结构体构建
type PlansApproval struct {
ID uint
Plans_Id int //plans编号
UpdateUser int //更新者
Approval string //批示内容
Record_date time.Time
CreateUser int
ProfileID int
Profile Profile `gorm:"ForeignKey:ProfileID;AssociationForeignKey:ID;"`
PlansApprovalsReply []PlansApprovalsReply `gorm:"ForeignKey:ID;AssociationForeignKey:ID;"`
}
type PlansApprovalsReply struct {
ID int
Plans_id int
Plans_approvals_reply_content string
Plans_approvals_reply_date time.Time
Plans_approvals_reply_user int
User User `gorm:"ForeignKey:Plans_approvals_reply_user;AssociationForeignKey:ID"`
}
type PlansComment struct {
ID uint
Plans_Id int //plans编号
CreateUser int
UpdateUser int //更新者
Record_date time.Time
Comment string //评论内容
ProfileID int
Profile Profile `gorm:"ForeignKey:ProfileID;AssociationForeignKey:ID"`
User User `gorm:"ForeignKey:ProfileID;AssociationForeignKey:ID"`
PlansCommentsReply []PlansCommentsReply `gorm:"ForeignKey:ID;AssociationForeignKey:ID;"`
}
type PlansCommentsReply struct {
ID int
Plans_id uint
Plans_comment_reply_content string
Plans_comment_reply_date time.Time
Plans_comment_reply_user int
User User `gorm:"ForeignKey:Plans_comment_reply_user;AssociationForeignKey:ID"`
}
type User struct {
gorm.Model
Username string
Avatar string `gorm:"default:'/img/user.jpg'"`
Profile_id int
}
type Profile struct {
gorm.Model
Realname string `form:"Realname" json:"Realname"`
Sex int `form:"Sex" json:"Sex,string"`
Birth time.Time `form:"Birth" json:"Birth" time_format:"2006-01-02"`
Email string `form:"Email" json:"Email"`
Webchat string `form:"Webchat" json:"Webchat"`
Qq string `form:"Qq" json:"Qq"`
Phone string `form:"Phone" json:"Phone"`
Tel string `form:"Tel" json:"Tel"`
Address string `form:"Address" json:"Address"`
Emercontact string `form:"Emercontact" json:"Emercontact"`
Emerphone string `form:"Emerphone" json:"Emerphone"`
Departid int64
Positionid int64
Lognum int
Ip string
Lasted int64
Depart Depart `gorm:"ForeignKey:Departid;AssociationForeignKey:ID"`
User User `gorm:"ForeignKey:ID;AssociationForeignKey:ID;"`
}
type Depart struct {
ID int
Name string
Desc string
}
预加载
func GetPlansCommentByPlansidList(p PlansComment, q util.Query) (results map[string]interface{}, err error) {
results = make(map[string]interface{})
var pc []PlansComment
pc = make([]PlansComment, 0)
var total int
err = db.Orm.Debug().Preload("Profile.Depart").Preload("PlansCommentsReply.User").Preload("Profile.User").First(&pc, p.CreateUser).Where("plans_id = ?", p.Plans_Id).Find(&pc).Error
db.Orm.Table("plans_comments").Where("plans_id = ?", p.Plans_Id).Where("deleted_at IS NULL").Count(&total)
results["items"] = pc
results["total"] = total
return results, err
}
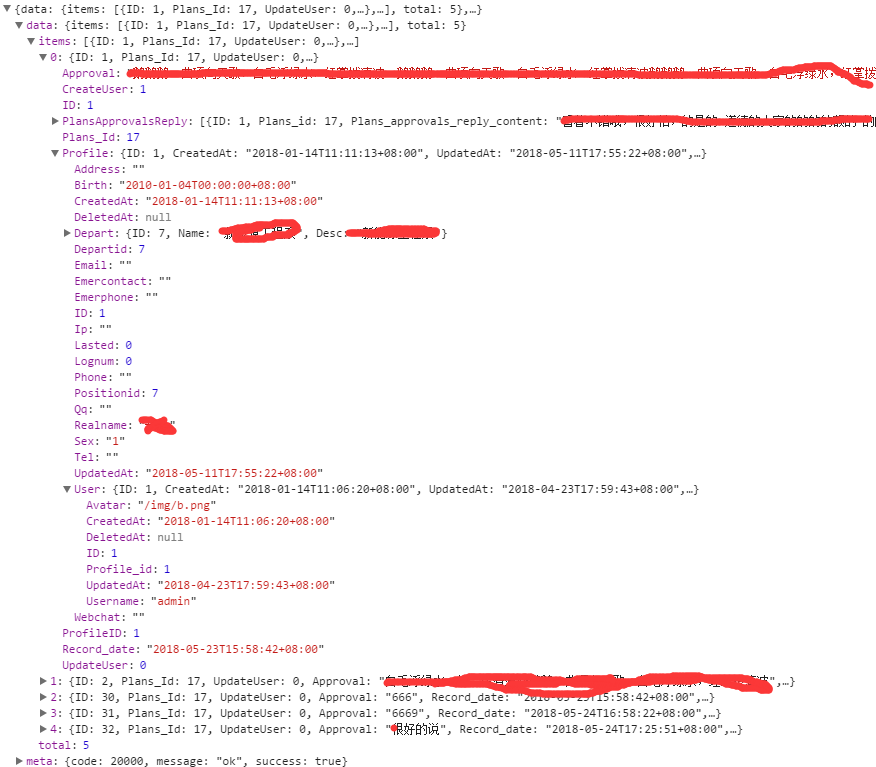