版本1
主要知识点
- axios数据的获取&传送
- 数组数据(里面装对象)的显示
- axios链接中未知变量的获取方式
- 点击替换文本框中内容,形参~(那行唯一的那个变量就是形参)
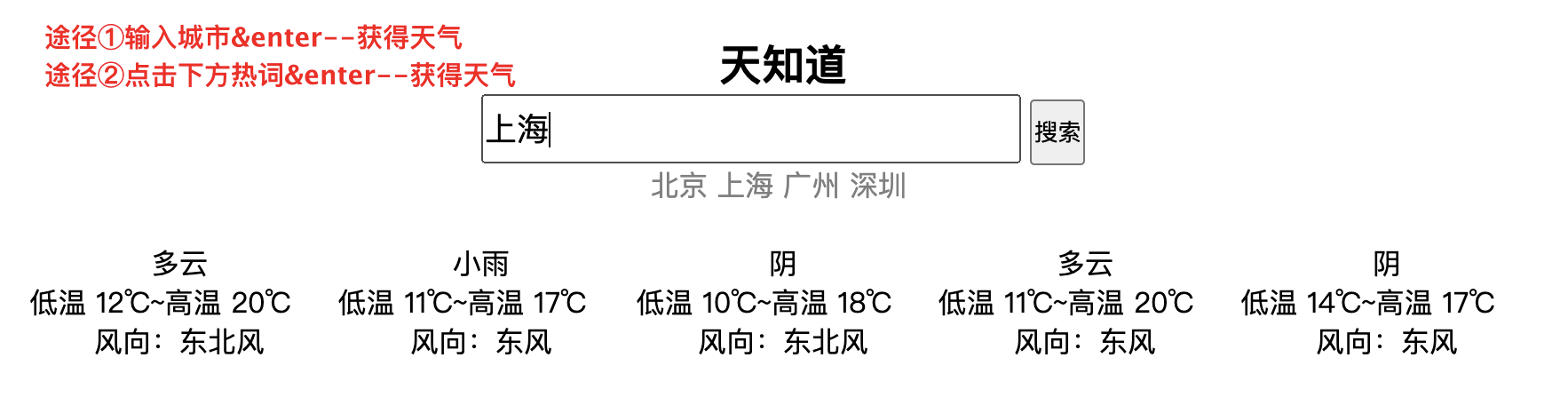
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>天气查询</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<!-- 官方axios在线地址 -->
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<!-- <script src="main.js"></script> -->
<!-- 请求地址:http://wthrcdn.etouch.cn/weather_mini
示例:http://wthrcdn.etouch.cn/weather_mini?city=深圳
请求方法:get
请求参数:city -->
<style>
* {
margin: 0;
padding: 0;
}
#app {
margin: 100px auto;
text-align: center;
}
#app ul {
margin: 0 auto;
text-align: center;
850px;
height: 80px;
}
input {
outline: none;
300px;
height: 35px;
font-size: 18px;
}
button {
height: 37px;
}
a {
text-decoration: none;
color: grey;
}
li {
float: left;
list-style: none;
170px;
height: 70px;
}
</style>
</head>
<body>
<div id="app">
<h2>天知道</h2>
<input type="text" placeholder="请输入查询的城市名称…" v-model="cityName" @keyup.enter="getCity">
<button>搜索</button>
<br>
<span>
<a href="#" v-for="aa in arr" @click="transform(aa)">{{aa}} </a>
</span>
<ul>
<li v-for="aaa in weatherCondition">
<!-- 对象的数值获取就是用.的 -->
{{aaa.data}}
<br> {{aaa.type}}
<br> {{aaa.low}}~{{aaa.high}}
<br> 风向:{{aaa.fengxiang}}
</li>
</ul>
</div>
<script>
var app = new Vue({
el: "#app",
data: {
arr: ["北京", "上海", "广州", "深圳"],
cityName: "南昌",
weatherCondition: []
},
methods: {
getCity: function() {
// cityName内容用字符串的形式传过去~~
axios.get("http://wthrcdn.etouch.cn/weather_mini?city=" + this.cityName).then((response) => {
// console.log(response.data.data.forecast);
this.weatherCondition = response.data.data.forecast;
}, (error) => {
console.log(error);
})
},
transform: function(aa) {
this.cityName = aa; //我想到了形参,我真棒hhhh
this.getCity();//直接调用函数,与下行效果相同
//axios.get("http://wthrcdn.etouch.cn/weather_mini?city=" + this.cityName).then((response) => {
this.weatherCondition = response.data.data.forecast;
})
}
}
})
</script>
</body>
</html>
版本2
简练要点
- 导入的包依旧写在html页面
- 分离:导入一下main.js就行(放在vue代码后面哩)
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>天气查询小案例</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<!-- 官方axios在线地址 -->
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<style>
* {
margin: 0;
padding: 0;
}
#app {
margin: 100px auto;
text-align: center;
}
#app input {
200px;
height: 30px;
}
#app button {
height: 32px;
}
input {
outline: none;
font-size: 15px;
}
a {
text-decoration: none;
color: grey;
}
</style>
</head>
<body>
<div id="app">
<h2>天知道</h2>
<input type="text" placeholder="请输入需要查询的城市…" v-model=cityName @keyup.enter="search">
<button>搜索</button>
<br>
<a href="#" v-for="iterms in hotwords" @click="transform(iterms)">{{iterms}} </a>
<ul>
<li v-for="ite in weatherCondition">
{{ite.data}}
<br> {{ite.type}}{{ite.fengxiang}}
<br> {{ite.low}}~{{ite.high}}
</li>
</ul>
</div>
<script src="main.js"></script>
</body>
</html>
js
// 请求地址:http://wthrcdn.etouch.cn/weather_mini
// 示例:http://wthrcdn.etouch.cn/weather_mini?city=深圳
// 请求方法:get
// 请求参数:city
var area = new Vue({
el: "#app",
data: {
cityName: "南昌",
hotwords: ["北京", "上海", "广州", "深圳"],
weatherCondition: []
},
methods: {
search: function() {
axios.get("http://wthrcdn.etouch.cn/weather_mini?city=" + this.cityName).then((response) => { this.weatherCondition = response.data.data.forecast; }, (err) => { console.log(err); });
},
transform: function(iterms) {
this.cityName = iterms;//文本框中文字自动替换
axios.get("http://wthrcdn.etouch.cn/weather_mini?city=" + this.cityName).then((response) => { this.weatherCondition = response.data.data.forecast; }, (err) => { console.log(err); });
}
}
})