AliasRegistry
1 //AliasRegistry 2 3 4 5 package org.springframework.core; 6 7 /** 8 * Common interface for managing aliases. Serves as super-interface for 9 * {@link org.springframework.beans.factory.support.BeanDefinitionRegistry}. 10 * 11 * @author Juergen Hoeller 12 * @since 2.5.2 13 */ 14 15 /** 16 17 注册,移除,判断, 获取 18 **/ 19 20 21 public interface AliasRegistry { 22 23 /** 24 * Given a name, register an alias for it. 25 * @param name the canonical name 26 * @param alias the alias to be registered 27 * @throws IllegalStateException if the alias is already in use 28 * and may not be overridden 29 */ 30 // 31 void registerAlias(String name, String alias); 32 33 /** 34 * Remove the specified alias from this registry. 35 * @param alias the alias to remove 36 * @throws IllegalStateException if no such alias was found 37 */ 38 void removeAlias(String alias); 39 40 /** 41 * Determine whether this given name is defines as an alias 42 * (as opposed to the name of an actually registered component). 43 * @param name the name to check 44 * @return whether the given name is an alias 45 */ 46 boolean isAlias(String name); 47 48 /** 49 * Return the aliases for the given name, if defined. 50 * @param name the name to check for aliases 51 * @return the aliases, or an empty array if none 52 */ 53 String[] getAliases(String name); 54 55 }
SimpleAliasRegistry
//SimpleAliasRegistry package org.springframework.core; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; import java.util.concurrent.ConcurrentHashMap; import org.springframework.util.Assert; import org.springframework.util.StringUtils; import org.springframework.util.StringValueResolver; /** * Simple implementation of the {@link AliasRegistry} interface. * Serves as base class for * {@link org.springframework.beans.factory.support.BeanDefinitionRegistry} * implementations. * * @author Juergen Hoeller * @since 2.5.2 */ /** 实现了父接口中方法,并且增加了 **/ public class SimpleAliasRegistry implements AliasRegistry { //缓存别名和类名的地方 /** Map from alias to canonical name */ private final Map<String, String> aliasMap = new ConcurrentHashMap<String, String>(16); //注册的过程 检测-----》类名和别名是不是空的----->判断是否相等,,相等就移除--->否则使用别名获取实际名,存在---->直接结束 @Override public void registerAlias(String name, String alias) { Assert.hasText(name, "'name' must not be empty"); Assert.hasText(alias, "'alias' must not be empty"); if (alias.equals(name)) { this.aliasMap.remove(alias); } else { String registeredName = this.aliasMap.get(alias); if (registeredName != null) { if (registeredName.equals(name)) { // An existing alias - no need to re-register return; } if (!allowAliasOverriding()) { throw new IllegalStateException("Cannot register alias '" + alias + "' for name '" + name + "': It is already registered for name '" + registeredName + "'."); } } checkForAliasCircle(name, alias); this.aliasMap.put(alias, name); } } /** * Return whether alias overriding is allowed. * Default is {@code true}. */ protected boolean allowAliasOverriding() { return true; } /** * Determine whether the given name has the given alias registered. * @param name the name to check * @param alias the alias to look for * @since 4.2.1 */ public boolean hasAlias(String name, String alias) { for (Map.Entry<String, String> entry : this.aliasMap.entrySet()) { String registeredName = entry.getValue(); if (registeredName.equals(name)) { String registeredAlias = entry.getKey(); return (registeredAlias.equals(alias) || hasAlias(registeredAlias, alias)); } } return false; } @Override public void removeAlias(String alias) { String name = this.aliasMap.remove(alias); if (name == null) { throw new IllegalStateException("No alias '" + alias + "' registered"); } } @Override public boolean isAlias(String name) { return this.aliasMap.containsKey(name); } @Override public String[] getAliases(String name) { List<String> result = new ArrayList<String>(); synchronized (this.aliasMap) { retrieveAliases(name, result); } return StringUtils.toStringArray(result); } /** * Transitively retrieve all aliases for the given name. * @param name the target name to find aliases for * @param result the resulting aliases list */ private void retrieveAliases(String name, List<String> result) { for (Map.Entry<String, String> entry : this.aliasMap.entrySet()) { String registeredName = entry.getValue(); if (registeredName.equals(name)) { String alias = entry.getKey(); result.add(alias); retrieveAliases(alias, result); } } } /** * Resolve all alias target names and aliases registered in this * factory, applying the given StringValueResolver to them. * <p>The value resolver may for example resolve placeholders * in target bean names and even in alias names. * @param valueResolver the StringValueResolver to apply */ //批量检验别名 public void resolveAliases(StringValueResolver valueResolver) { Assert.notNull(valueResolver, "StringValueResolver must not be null"); synchronized (this.aliasMap) { Map<String, String> aliasCopy = new HashMap<String, String>(this.aliasMap); for (String alias : aliasCopy.keySet()) { String registeredName = aliasCopy.get(alias); String resolvedAlias = valueResolver.resolveStringValue(alias); String resolvedName = valueResolver.resolveStringValue(registeredName); if (resolvedAlias == null || resolvedName == null || resolvedAlias.equals(resolvedName)) { this.aliasMap.remove(alias); } else if (!resolvedAlias.equals(alias)) { String existingName = this.aliasMap.get(resolvedAlias); if (existingName != null) { if (existingName.equals(resolvedName)) { // Pointing to existing alias - just remove placeholder this.aliasMap.remove(alias); break; } throw new IllegalStateException( "Cannot register resolved alias '" + resolvedAlias + "' (original: '" + alias + "') for name '" + resolvedName + "': It is already registered for name '" + registeredName + "'."); } checkForAliasCircle(resolvedName, resolvedAlias); this.aliasMap.remove(alias); this.aliasMap.put(resolvedAlias, resolvedName); } else if (!registeredName.equals(resolvedName)) { this.aliasMap.put(alias, resolvedName); } } } } /** * Check whether the given name points back to the given alias as an alias * in the other direction already, catching a circular reference upfront * and throwing a corresponding IllegalStateException. * @param name the candidate name * @param alias the candidate alias * @see #registerAlias * @see #hasAlias */ //检查别名是否存在 protected void checkForAliasCircle(String name, String alias) { if (hasAlias(alias, name)) { throw new IllegalStateException("Cannot register alias '" + alias + "' for name '" + name + "': Circular reference - '" + name + "' is a direct or indirect alias for '" + alias + "' already"); } } /** * Determine the raw name, resolving aliases to canonical names. * @param name the user-specified name * @return the transformed name */ //查找别名对呀的原始类名 public String canonicalName(String name) { String canonicalName = name; // Handle aliasing... String resolvedName; do { resolvedName = this.aliasMap.get(canonicalName); if (resolvedName != null) { canonicalName = resolvedName; } } while (resolvedName != null); return canonicalName; } }
实现类:
registerAlias(String name, String alias) -----注册别名的方法,其实这个注册完的别名是放在了一个Map中
注册的过程:这个图是我从别的地方看到的,没画,其实可以看代码,很明了
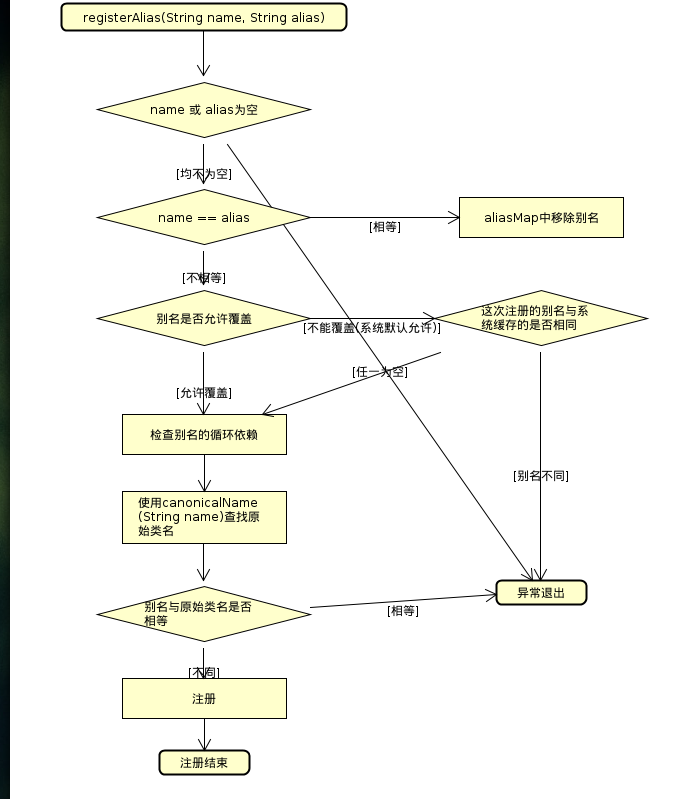
方法:public String[] getAliases(String name)
这个方法是获取别名的,其中使用了递归调用,使用了同步锁,
@Override public String[] getAliases(String name) { List<String> result = new ArrayList<String>(); synchronized (this.aliasMap) { retrieveAliases(name, result); } return StringUtils.toStringArray(result); }
递归部分:
private void retrieveAliases(String name, List<String> result) { for (Map.Entry<String, String> entry : this.aliasMap.entrySet()) { String registeredName = entry.getValue(); if (registeredName.equals(name)) { String alias = entry.getKey(); result.add(alias); retrieveAliases(alias, result); } } }