用户故事:
作为一个观众,我希望了解某一场比赛的比分,以便了解赛况。(满意条件:精确到每一局的结果比分)。
估计时间(min) | 实际时间(min) | |
计划 | 30 | 40 |
估计完成需要的时间 | 300 | 200 |
开发 | 180 | 200 |
需求分析 | 30 | 30 |
生成设计文档 | 20 | 20 |
设计复审 | 30 | 30 |
代码规范 | 20 | 20 |
具体设计 | 40 | 40 |
具体编码 | 230 | 200 |
代码复审 | 50 | 20 |
测试 | 40 | 40 |
报告 | 50 | 40 |
测试报告 | 20 | 10 |
计算工作量 | 10 | 10 |
事总结后 | 20 | 20 |
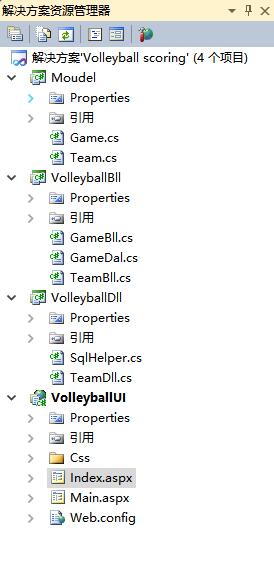
1 using System; 2 using System.Collections.Generic; 3 using System.Linq; 4 using System.Web; 5 using System.Web.UI; 6 using System.Web.UI.WebControls; 7 using VolleyballBll; 8 using Moudel; 9 10 namespace VolleyballUI 11 { 12 public partial class Index : System.Web.UI.Page 13 { 14 private TeamBll teamBll = new TeamBll(); 15 16 protected void Page_Load(object sender, EventArgs e) 17 { 18 if (!IsPostBack) 19 { 20 BindDropDownList(); 21 } 22 } 23 24 protected void btnSaveName_Click(object sender, EventArgs e) 25 { 26 Response.Write("保存事件"); 27 Team team = new Team(); 28 team.Name=TeamName.Text.Trim(); 29 if (teamBll.GetInsertTeamName(team)) 30 { 31 Response.Redirect("Index.aspx"); 32 } 33 else 34 { 35 Response.Write("<script>alert('添加失败')</script>"); 36 } 37 } 38 39 public void BindDropDownList() 40 { 41 DropDownListA.DataSource = teamBll.GetSelectAllTeams(); 42 DropDownListA.DataTextField = "Name"; 43 DropDownListA.DataValueField = "ID"; 44 DropDownListA.DataBind(); 45 DropDownListB.DataSource = teamBll.GetSelectAllTeams(); 46 DropDownListB.DataTextField = "Name"; 47 DropDownListB.DataValueField = "ID"; 48 DropDownListB.DataBind(); 49 } 50 51 protected void btnSave_Click(object sender, EventArgs e) 52 { 53 if (DropDownListA.SelectedItem.Text == DropDownListB.SelectedItem.Text) 54 { 55 Response.Write("<script>alert('同一支队伍之间不能比赛!')</script>"); 56 } 57 else 58 { 59 Response.Redirect("Main.aspx?TeamA=" + DropDownListA.SelectedItem.Text + "&TeamB=" + DropDownListB.SelectedItem.Text); 60 } 61 } 62 } 63 }
1 using System; 2 using System.Collections.Generic; 3 using System.Linq; 4 using System.Web; 5 using System.Web.UI; 6 using System.Web.UI.WebControls; 7 using Moudel; 8 using VolleyballBll; 9 10 namespace VolleyballUI 11 { 12 public partial class Main : System.Web.UI.Page 13 { 14 private Game game = new Game(); 15 16 private GameBll gamebll = new GameBll(); 17 18 protected void Page_Load(object sender, EventArgs e) 19 { 20 if (!IsPostBack) 21 { 22 TeamA.Text=Request.QueryString["TeamA"]; 23 TeamB.Text=Request.QueryString["TeamB"]; 24 Game game = new Game(); 25 game.TeamA = TeamA.Text; 26 game.TeamB = TeamB.Text; 27 if (gamebll.GetInsertGame(game)) 28 { 29 Response.Write("<script>alert('比赛开始!')