JAVA实验报告一
1.题目:定义并测试一个名为Student的类,包括的属性有“学号”、“姓名”以及三门课程“数学”、“英语”和“计算机”的成绩,包括的方法有计算三门课程的“总分”、“平均分”、“最高分”、“最低分”。
2.代码:
package Test;
public class Student {
private String stuno;
private String name;
private float math;
private float english;
private float computer;
public Student() {
super();
}
public Student(String stuno, String name, float math, float english, float computer) {
super();
this.stuno = stuno;
this.name = name;
this.math = math;
this.english = english;
this.computer = computer;
}
public float sum() {
return math + english + computer;
}
public float avg() {
return this.sum() / 3;
}
public float min() {
float min = math < english ? math : english;
return min < computer ? min : computer;
}
public float max() {
float max = math > english ? math : english;
return max > computer ? max : computer;
}
public String getStuno() {
return stuno;
}
public void setStuno(String stuno) {
this.stuno = stuno;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public float getMath() {
return math;
}
public void setMath(float math) {
this.math = math;
}
public float getEnglish() {
return english;
}
public void setEnglish(float english) {
this.english = english;
}
public float getComputer() {
return computer;
}
public void setComputer(float computer) {
this.computer = computer;
}
public void talk() {
System.out.println("我的名字叫" + name + ",我的学号是" + stuno + "。我的数学,英语,计算机三科的总分、平均分、最低以及最高分分别是:");
}
public static void main(String[] args) {
Student stu1; // 栈空间
// 实例化对象
stu1 = new Student();
stu1.setName("Holland");// 通过set和get方法访问private属性
stu1.setStuno("12345678");
stu1.talk();
Student student = new Student("Holland", "12345678", 100, 90, 85);
System.out.println(student.sum());
System.out.println(student.avg());
System.out.println(student.min());
System.out.println(student.max());
}
}
3.运行结果截图:
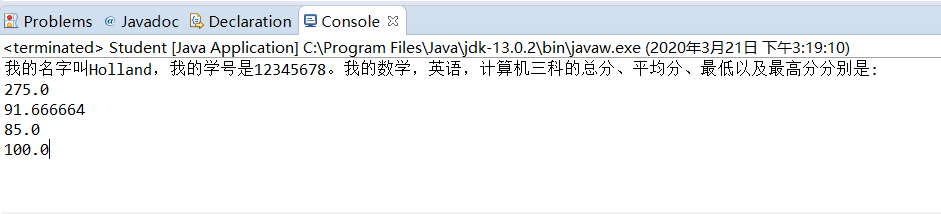
4.修改后的代码:
package Test;
public class Student {
//定义属性
private String stuno;
private String name;
private float math;
private float english;
private float computer;
//自动生成setter和getter方法
public String getStuno() {
return stuno;
}
public void setStuno(String stuno) {
this.stuno = stuno;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public float getMath() {
return math;
}
public void setMath(float math) {
this.math = math;
}
public float getEnglish() {
return english;
}
public void setEnglish(float english) {
this.english = english;
}
public float getComputer() {
return computer;
}
public void setComputer(float computer) {
this.computer = computer;
}
//构造方法
public Student(String stuno, String name, float math, float english, float computer) {
this.stuno = stuno;
this.name = name;
this.math = math;
this.english = english;
this.computer = computer;
}
//根据需求完成一些操作
public float sum() {
return math + english + computer;
}
public float avg() {
return this.sum() / 3;
}
public float min() {
float min = math < english ? math : english;
return min < computer ? min : computer;
}
public float max() {
float max = math > english ? math : english;
return max > computer ? max : computer;
}
public static void main(String[] args) {
Student student = new Student("12345678", "Holland", 100, 90, 85);
System.out.println("姓名:"+student.getName());
System.out.println("学号:"+student.getStuno());
System.out.println("总分:"+student.sum()+"分");
System.out.println("平均分:"+student.avg()+"分");
System.out.println("最低分:"+student.min()+"分");
System.out.println("最高分:"+student.max()+"分");
}
}
5.修改后代码的运行结果截图:
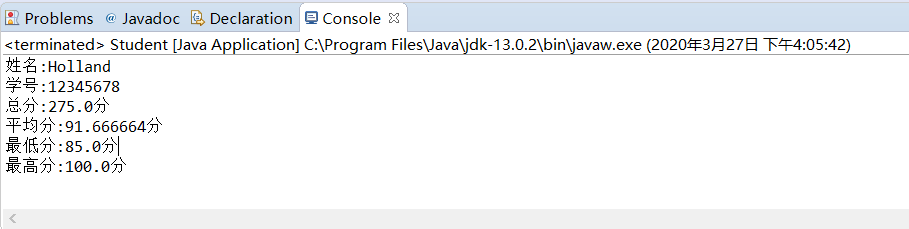