http://acm.hdu.edu.cn/showproblem.php?pid=1828
Time Limit: 6000/2000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Problem Description
A number of rectangular posters, photographs and other pictures of the same shape are pasted on a wall. Their sides are all vertical or horizontal. Each rectangle can be partially or totally covered by the others. The length of the boundary of the union of all rectangles is called the perimeter.
Write a program to calculate the perimeter. An example with 7 rectangles is shown in Figure 1.
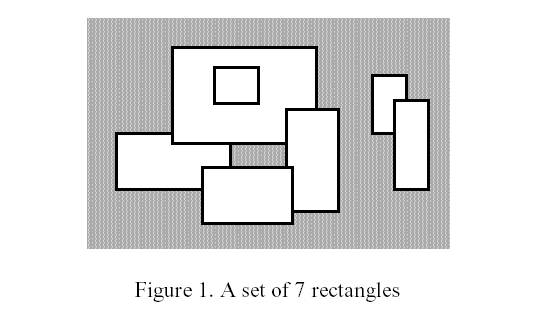
The corresponding boundary is the whole set of line segments drawn in Figure 2.
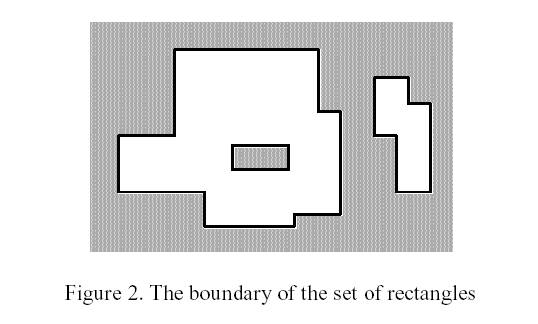
The vertices of all rectangles have integer coordinates.
Write a program to calculate the perimeter. An example with 7 rectangles is shown in Figure 1.
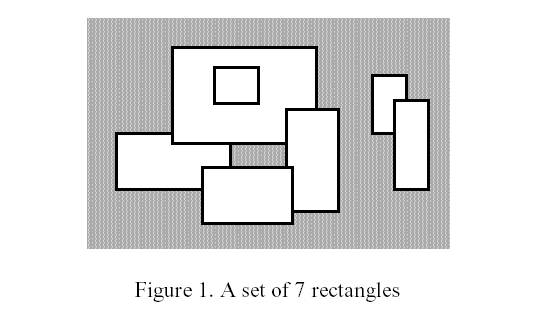
The corresponding boundary is the whole set of line segments drawn in Figure 2.
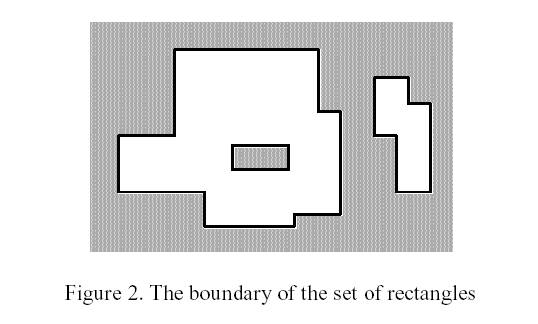
The vertices of all rectangles have integer coordinates.
Input
Your program is to read from standard input. The first line contains the number of rectangles pasted on the wall. In each of the subsequent lines, one can find the integer coordinates of the lower left vertex and the upper right vertex of each rectangle. The values of those coordinates are given as ordered pairs consisting of an x-coordinate followed by a y-coordinate.
0 <= number of rectangles < 5000
All coordinates are in the range [-10000,10000] and any existing rectangle has a positive area.
Please process to the end of file.
0 <= number of rectangles < 5000
All coordinates are in the range [-10000,10000] and any existing rectangle has a positive area.
Please process to the end of file.
Output
Your program is to write to standard output. The output must contain a single line with a non-negative integer which corresponds to the perimeter for the input rectangles.