</script>"); 30 } 31 } 32 } 33 34 public void Add(string ab) 35 { 36 game.PsA = Convert.ToInt32( PsA.Text); 37 game.PsB = Convert.ToInt32(PsB.Text); 38 game.ScoreA = Convert.ToInt32(ScoreA.Text); 39 game.ScoreB = Convert.ToInt32(ScoreB.Text); 40 game.PartNum = game.PsA + game.PsB; 41 if (ab == "a") 42 { 43 game.ScoreA += 1; 44 ScoreA.Text = game.ScoreA.ToString(); 45 one(); 46 Victory(); 47 } 48 else 49 { 50 game.ScoreB += 1; 51 ScoreB.Text = game.ScoreB.ToString(); 52 one(); 53 Victory(); 54 } 55 56 } 57 58 protected void AddA_Click(object sender, EventArgs e) 59 { 60 string ab = "a"; 61 Add(ab); 62 } 63 64 protected void AddB_Click(object sender, EventArgs e) 65 { 66 string ab = "b"; 67 Add(ab); 68 } 69 70 public void one() 71 { 72 if (game.PartNum < 4)//前四局的加分计算和判断。 73 { 74 if (game.ScoreA >= 24 && game.ScoreB >= 24)//当两队24分平的情况。 75 { 76 if (Math.Abs(game.ScoreA - game.ScoreB) == 2) 77 { 78 if (game.ScoreA > game.ScoreB) 79 { 80 game.PsA += 1; 81 PsA.Text = game.PsA.ToString(); 82 gamebll.GetInsertGame(game); 83 game.ScoreA = 0; 84 ScoreA.Text = game.ScoreA.ToString(); 85 game.ScoreB = 0; 86 ScoreB.Text = game.ScoreB.ToString(); 87 } 88 else 89 { 90 game.PsB += 1; 91 PsB.Text = game.PsB.ToString(); 92 gamebll.GetInsertGame(game); 93 game.ScoreA = 0; 94 ScoreA.Text = game.ScoreA.ToString(); 95 game.ScoreB = 0; 96 ScoreB.Text = game.ScoreB.ToString(); 97 } 98 } 99 } 100 else if(game.ScoreA<24||game.ScoreB<24) //当两队没有达到24分平的时候。 101 { 102 if (game.ScoreA == 25) 103 { 104 game.PsA += 1; 105 PsA.Text = game.PsA.ToString(); 106 gamebll.GetInsertGame(game); 107 game.ScoreA = 0; 108 ScoreA.Text = game.ScoreA.ToString(); 109 game.ScoreB = 0; 110 ScoreB.Text = game.ScoreB.ToString(); 111 } 112 else if (game.ScoreB == 25) 113 { 114 game.PsB += 1; 115 PsB.Text = game.PsB.ToString(); 116 gamebll.GetInsertGame(game); 117 game.ScoreA = 0; 118 ScoreA.Text = game.ScoreA.ToString(); 119 game.ScoreB = 0; 120 ScoreB.Text = game.ScoreB.ToString(); 121 } 122 } 123 } 124 else if (game.PartNum == 4)//第五局的加分计算和判断。 125 { 126 if (game.ScoreA >= 14 && game.ScoreB >= 14)//当两队24分平的情况。 127 { 128 if (Math.Abs(game.ScoreA - game.ScoreB) == 2) 129 { 130 if (game.ScoreA > game.ScoreB) 131 { 132 game.PsA += 1; 133 PsA.Text = game.PsA.ToString(); 134 gamebll.GetInsertGame(game); 135 } 136 else 137 { 138 game.PsB += 1; 139 PsB.Text = game.PsB.ToString(); 140 gamebll.GetInsertGame(game); 141 } 142 } 143 } 144 else if (game.ScoreA < 14 || game.ScoreB < 14) //当两队没有达到24分平的时候。 145 { 146 if (game.ScoreA == 15) 147 { 148 game.PsA += 1; 149 PsA.Text = game.PsA.ToString(); 150 gamebll.GetInsertGame(game); 151 } 152 else if (game.ScoreB == 15) 153 { 154 game.PsB += 1; 155 PsB.Text = game.PsB.ToString(); 156 gamebll.GetInsertGame(game); 157 } 158 } 159 } 160 else 161 { 162 Response.Write("<script>alert('比赛已结束');</script>"); 163 } 164 } 165 166 public void Victory() 167 { 168 if (game.PsA==3||game.PsB==3) 169 { 170 game.ScoreA = 0; 171 ScoreA.Text = game.ScoreA.ToString(); 172 game.ScoreB = 0; 173 ScoreB.Text = game.ScoreB.ToString(); 174 AddA.Visible = false; 175 AddB.Visible = false; 176 Response.Write("<script>alert('比赛已结束');</script>"); 177 } 178 } 179 } 180 }