The Doors
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 8819 | Accepted: 3395 |
Description
You are to find the length of the shortest path through a chamber containing obstructing walls. The chamber will always have sides at x = 0, x = 10, y = 0, and y = 10. The initial and final points of the path are always (0, 5) and (10, 5). There will also be from 0 to 18 vertical walls inside the chamber, each with two doorways. The figure below illustrates such a chamber and also shows the path of minimal length.
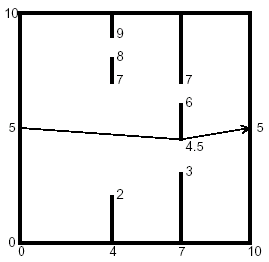
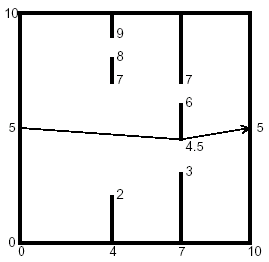
Input
The input data for the illustrated chamber would appear as follows.
2
4 2 7 8 9
7 3 4.5 6 7
The first line contains the number of interior walls. Then there is a line for each such wall, containing five real numbers. The first number is the x coordinate of the wall (0 < x < 10), and the remaining four are the y coordinates of the ends of the doorways in that wall. The x coordinates of the walls are in increasing order, and within each line the y coordinates are in increasing order. The input file will contain at least one such set of data. The end of the data comes when the number of walls is -1.
2
4 2 7 8 9
7 3 4.5 6 7
The first line contains the number of interior walls. Then there is a line for each such wall, containing five real numbers. The first number is the x coordinate of the wall (0 < x < 10), and the remaining four are the y coordinates of the ends of the doorways in that wall. The x coordinates of the walls are in increasing order, and within each line the y coordinates are in increasing order. The input file will contain at least one such set of data. The end of the data comes when the number of walls is -1.
Output
The output should contain one line of output for each chamber. The line should contain the minimal path length rounded to two decimal places past the decimal point, and always showing the two decimal places past the decimal point. The line should contain no blanks.
Sample Input
1 5 4 6 7 8 2 4 2 7 8 9 7 3 4.5 6 7 -1
Sample Output
10.00 10.06
题目大意
在一个长宽均为10,入口出口分别为(0,5)、(10,5)的房间里,有几堵墙,每堵墙上有两个缺口,求入口到出口的最短路经。
题解:
计算几何上套一个最短路,主要问题在于建图。
每加入一个点,考虑之前所有的点和它连边,判断中间是否有线段阻隔,如果有,则不能连边。
记得加入起点和终点。
最后直接输出最短路的答案就可以了。
AC代码如下:
#include<iostream> #include<cstdio> #include<cstdlib> #include<cstring> #include<cmath> #include<algorithm> #include<queue> using namespace std; int n,cnt,top; struct Vector { double x,y; Vector(){} Vector(double xx,double yy){x=xx;y=yy;} Vector operator+(Vector b) { Vector ans; ans.x=x+b.x;ans.y=y+b.y; return ans; } Vector operator-(Vector b) { Vector ans; ans.x=x-b.x;ans.y=y-b.y; return ans; } double operator*(Vector b) { return x*b.y-b.x*y; } double operator^(Vector b) { return x*b.x+y*b.y; } }p[1005]; struct node { Vector a,b; node(){} node(Vector aa,Vector bb){a=aa;b=bb;} }q[1005]; bool pd(Vector a,Vector b) { int i; for(i=1;i<=top;i++) if(q[i].a.x<b.x&&q[i].a.x>a.x) { if(((a-b)*(q[i].b-a))*((a-b)*(q[i].a-a))<0) return 0; } return 1; } struct Edge { int next,to; double dis; }edge[2005]; int head[1005],size; void putin(int from,int to,double dis) { size++; edge[size].to=to; edge[size].next=head[from]; edge[size].dis=dis; head[from]=size; } double suan(int j,int cnt) { return sqrt((p[j].x-p[cnt].x)*(p[j].x-p[cnt].x)+(p[j].y-p[cnt].y)*(p[j].y-p[cnt].y)); } double dist[1005]; bool vis[1005]; double SPFA() { int i; memset(dist,127,sizeof(dist)); memset(vis,0,sizeof(vis)); queue<int>mem; mem.push(1); vis[1]=1;dist[1]=0; while(!mem.empty()) { int x=mem.front();mem.pop(); vis[x]=0; for(i=head[x];i!=-1;i=edge[i].next) { int y=edge[i].to; if(dist[y]>dist[x]+edge[i].dis) { dist[y]=dist[x]+edge[i].dis; if(!vis[y]) { mem.push(y); vis[y]=1; } } } } return dist[cnt]; } int main() { int i,j; while(1) { scanf("%d",&n); if(n==-1)break; memset(head,-1,sizeof(head)); size=top=cnt=0; p[++cnt]=Vector(0,5); for(i=1;i<=n;i++) { double x,a,b,c,d; scanf("%lf%lf%lf%lf%lf",&x,&a,&b,&c,&d); p[++cnt]=Vector(x,a); for(j=1;j<=cnt-1;j++)if(pd(p[j],p[cnt]))putin(j,cnt,suan(j,cnt)); p[++cnt]=Vector(x,b); for(j=1;j<=cnt-1;j++)if(pd(p[j],p[cnt]))putin(j,cnt,suan(j,cnt)); p[++cnt]=Vector(x,c); for(j=1;j<=cnt-1;j++)if(pd(p[j],p[cnt]))putin(j,cnt,suan(j,cnt)); p[++cnt]=Vector(x,d); for(j=1;j<=cnt-1;j++)if(pd(p[j],p[cnt]))putin(j,cnt,suan(j,cnt)); q[++top]=node(Vector(x,0),Vector(x,a)); q[++top]=node(Vector(x,b),Vector(x,c)); q[++top]=node(Vector(x,d),Vector(x,10)); } p[++cnt]=Vector(10,5); for(j=1;j<=cnt-1;j++)if(pd(p[j],p[cnt]))putin(j,cnt,suan(j,cnt)); printf("%.2lf ",SPFA()); } return 0; }