今天完成了一个学生类和教师类其父类是一个人类
以下是实现的代码和截图
学生类代码
package a20200727;
import java.math.BigDecimal;
public class Student extends Person{
private String sNo;
private String className;
private double score;
Student(String sNo,String className,double score){
this.sNo=sNo;
this.className = className;
this.score=score;
System.out.print("Student Constructor run ");
}
Student(){
this.sNo=null;
this.className=null;
this.score=0.0;
System.out.print("Student Constructor run ");
}
Student(Student s){
this.sNo=s.sNo;
this.score=s.score;
this.className=s.className;
System.out.print("Student CopyConstructor run ");
}
public void finalize() {
System.out.print("Student Destructor run ");
}
public void setSNo(String sNo) {
this.sNo=sNo;
}
public void setClassName(String className) {
this.className=className;
}
public void setScore(double score) {
this.score=score;
}
public void setSNo() {
this.sNo=null;
}
public void setClassName() {
this.className=null;
}
public void setScore() {
this.score=0.0;
}
public String getSNo() {
return this.sNo;
}
public String getClassName() {
return this.className;
}
public double getScore() {
return this.score;
}
public void show() {
BigDecimal s1 = new BigDecimal(this.score);
double s2 = s1.setScale(0,BigDecimal.ROUND_HALF_UP).doubleValue();
System.out.print("No="+this.getNo()+",Name="+this.getName()+" "+"SNo="+this.sNo+",ClassName="+this.className+",Score="+(int)s2+" ");
}
}教师类代码
private String sNo;
private String className;
private double score;
Student(String sNo,String className,double score){
this.sNo=sNo;
this.className = className;
this.score=score;
System.out.print("Student Constructor run ");
}
Student(){
this.sNo=null;
this.className=null;
this.score=0.0;
System.out.print("Student Constructor run ");
}
Student(Student s){
this.sNo=s.sNo;
this.score=s.score;
this.className=s.className;
System.out.print("Student CopyConstructor run ");
}
public void finalize() {
System.out.print("Student Destructor run ");
}
public void setSNo(String sNo) {
this.sNo=sNo;
}
public void setClassName(String className) {
this.className=className;
}
public void setScore(double score) {
this.score=score;
}
public void setSNo() {
this.sNo=null;
}
public void setClassName() {
this.className=null;
}
public void setScore() {
this.score=0.0;
}
public String getSNo() {
return this.sNo;
}
public String getClassName() {
return this.className;
}
public double getScore() {
return this.score;
}
public void show() {
BigDecimal s1 = new BigDecimal(this.score);
double s2 = s1.setScale(0,BigDecimal.ROUND_HALF_UP).doubleValue();
System.out.print("No="+this.getNo()+",Name="+this.getName()+" "+"SNo="+this.sNo+",ClassName="+this.className+",Score="+(int)s2+" ");
}
}教师类代码
package a20200727;
import java.math.BigDecimal;
public class Teacher extends Person{
private String tNo;
private String departmrntName;
private double wages;
Teacher(String t,String d,double w){
this.tNo=t;
this.departmrntName=d;
this.wages=w;
System.out.print("Teacher Constructor run ");
}
Teacher(){
this.tNo=null;
this.departmrntName=null;
this.wages=0.0;
System.out.print("Teacher Constructor run ");
}
Teacher(Teacher t){
this.departmrntName=t.departmrntName;
this.tNo=t.tNo;
this.wages=t.wages;
System.out.print("Teacher CopyConstructor run ");
}
public void finalize() {
System.out.print("Teacher Destructor run ");
}
public void setTNo(String s) {
this.tNo=s;
}
public void setTNo() {
this.tNo=null;
}
public void setDepartmentName(String d) {
this.departmrntName=d;
}
public void setDepartmentName() {
this.departmrntName=null;
}
public void setWages(double w) {
this.wages=w;
}
public void setWages() {
this.wages=0.0;
}
public String getTNo() {
return this.tNo;
}
public String getDepartmentName() {
return this.departmrntName;
}
public double getWages() {
return this.wages;
}
public void show() {
BigDecimal s1 = new BigDecimal(this.wages);
double s2 = s1.setScale(0,BigDecimal.ROUND_HALF_UP).doubleValue();
System.out.print("No="+this.getNo()+",Name="+this.getName()+" TNo="+this.tNo+",DepartmentName="+this.departmrntName+",Wages="+(int)s2+" ");
}
}
private String tNo;
private String departmrntName;
private double wages;
Teacher(String t,String d,double w){
this.tNo=t;
this.departmrntName=d;
this.wages=w;
System.out.print("Teacher Constructor run ");
}
Teacher(){
this.tNo=null;
