1.mybatis执行流程:
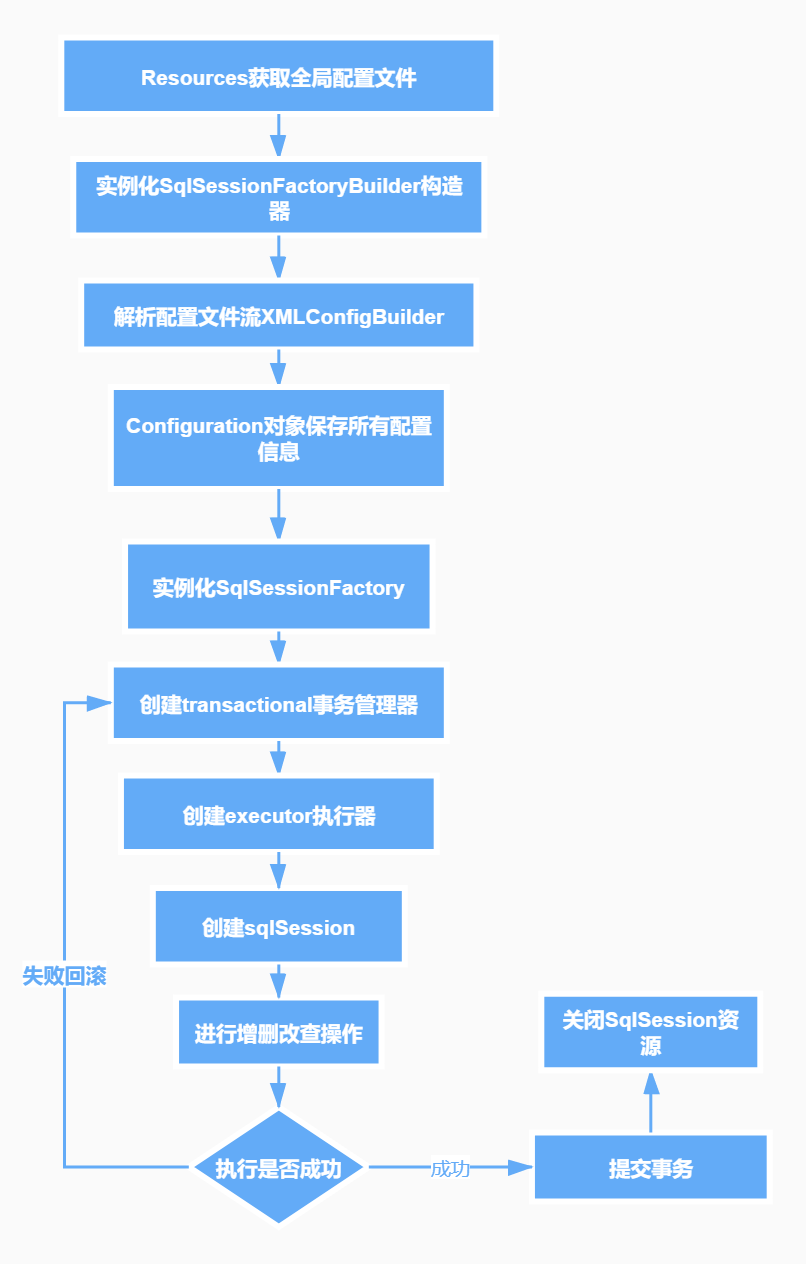
2.依赖
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.16</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.2</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.projectlombok/lombok -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.12</version>
<scope>provided</scope>
</dependency>
3. mybatis多对一查询:
示例:多个学生对应一个老师。
实体类示例:
@Data
public class Teacher {
private int id;
private String name;
}
@Data
public class Student {
private int id;
private String name;
private Teacher teacher;
}
工具类
public class MybatisUtils {
private static SqlSessionFactory sqlSessionFactory;
static {
try {
InputStream inputStream = Resources.getResourceAsStream("mybatis-config.xml");
sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
} catch (IOException e) {
e.printStackTrace();
}
}
public static SqlSession getSqlSession(){
return sqlSessionFactory.openSession();
}
}
xml示例:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.sf.dao.StudentMapper">
<!--需求:获取所有学生及对应老师的信息-->
<!--子查询-->
<select id="getStudent" resultMap="studentTeacher">
select * from student
</select>
<!--association关联属性,property属性名,column数据库列名,javaType属性类型。-->
<resultMap id="studentTeacher" type="student">
<association property="teacher" column="tid" javaType="teacher" select="getTeacher"/>
</resultMap>
<select id="getTeacher" resultType="teacher">
select * from teacher where id = #{tid}
</select>
<!--按结果嵌套查询-->
<select id="getStudent2" resultMap="studentTeacher2">
SELECT s.id sid,s.name sname,t.name tname,t.id tid
FROM student s,teacher t
WHERE s.tid = t.id
</select>
<resultMap id="studentTeacher2" type="student">
<result property="id" column="sid"/>
<result property="name" column="sname"/>
<association property="teacher" javaType="teacher">
<result property="name" column="tname"/>
<result property="id" column="tid"/>
</association>
</resultMap>
</mapper>
测试:
@Test
public void testStudent2(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
StudentMapper mapper = sqlSession.getMapper(StudentMapper.class);
List<Student> student = mapper.getStudent2();
for (Student student1 : student) {
System.out.println(student1);
}
}
4.一对多查询:
示例:一个老师拥有多个学生
实体类示例:
@Data
public class Student {
private int id;
private String name;
private int tid;
}
@Data
public class Teacher {
private int id;
private String name;
private List<Student> students;
}
xml示例
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.sf.dao.TeacherMapper">
<!--需求:查询老师信息,并查出其所拥有的学生信息-->
<!--方法一:按结果嵌套处理-->
<select id="getTeacher2" resultMap="teacherStudent">
select t.id tid,t.name tname,s.id sid,s.name sname
from teacher t,student s
where t.id = s.tid and t.id = #{tid}
