设计模式-适配器模式(adapter)
适配器模式定义
将两个不兼容的类合在一起使用,属于结构型模式,需要有Adaptee(被适配者)和Adapter(适配器)两个身份。为何使用适配器模式
经常碰到要将两个没有关系的类组合在一起使用,第一解决方案是:修改各自类的接口,但是我们没有代码,或者不愿意为一个应用修改各自的接口。那么这种Case下,Adapter可以很好的解决这个问题。使用这两个接口的合体。
如何使用适配器模式
适配器模式有类的适配器模式和对象的适配器模式两种不同的形式。
对象的适配器模式:
结构图:
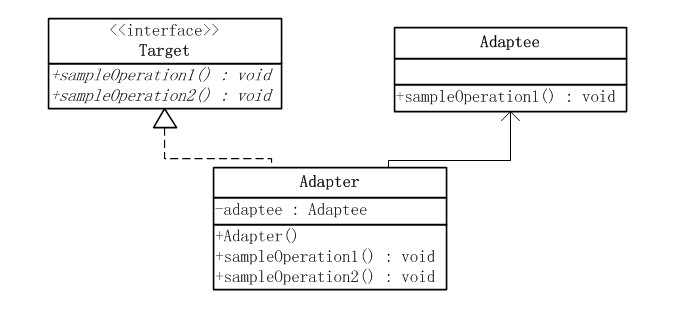
上图中Adaptee中没有sampleOperation2()这个方法,然而客户端需要这个方法。为了使Adaptee能够使用这个方法,提供一个中间环节,既Adapter,把Adaptee和Target连接起来。把Adaptee委派给adapter
Target:
package com.designpatten.adapter.object; public interface Target { void sampleOperation1() ; void sampleOperation2() ; }
Adaptee:被适配者
package com.designpatten.adapter.object; public class Adaptee { public void sampleOperation1(){ System.out.println("Adaptee ---> sampleOperation1()"); } }Adapter:适配器
package com.designpatten.adapter.object; public class Adapter implements Target { private Adaptee adaptee ; @Override public void sampleOperation2() { //实现Adaptee需要做的工作 System.out.println("object.Adapter-------->sampleOperation2"); adaptee.sampleOperation1() ; } @Override public void sampleOperation1() { // TODO Auto-generated method stub } public void setAdaptee( Adaptee adaptee ){ this.adaptee = adaptee; } }Client:
package com.designpatten.adapter.object; public class Client { public static void main(String[] args) { Adapter adapter = new Adapter() ; adapter.setAdaptee(new Adaptee()) ; adapter.sampleOperation2() ; } }
类的适配器模式:
结构图:
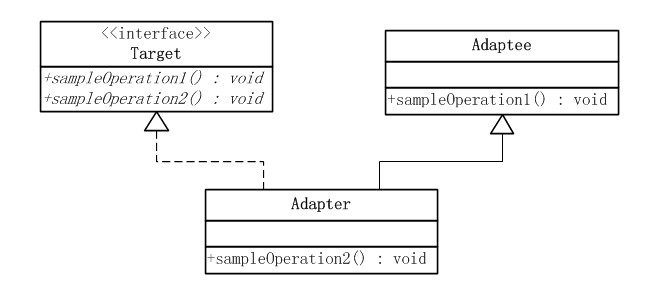
上图中Adaptee中没有sampleOperation2()这个方法,然而客户端需要这个方法。为了使Adaptee能够使用这个方法,提供一个中间环节,既Adapter,把Adaptee和Target连接起来。Adapter继承Adaptee.
Target:
package com.designpatten.adapter.classes; public interface Target { void sampleOperation1() ; void sampleOperation2() ; }
Adaptee:被适配者
package com.designpatten.adapter.classes; public class Adaptee { public void sampleOperation1(){ System.out.println("Adaptee ---> sampleOperation1()"); } }
Adapter:适配器
package com.designpatten.adapter.classes; public class Adapter extends Adaptee implements Target { @Override public void sampleOperation2() { System.out.println("classes.Adapter--------------->sampleOperation2"); } }
Client:
package com.designpatten.adapter.classes; public class Client { public static void main(String[] args) { Adapter adapter = new Adapter() ; adapter.sampleOperation2() ; } }
类适配器和对象适配器的权衡
对象适配器: 1. 使用组合的方式,是动态的方式
2. 一个适配器可以把多种不同的源适配到同一个目标。换言之,同一个适配器可一个把源类和它的子类都适配到目标接口。因为对象适配器采用对象组合的关系,只要对象类正确,是不是子类都无所谓。
3. 要重新定义Adaptee的行为比较困难,这种情况下,需要定义Adaptee的子类来实现重定义,然后让适配器组合子类。虽然重定义Adaptee的行为比较困难,但是要增加一些新的行为则很方便,而且新增加的行为可以同时适于所有的源。
类适配器:
1. 使用的是继承的方式,是静态的方式
2. 由于适配器直接继承了Adaptee,使得适配器不能和Adaptee的子类一起工作,因为继承是静态的关系,当适配器继承了Adaptee后,就不可能在去处理Adaptee的子类了。
3. 适配器可以重新定义Adaptee的部分行为,相当于子类覆盖父类的部分实现方法。
建议尽量使用对象适配器的实现方式,多用合成/聚合、少用继承。当然,具体问题具体分析,根据需要来选用实现方式,最适合的才是最好的。
适配器模式的优缺点
优点: 1. 更好的复用性
系统需要使用现有的类,而此类的接口不符合系统的需要。那么通过适配器模式就可以让这些功能得到更好的复用。
2. 更好的扩展性
在实现适配器功能的时候,可以调用自己开发的功能,从而自然地扩展系统的功能。
缺点:
过多的使用适配器,会让系统非常零乱,不易整体进行把握。比如,明明看到调用的是A接口,其实内部被适配成了B接口的实现,一个系统如果太多出现这种情况,无异于一场灾难。因此如果不是很有必要,可以不使用适配器,而是直接对系统进行重构。
参考: