使用
<CalendarMonth
default={true}
method={this.state.checkType}
room_id={this.state.roomNoValue.length > 0 && this.state.roomNoValue[0]}
setCurrent={(current) => {
this.setState({
current,
bookingButton:false
})
const currentTime = moment().format('HH:mm')
if(this.state.forbid){
this.setState({
bookingButton:false
})
return
}
this.setState({
bookingButton:this.state.checkType ===1? moment().format('YYYY-MM-DD') === current&&this.state.lastTime > currentTime? true:
( moment(moment().format('YYYY-MM-DD')).isBefore(current)?!this.state.forbid:false):
moment().format('YYYY-MM-DD') === current[0]? true:( moment(moment().format('YYYY-MM-DD')).isBefore(current[0])?true:false),
lastTime:this.state.lastTime
})
}} time={moment(this.state.date).format('YYYY-MM')}/>
详细代码
import React, {Component} from 'react';
import DateItem from "./DateItem";
import PropTypes from 'prop-types';
import moment from "moment";
import HttpUtils from "../../../utils/HttpUtils";
import {ActivityIndicator} from "antd-mobile";
// import console = require("console");
const days = [ '日', '一', '二', '三', '四', '五', '六'];
class CalendarMonth extends Component {
//method 1:按小时 2:按天
static defaultProps = {
time: moment().format('YYYY-MM'),
method: 2,
room_id: 65,
default: true
}
static propTypes = {
//YYYY-MM-DD
room_id: PropTypes.number,
time: PropTypes.string,
// time: PropTypes.,
method: PropTypes.number,
default: PropTypes.bool
}
constructor(props) {
super(props);
this.state = {
list: [],
data: [],
week: 0,
current: props.default ? moment().format('YYYY-MM-DD') : '',
}
}
getList() {
this.setState({loading: true})
HttpUtils.postForm('/api/room/order/list', {
room_id: this.props.room_id,
start_date: moment(this.props.time).date(1).format('YYYY-MM-DD'),
end_date: moment(this.props.time).date(moment().daysInMonth()).format('YYYY-MM-DD')
}).then(res => {
if (res.status === 10000) {
this.getData(res.data);
}
})
}
componentDidMount() {
if (this.props.room_id) {
this.getList();
} else {
this.getData()
}
}
componentDidUpdate(prevProps, prevState) {
if (prevProps.time !== this.props.time || prevProps.room_id !== this.props.room_id) {
this.getList();
}
}
getData = (data) => {
const day = moment(this.props.time).daysInMonth();
const list = [];
for (let i = 0; i < day; i++) {
list.push({
title: i + 1,
time: moment(this.props.time).date(i + 1).format('YYYY-MM-DD'),
count: data && data.find((item) => item.date === moment(this.props.time).date(i + 1).format('YYYY-MM-DD')) ? data.find((item) => item.date === moment(this.props.time).date(i + 1).format('YYYY-MM-DD')).count : 0
})
}
this.setState({
list,
disabled: data ? data.map((item) => item.date) : [],
week: moment(this.props.time).day(),
loading: false
})
}
render() {
const {week} = this.state;
const {method} = this.props;
return (
<div className={'month_card'} style={{position: 'relative'}}>
{this.state.loading &&
<div style={{
height: '100%',
'100%',
position: 'absolute',
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
top: 0,
left: 0,
backgroundColor: 'rgba(255,255,255,0.48)'
}}>
<ActivityIndicator size={'large'}/>
</div>
}
<div style={{filter: this.state.loading ? 'blur(3px)' : ''}}>
<div className='month_title'>
{days.map((item, index) => (
<span key={index}>{item}</span>
))}
</div>
<div style={{flex: 1}}>
<div className='month'>
{week > 0 ? days.slice(0, week).map((item, index) => (
<span className='empty' key={'month' + index}></span>
)) : null}
{this.state.list.map((item, index) => (
<div className='item' onClick={() => {
if (method === 1) {
this.setState({
current: item.time
}, () => {
this.props.setCurrent && this.props.setCurrent(item.time);
})
} else {
if (method === 2 && item.count > 0) {
return;
}
if (this.state.current && this.state.current[0] === this.state.current[1] && moment(item.time).isAfter(this.state.current[0])) {
let start = moment(this.state.current[0]);
for (let i = 0; i <= moment(item.time).diff(start, 'days'); i++) {
if (this.state.disabled.indexOf(start.add(i, 'd').format('YYYY-MM-DD')) !== -1) {
this.setState({
current: [item.time, item.time]
}, () => {
this.props.setCurrent && this.props.setCurrent(this.state.current);
})
return;
}
}
this.setState({
current: [this.state.current[0], item.time]
}, () => {
this.props.setCurrent && this.props.setCurrent(this.state.current);
})
} else {
this.setState({
current: [item.time, item.time]
}, () => {
this.props.setCurrent && this.props.setCurrent(this.state.current);
})
}
}
}}
>
<DateItem item={item} key={'month01_' + index}
disabled={ moment(this.props.time).date(index + 1).format('YYYY-MM-DD')<moment(new Date()).format('YYYY-MM-DD')?true: method === 2 ? item.count > 0 : false}
current={method === 1 ? this.state.current === item.time : this.state.current.length === 2 ? moment(item.time).isBetween(moment(this.state.current[0]).subtract(1, 'd'), moment(this.state.current[1]).add(1, 'd')) : false}/>
</div>
))}
</div>
</div>
</div>
</div>
)
}
}
export default CalendarMonth;
额外文本
import React, {Component} from 'react';
export default class DateItem extends Component {
constructor(props) {
super(props);
this.state = {
category: 0
}
}
componentDidMount() {
// this.getActivityStatus();
}
componentDidShow() {
}
render() {
const {item} = this.props;
return (
<div>
<div>
<span className={this.props.disabled?'disabled-item':this.props.current ? 'current-item' : 'inactive-item'}>{item.title}</span>
{item.count !== 0 && <span className={'book-item'}>{item.count}场</span>}
</div>
</div>
)
}
}
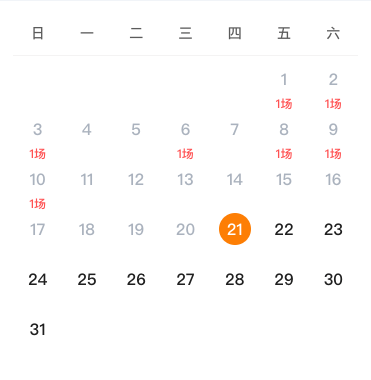