</select>
<resultMap id="teacherStudent" type="teacher">
<result property="id" column="tid"/>
<result property="name" column="tname"/>
<!--使用collection标签,ofType 指映射到集合中的泛型类型-->
<collection property="students" ofType="student">
<result property="id" column="sid"/>
<result property="name" column="sname"/>
<result property="tid" column="tid"/>
</collection>
</resultMap>
<!--方法二:按查询嵌套处理-->
<select id="getTeacher3" resultMap="teacherStudent3">
select * from teacher where id = #{tid}
</select>
<resultMap id="teacherStudent3" type="teacher">
<!--javaType指实体类中属性的类型,ofType 指映射到集合中的泛型类型-->
<collection property="students" javaType="ArrayList" ofType="student" select="getStudentByTeacherId" column="id"/>
</resultMap>
<select id="getStudentByTeacherId" resultType="student">
select * from student where tid = #{tid}
</select>
</mapper>
测试:
@Test
public void testTeacher3(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
TeacherMapper mapper = sqlSession.getMapper(TeacherMapper.class);
Teacher teacher = mapper.getTeacher3(1);
System.out.println(teacher);
}
5.mybatis动态sql:
指根据不同的查询条件,生成不同的sql语句。
实体类示例
@Data
public class Blog implements Serializable {
private String id;
private String title;
private String author;
private Date createTime;
private int views;
}
xml示例
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.sf.mapper.BlogMapper">
<cache eviction="LRU"/>
<!--插入数据-->
<insert id="addBook" parameterType="Blog">
insert into blog(id,title,author,create_time,views)
values (#{id},#{title},#{author},#{createTime},#{views});
</insert>
<!--sql片段,抽取共有部分,简化代码-->
<sql id="if-title-author">
<if test="title !=null">
title =#{title}
</if>
<if test="author !=null">
and author = #{author}
</if>
</sql>
<!--使用sql片段-->
<select id="queryBlogIf" parameterType="map" resultType="blog">
select * from blog
<where>
<include refid="if-title-author"></include>
</where>
</select>
<!--foreach对集合进行遍历-->
<select id="queryBlogForeach" parameterType="list" resultType="blog">
select * from blog
<where>
id in
<foreach collection="list" item="arrayList" open="(" close=")" separator="," >
#{arrayList}
</foreach>
</where>
</select>
<!--choose类似于java中switch语句,满足一项即返回-->
<select id="queryBlogChoose" resultType="blog" parameterType="map">
select * from blog
<where>
<choose>
<when test="title !=null">
and title = #{title}
</when>
<when test="author !=null">
and author = #{author}
</when>
<otherwise>
and views = #{views}
</otherwise>
</choose>
</where>
</select>
<!--set会删掉额外的逗号,用于更新-->
<update id="updateBlog" parameterType="map">
update blog
<set>
<if test="title !=null">
title =#{title},
</if>
<if test="author!=null">
author = #{author}
</if>
</set>
where id = #{id}
</update>
</mapper>