Sample Input
7 -15 0 5 10 -5 8 20 25 15 -4 24 14 0 -6 16 4 2 15 10 22 30 10 36 20 34 0 40 16
Sample Output
228
题意:
有多个矩形,矩形的两边平行于坐标轴,这些矩形之间可能存在相互覆盖,求周长。
思路:
记录每个矩形的两条竖边(x1,y1,y2)和(x2,y1,y2),将所有的竖边按照x从小到大排序,然后一条一条竖边开始计算周长,那么以竖边所在的垂直于x轴的直线即是扫描线。
每次移动到一条新的竖边的时候,我们需要计算所在竖边扫描线上有用边长(即当前竖边的有用部分,可能当前的竖边被覆盖部分),以及加上当前扫描线与上一条扫描线之前的横边长 * 横边条数,
一直计算到最后一条竖边,即是完整周长了。
用一次扫描线,离散y坐标,按x从左到右扫描,统计每次总和的更改值,这样可以得到所有纵向边的和,对于横向边,可以用(Line[i].x - Line[i-1].x)*SegTree[1].num*2.前面的(Line[i].x - Line[i-1].x)相邻的两条线
段的x坐标的差,SegTree[1].num代表此时在线段树中一共有几条线段,每一条线段,就会增加这条线段的两个端点带来的横边。所以只要统计到当时有多少段覆盖的边,就可以得到那一段的横向的增加值
统计某一时刻有多少线段覆盖,可以用lf , rf记录这一个节点的两个端点是不是已经覆盖,如果覆盖值为1,那么这一段的num就是1,合并两个节点的时候,父节点的num等于左右子节点的num和,如果左节点
的rf与右节点的lf都是1,那么父节点的num值减去1。最后得到统计整个线段是由几个线段组成。
代码如下:
1 #include <stdio.h> 2 #include <string.h> 3 #include <iostream> 4 #include <string> 5 #include <math.h> 6 #include <algorithm> 7 #include <vector> 8 #include <queue> 9 #include <set> 10 #include <stack> 11 #include <map> 12 #include <math.h> 13 const int INF=0x3f3f3f3f; 14 typedef long long LL; 15 const int mod=1e9+7; 16 const int maxn=1e5+10; 17 using namespace std; 18 19 const int N=5005; 20 struct Line_node 21 { 22 int x;//横坐标 23 int y1,y2;//矩形纵向线段的左右端点 24 int flag;//标记是入边还是出边 25 bool operator < (const Line_node &s) 26 { 27 if(x==s.x) 28 return flag>s.flag; 29 else 30 return x<s.x; 31 } 32 }Line[N*2]; 33 34 struct SegTree_node 35 { 36 int l; 37 int r; 38 bool lf,rf;//左右边界点是否被覆盖; 39 int cover_len; 40 int cover_num; 41 int num;//矩形数目 42 }SegTree[maxn<<2]; 43 44 vector<int> vt; 45 46 void Build(int l,int r,int rt) 47 { 48 SegTree[rt].l=l; 49 SegTree[rt].r=r; 50 SegTree[rt].cover_len=0; 51 SegTree[rt].cover_num=0; 52 SegTree[rt].num=0; 53 SegTree[rt].lf=SegTree[rt].rf=false; 54 if(l+1==r) 55 return ; 56 int mid=(l+r)>>1; 57 Build(l,mid,rt<<1); 58 Build(mid,r,rt<<1|1); 59 } 60 61 void PushUp(int rt) 62 { 63 int l=SegTree[rt].l; 64 int r=SegTree[rt].r; 65 if(SegTree[rt].cover_num>0) 66 { 67 SegTree[rt].cover_len=vt[r]-vt[l]; 68 SegTree[rt].lf=SegTree[rt].rf=true; 69 SegTree[rt].num=1; 70 return ; 71 } 72 // if(l+1==r) 73 // { 74 // SegTree[rt].cover_len=0; 75 // SegTree[rt].lf=SegTree[rt].rf=false; 76 // SegTree[rt].num=0; 77 // return ; 78 // } 79 SegTree[rt].cover_len=SegTree[rt<<1].cover_len+SegTree[rt<<1|1].cover_len; 80 SegTree[rt].num=SegTree[rt<<1].num+SegTree[rt<<1|1].num-(SegTree[rt<<1].rf & SegTree[rt<<1|1].lf);//&按位与 81 SegTree[rt].lf=SegTree[rt<<1].lf; 82 SegTree[rt].rf=SegTree[rt<<1|1].rf; 83 } 84 85 void Update(Line_node t,int rt) 86 { 87 int l=SegTree[rt].l; 88 int r=SegTree[rt].r; 89 if(t.y1<=vt[l]&&t.y2>=vt[r]) 90 { 91 SegTree[rt].cover_num+=t.flag; 92 PushUp(rt); 93 return ; 94 } 95 int mid=(l+r)>>1; 96 if(t.y1<vt[mid]) 97 Update(t,rt<<1); 98 if(t.y2>vt[mid]) 99 Update(t,rt<<1|1); 100 PushUp(rt); 101 } 102 103 int main() 104 { 105 int n; 106 while (~scanf("%d",&n)) 107 { 108 vt.clear(); 109 for(int i=0;i<n;i++) 110 { 111 int x1,x2,y1,y2; 112 scanf("%d %d %d %d",&x1,&y1,&x2,&y2); 113 Line[i*2].x=x1; 114 Line[i*2].y1=y1; 115 Line[i*2].y2=y2; 116 Line[i*2].flag=1; 117 118 Line[i*2+1].x=x2; 119 Line[i*2+1].y1=y1; 120 Line[i*2+1].y2=y2; 121 Line[i*2+1].flag=-1; 122 vt.push_back(y1); 123 vt.push_back(y2); 124 } 125 sort(Line,Line+2*n); 126 //y坐标离散化 127 sort(vt.begin(),vt.end()); 128 int num=unique(vt.begin(),vt.end())-vt.begin();//去重并求出离散完的个数 129 Build(0,num-1,1); 130 int ans=0;//存累计面积 131 int prelen=0;//前一个L值,刚开始是0 132 for(int i=0;i<n*2;i++) 133 { 134 if(i>0) 135 {//SegTree[1].num代表目前线分成了几段,每段两个点,每个点一条横变 136 ans+=SegTree[1].num*2*(Line[i].x-Line[i-1].x);//先加横边 137 } 138 Update(Line[i],1);//更新线段树中维护的线 139 ans+=abs(SegTree[1].cover_len-prelen);//再加维护的线长度的变化值 140 prelen=SegTree[1].cover_len; 141 } 142 printf("%d ",ans); 143 } 144 return 0; 145 }