Sudoku
Time Limit: 2000MS | Memory Limit: 65536K | |||
Total Submissions: 11694 | Accepted: 5812 | Special Judge |
Description
Sudoku is a very simple task. A square table with 9 rows and 9 columns is divided to 9 smaller squares 3x3 as shown on the Figure. In some of the cells are written decimal digits from 1 to 9. The other cells are empty. The goal is to fill the empty cells with decimal digits from 1 to 9, one digit per cell, in such way that in each row, in each column and in each marked 3x3 subsquare, all the digits from 1 to 9 to appear. Write a program to solve a given Sudoku-task.
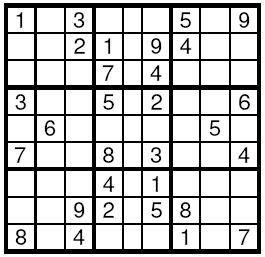
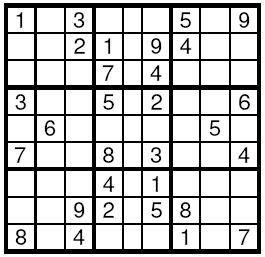
Input
The input data will start with the number of the test cases. For each test case, 9 lines follow, corresponding to the rows of the table. On each line a string of exactly 9 decimal digits is given, corresponding to the cells in this line. If a cell is empty it is represented by 0.
Output
For each test case your program should print the solution in the same format as the input data. The empty cells have to be filled according to the rules. If solutions is not unique, then the program may print any one of them.
Sample Input
1 103000509 002109400 000704000 300502006 060000050 700803004 000401000 009205800 804000107
Sample Output
143628579 572139468 986754231 391542786 468917352 725863914 237481695 619275843 854396127
Source
(转)
大致题意:
九宫格问题,也有人叫数独问题
把一个9行9列的网格,再细分为9个3*3的子网格,要求每行、每列、每个子网格内都只能使用一次1~9中的一个数字,即每行、每列、每个子网格内都不允许出现相同的数字。
0是待填位置,其他均为已填入的数字。
要求填完九宫格并输出(如果有多种结果,则只需输出其中一种)
如果给定的九宫格无法按要求填出来,则输出原来所输入的未填的九宫格
解题思路:
DFS试探,失败则回溯
用三个数组进行标记每行、每列、每个子网格已用的数字,用于剪枝
bool row[10][10]; //row[i][x] 标记在第i行中数字x是否出现了
bool col[10][10]; //col[j][y] 标记在第j列中数字y是否出现了
bool grid[10][10]; //grid[k][x] 标记在第k个3*3子格中数字z是否出现了
row 和 col的标记比较好处理,关键是找出grid子网格的序号与 行i列j的关系
即要知道第i行j列的数字是属于哪个子网格的
首先我们假设子网格的序号如下编排:
由于1<=i、j<=9,我们有: (其中“/”是C++中对整数的除法)
令a= i/3 , b= j/3 ,根据九宫格的 行列 与 子网格 的 关系,我们有:
不难发现 3a+b=k
即 3*(i/3)+j/3=k
又我在程序中使用的数组下标为 1~9,grid编号也为1~9
因此上面的关系式可变形为 3*((i-1)/3)+(j-1)/3+1=k
有了这个推导的关系式,问题的处理就变得非常简单了,直接DFS即可
#include<iostream> #include<cstdio> #include<cstring> using namespace std; int map[10][10]; bool row[10][10]; //row[i][x] 标记在第i行中数字x是否出现了 bool col[10][10]; //col[j][y] 标记在第j列中数字y是否出现了 bool grid[10][10]; //grid[k][z ] 标记在第k个3*3子格中数字z是否出现了 bool DFS(int x,int y){ if(x==10) return true; bool flag=false; if(map[x][y]!=0){ if(y==9) flag=DFS(x+1,1); else flag=DFS(x,y+1); //return flag; if(flag) //回溯 return true; else return false; }else{ int k=3*((x-1)/3)+(y-1)/3+1; for(int i=1;i<=9;i++) //枚举数字1~9填空 if(!row[x][i] && !col[y][i] && !grid[k][i]){ map[x][y]=i; row[x][i]=true; col[y][i]=true; grid[k][i]=true; if(y==9) flag=DFS(x+1,1); else flag=DFS(x,y+1); if(flag) //回溯,继续枚举 return true; else{ map[x][y]=0; row[x][i]=false; col[y][i]=false; grid[k][i]=false; } } } return false; } int main(){ //freopen("input.txt","r",stdin); int t; scanf("%d",&t); while(t--){ memset(row,false,sizeof(row)); memset(col,false,sizeof(col)); memset(grid,false,sizeof(grid)); char str[10][10]; for(int i=1;i<=9;i++){ //scanf("%s",str[i]+1); //为啥这样输入就不行了呢??纳闷ing,明天再弄吧 for(int j=1;j<=9;j++){ cin>>str[i][j]; map[i][j]=str[i][j]-'0'; if(map[i][j]){ int k=3*((i-1)/3)+(j-1)/3+1; row[i][map[i][j]]=true; col[j][map[i][j]]=true; grid[k][map[i][j]]=true; } } } DFS(1,1); for(int i=1;i<=9;i++){ for(int j=1;j<=9;j++) printf("%d",map[i][j]); printf(" "); } } return 0; }
下面这个很快:
#include <iostream> #include<cstdio> #include<cstring> using namespace std; int num,v[100][2],map[10][10]; //bool pd[10][10]; //判断输入的时候是否为零 bool judge(int x,int y,int k){ int i,j,it,jt; for(i=0;i<9;i++){ if(map[i][y]==k) return false; if(map[x][i]==k) return false; } it=(x/3)*3; jt=(y/3)*3; for(i=0;i<3;i++) for(j=0;j<3;j++) if(map[i+it][j+jt]==k) return false; return true; } int dfs(int cap){ int i,x,y; if(cap<0) return 1; for(i=1;i<=9;i++){ x=v[cap][0]; y=v[cap][1]; if(judge(x,y,i)){ map[x][y]=i; if(dfs(cap-1)) return 1; map[x][y]=0; } } return 0; } int main(){ int t,i,j; char c; scanf("%d ",&t); while(t--){ num=0; for(i=0;i<9;i++,getchar()) for(j=0;j<9;j++){ scanf("%c",&c); map[i][j]=c-'0'; if(map[i][j]==0){ //将为空的点的坐标全部记录下来,等下需要用暴力解决 v[num][0]=i; v[num++][1]=j; } } dfs(num-1); for(i=0;i<9;i++){ for(j=0;j<9;j++) printf("%d",map[i][j]); printf(" "); } } return 0; }