Rick and his co-workers have made a new radioactive formula and a lot of bad guys are after them. So Rick wants to give his legacy to Morty before bad guys catch them.
There are n planets in their universe numbered from 1 to n. Rick is in planet number s (the earth) and he doesn't know where Morty is. As we all know, Rick owns a portal gun. With this gun he can open one-way portal from a planet he is in to any other planet (including that planet). But there are limits on this gun because he's still using its free trial.
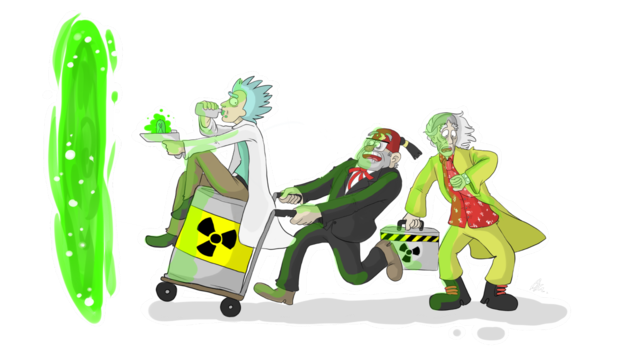
By default he can not open any portal by this gun. There are q plans in the website that sells these guns. Every time you purchase a plan you can only use it once but you can purchase it again if you want to use it more.
Plans on the website have three types:
- With a plan of this type you can open a portal from planet v to planet u.
- With a plan of this type you can open a portal from planet v to any planet with index in range [l, r].
- With a plan of this type you can open a portal from any planet with index in range [l, r] to planet v.
Rick doesn't known where Morty is, but Unity is going to inform him and he wants to be prepared for when he finds and start his journey immediately. So for each planet (including earth itself) he wants to know the minimum amount of money he needs to get from earth to that planet.
The first line of input contains three integers n, q and s (1 ≤ n, q ≤ 105, 1 ≤ s ≤ n) — number of planets, number of plans and index of earth respectively.
The next q lines contain the plans. Each line starts with a number t, type of that plan (1 ≤ t ≤ 3). If t = 1 then it is followed by three integers v, u and w where w is the cost of that plan (1 ≤ v, u ≤ n, 1 ≤ w ≤ 109). Otherwise it is followed by four integers v, l, r and w where w is the cost of that plan (1 ≤ v ≤ n, 1 ≤ l ≤ r ≤ n, 1 ≤ w ≤ 109).
In the first and only line of output print n integers separated by spaces. i-th of them should be minimum money to get from earth to i-th planet, or - 1 if it's impossible to get to that planet.
3 5 1
2 3 2 3 17
2 3 2 2 16
2 2 2 3 3
3 3 1 1 12
1 3 3 17
0 28 12
4 3 1
3 4 1 3 12
2 2 3 4 10
1 2 4 16
0 -1 -1 12
In the first sample testcase, Rick can purchase 4th plan once and then 2nd plan in order to get to get to planet number 2.
【分析】在一条数轴上,某人初始位置在s点,它有三种功能枪,第一种就是 从u点开一条到v点的即时通道,第二种是开一条从u到[l,r]区间任一点的通道,
第三种是开一条从[l,r]区间任一点到v的通道,所有通道都是单向的即时的,而且一种枪买来只能用一次,用完了还想使用的话就得花钱买,然后问从s点到其他各点
的最小花费。
很明显这是个最短路。问题是从一个点到区间,需要将区间所有的点都建边,这样指定超时。看到区间,于是我们想到线段树。。。对于每个区间我们找到它对应的几个根节点,然后建边,注意,这里要建两棵树分别代表 左端点和右端点,然后就是dij了。
#include <cstdio> #include <map> #include <algorithm> #include <vector> #include <iostream> #include <set> #include <queue> #include <string> #include <cstdlib> #include <cstring> #include <cmath> using namespace std; typedef pair<int,int>pii; typedef long long LL; const int N=6e6+5; const int mod=1e9+7; int n,m,s,cnt,idl[N<<1],idr[N<<1]; bool vis[N]; LL d[N]; vector<pii>edg[N]; void buildl(int rt,int l,int r) { idl[rt]=++cnt; if(l==r)return ; int m=l+r>>1; buildl(rt<<1,l,m); buildl(rt<<1|1,m+1,r); edg[idl[rt<<1]].push_back(make_pair(idl[rt],0)); edg[idl[rt<<1|1]].push_back(make_pair(idl[rt],0)); } void buildr(int rt,int l,int r) { idr[rt]=++cnt; if(l==r)return ; int m=l+r>>1; buildr(rt<<1,l,m); buildr(rt<<1|1,m+1,r); edg[idr[rt]].push_back(make_pair(idr[rt<<1],0)); edg[idr[rt]].push_back(make_pair(idr[rt<<1|1],0)); } void pre(int rt,int l,int r) { if(l==r) { edg[l].push_back(make_pair(idl[rt],0)); edg[idr[rt]].push_back(make_pair(l,0)); return; } int m=l+r>>1; pre(rt<<1,l,m); pre(rt<<1|1,m+1,r); } void addl(int rt,int l,int r,int L,int R,int w){ if(L<=l&&r<=R){ edg[idl[rt]].push_back(make_pair(cnt,w)); return; } int mid=(l+r)/2; if(L<=mid)addl(rt*2,l,mid,L,R,w); if(R>mid)addl(rt*2+1,mid+1,r,L,R,w); } void addr(int rt,int l,int r,int L,int R){ if(L<=l&&r<=R){ edg[cnt].push_back(make_pair(idr[rt],0)); return; } int mid=(l+r)/2; if(L<=mid)addr(rt*2,l,mid,L,R); if(R>mid)addr(rt*2+1,mid+1,r,L,R); } struct man{ int v; LL w; bool operator<(const man &e)const{ return w>e.w; } }; priority_queue<man>q; void dij(int s){ for(int i=0;i<=cnt;i++)d[i]=-1,vis[i]=0; d[s]=0; q.push(man{s,0}); while(!q.empty()){ int u=q.top().v;q.pop(); if(vis[u])continue; vis[u]=1; for(int i=0;i<edg[u].size();++i){ int v=edg[u][i].first,w=edg[u][i].second; if(!vis[v]&&(d[v]==-1||d[v]>d[u]+w)){ d[v]=d[u]+w; q.push(man{v,d[v]}); } } } } int main() { int w; scanf("%d%d%d",&n,&m,&s); cnt=n; buildl(1,1,n); buildr(1,1,n); pre(1,1,n); while(m--) { ++cnt; int op,l,r,x,y; scanf("%d",&op); if(op==1) { scanf("%d%d%d",&x,&y,&w); addl(1,1,n,x,x,w); addr(1,1,n,y,y); } else if(op==2) { scanf("%d%d%d%d",&x,&l,&r,&w); addl(1,1,n,x,x,w); addr(1,1,n,l,r); } else { scanf("%d%d%d%d",&x,&l,&r,&w); addl(1,1,n,l,r,w); addr(1,1,n,x,x); } } dij(s); for(int i=1;i<=n;i++)printf("%lld%c",d[i],i==n?' ':' '); return 0; }