//Services.RegisterTestServiceHandler(myservice.Server(), new(ServicesImpl.TestService))
Services.RegisterUserServiceHandler(myservice.Server(), new(ServicesImpl.UserService))
myservice.Run()
}
set MICRO_REGISTRY=etcdv3
set MICRO_REGISTRY_ADDRESS=106.12.72.181:23791
set MICRO_API_NAMESPACE=api.xiahualou.com
set MICRO_API_HANDLER=rpc
micro api
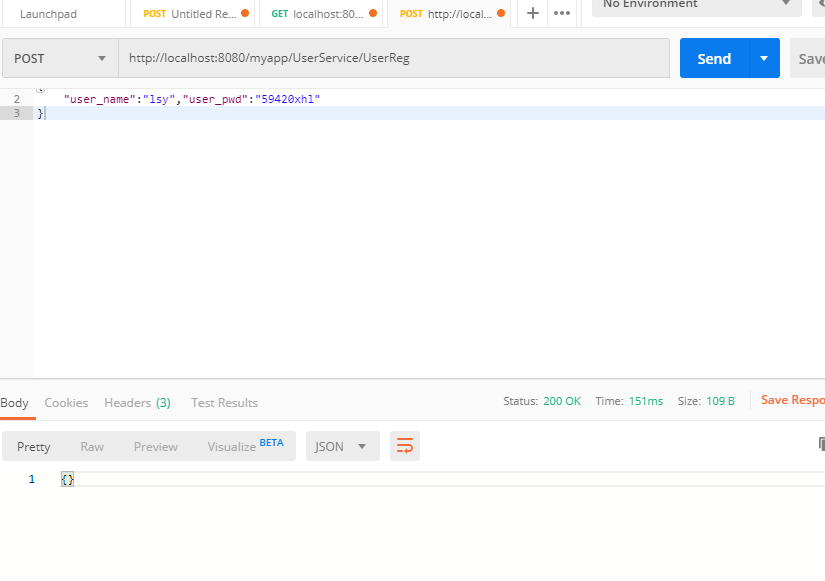