this.departmrntName=null;
this.wages=0.0;
System.out.print("Teacher Constructor run ");
}
Teacher(Teacher t){
this.departmrntName=t.departmrntName;
this.tNo=t.tNo;
this.wages=t.wages;
System.out.print("Teacher CopyConstructor run ");
}
public void finalize() {
System.out.print("Teacher Destructor run ");
}
public void setTNo(String s) {
this.tNo=s;
}
public void setTNo() {
this.tNo=null;
}
public void setDepartmentName(String d) {
this.departmrntName=d;
}
public void setDepartmentName() {
this.departmrntName=null;
}
public void setWages(double w) {
this.wages=w;
}
public void setWages() {
this.wages=0.0;
}
public String getTNo() {
return this.tNo;
}
public String getDepartmentName() {
return this.departmrntName;
}
public double getWages() {
return this.wages;
}
public void show() {
BigDecimal s1 = new BigDecimal(this.wages);
double s2 = s1.setScale(0,BigDecimal.ROUND_HALF_UP).doubleValue();
System.out.print("No="+this.getNo()+",Name="+this.getName()+" TNo="+this.tNo+",DepartmentName="+this.departmrntName+",Wages="+(int)s2+" ");
}
}
人类代码
package a20200727;
public class Person {
private String no;
private String name;
Person(String no,String name){
this.no=no;
this.name=name;
System.out.print("Person Constructor run ");
}
Person(){
this.no=null;
this.name=null;
System.out.print("Person Constructor run ");
}
Person(Person p){
this.no=p.no;
this.name=p.name;
System.out.print("Person CopyConstructor run ");
}
public void finalize() {
System.out.print("Person Destructor run ");
}
public void setNo(String no) {
this.no=no;
}
public void setName(String name) {
this.name=name;
}
public String getNo() {
return this.no;
}
public String getName() {
return this.name;
}
public void setNo() {
this.no=null;
}
public void setName() {
this.name=null;
}
public void show() {
System.out.print("No="+this.no+",Name="+this.name+" ");
}
}
private String no;
private String name;
Person(String no,String name){
this.no=no;
this.name=name;
System.out.print("Person Constructor run ");
}
Person(){
this.no=null;
this.name=null;
System.out.print("Person Constructor run ");
}
Person(Person p){
this.no=p.no;
this.name=p.name;
System.out.print("Person CopyConstructor run ");
}
public void finalize() {
System.out.print("Person Destructor run ");
}
public void setNo(String no) {
this.no=no;
}
public void setName(String name) {
this.name=name;
}
public String getNo() {
return this.no;
}
public String getName() {
return this.name;
}
public void setNo() {
this.no=null;
}
public void setName() {
this.name=null;
}
public void show() {
System.out.print("No="+this.no+",Name="+this.name+" ");
}
}
主函数代码
package a20200727;
import java.util.Scanner;
public class main {
public static void main(String[] arg) {
String s1="130502190001010332";
String s2="doublebest";
String s3="20181234";
String s4="铁 1801";
double value=60.67;
Student stu1=new Student(s3,s4,value);
stu1.setName(s2);
stu1.setNo(s1);
stu1.show();
Student stu2 = new Student(stu1);
stu2.setName(s2);
stu2.setNo(s1);
Scanner S3 = new Scanner(System.in);
Scanner S4 = new Scanner(System.in);
Scanner v = new Scanner(System.in);
s3 = S3.next();
s4 = S4.next();
value = v.nextDouble();
stu2.setSNo(s3);
stu2.setClassName(s4);
stu2.show();
Teacher t1 = new Teacher(s3,s4,value);
t1.setName(s2);
t1.setNo(s1);
t1.show();
Teacher t2 = new Teacher(t1);
t2.setName(s2);
t2.setNo(s1);
t2.setTNo(s3);
t2.setDepartmentName(s3);
t2.show();
stu1=null;
stu2=null;
t1=null;
t2=null;
System.gc();
}
}截图如下
public static void main(String[] arg) {
String s1="130502190001010332";
String s2="doublebest";
String s3="20181234";
String s4="铁 1801";
double value=60.67;
Student stu1=new Student(s3,s4,value);
stu1.setName(s2);
stu1.setNo(s1);
stu1.show();
Student stu2 = new Student(stu1);
stu2.setName(s2);
stu2.setNo(s1);
Scanner S3 = new Scanner(System.in);
Scanner S4 = new Scanner(System.in);
Scanner v = new Scanner(System.in);
s3 = S3.next();
s4 = S4.next();
value = v.nextDouble();
stu2.setSNo(s3);
stu2.setClassName(s4);
stu2.show();
Teacher t1 = new Teacher(s3,s4,value);
t1.setName(s2);
t1.setNo(s1);
t1.show();
Teacher t2 = new Teacher(t1);
t2.setName(s2);
t2.setNo(s1);
t2.setTNo(s3);
t2.setDepartmentName(s3);
t2.show();
stu1=null;
stu2=null;
t1=null;
t2=null;
System.gc();
}
}截图如下
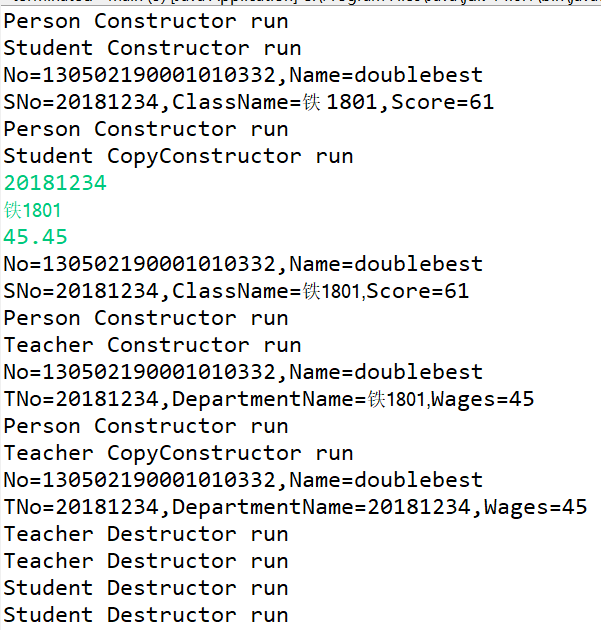